How to Create a Seamless Web3 Onboarding Flow for Your dApp
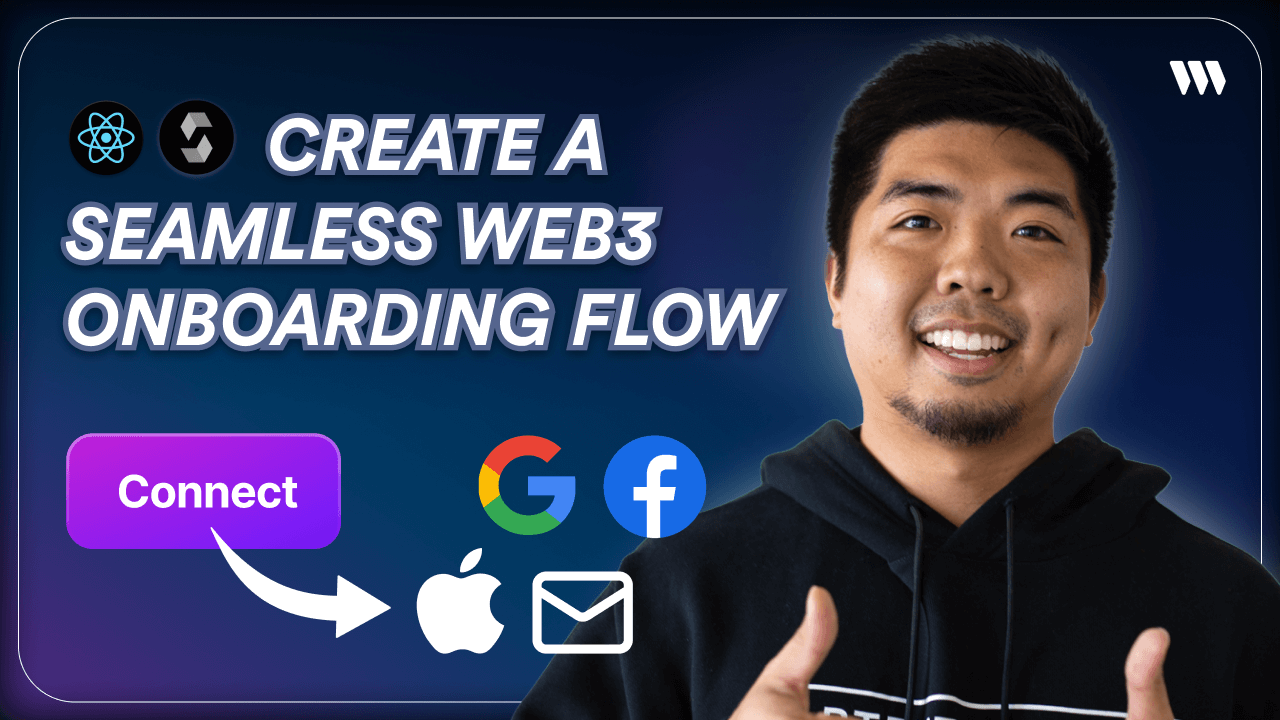
Introduction
Web3 onboarding can be a daunting and frustrating experience for users who are new to blockchain technology. With high restrictions on what's allowed in app stores for blockchain applications, and the complexity of managing seed phrases and gas fees, it's no wonder that many people feel that onboarding into web3 apps simply sucks.
However, there are new tools and technologies emerging that are making the onboarding experience much smoother. By implementing the right onboarding flow for your users, you can abstract away a lot of the blockchain complexities and provide an experience more similar to web2 apps.
In this guide, we'll dive into the different options available for onboarding users into your web3 application using thirdweb's Connect SDK. Whether your target audience is experienced with web3 or completely new to it, you'll learn how to create an onboarding flow that is optimized for them.
Here's what we'll cover:
- The traditional web3 onboarding flow with a web3 wallet (e.g. Metamask)
- Using In-App Wallets allowing email and social login
- Combining ERC-4337 (Account Abstraction) with In-App Wallets
By the end, you'll be ready to build an onboarding flow that eliminates major user friction points and gets more users into your app.
Want to jump straight into the code? Check out the video tutorial:
Setup Client and Chain
To use ConnectButton
you will need to create a client and define your chain.
- Create a client using
createThirdwebClient
import { createThirdwebClient } from 'thirdweb';
const CLIENT_ID = process.env.NEXT_PUBLIC_CLIENT_ID as string;
export const client = createThirdwebClient({
clientId: CLIENT_ID,
});
- Define your chain using
defineChain
import { defineChain } from "thirdweb";
export const chain = defineChain( <chain_id> );
Traditional Web3 Onboarding Flow
The traditional web3 onboarding flow usually involves connecting a MetaMask wallet (or other web3 wallet like Coinbase Wallet) to the app.
For users who are already familiar with web3, this can be a straightforward process. You simply display a "Connect" button, which opens up a modal, where the user can select which account they want to connect with.
Here's how to set this up with thirdweb's Connect SDK:
- Add a
ConnectButton
UI component to your app
import { ConnectButton } from "thirdweb/react";
const YourApp = () => {
return (
<ConnectButton
client={client}
chain={chain}
/>
);
};
- Configure wallets shown in connect modal
import { ConnectButton } from "thirdweb/react";
import { createWallet } from "thirdweb/wallets";
//Add wallets you want shown into array
const wallets = [
createWallet("io.metamask"),
createWallet("com.coinbase.wallet"),
];
const YourApp = () => {
return (
<ConnectButton
client={client}
chain={CHAIN}
wallets={wallets}
/>
)
};
That's it! You now have a fully functional Connect button that will allow users to connect their web3 wallet to your app.
However, there are some downsides to this flow for users who are new to web3:
- They need to already have a web3 wallet set up
- They need to have their wallet funded with the correct token for gas fees
- Signing transactions to interact with your app can be intimidating with confusing information
So while this traditional onboarding may work great for your web3-native users, it presents major friction for those new to web3. Let's look at how to optimize the flow for those users.
Custom Styling the Connect Button
In addition to selecting which wallets to display, you can also fully customize the look and feel of the Connect Button. thirdweb provides a playground where you can change colors, fonts, and more to match your app's design.
To style the button:
- Go to the Connect Button Playground
- Customize the button's appearance using the options provided
- Copy the generated code
Web2-Style Onboarding With In-App Wallets
For users who are not familiar with web3 wallets, an easier onboarding flow is to allow them to sign in with something they are already used to, like an email address or social login.
With thirdweb's In-App Wallets, you can automatically generate a wallet for a user when they sign in, abstracting away the wallet management complexities from the user.
Here's how to implement this flow:
- Taking our
ConnectButton
from the traditional onboarding flow we just need to addinAppWallet
in our array of wallets
import { ConnectButton } from "thirdweb/react";
import { inAppWallet } from "thirdweb/wallets";
//Add In-App Wallet
const wallets = [
inAppWallet(),
];
const YourApp = () => {
return (
<ConnectButton
client={client}
chain={chain}
wallets={wallets}
connectButton={{
label: "Login"
}}
/>
)
};
ConnectButton
by changing the label
This will prompt the user to enter their email address, and then send them an OTP to sign in.
The major benefit of this flow is that the user doesn't need to worry about anything related to web3 wallets. They can simply use their email to create an account, making it feel like a familiar web2 login experience.
However, there are some downsides to this flow for users:
- They still need to have their wallet funded with the correct token for gas fees
- They will need to send funds to a completely new wallet address created for them
So while onboarding with In-App Wallets may work great for creating wallets for your users, it still presents some friction on getting funds to interact with the blockchain. Let's look at how to optimize this with the use of Account Abstraction.
Fiat Onramp, Swap, and Bridge
One of the friction points besides creating a wallet is funding a wallet. With thirdweb's ConnectButton
you now have the ability to do that all within your app.
To enable this, you just need to add a ConnectButton
UI component to your app and any user will have the ability to "Buy" tokens on supported chains.
Gasless Transactions with Account Abstraction
Even if you abstract away the wallet management from the user, they still need to have their wallet funded with the native token required for gas fees on the network you're using.
This presents two challenges for onboarding:
- You need to prompt the user to acquire the token on the correct network
- The user needs to bridge/swap that token into their generated In-App Wallet
We can solve both of these challenges by enabling gasless transactions with ERC-4337 (Account Abstraction). This allows us to cover the gas fees on behalf of the user, so they can start interacting with our app with zero token balance.
Here's how to implement In-App Wallets with Account Abstraction:
- Deploy an Account Factory smart contract on the network your app uses
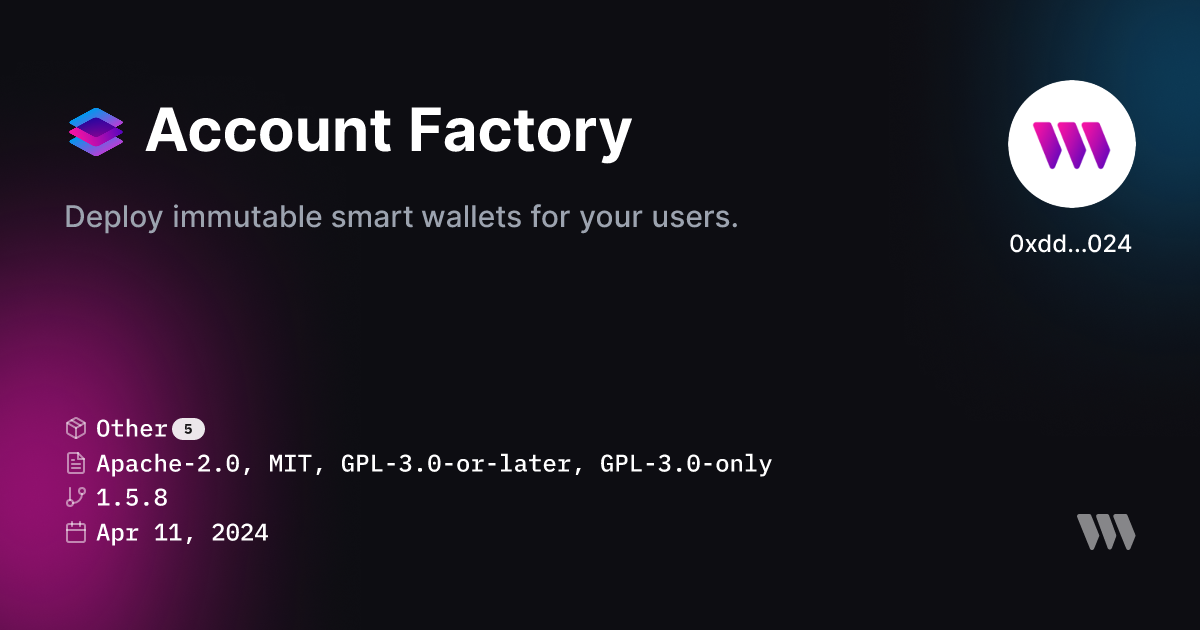
- Add Account Abstraction to you
ConnectButton
with In-App Wallet
import { ConnectButton } from "thirdweb/react";
import { inAppWallet } from "thirdweb/wallets";
const wallets = [
inAppWallet(),
];
const accountAbstraction = {
chain: chain,
factoryAddress: "<contract_address>",
gasless: true,
}
export const AALogin = () => {
return (
<ConnectButton
client={client}
chain={chain}
wallets={wallets}
accountAbstraction={accountAbstraction}
connectButton={{
label: "AA Login"
}}
/>
)
};
In this example, we can use Smart Wallets (Account Abstraction) and the use of a paymaster to sponsor the gas fees needed for the user. This means that a user can sign-in with an email or social login and instantly start using our app and interacting with the blockchain without needing to fund their wallet.
Choosing the Right Onboarding Flow
With these options available, you can choose the onboarding flow that makes the most sense for your app and target users:
- If your audience is mostly web3 natives, the traditional flow may be sufficient
- If you're targeting some web3 beginners, using In-App Wallets can abstract away some of the complexities
- If you expect many non-crypto-native users, adding gasless transactions and a fiat onramp will be crucial to maximize adoption
The key is to deeply understand your users and implement an onboarding flow that meets them where they're at. With the right flow in place, you'll be able to seamlessly bring more users into your app, even if they have zero prior web3 knowledge.
Conclusion
Creating a seamless onboarding experience is crucial for the mass adoption of web3 applications. By leveraging tools like thirdweb's Connect SDK, In-App Wallets, Account Abstraction, gasless transactions, and fiat onramps, developers can abstract away the complexities of web3 and provide an experience that rivals web2 apps.
The traditional onboarding flow may work well for web3 natives, but it presents significant barriers for newcomers. Using In-App Wallets allows users to get started with just an email or social login. Account Abstraction takes this a step further by enabling gasless transactions, so users don't even need to acquire or manage tokens. And for those who do want to purchase tokens, integrating a fiat onramp provides a familiar checkout experience.
By understanding your target audience and implementing the right combination of these onboarding optimizations, you can dramatically increase adoption of your web3 app. Whether you're building for crypto enthusiasts or complete beginners, thirdweb provides the tools you need to create a tailored onboarding flow.
The web3 onboarding landscape is rapidly evolving, and new solutions are emerging all the time. As a developer, it's important to stay on top of these trends and continuously work to improve your user experience. With the right approach, we can break down the barriers to entry and make web3 accessible to everyone.