Using the Web3 SDK: Frontend or Backend?
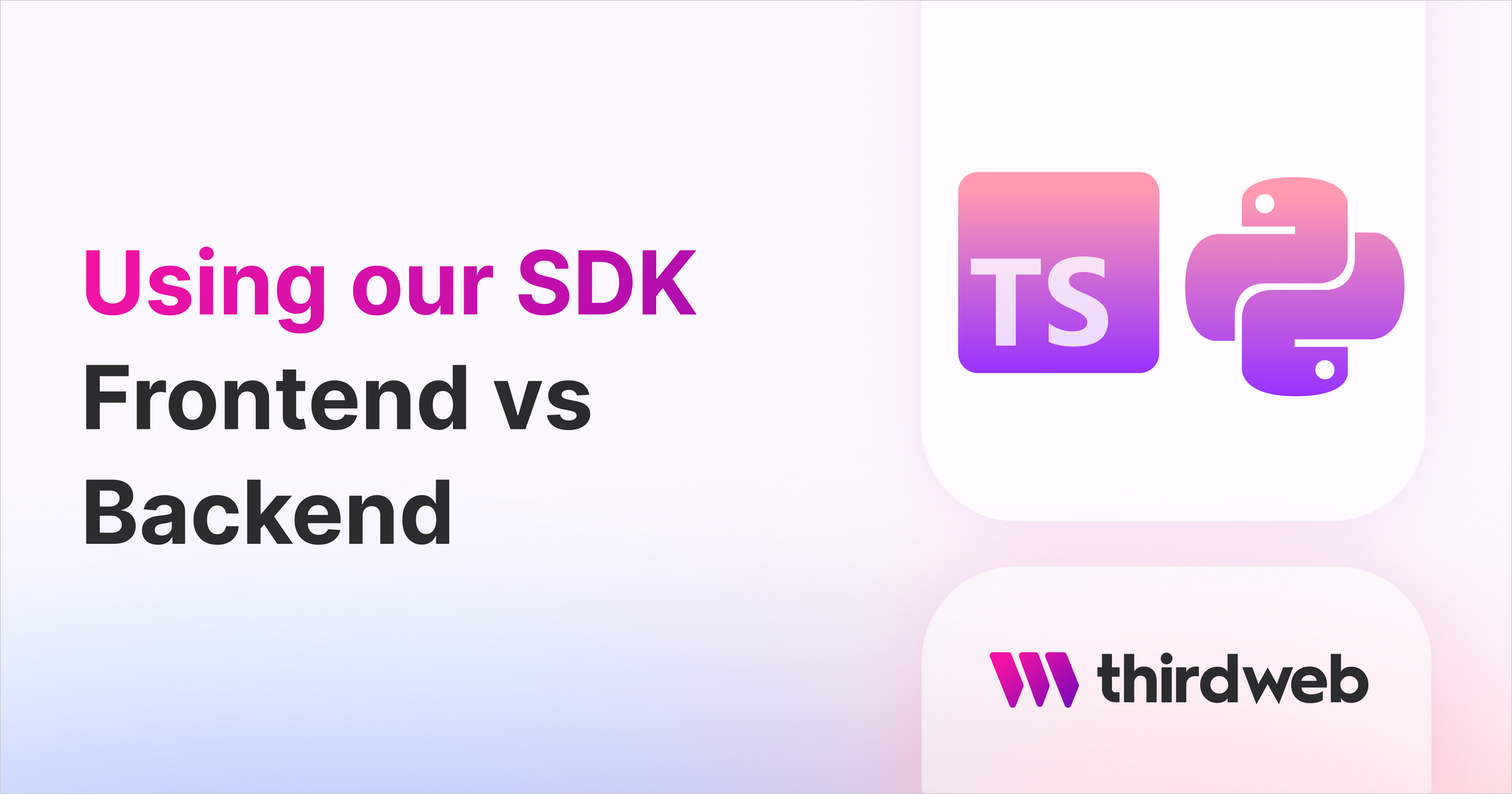
This guide shows how to use our SDK effectively and where your code should live depending on your use case.
Frontend
Here is a typical use case. You have an app (or are building one) and you want users to claim an NFT.
You have everything set up and just want that button. In this case, you integrate our SDK into the frontend.
The user triggers a transaction and the frontend (client-side) interacts with the blockchain.
Example: I click on the claim
button and the client-side makes sure my wallet pops up, and that I authorize the transaction.
In this case, you can use the React and TypeScript SDKs to help build out your application.
The first step is to wrap your application in the ThirdwebProvider
.
import { ChainId, ThirdwebProvider } from "@thirdweb-dev/react";
const App = () => {
return (
<ThirdwebProvider desiredChainId={ChainId.Mainnet}>
<YourApp />
</ThirdwebProvider>
);
};
Any transaction you attempt to make now will be made with the connected wallet (if they approve the transaction).
To connect to a user's wallet, you can use one of our wallet connection hooks.
Then, connect to your smart contract with one of our contract hooks and start interacting with it.
Below is an example of how to combine the useMetamask hook with the
useNFTCollection hook to mint an NFT from the connected wallet on the frontend:
import { useAddress, useMetamask, useNFTCollection } from "@thirdweb-dev/react";
const MintNFTComponent = () => {
// Read the connected wallet's address (undefined if no connected wallet)
const address = useAddress();
// Function to connect the user's MetaMask wallet.
const connectWallet = useMetamask();
// Connect to your smart contract using the React SDK's hooks
const nftCollection = useNFTCollection("<NFT-COLLECTION-CONTRACT-ADDRESS>");
async function mint() {
// If no address is connected, request wallet connection
if (!address) {
connectWallet();
}
// Otherwise, use the connected wallet to mint the NFT
else {
const transaction = await nftCollection.mintToSelf({
name: "My NFT",
});
}
}
return <button onClick={mint}>Mint</button>;
};
Backend
If you are writing scripts or running a back-end, you can connect to the blockchain using the thirdweb SDK in two different ways:
- Use a read-only connection
- Use your private key to establish a write connection directly from your wallet
Reading Only Connection
If we just want to read out some data, we don't need to pass our private keys.
import { ThirdwebSDK } from "@thirdweb-dev/sdk";
// Create a read-only instance of the ThirdwebSDK
const sdk = new ThirdwebSDK("mumbai"); // configure this to your network
Writing Connection (Private Key)
To use the SDK to sign transactions on the server-side, the initialization is different and requires your wallet's private key.
Ensure you store and access your private key securely.
- Check if you need to use a private key for your application.
- Never directly expose your private key in your source code.
- Never commit any file that may contain your private key to your source control.
- Never use a private key for a frontend (website/dapp) application.
If you are unsure how to securely store and access your private key, please do not proceed.
Learn more about securely accessing your private key.
To get your private key, follow this guide on how to Export your private key.
Then use the .fromPrivateKey
method to create a new instance of the SDK:
import { ThirdwebSDK } from "@thirdweb-dev/sdk";
// Learn more about securely accessing your private key: https://portal.thirdweb.com/web3-sdk/set-up-the-sdk/securing-your-private-key
const privateKey = "<your-private-key-here>",
// instantiate the SDK based on your private key, with the desired chain to connect to
const sdk = ThirdwebSDK.fromPrivateKey(privateKey, "mumbai");