How to Create Pre-Generated Wallets for Web3 Apps
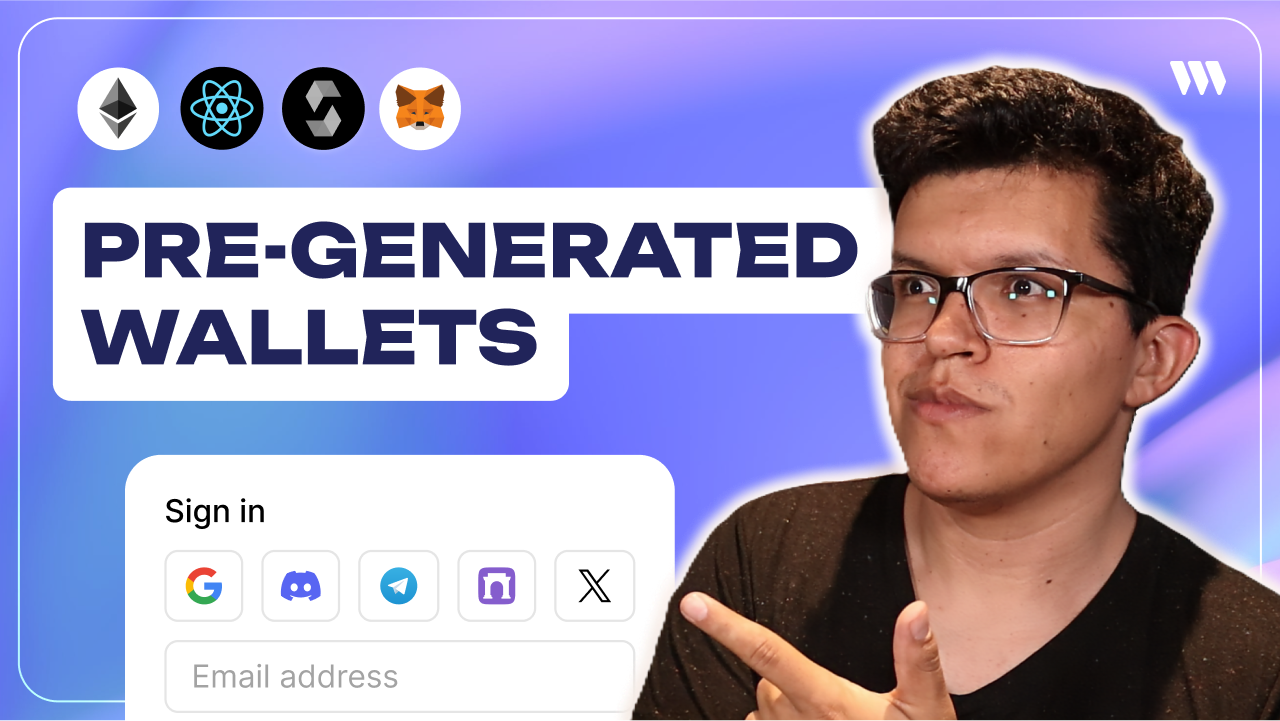
One of the biggest barriers to adoption in crypto is wallet management. Many users get confused or intimidated by the process of creating wallets and managing private keys. This is where pre-generated wallets come in—they allow you to create wallets for your users before they even log into your application.
Today, I'll show you how to build a newsletter application that automatically creates a wallet for users when they submit their email addresses and sends them an exclusive NFT as a reward. When they later sign in with that same email, the wallet will be automatically connected to their account, with their NFT already waiting for them.
Follow along the video guide here:
What Are Pre-Generated Wallets?
Pre-generated wallets let you create wallets on behalf of your users, linked to identifiers like email addresses or social media accounts. This approach lets you:
- Send assets to users before they log in
- Remove barriers for non-crypto-native users
- Provide rewards or in-game items automatically
- Connect wallets directly to users' existing accounts
Building Our Newsletter Application
We'll create a simple application that:
- Lets users sign up with just an email
- Automatically creates a wallet for them
- Sends them an exclusive NFT as a reward
- Shows them their NFT when they log in
Let's get started!
Step 1: Create a New Project
First, let's create a new project using the thirdweb CLI:
npx thirdweb@latest create app
Select the Next.js template and name your project (I'm using "pregenerated-wallets").
Step 2: Creating the Pre-Generated Wallet API
Let's create a server that will handle wallet creation. First, create a new folder called "scripts" in your project root, and add a file called generate.mjs
:
import fetch from 'node-fetch';
import express from 'express';
import cors from 'cors';
import { createThirdwebClient, getContract, sendTransaction } from 'thirdweb';
import { privateKeyToAccount, sendTransferFrom } from 'thirdweb';
import dotenv from 'dotenv';
dotenv.config();
const app = express();
const PORT = 3002;
// CORS settings for local development
app.use(cors({
origin: 'http://localhost:3000',
methods: ['GET', 'POST'],
credentials: true
}));
// Middleware for parsing JSON
app.use(express.json());
// Endpoint for newsletter subscription
app.post('/subscribe', async (req, res) => {
const email = req.body.email;
// Check if email exists
if (!email) {
return res.status(400).json({ message: "Email is required" });
}
// Check for private key and API key
const privateKey = process.env.PRIVATE_KEY;
const thirdwebApiKey = process.env.THIRDWEB_API_KEY;
if (!privateKey || !thirdwebApiKey) {
return res.status(500).json({ message: "Server configuration error" });
}
const URL = 'https://api.thirdweb.com/v1/wallet';
const headers = {
'x-secret-key': thirdwebApiKey,
'Content-Type': 'application/json'
};
const body = JSON.stringify({
strategy: 'email',
email: email
});
try {
// Create wallet
const response = await fetch(URL, {
method: 'POST',
headers,
body
});
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
const data = await response.json();
console.log(data);
// Send NFT to the new wallet
try {
const NFT_CONTRACT_ADDRESS = "YOUR_NFT_CONTRACT_ADDRESS";
const chain = "arbitrum-sepolia";
const client = createThirdwebClient({
privateKey: process.env.PRIVATE_KEY,
chain
});
const account = privateKeyToAccount(process.env.PRIVATE_KEY, client);
const nftContract = getContract({
address: NFT_CONTRACT_ADDRESS,
client,
chain
});
const transaction = sendTransferFrom({
contract: nftContract,
from: account,
to: data.address,
tokenId: 0n,
amount: 1n,
data: ""
});
const txData = await sendTransaction(transaction, account);
console.log("Transaction Hash:", txData.transactionHash);
} catch (error) {
console.error("Error sending NFT:", error);
}
res.status(200).json({ message: "Successfully subscribed" });
} catch (error) {
console.error("Error:", error);
res.status(500).json({ message: "Subscription failed, please try again" });
}
});
// Start server
app.listen(PORT, () => {
console.log(`Server running on port ${PORT}`);
});
Remember to install the required dependencies:
npm install node-fetch cors express thirdweb dotenv
Step 3: Setting Up Environment Variables
Create a .env
file in your project root and add:
THIRDWEB_API_KEY=your_thirdweb_api_key
PRIVATE_KEY=your_wallet_private_key
Make sure to use a test wallet for development purposes only. Never store production private keys in code or environment files.
Step 4: Creating the NFT Contract
Before we create the frontend, we need to deploy an NFT contract:
- Go to the thirdweb dashboard (https://thirdweb.com/dashboard)
- Click "Deploy Contract"
- Select "Edition NFT" (ERC-1155)
- Fill in the details:
- Name: "Newsletter NFT Reward"
- Symbol: (any symbol)
- Description: "An NFT reward for subscribing to the newsletter"
- Add an image
- Deploy to Arbitrum Sepolia testnet
- Mint an NFT:
- Name: "Newsletter Exclusive NFT"
- Description: "Exclusive reward for newsletter subscribers"
- Initial supply: 100,000
- Click "Mint"
- Copy the contract address - you'll need to add it to your server code
Step 5: Building the Frontend
Now let's create the frontend components:
First, set up your client ID. Find your Client ID in your thirdweb dashboard and add it to your .env.local
file:
NEXT_PUBLIC_THIRDWEB_CLIENT_ID=your_client_id
Create the Navbar Component
Create a file at components/navbar.tsx
:
'use client';
import { ConnectButton, embeddedWallet } from '@thirdweb-dev/react';
import Link from 'next/link';
import Image from 'next/image';
export default function Navbar() {
const wallets = [
embeddedWallet({
auth: {
options: ['email']
}
})
];
return (
<nav className="bg-white shadow-md">
<div className="max-w-7xl mx-auto px-4 sm:px-6 lg:px-8">
<div className="flex justify-between h-16">
<div className="flex">
<Link href="/" className="flex items-center">
<Image
src="/img/wordmark-dark.png"
alt="Logo"
width={180}
height={50}
/>
</Link>
</div>
<div className="flex items-center">
<Link href="/profile" className="mr-4 text-gray-700 hover:text-gray-900">
Profile
</Link>
<ConnectButton
theme={"light"}
modalSize={"wide"}
modalTitle={"Sign in to Newsletter"}
welcomeScreen={{
title: "Welcome to our Newsletter",
subtitle: "Sign in to claim your exclusive NFT"
}}
modalTitleIconUrl={null}
switchToActiveChain={true}
wallets={wallets}
/>
</div>
</div>
</div>
</nav>
);
}
Create the Footer Component
Create a file at components/footer.tsx
:
export default function Footer() {
return (
<footer className="bg-gray-100 mt-auto">
<div className="max-w-7xl mx-auto py-6 px-4 sm:px-6 lg:px-8">
<div className="flex justify-between items-center">
<div>
<p className="text-gray-500 text-sm">© 2025 Newsletter App</p>
</div>
<div className="flex space-x-6">
<a href="#" className="text-gray-500 hover:text-gray-900">
Terms
</a>
<a href="#" className="text-gray-500 hover:text-gray-900">
Privacy
</a>
<a href="#" className="text-gray-500 hover:text-gray-900">
Contact
</a>
</div>
</div>
</div>
</footer>
);
}
Create the Hero Component
Create a file at app/hero.tsx
:
'use client';
import { useState } from 'react';
import { Inter } from 'next/font/google';
const inter = Inter({ subsets: ['latin'] });
export default function Hero() {
const [email, setEmail] = useState('');
const [status, setStatus] = useState('');
const handleSubmit = async (e) => {
e.preventDefault();
try {
const response = await fetch('http://localhost:3002/subscribe', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ email })
});
if (response.ok) {
setStatus('Successfully subscribed!');
setEmail('');
} else {
setStatus('Subscription failed. Please try again.');
}
} catch (error) {
console.error('Error:', error);
setStatus('Subscription failed. Please try again.');
}
};
return (
<div className="bg-white">
<div className="max-w-7xl mx-auto py-16 px-4 sm:py-24 sm:px-6 lg:px-8">
<div className="text-center">
<h1 className="text-4xl font-extrabold tracking-tight text-gray-900 sm:text-5xl md:text-6xl">
<span className="block">Welcome to our</span>
<span className="block text-indigo-600">Newsletter</span>
</h1>
<p className="mt-3 max-w-md mx-auto text-base text-gray-500 sm:text-lg md:mt-5 md:text-xl md:max-w-3xl">
Join for free and receive an exclusive NFT!
</p>
<div className="mt-5 max-w-md mx-auto sm:flex sm:justify-center md:mt-8">
<div className="mt-3 rounded-md shadow sm:mt-0 sm:ml-3 w-full">
<form onSubmit={handleSubmit} className="sm:flex">
<input
type="email"
name="email"
id="email"
className="py-3 px-4 block w-full rounded-md border border-gray-300 shadow-sm focus:ring-indigo-500 focus:border-indigo-500 sm:text-sm"
placeholder="Enter your email"
value={email}
onChange={(e) => setEmail(e.target.value)}
required
/>
<button
type="submit"
className="mt-3 w-full px-6 py-3 border border-transparent text-base font-medium rounded-md text-white bg-indigo-600 shadow-sm hover:bg-indigo-700 focus:outline-none focus:ring-2 focus:ring-offset-2 focus:ring-indigo-500 sm:mt-0 sm:ml-3 sm:flex-shrink-0 sm:inline-flex sm:items-center sm:w-auto"
>
Subscribe
</button>
</form>
</div>
</div>
{status && (
<p className={`mt-3 ${status.includes('failed') ? 'text-red-500' : 'text-green-500'}`}>
{status}
</p>
)}
</div>
</div>
</div>
);
}
Update the Main Page
Update your app/page.tsx
:
'use client';
import { Inter } from 'next/font/google';
import Hero from './hero';
const inter = Inter({ subsets: ['latin'] });
export default function Home() {
return (
<main className={`flex min-h-screen flex-col ${inter.className}`}>
<Hero />
</main>
);
}
Update the Layout
Update your app/layout.tsx
:
import './globals.css';
import { ThirdwebProvider } from '@thirdweb-dev/react';
import Navbar from '../components/navbar';
import Footer from '../components/footer';
export default function RootLayout({
children,
}: {
children: React.ReactNode;
}) {
return (
<html lang="en">
<body>
<ThirdwebProvider
clientId={process.env.NEXT_PUBLIC_THIRDWEB_CLIENT_ID}
activeChain="arbitrum-sepolia"
>
<div className="flex flex-col min-h-screen">
<Navbar />
<div className="flex-grow">
{children}
</div>
<Footer />
</div>
</ThirdwebProvider>
</body>
</html>
);
}
Step 6: Creating the Profile Page
Lastly, create a profile page to display the user's NFTs at app/profile/page.tsx
:
'use client';
import { useEffect, useState } from 'react';
import { useActiveWallet, getContract } from '@thirdweb-dev/react';
import Image from 'next/image';
export default function Profile() {
const [nfts, setNfts] = useState([]);
const [loading, setLoading] = useState(true);
const wallet = useActiveWallet();
useEffect(() => {
const fetchNFTs = async () => {
if (!wallet) {
setLoading(false);
return;
}
try {
const NFT_CONTRACT_ADDRESS = "YOUR_NFT_CONTRACT_ADDRESS";
const nftContract = await getContract(NFT_CONTRACT_ADDRESS);
const walletAddress = await wallet.getAddress();
// Get NFTs for the wallet
const ownedNFTs = await nftContract.erc1155.getOwned(walletAddress);
setNfts(ownedNFTs);
} catch (error) {
console.error("Error fetching NFTs:", error);
} finally {
setLoading(false);
}
};
fetchNFTs();
}, [wallet]);
if (!wallet) {
return (
<div className="max-w-7xl mx-auto py-16 px-4 text-center">
<h1 className="text-3xl font-bold text-gray-900">Please connect your wallet</h1>
<p className="mt-2 text-gray-600">Sign in with your email to view your NFTs</p>
</div>
);
}
if (loading) {
return (
<div className="max-w-7xl mx-auto py-16 px-4 text-center">
<p>Loading your NFTs...</p>
</div>
);
}
return (
<div className="max-w-7xl mx-auto py-16 px-4 sm:py-24 sm:px-6 lg:px-8">
<h1 className="text-3xl font-bold text-gray-900 mb-8">Your NFTs</h1>
{nfts.length === 0 ? (
<p>You don't have any NFTs yet.</p>
) : (
<div className="grid grid-cols-1 sm:grid-cols-2 lg:grid-cols-3 gap-6">
{nfts.map((nft) => (
<div key={nft.metadata.id} className="border rounded-lg overflow-hidden shadow-lg">
{nft.metadata.image && (
<div className="relative h-60 w-full">
<Image
src={nft.metadata.image}
alt={nft.metadata.name || "NFT"}
fill
style={{ objectFit: "cover" }}
/>
</div>
)}
<div className="p-4">
<h2 className="text-xl font-semibold">{nft.metadata.name}</h2>
<p className="text-gray-600 mt-2">{nft.metadata.description}</p>
<p className="text-sm text-gray-500 mt-2">Quantity: {nft.quantityOwned}</p>
</div>
</div>
))}
</div>
)}
</div>
);
}
Also, update your next.config.mjs
to allow image domains:
/** @type {import('next').NextConfig} */
const nextConfig = {
images: {
domains: ['ipfs.io', 'gateway.ipfscdn.io'],
},
};
export default nextConfig;
Running the Application
- Start the server:
node scripts/generate.mjs
- In a separate terminal, start the frontend:
npm run dev
- Navigate to http://localhost:3000 in your browser
Testing the Application
- Enter an email in the newsletter signup form and click "Subscribe"
- The server will create a pre-generated wallet and send an NFT to it
- Click the "Connect" button and sign in with the same email
- You'll be connected to the pre-generated wallet
- Visit the "Profile" page to see your exclusive NFT
Conclusion
Pre-generated wallets offer a simple way to onboard users to Web3 applications without the complexity of traditional wallet creation. By linking wallets to familiar authentication methods like email, you can provide a seamless experience while still giving users access to blockchain assets.
This approach is particularly useful for:
- Gaming applications that want to reward players
- Loyalty programs using tokens or NFTs
- Content platforms with exclusive digital assets
- Any application looking to simplify Web3 onboarding
By implementing this pattern, you're removing a significant barrier to adoption while still giving users all the benefits of blockchain technology.
Happy building!