Build a Web3 Mobile App
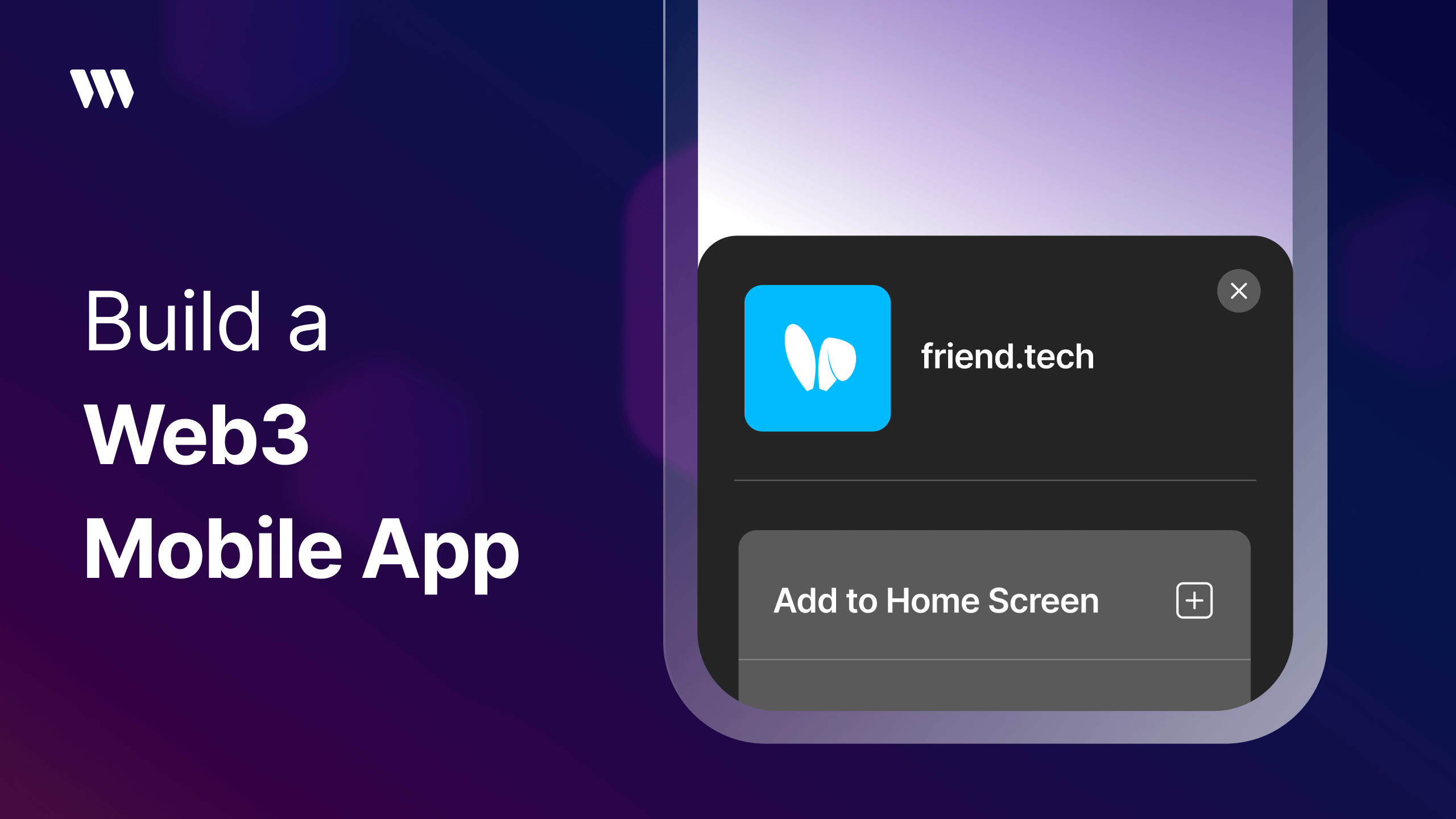
⚠️ Warning: This guide currently uses v4 of the Connect SDK. For v5 (latest) code snippets, please check out our documentation while this guide is being updated. ⚠️
This guide will show you how to create a mobile web3 app using react native and thirdweb! We'll build an app where users can connect their wallets, and claim NFTs!
If you prefer watching videos over reading guides, you can check out this video below:
We'll walk through the following:
- Creating an NFT Drop Contract
- Creating a React Native App
- Adding claim functionality
Before we begin, you can access the complete source code for this template on GitHub.
Let's get started!
Deploy the NFT Drop Smart Contract
To begin, head to the Contracts page in your thirdweb Dashboard and hit "Deploy new contract":
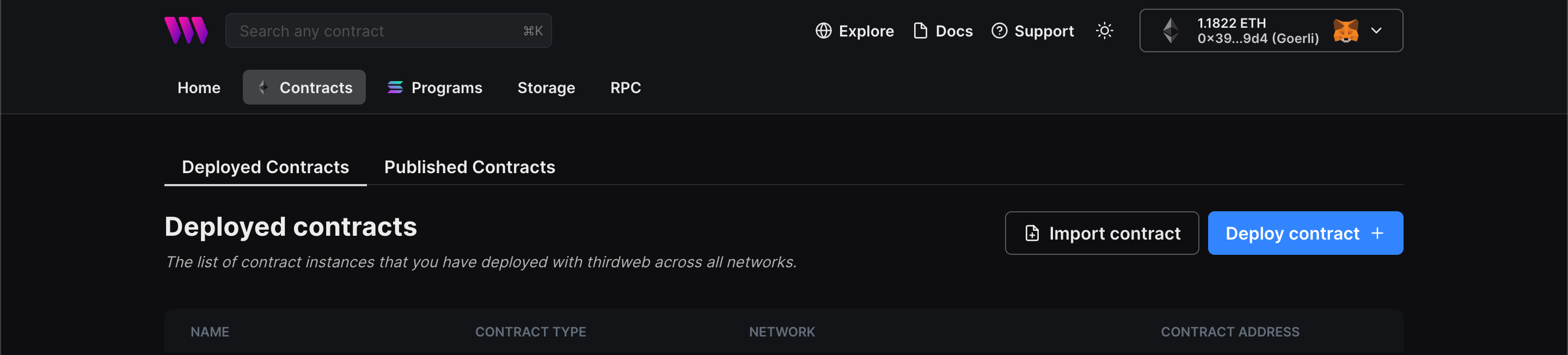
You will be taken to our Explore page — where you can browse smart contracts built by the top protocols in web3 and deploy them in just a few clicks!
Note: You can also use the thirdweb CLI to set up a smart contract environment by running the below command from your terminal:
npx thirdweb create contract
This will take you through an easy-to-follow flow of steps for you to create your contract. Learn more about this in our CLI guide.
Otherwise, let's get back to Explore:
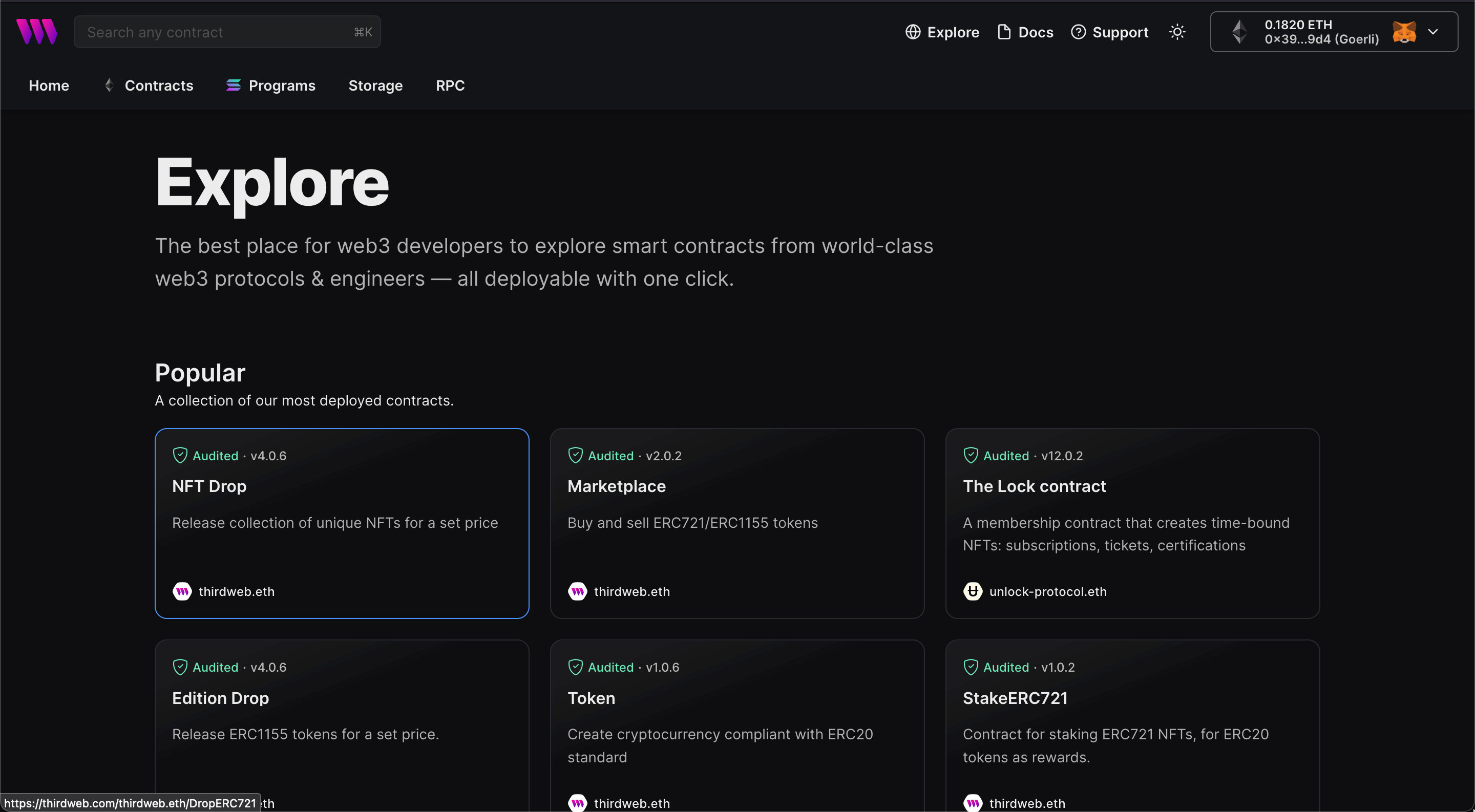
Here, select your smart contract of choice. For this guide, we're going to use the NFT Drop (ERC721A) contract to create our NFT collection:
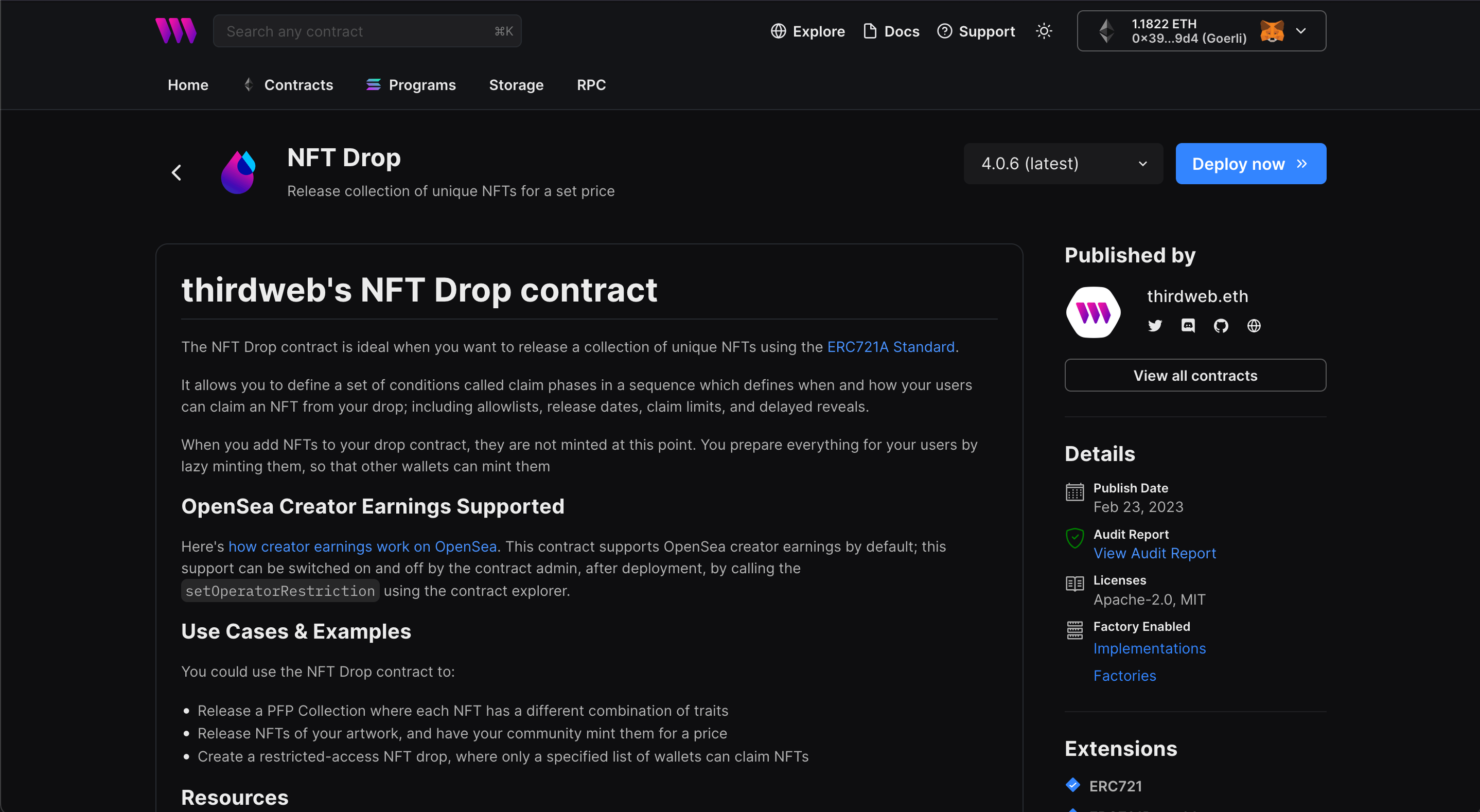
Set up your smart contract with an image, name, description, etc., and configure which wallet address will receive the funds from primary and secondary sales:
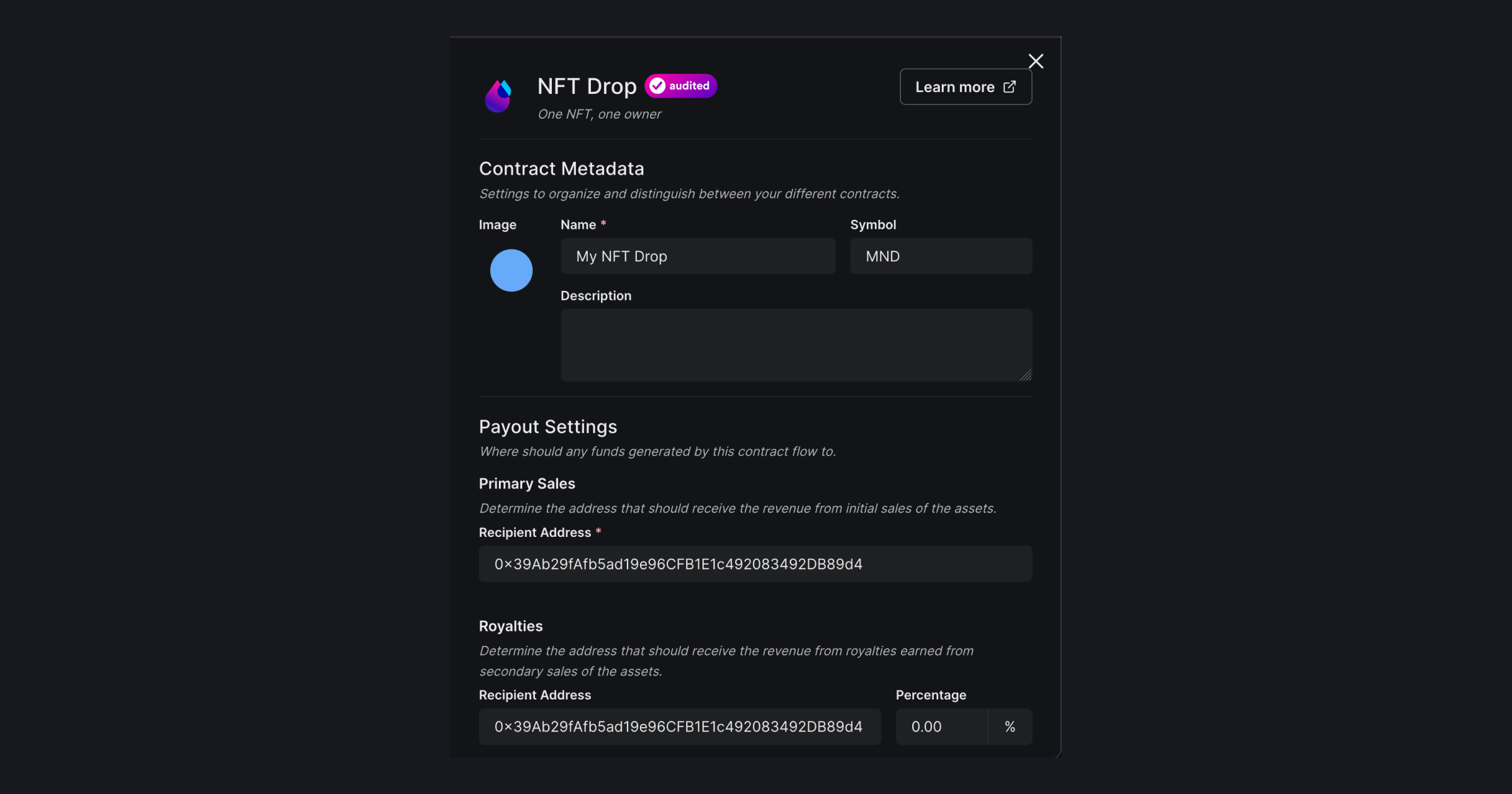
You can select any network you like; for this guide, I am choosing Goerli. Learn more about the different networks we have available below:
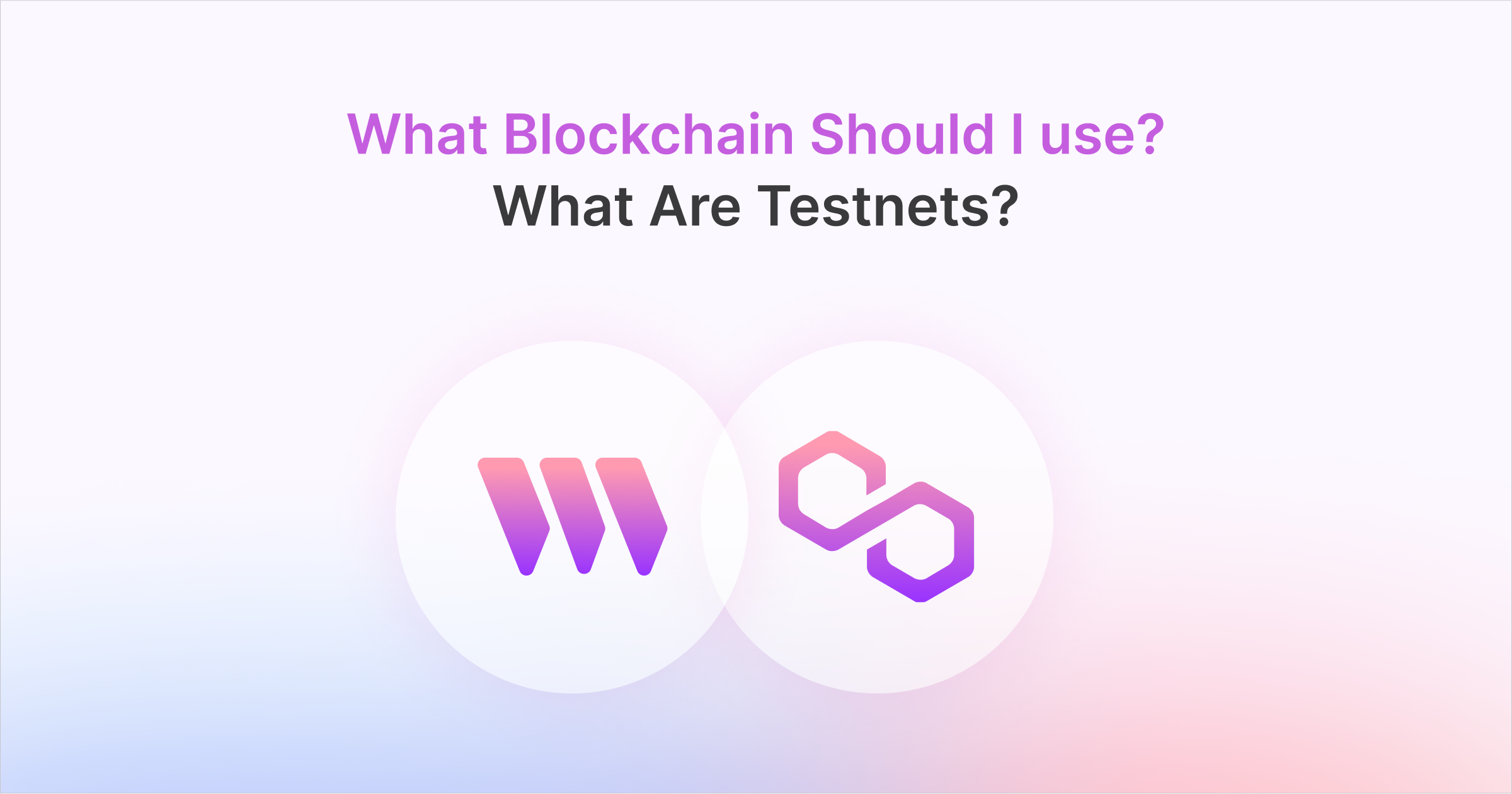
Once the contract is deployed you will be able to see the contract dashboard.
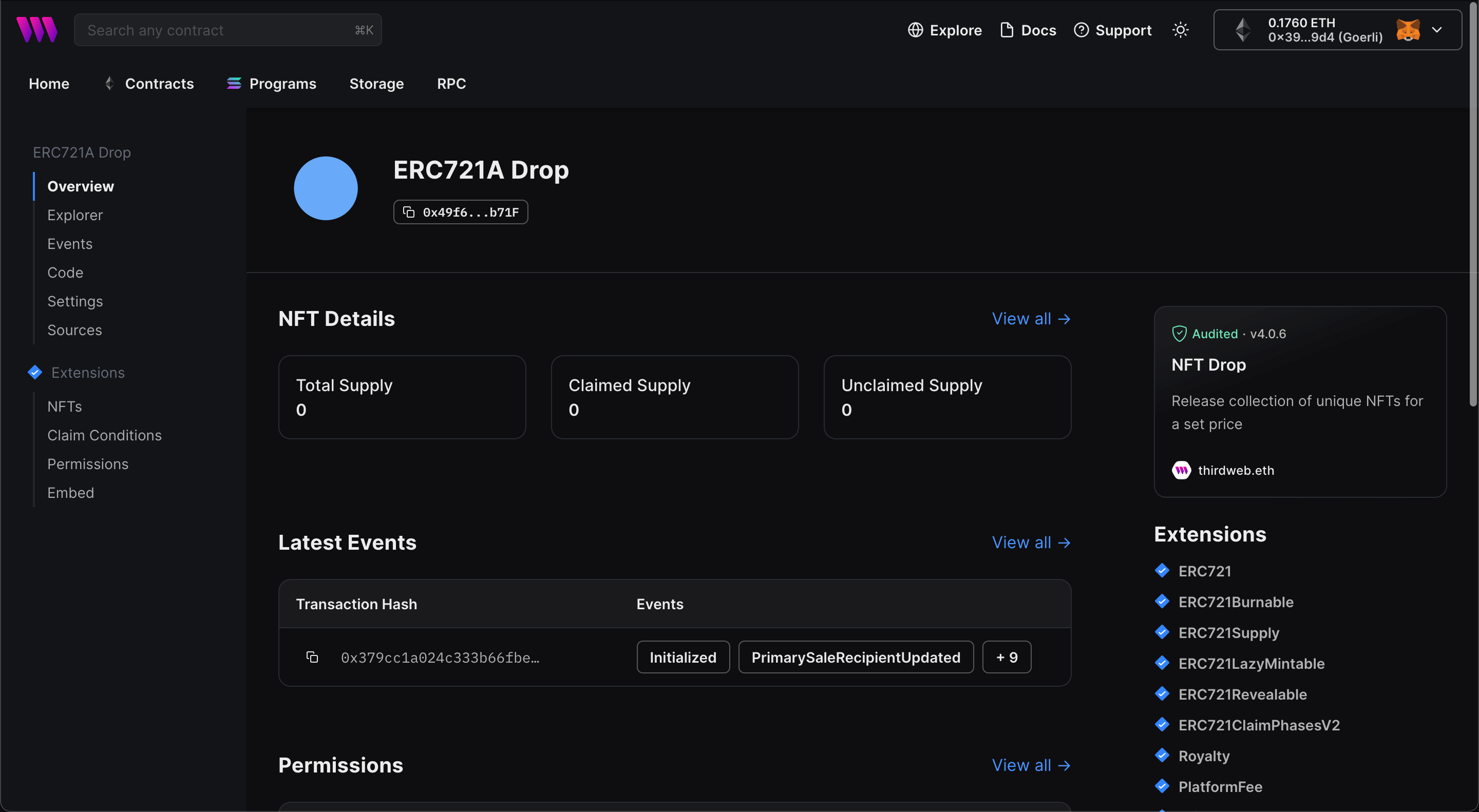
Batch upload NFTs
We'll now upload a bunch of NFTs to our contract for people to claim! Head over to the NFTs tab and click on "Batch Upload":
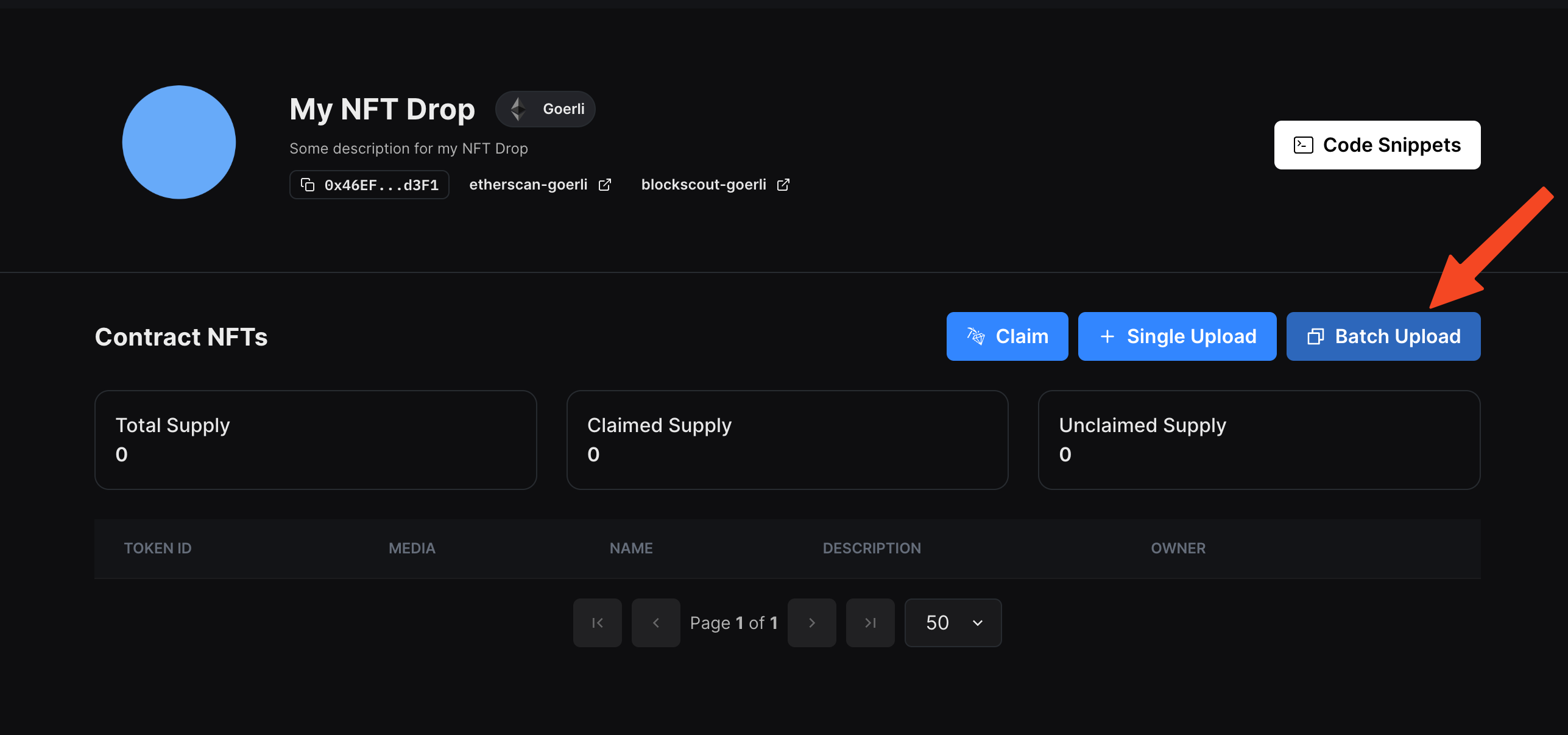
A drawer will slide in, upload your NFTs here:
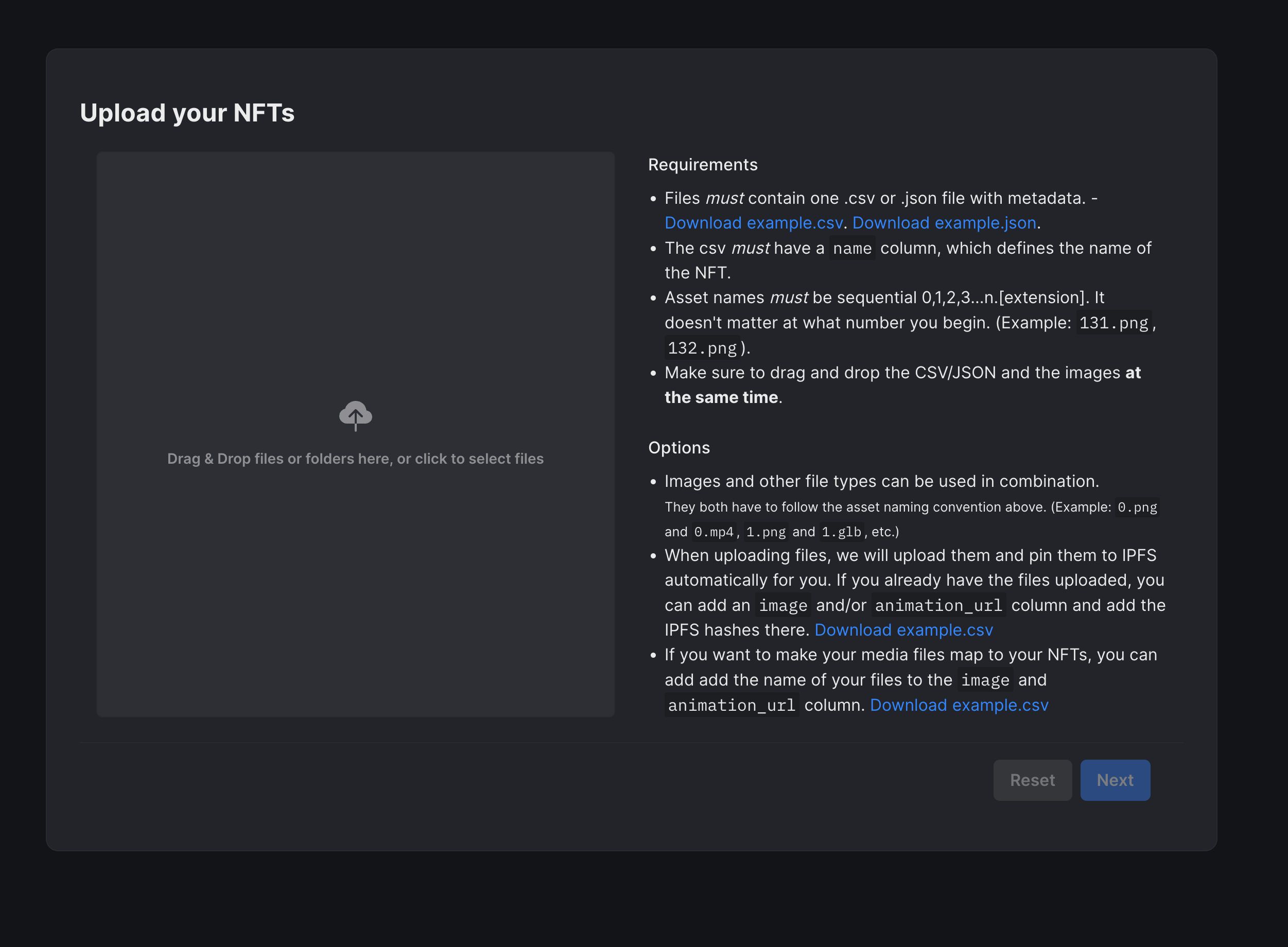
Once it is uploaded you'll be able to preview the NFTs. You can go through all your NFTs and if everything looks good click on Next:
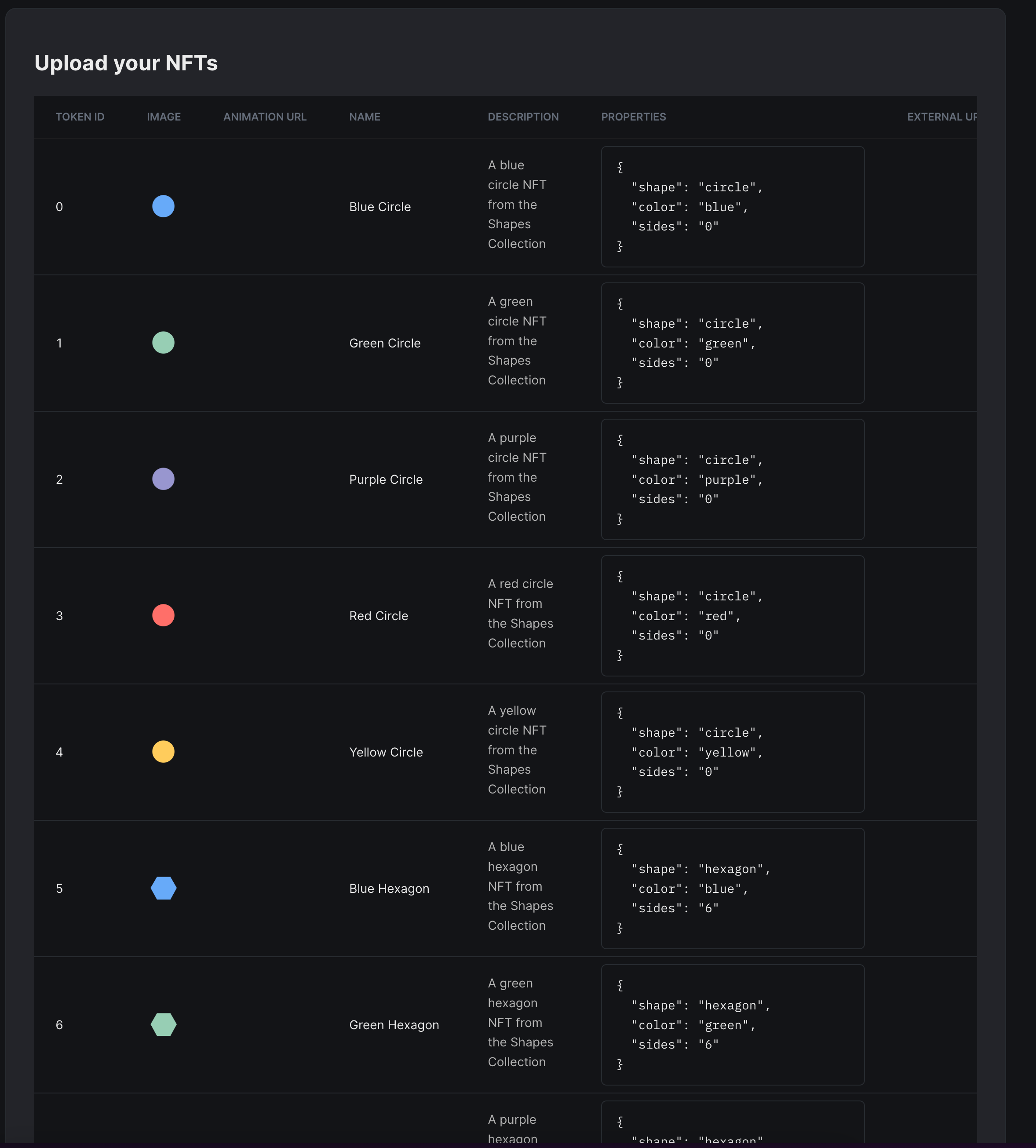
Select when you want to reveal your NFTs, and click on upload!
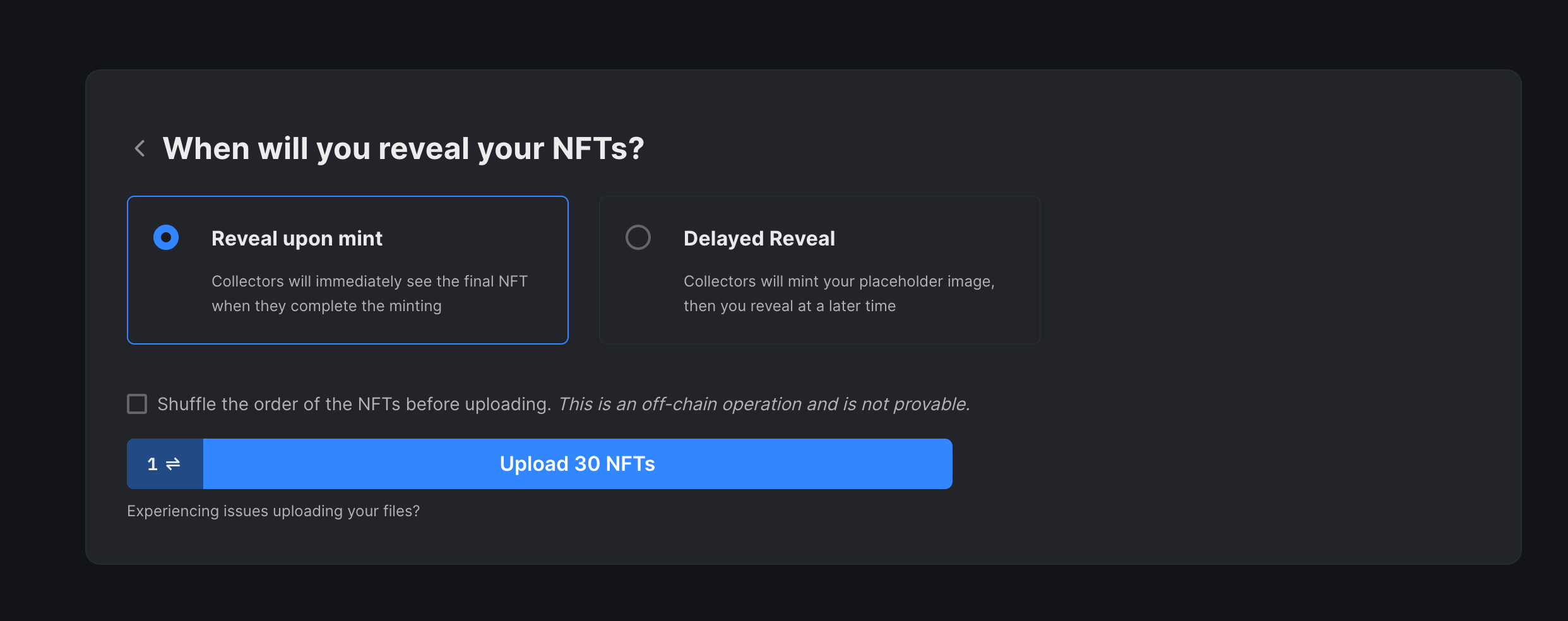
Once they are uploaded you'll be able to see all your NFTs in the NFTs tab:
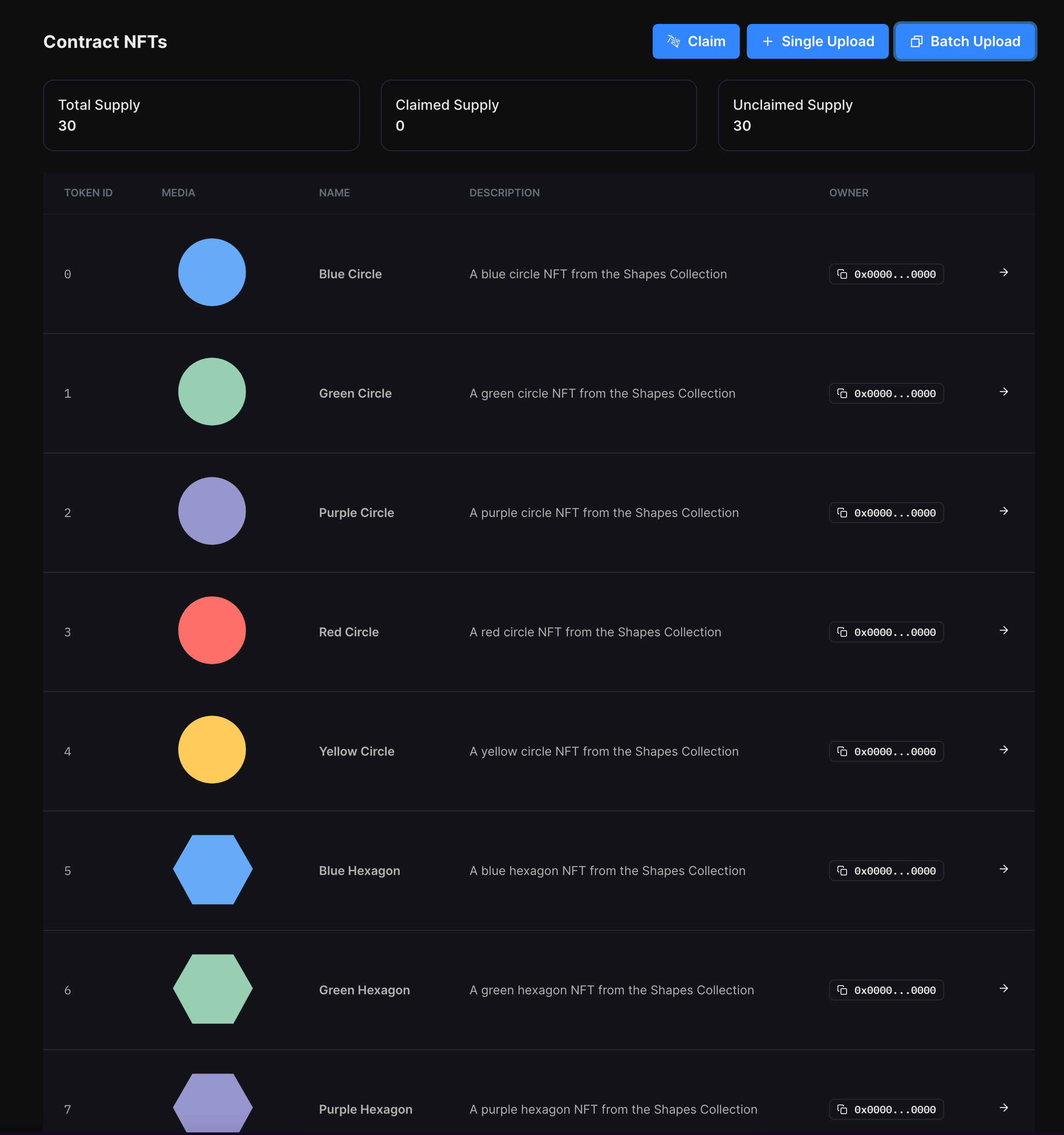
Set Up Claim Conditions
Claim conditions are the criteria that define who, when, and how users can claim an NFT from your drop; such as release dates, allowlists, and claim limits.
To add a claim phase, head to the Claim Conditions
tab and click Add Initial Claim Phase
:
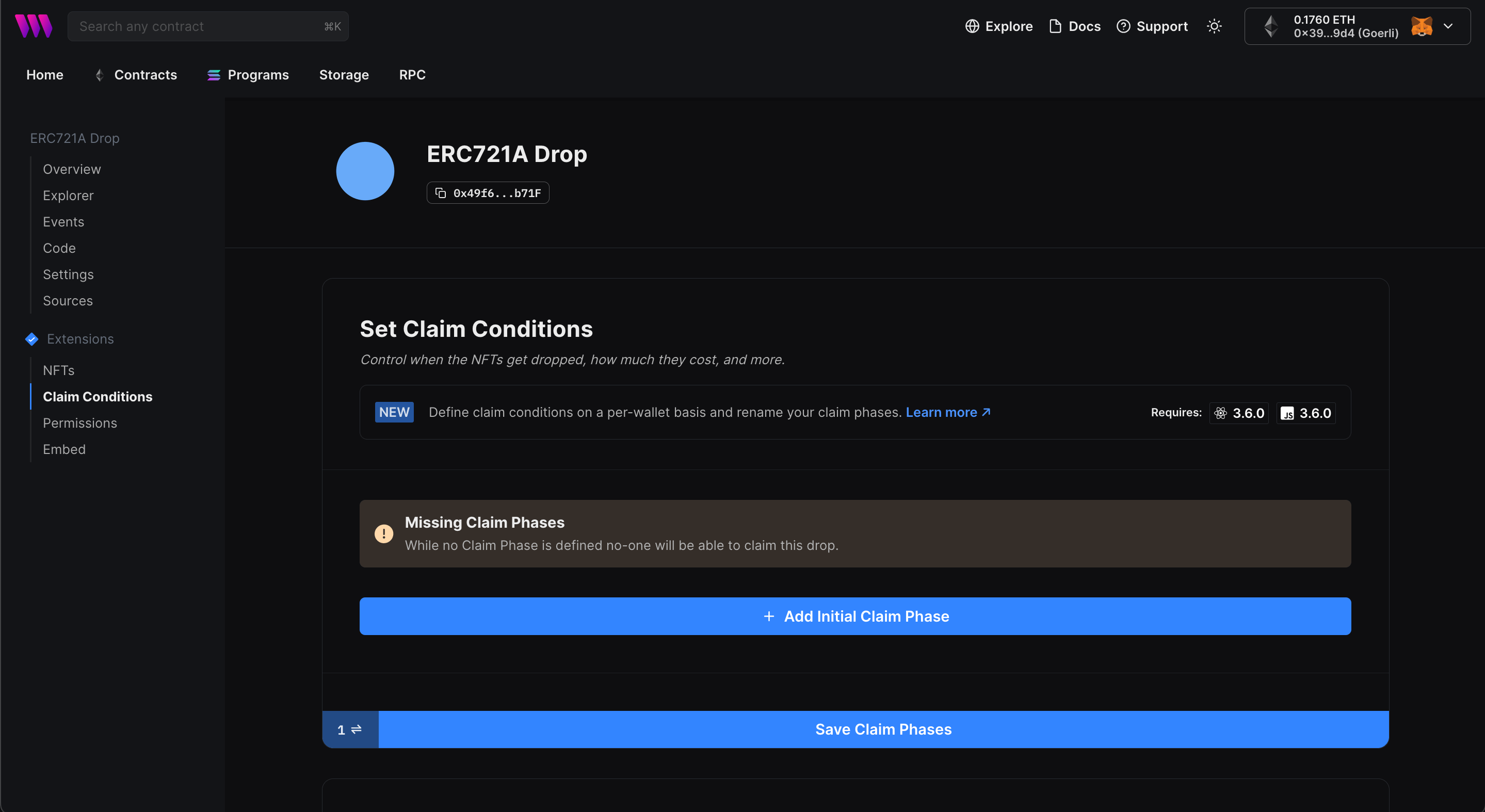
Here, you can configure the price, release date, and more for your NFT drop:
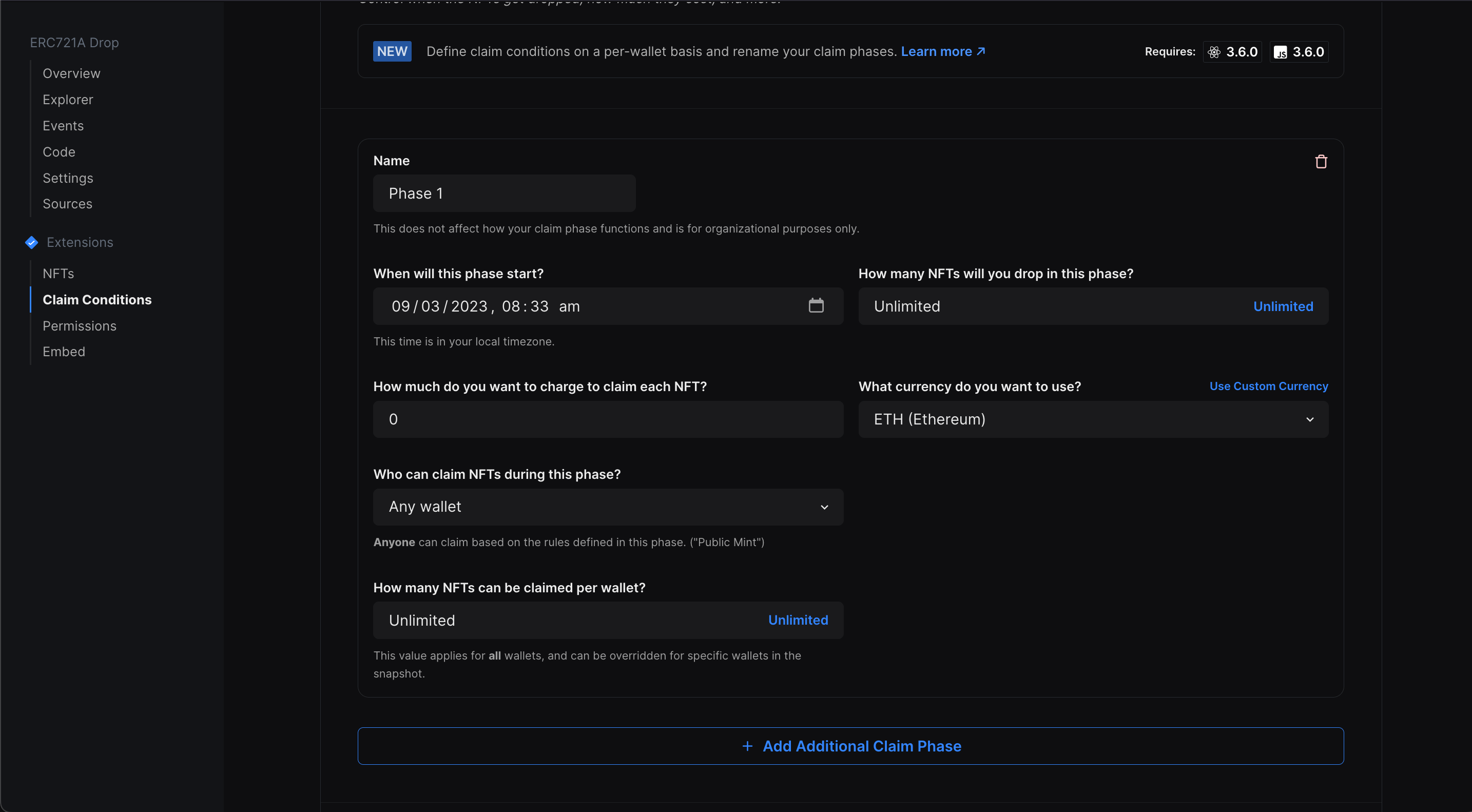
Update the details as you want and save the claim phase!
Creating the react native app
Mobile development requires a bit more setup compared to web development, so make sure you have everything configured properly. You can check the setting up the development environment docs.
Using the thirdweb CLI, create a new React Native & TypeScript project with the React Native SDK preconfigured for you using the following command:
npx thirdweb create app
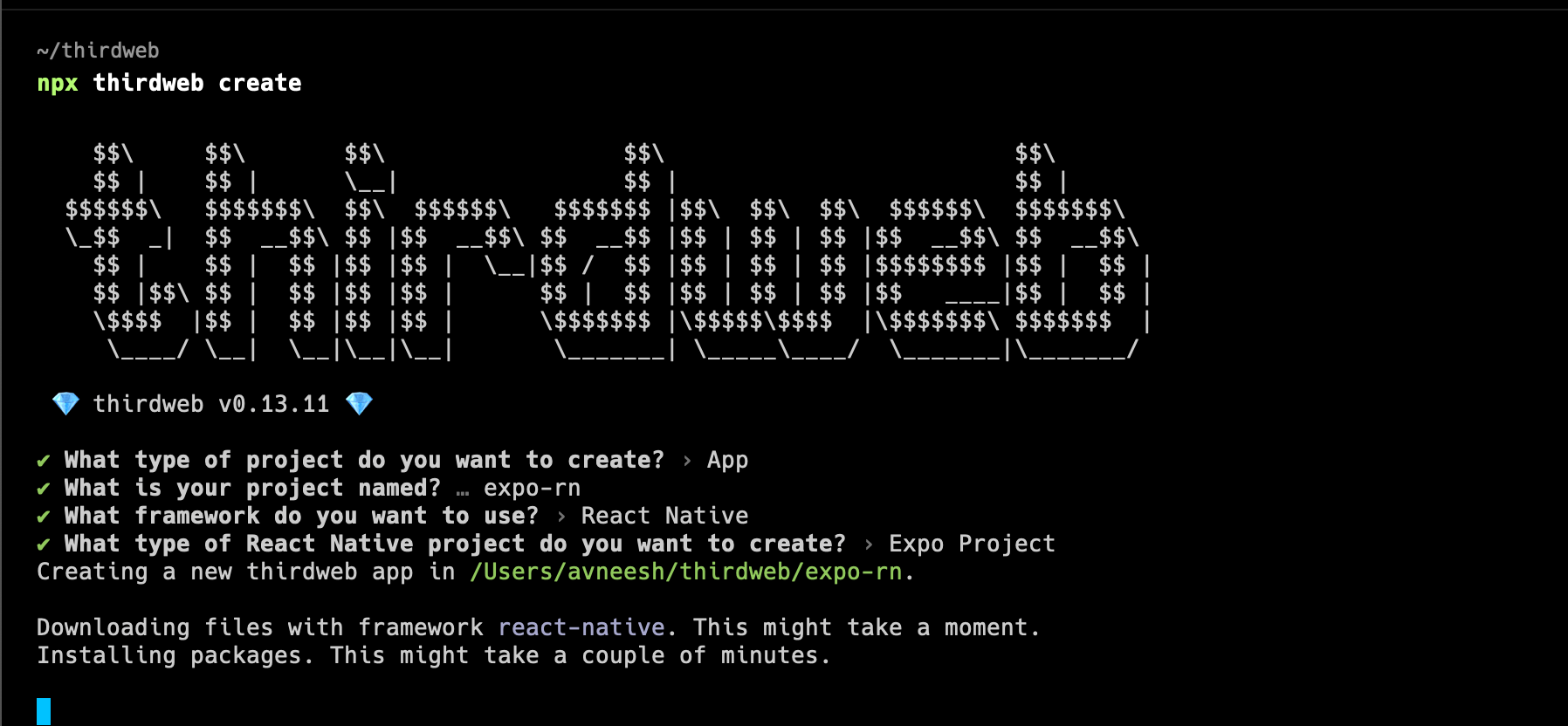
For this demo I am creating an expo app but you can create a react native cli project as well.
Once the app is created, create a new .env
file and add your client ID like this:
EXPO_PUBLIC_TW_CLIENT_ID=CLIENT_ID_HERE
Now, let's start our app! To run the app on an ios simulator run:
yarn run ios
And if you want to run it on your android simulator run:
yarn run android
Give it some time to compile, once it's complied you'll be able to see a simple starter app:
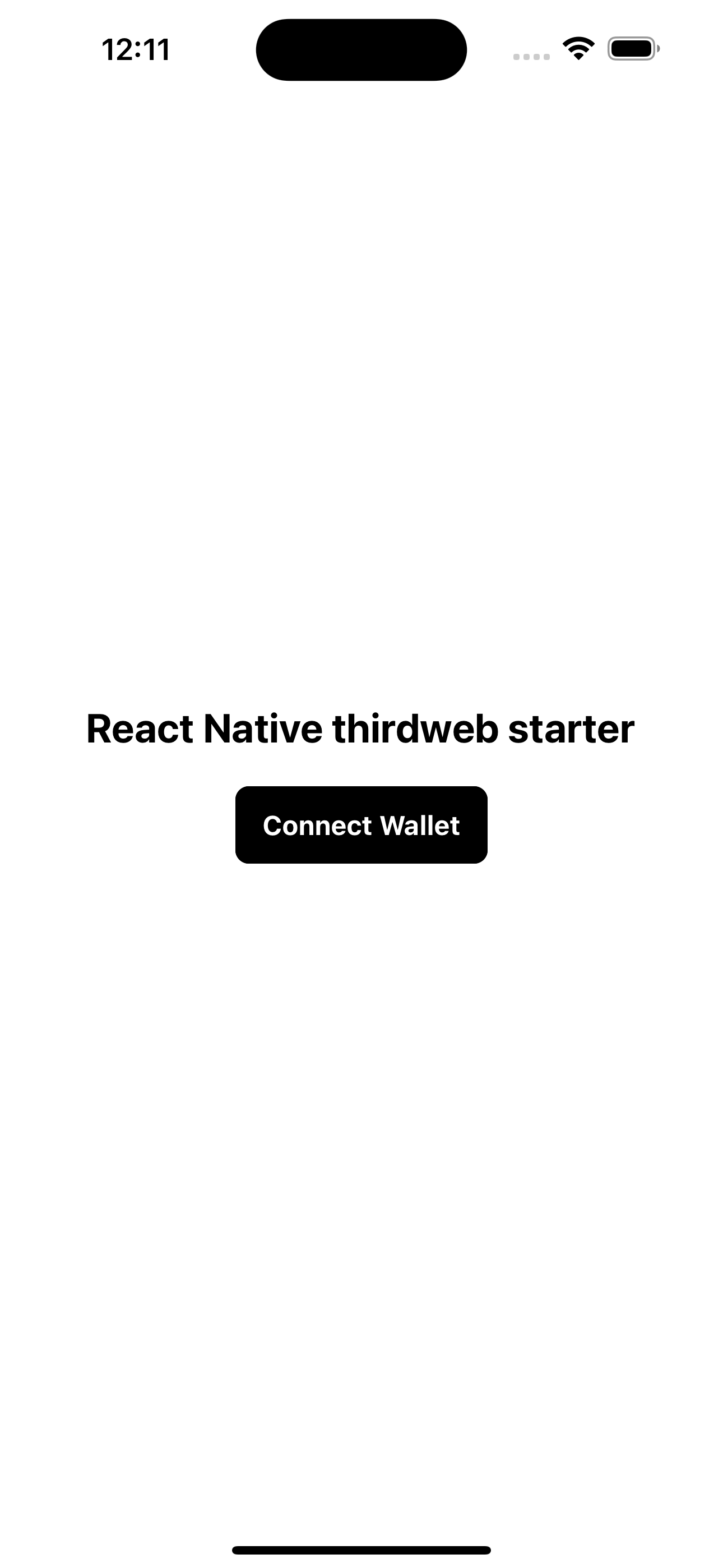
Now, let's get started with our build! Firstly, change the activechain
to the chain you deployed your contract to, in my case goerli
.
We can now use our hooks to get the contract metadata like this:
const contractAddress = "0x46EF402f8b80419B30a3912bCD27E7d219eBd3F1";
const { contract } = useContract(contractAddress, "nft-drop");
const { data: contractMetadata } = useContractMetadata(contract);
We can use this metadata to render it on the screen like this:
<View style={styles.nftCard}>
<Image
style={styles.nftImage}
source={{
uri: contractMetadata?.image,
}}
/>
<Text style={styles.title}>{contractMetadata?.name}</Text>
<Text style={styles.descriptionText}>
{contractMetadata?.description}
</Text>
</View>
If you check your app you should be able to see the metadata of your contract:
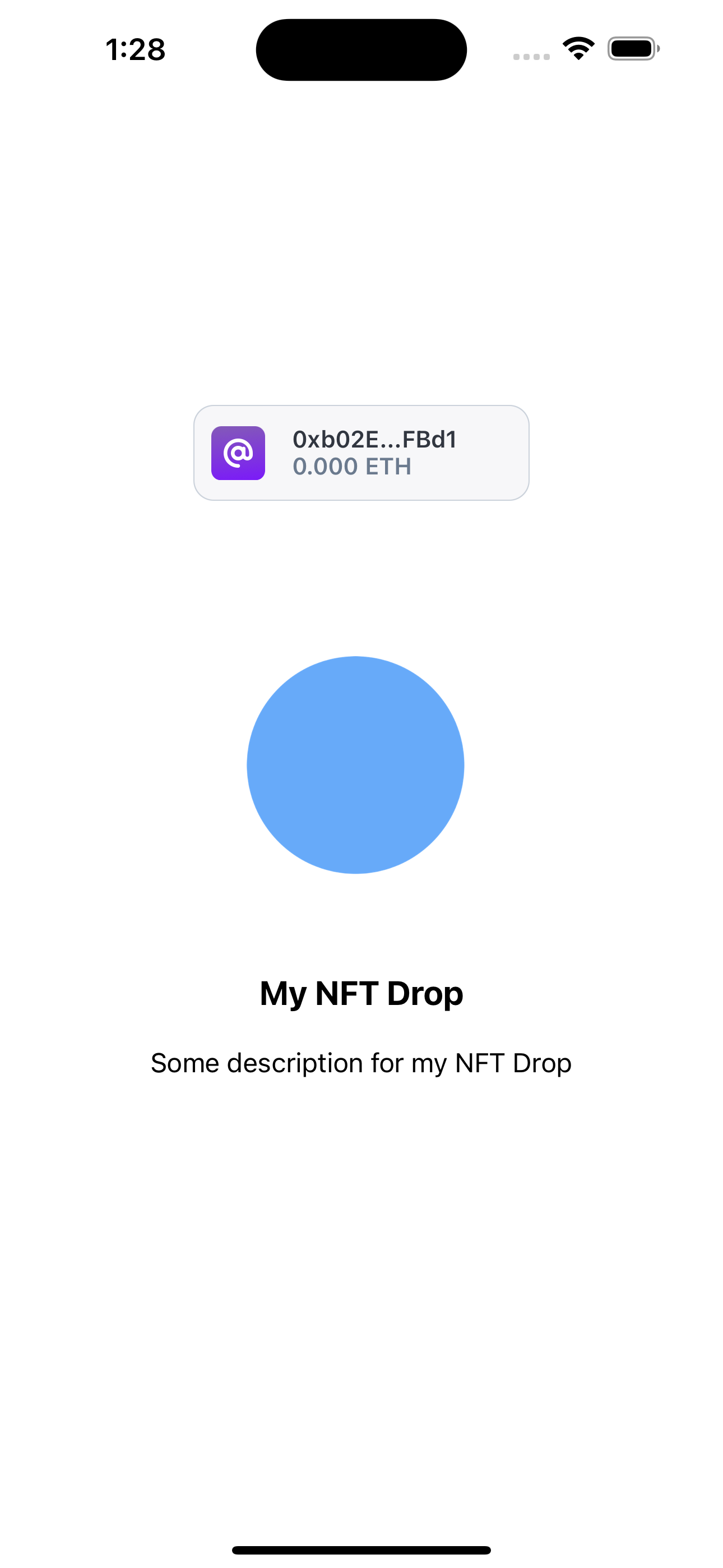
Let's now add a claim button! For the claim button we'll use the Web3Button
:
<Web3Button
contractAddress={contractAddress}
action={(contract) => contract.erc721.claim(1)}
>
Claim
</Web3Button>
You'll now be able to see a claim button! If you click claim you'll be prompted to sign a transaction and once the transaction goes through you will get your NFT in your wallet!
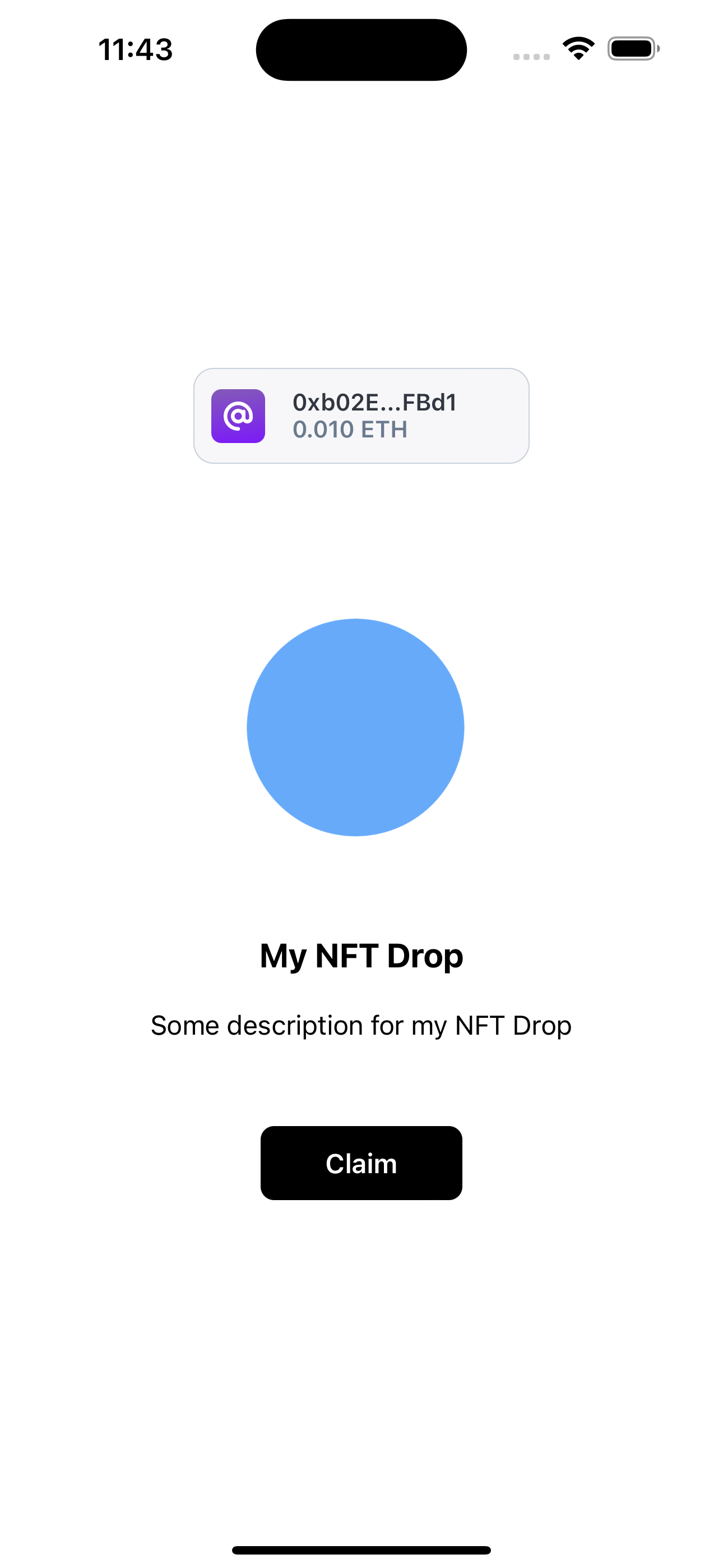
Let's now fetch the number of NFTs a wallet owns so we can display that as well!
First, use the useNFTBalance
hook to get the balance:
const { data: nftBalance } = useNFTBalance(contract, address);
Now, we can display the balance like this:
<Text>You own: {nftBalance && nftBalance?.toNumber() | 0} NFT(s)</Text>
If you have claimed an NFT, you should be able to see you own 1 NFT!
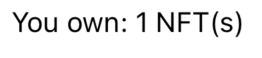
Conclusion
This guide taught us how to use the thirdweb react native SDK to create an NFT claiming app.
You learned a lot, now pat yourself on the back and share your amazing apps with us on the thirdweb discord! If you want to look at the code, check out the GitHub Repository.
Need help?
For support, join the official thirdweb Discord server or share your thoughts on our feedback board.