Build a Token Minting Button with React
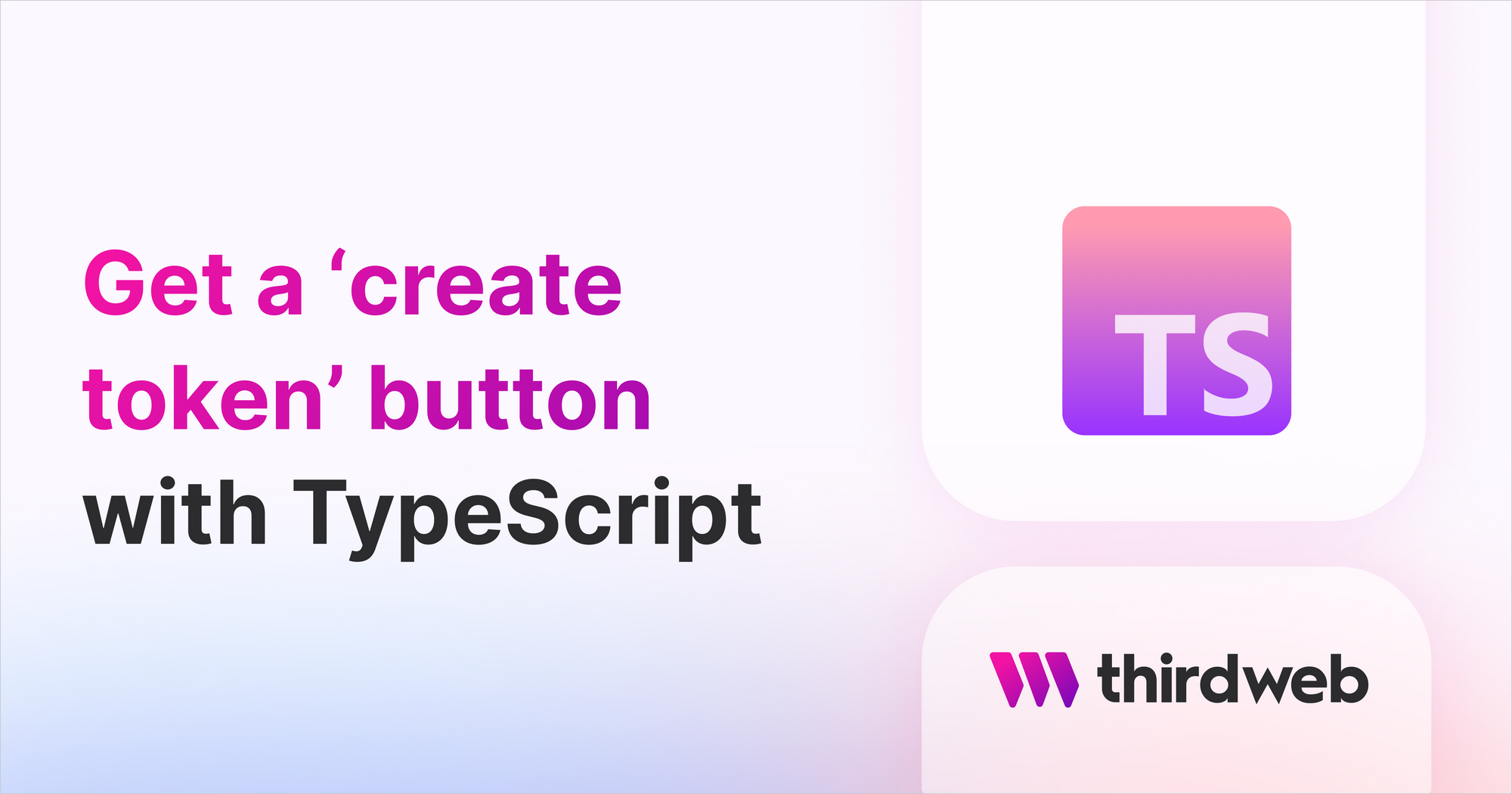
⚠️ Warning: This guide currently uses v4 of the Connect SDK. For v5 (latest) code snippets, please check out our documentation while this guide is being updated. ⚠️
In this guide, we'll show you how to create a button in your app that allows users to mint ERC20 tokens! You'll build a simple React application that:
- Allows users to view their balance of the token
- Mint more tokens by clicking a button
If you want to know more about the token contract, check out our pre-built contract page.
Getting Started
To get started with a new thirdweb project, use the CLI:
npx thirdweb create
Select Create React App
and JavaScript
.
Viewing Token Balance
Inside your App.js
file, let's connect to the Token
smart contract and view our balance!
import {
useAddress,
useDisconnect,
useMetamask,
useToken,
useTokenBalance,
} from "@thirdweb-dev/react";
export default function Home() {
// Hooks to connect to the user's MetaMask Wallet and view their address
const address = useAddress();
const connectWithMetamask = useMetamask();
const disconnectWallet = useDisconnect();
// Connect to the token smart contract
const token = useToken("0x7A51F9201A8e4d52697c06e4D7C33AF3Ce940ae8"); // your token contract address here
// Get the balance of the connected wallet
const { data: tokenBalance } = useTokenBalance(token, address);
return (
<div>
{address ? (
<>
<button onClick={disconnectWallet}>Disconnect Wallet</button>
<p>Your address: {address}</p>
<p>
Your balance: {tokenBalance?.displayValue} {tokenBalance?.symbol}
</p>
</>
) : (
<button onClick={connectWithMetamask}>Connect Wallet</button>
)}
</div>
);
}
Minting Tokens
To mint tokens, let's import the useMintToken
hook:
const { mutate: mintToken } = useMintToken(token);
Then, attach this mintToken
function to a button:
<button
onClick={() =>
mintToken({
amount: 1,
to: address,
})
}
>
Mint Tokens
</button>
That's it!
You've just minted tokens in your own web application!
Congratulations!
Check out what else you can do with the token contract on our pre-built contract page.