Build a Blockchain Game using ContractKit
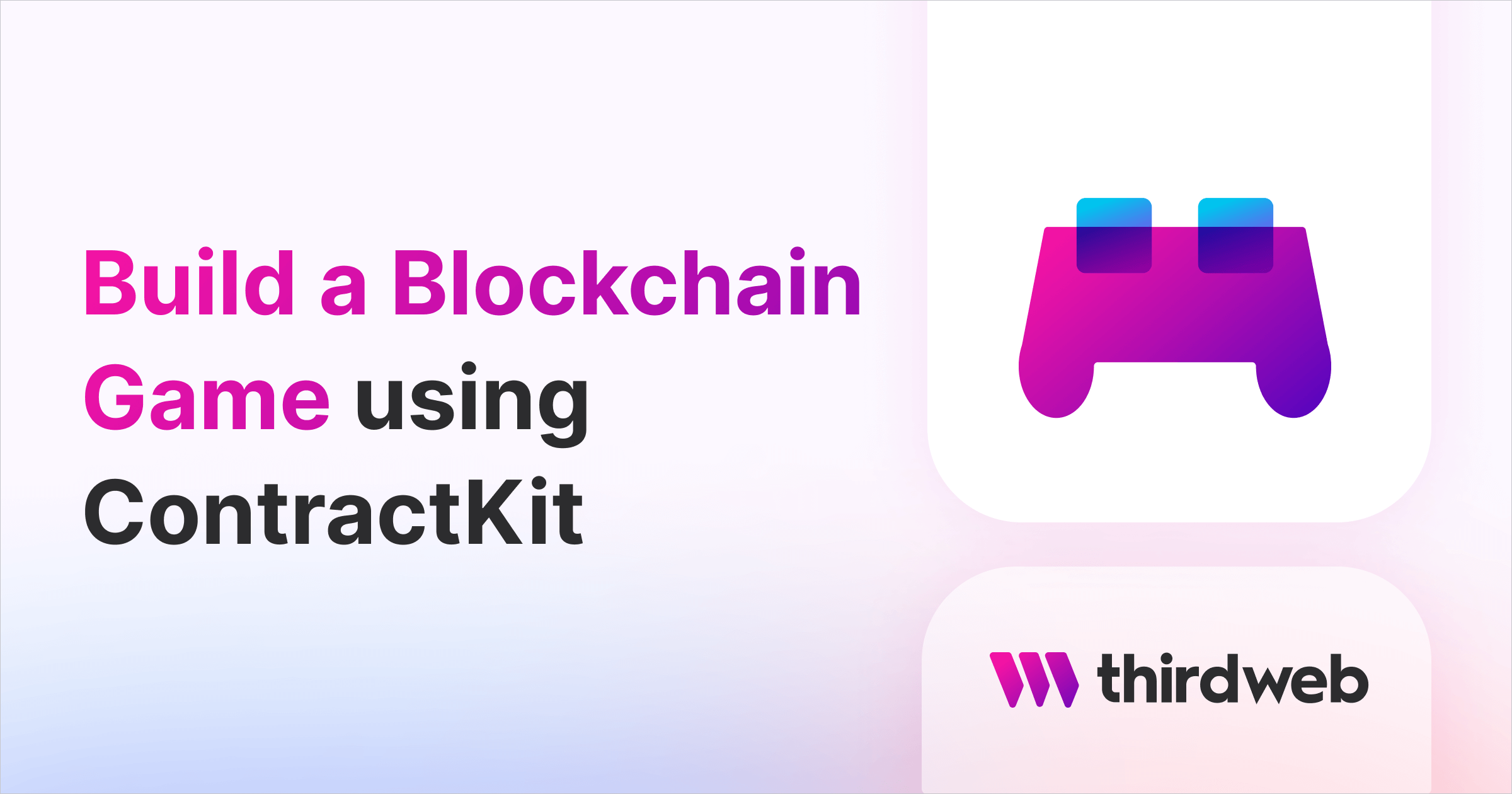
⚠️ Warning: This guide currently uses v4 of the Connect SDK. For v5 (latest) code snippets, please check out our documentation while this guide is being updated. ⚠️
In this guide, We'll show you how to build a blockchain game by customizing the ERC 1155 smart contract from thirdweb's contractKit.
Before we get started below are some helpful resources where you can learn more about the tools and resources we're going to be using in this guide.
- Contract Kits and ERC1155LazyMint Contract
- Publish and Deploy
- Complete source code
- Video walkthrough
- Live link to the game
Let's start!
Creating the Smart Contract
The game we're going to create will allow users to:
- Claim a level 1 kitten NFT
- Transfer the kitten NFT to be awarded a level 2 cat NFT!
To build the smart contract environment we will be using thirdweb's cli.
Let's create a folder for the project where the smart contract will go. To do that open your terminal and execute the following commands.
mkdir game-contract
cd game-contract
Now that the folder has been created go ahead and execute the commands below to create the smart contract.
npx thirdweb@latest create contract
It will give you a few options such as the framework you want to use and the folder name etc, Choose whatever you like and pick the ERC1155 + Lazy Mint
contract in the contracts section.
- The picture below shall clarify the process if you had any difficulties.
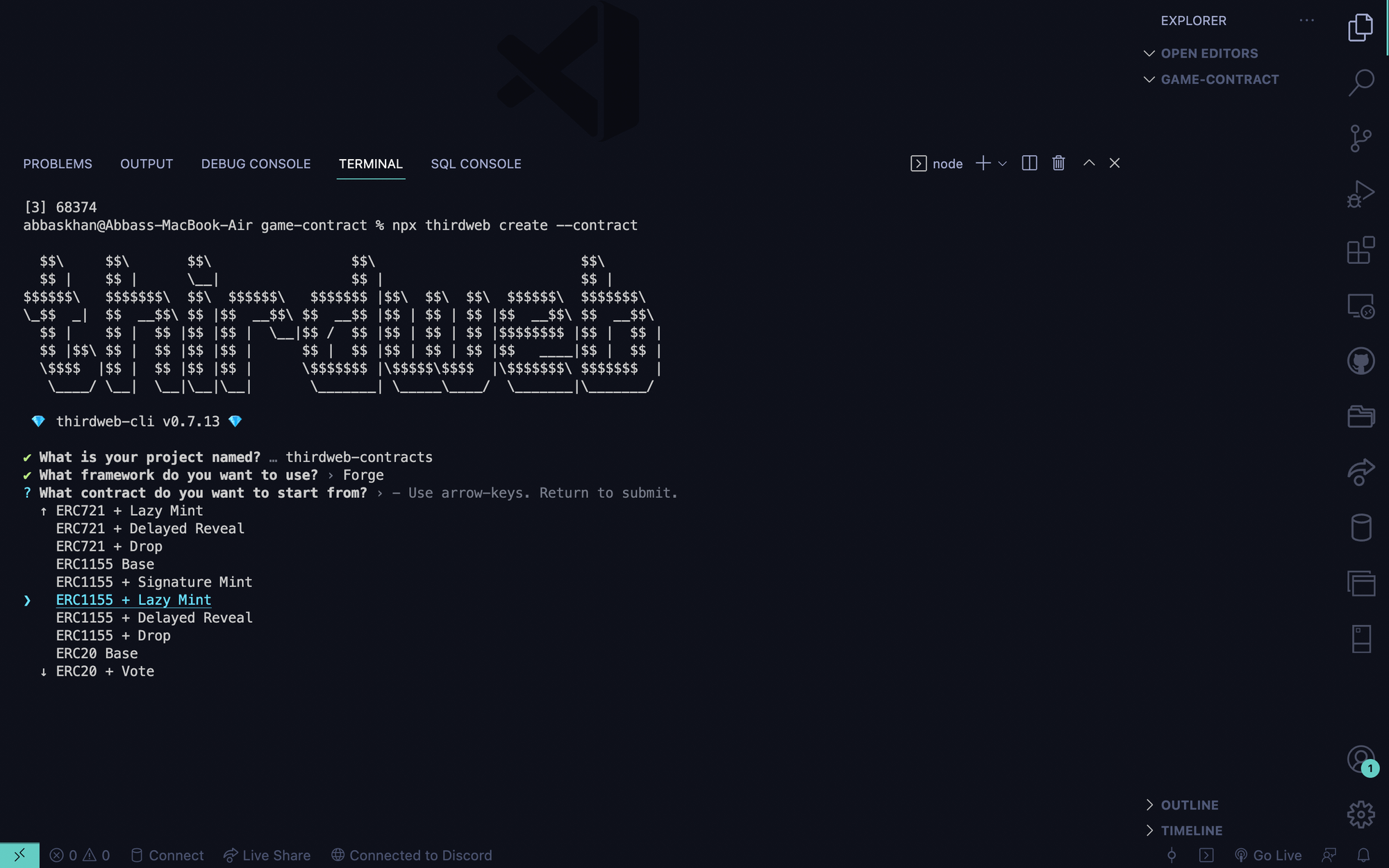
Now you have a project ready to go!
Building the contract
Initially, we will import and inherit the ERC1155LazyMint
contract, Since we want to use LazyMint to create our cats and prepare them for other players to start claiming the NFTs as well.
Let's go ahead and add logic to our smart contract in the Contract.sol
file and pass in the necessary parameters required by the base contract.
// SPDX-License-Identifier: Apache-2.0
pragma solidity ^0.8.0;
import "@thirdweb-dev/contracts/base/ERC1155LazyMint.sol";
// EXTENDING THE BASE CONTRACT
contract CatAttackNFT is ERC1155LazyMint {
// The ERC1155 contract requires these parameters so we will just keep the name and symbol for the deployer to set.
//and set up the royaltyRecipient to the deployer and royaltyBps to 0
constructor(
string memory _name,
string memory _symbol
)
ERC1155LazyMint(
_name,
_symbol,
msg.sender, // royaltyRecipient set to the deployer here
0 // royalty amount to 0
)
{}
}
Adding custom function
Next, we will create an event named LevelUp
which will help us keep track of the level of a specific NFT.
We'll also add a function called claimKitten
that will allow the user to claim a level 1 NFT; which will then be used to transfer to another address to level up.
function claimKitten() external {
// The claim function requires a reciever address which will be the deployer again
// and then we will set the tokenId as 0 since it will be a kitten and the amount will be 1
claim(msg.sender, 0, 1)
// claiming a kitten will enter the game at level 1
emit LevelUp(msg.sender, 1)
}
Overriding the transfer function
Here, we're overriding a function from the base contract called safeTransferFrom
instead of writing our own function.
This allows us to customize what happens whenever a user transfers an NFT.
Let's override this function so that transferring our level 1 NFTs (AKA kittens) to another address results in being minted a level 2 cat
NFT!
function safeTransferFrom(
address from,
address to,
uint256 id,
uint256 amount,
bytes memory data
)
// override keyword since this function is being overridden
public override gameNotPaused {
// can only transfer kittens
require(id == 0, "This cat is not transferable!");
// Also the super keyword in Solidity gives access to the immediate parent contract
// from which the current contract is derived. In our case it would be the ERC1155LazyMint contract
super.safeTransferFrom(from, to, id, amount, data);
if(from != to && id == 0) {
// transfering level 1 NFT gives you a level 2 NFT
_mint(from, 1, 1, "");
emit LevelUp(to, 1); // receiver levels up to 1
emit LevelUp(from, 2); // sender levels up to 2
}
}
Alrightyyyyy! It's time to deploy our smart contract! All you need to do is to execute the command below:
npx thirdweb deploy
This will take you to the dashboard where you can populate the parameters of your smart contract constructor.
Click here to deploy the smart contract from this guide
After the contract is deployed, in the NFTs
section, go ahead and batch upload a CSV file similar to the file below, (or feel free to upload this file):
Conclusion
We were able to customize the base contract and implement logic the way we wanted. We were also able to understand how ERC 1155 token standard can be used for upgradeable NFTs and how thirdweb's prebuilt contracts can be very useful in achieving this goal.
If you have any questions regarding this guide feel free to reach out to us on discord, If you want to build a front around this feel free to check out the GitHub repository.