What is an NFT Smart Contract?
NFT smart contracts are digital agreements that define ownership records, transfer protocols, royalties and metadata for non-fungible tokens.
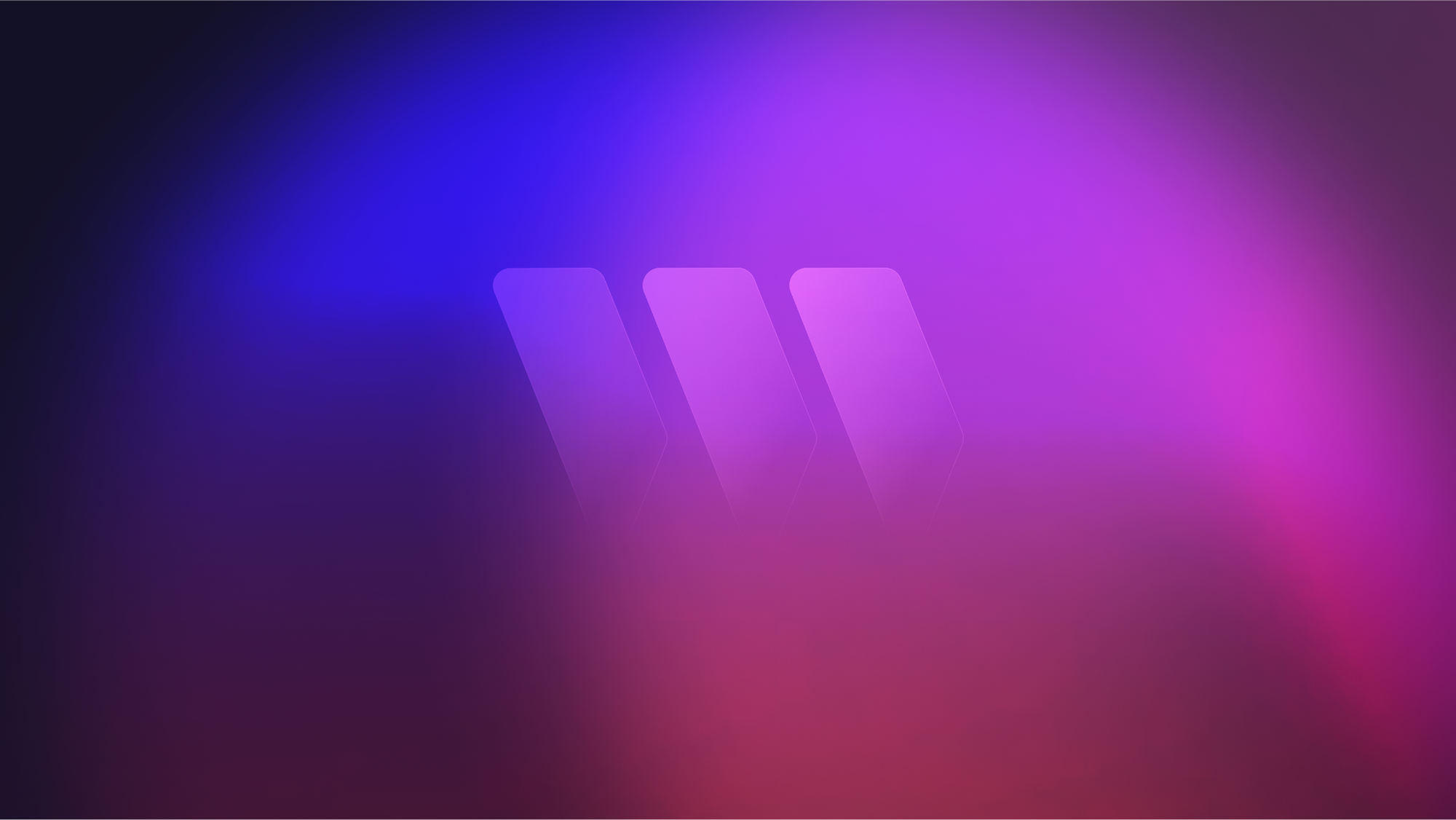
Non-fungible tokens (NFTs) are digital assets with verifiable ownership on a blockchain. The term "non-fungible" means each token is unique and can’t be directly swapped with another because they don’t share the same attributes or value. It’s like comparing two pieces of art—each one is different, and its value depends on the artist and how special the piece is.
At their core, NFTs are made possible by smart contracts. These are self-executing agreements on a decentralized network, which makes them secure, tamper-proof, and publicly accessible. There are multiple types of smart contracts, but NFT smart contracts are some of the most widely used. Imagine you create a digital piece of art that can be copied endlessly. An NFT smart contract adds a verifiable stamp of authenticity and an ownership record that anyone can check.
This article provides a comprehensive guide to NFT smart contracts, focusing on the EVM ecosystem, including how they work, their token standards, deployment methods, features, and potential.
What is an NFT Smart Contract?
NFT smart contracts are digital agreements that define ownership records, transfer protocols, royalties and metadata for non-fungible tokens. Most smart contracts on Ethereum are written in Solidity and operate on EVM-compatible blockchains like Ethereum or Base.
NFT smart contracts establish rules that track ownership and ensure each asset is unique and non-replicable. And some NFT smart contracts can also define different rules, functionalities. Let’s take a look at the most common types of smart contracts you'll find powering NFTs.
NFT Token Standards: Types of Smart Contracts Used
ERC-721
The ERC-721 standard is the basis for most NFTs, ensuring that each token is unique, with its own distinct ownership and metadata. Introduced in the EIP-721 in 2018, this standard is commonly used for digital collectibles, art, and in-game assets. It includes some key features:
- One token, one owner: Each token is owned by a single individual or entity at any given time.
- Core functions: Provides functions like
ownerOf
(to check ownership) andtokenURI
(to access metadata). - Transfer approvals: Enables token owners to approve others to transfer their tokens.
ERC-721A
The ERC-721A standard is a gas-efficient implementation of ERC-721, designed to significantly reduce costs when minting multiple NFTs in a single transaction. It has become a popular choice for projects focused on improving user experience and minimizing gas fees during high-demand drops.
Key features of ERC-721A:
- Batch minting: Mint multiple NFTs for nearly the same gas cost as a single NFT.
- Optimized storage: Reduces redundant storage updates for better efficiency.
- Backward compatibility: Fully compatible with existing ERC-721 infrastructure.
ERC-721C
The ERC-721C standard enables enforceable on-chain royalties and customizable transfer policies, allowing creators to manage how their NFTs are traded while remaining backward compatible with ERC-721.
Key features of ERC-721C:
- Enforceable royalties: Ensures creators receive royalties through transfer policies.
- Custom transfer rules: Allows whitelisting of specific platforms and addresses.
- Combat wash trading: Helps reduce manipulative trading practices in NFT markets.
ERC-1155
The ERC-1155 standard is a multi-token framework that allows a single smart contract to manage various token types, including fungible, non-fungible, and semi-fungible tokens. It is widely used for games, collectibles, and platforms needing efficient token management.
Key features of ERC-1155:
- Batch transfers: Transfer multiple token types in a single transaction, reducing gas costs.
- Flexible design: Combines fungible and non-fungible tokens within the same contract.
- Efficient storage: Uses a single contract to represent numerous token types, optimizing blockchain usage.
Each token standard addresses specific pain points and improves upon previous implementations. Introducing standardization simplifies future development of these smart contracts, but also shortens build times and minimizes the potential of vulnerabilities.
Importantly though, standardization doesn't mean limitation: developers can also use these contracts as a base and add custom functionalities. Let’s explore some potential features for NFT smart contracts.
Potential Features of NFT Smart Contracts
Royalties
Royalties in NFT smart contracts ensure creators earn a percentage of secondary sales. This is particularly useful for artists and content creators who want consistent revenue from their work. Certain standards even enforce royalties across marketplaces like ERC721-C, and deploying them is pretty easy with the correct guide.
Dynamic Metadata
Allows NFTs to change attributes over time, often based on user interactions or external events.
Fractional Ownership
Some NFT smart contracts also allow you to divide NFTs into smaller, tradable fractions, enabling a wider audience to share ownership of a single asset without losing agency over their portion.
Unlockable Content
Unlockable content provides hidden perks, such as files or access to exclusive experiences, available only to the NFT’s owner, like adding the ability to access certain virtual spaces, private meet and greets and more. These are just a few examples of potential uses and features, but the possibilities are only limited by the developer’s imagination.
Using thirdweb Contracts, you can unlock all of these features and more by deploying modular contracts!
So, how can these features be applied to an NFT smart contract? Most NFTs are written in Solidity, so let’s dive into the foundational functionalities of an NFT implemented in this language.
How Does an NFT Smart Contract Work?
The base EIP-721 proposal outlines the essential functionalities a smart contract must include to be considered an NFT. Let’s explore the most common functions.
Common Functions and Calls in NFT Smart Contracts
Token Metadata: Stores and retrieves the NFT’s unique attributes.
solidity
Copy code
function tokenURI(uint256 tokenId) public view override returns (string memory) {
return metadata[tokenId];
}
Transfer: Enables secure transfers of NFTs between wallets.
function transferFrom(address from, address to, uint256 tokenId) public {
_transfer(from, to, tokenId);
}
Minting: Creates a new NFT and assigns ownership to a specific wallet.
function mint(address to, uint256 tokenId) public {
_safeMint(to, tokenId);
}
Metadata and IPFS Integration
A bit of history: when the first NFTs were deployed to Ethereum mainnet, developers quickly realized that storing all asset metadata onchain was prohibitively expensive, resulting in transactions with excessively high gas fees.
To address this, developers chose not to store asset metadata directly onchain. Instead, they introduced a reference system using TokenURI
—a link that points to the metadata.
These metadata files are often hosted using decentralized storage solutions like IPFS (InterPlanetary File System), which ensures both accessibility and decentralization. Another popular option is Arweave, which provides decentralized hosting and permanent storage for files and databases.
Metadata can also be stored directly on-chain by encoding assets like images as strings. This approach ensures immutability, preventing issues like broken links that can occur with off-chain solutions. It's particularly valuable if you need to manage collection attributes on-chain, such as for a Web3 game. However, this method significantly increases gas costs, so it’s important to get the right balance between on-chain and off-chain data depending on your project’s priorities.
Example of metadata JSON:
json
Copy code
{
"name": "MyNFT",
"description": "A unique NFT",
"image": "ipfs://QmExampleHash"
}
The URI is stored within the smart contract and accessed via calls like tokenURI
.
How to Create and Deploy an NFT Drop Contract with thirdweb
thirdweb provides a seamless way to create and deploy NFT smart contracts without requiring extensive coding expertise. Here’s a step-by-step guide to launching an NFT drop contract:
Step 1: Sign up and sign in
Install a web3 wallet and connect it to your preferred blockchain network, such as Ethereum or Polygon, create a thirdweb account and connect your wallet.
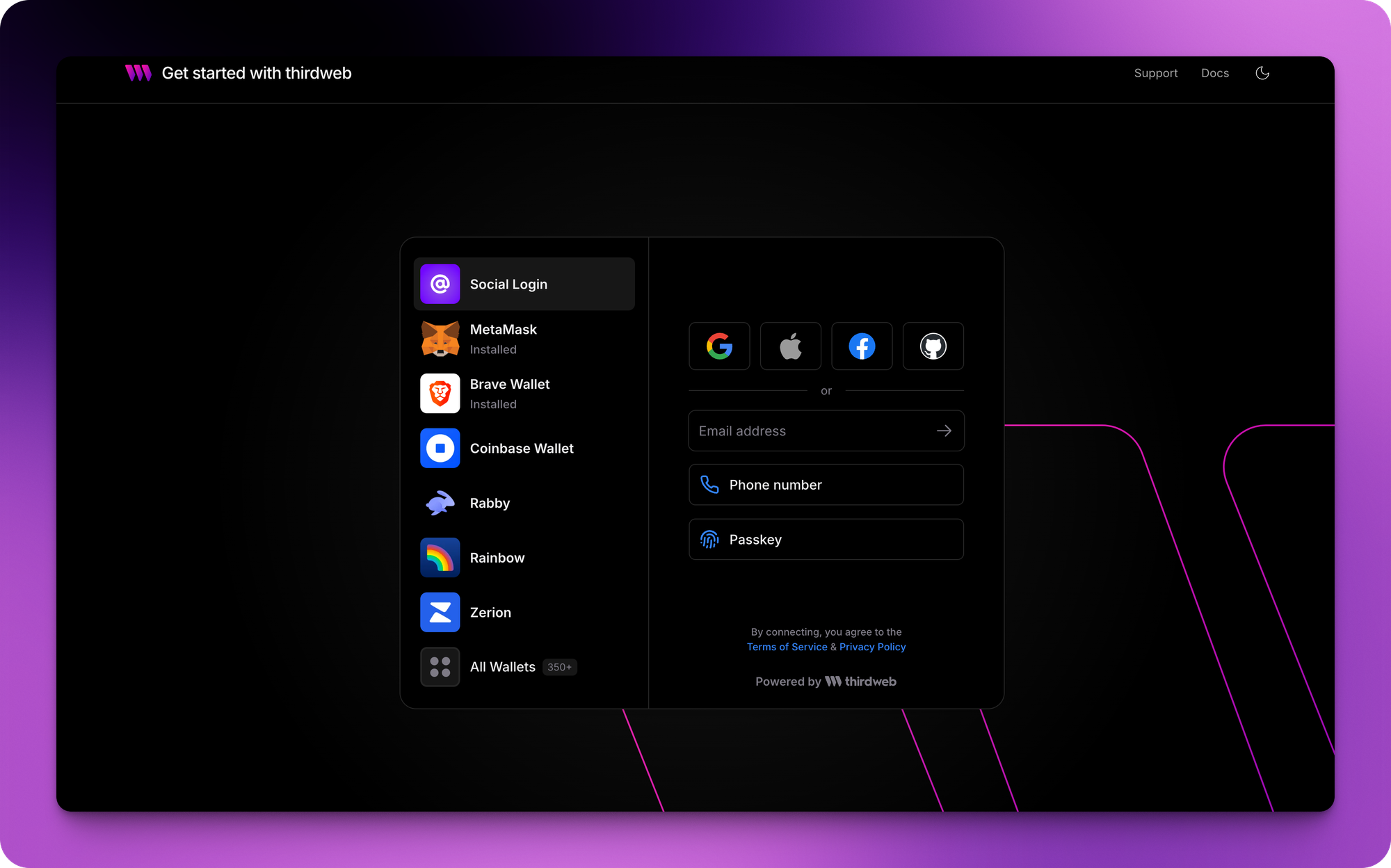
Step 2: Access thirdweb’s Dashboard
Visit thirdweb Dashboard, navigate to the Contracts sub-menu and hit "Deploy contract".
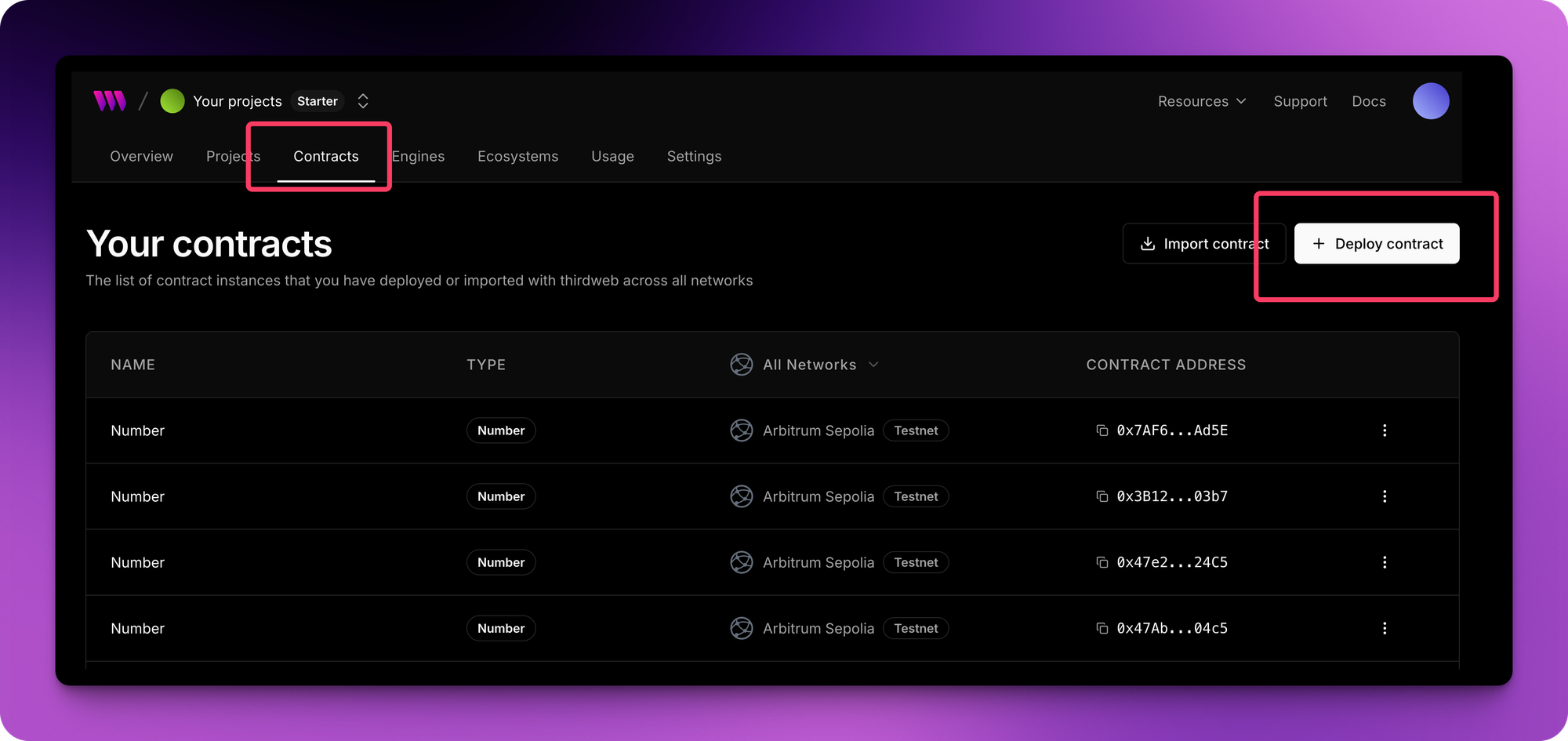
Step 3: Select a NFT contract from the list
Via thirdweb Explore, you have a range of NFT smart contact templates to choose from. For this example we are going to select select the NFTDrop 721 contract template.
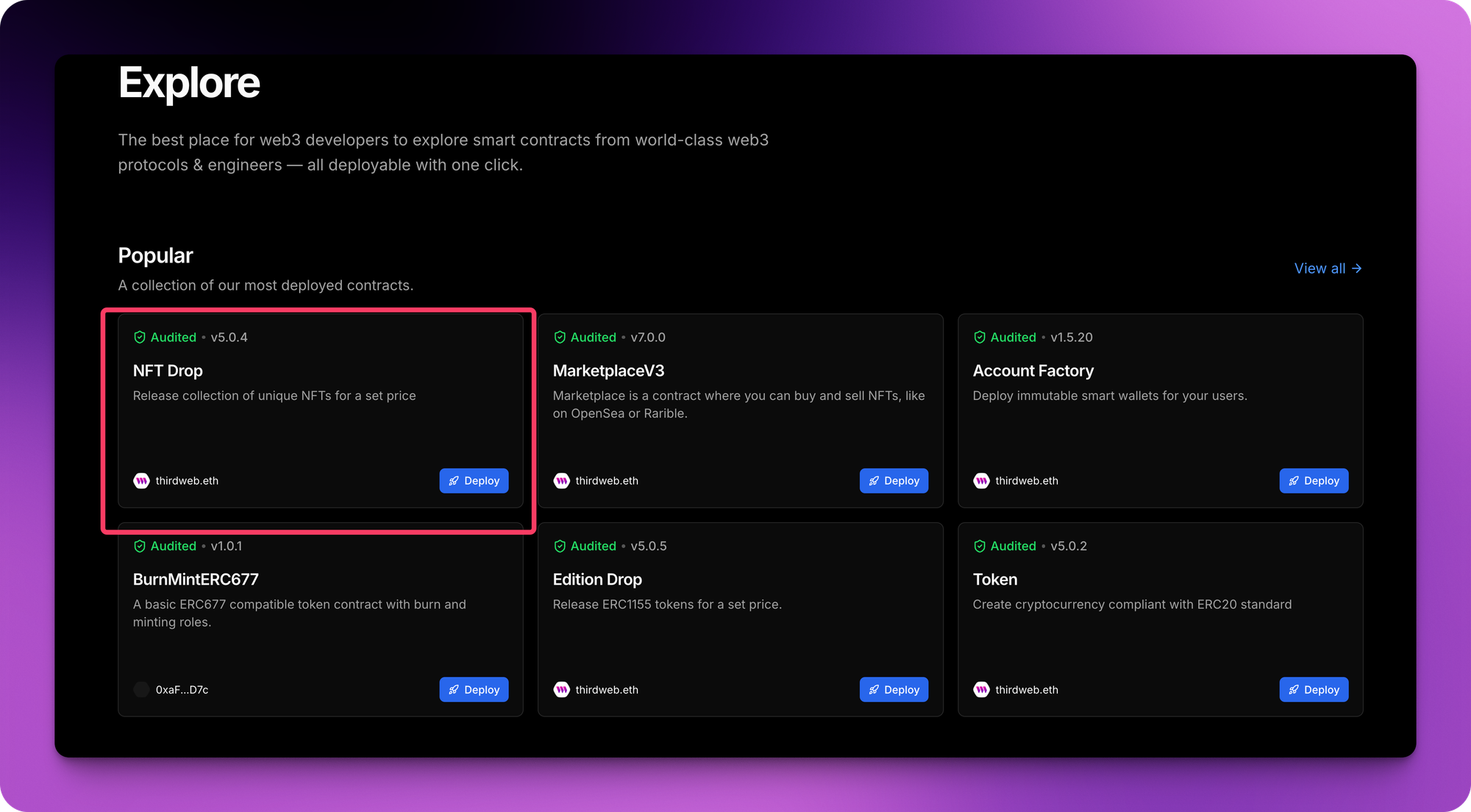
Step 4: Configure Your Contract
Name your collection and define details like the token symbol, max supply, royalty fees and recipient wallet addresses.
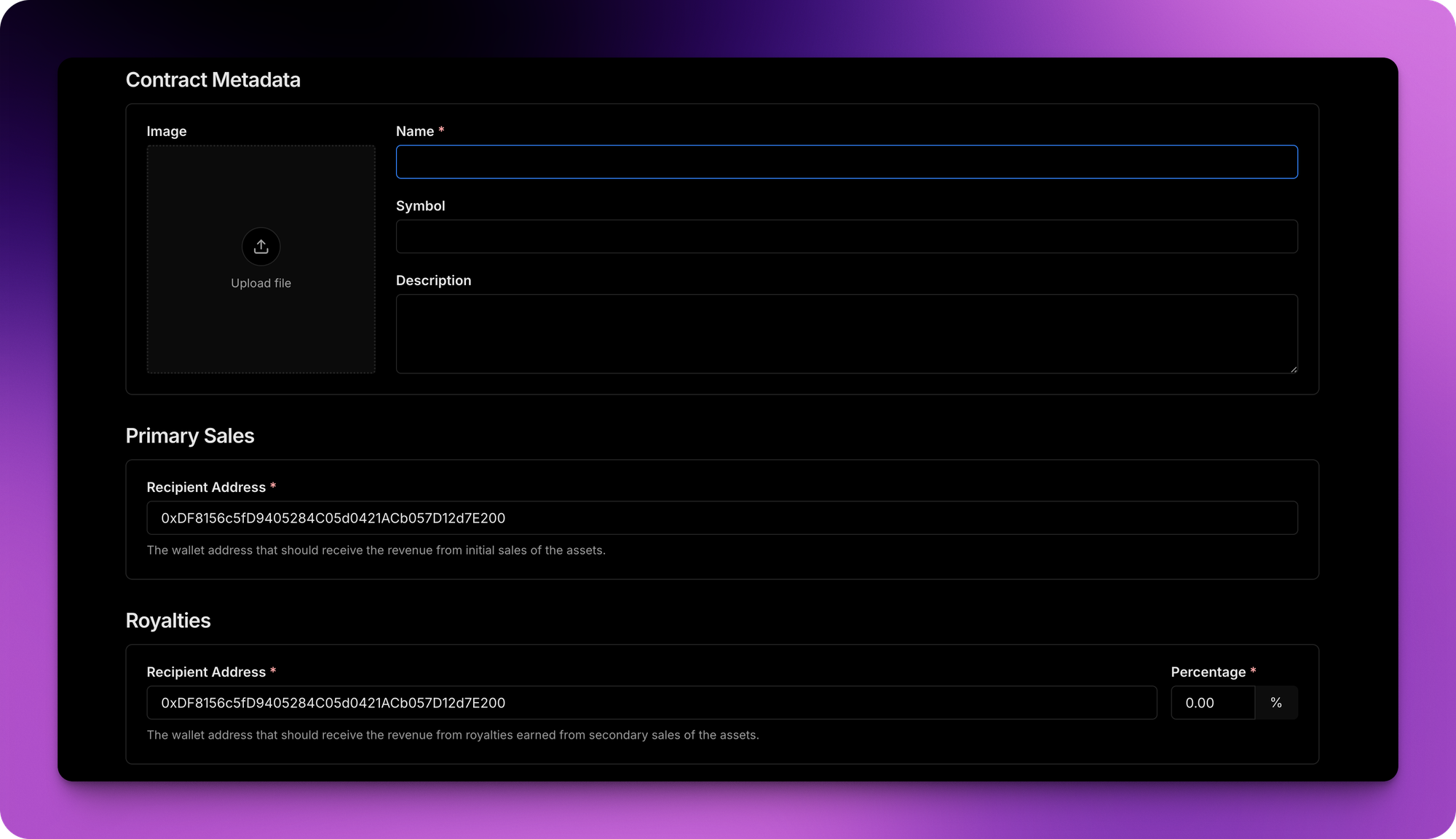
Step 5: Upload Metadata
Prepare metadata files (JSON) for your NFTs and upload assets to IPFS using thirdweb’s integrated tools.
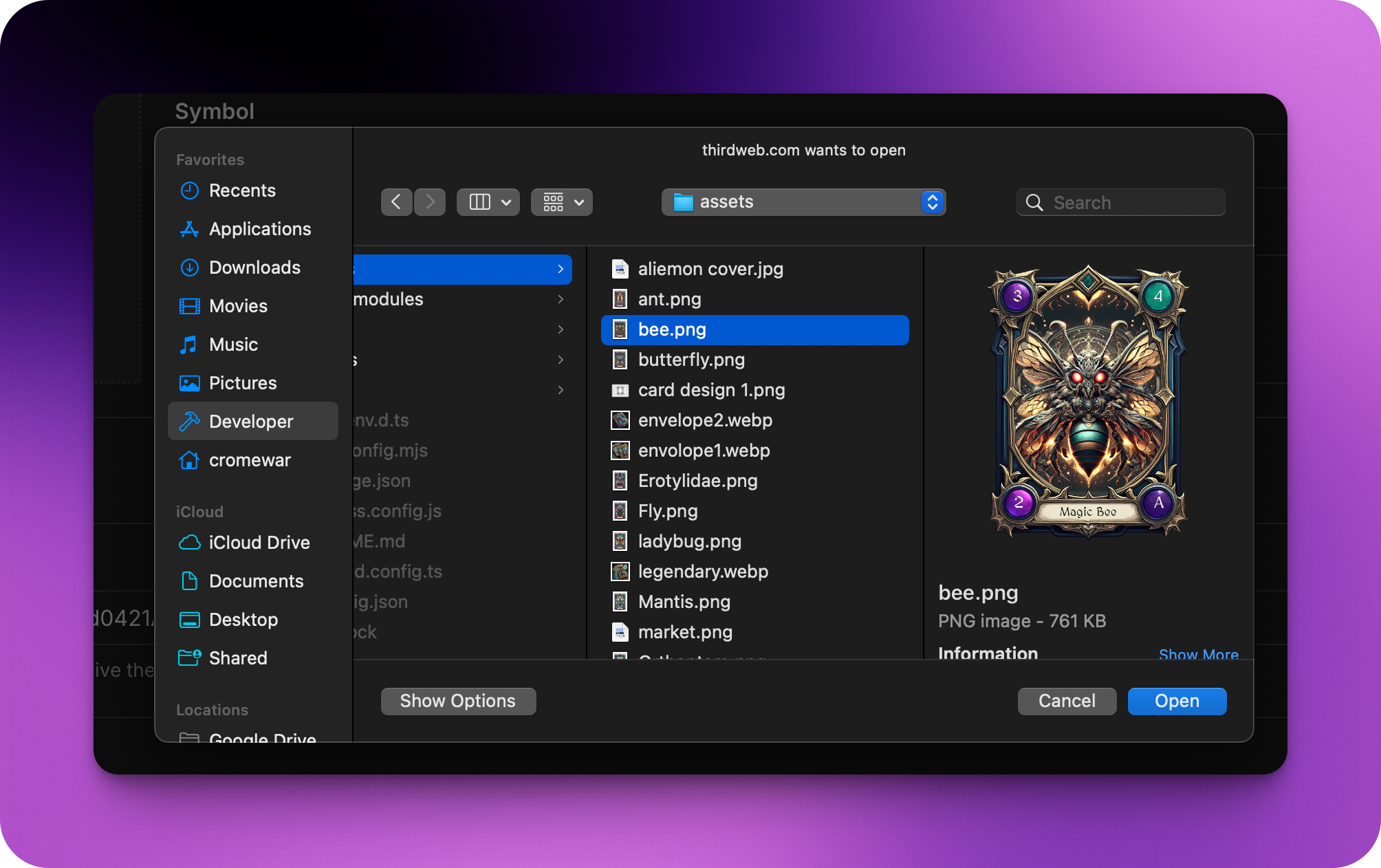
Step 6: Deploy your NFT smart contract to Blockchain
Select your desired blockchain network and confirm deployment in your web3 wallet.
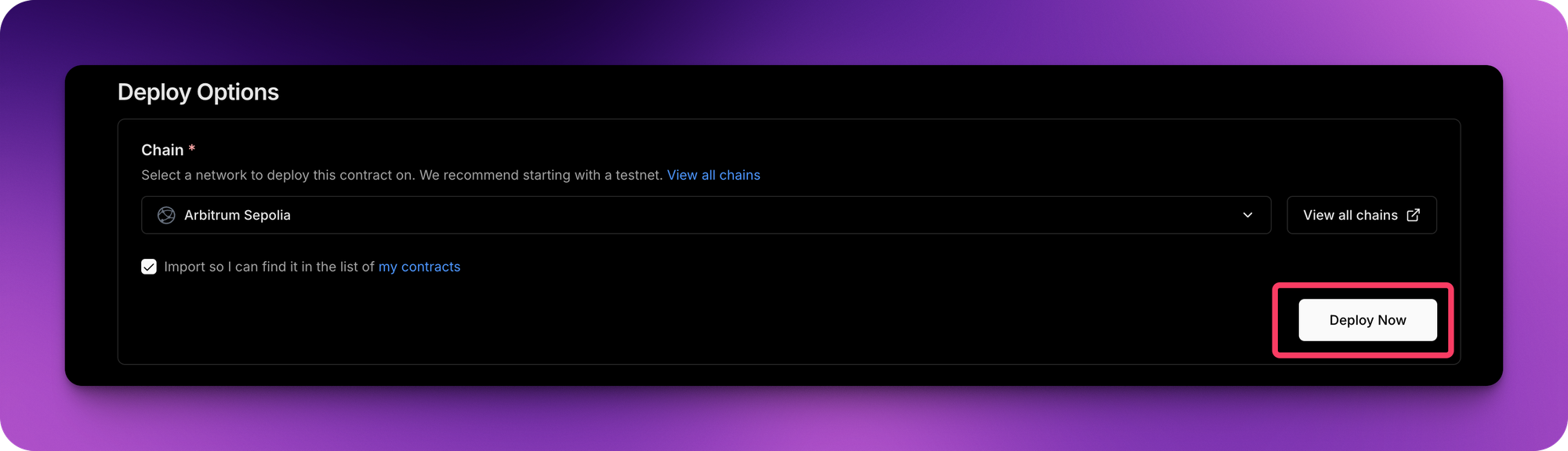
Step 7: Manage Minting and Drops
Use the thirdweb dashboard to schedule drops or mint NFTs directly.
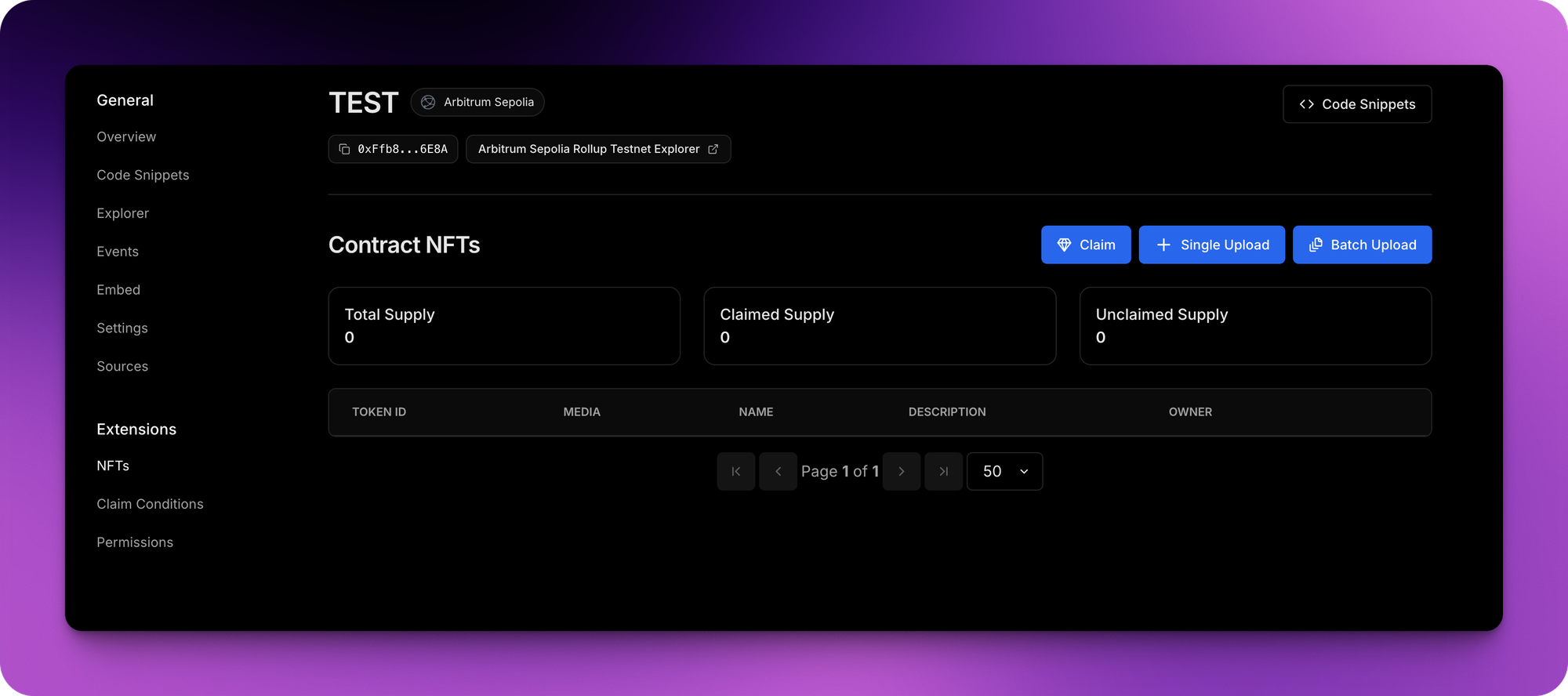
Simply focus on your vision; thirdweb handles the technical complexities.
NFT Smart Contracts: The Potential
NFTs go beyond creating digital collectibles with verifiable ownership—they are a framework for innovation. While they’ve primarily been used for digital art collections and in-game assets, their potential applications are much broader. With the tokenization of real-world assets, NFTs could streamline processes requiring ownership verification. Imagine using an NFT as a secure, tamper-proof digital deed for your house, a verifiable ticket for a flight, or even proof of ownership for rare physical items. As technology evolves, NFTs have the potential to become integral to systems that demand transparency, security, and trust.