Mint An NFT Using Next.js
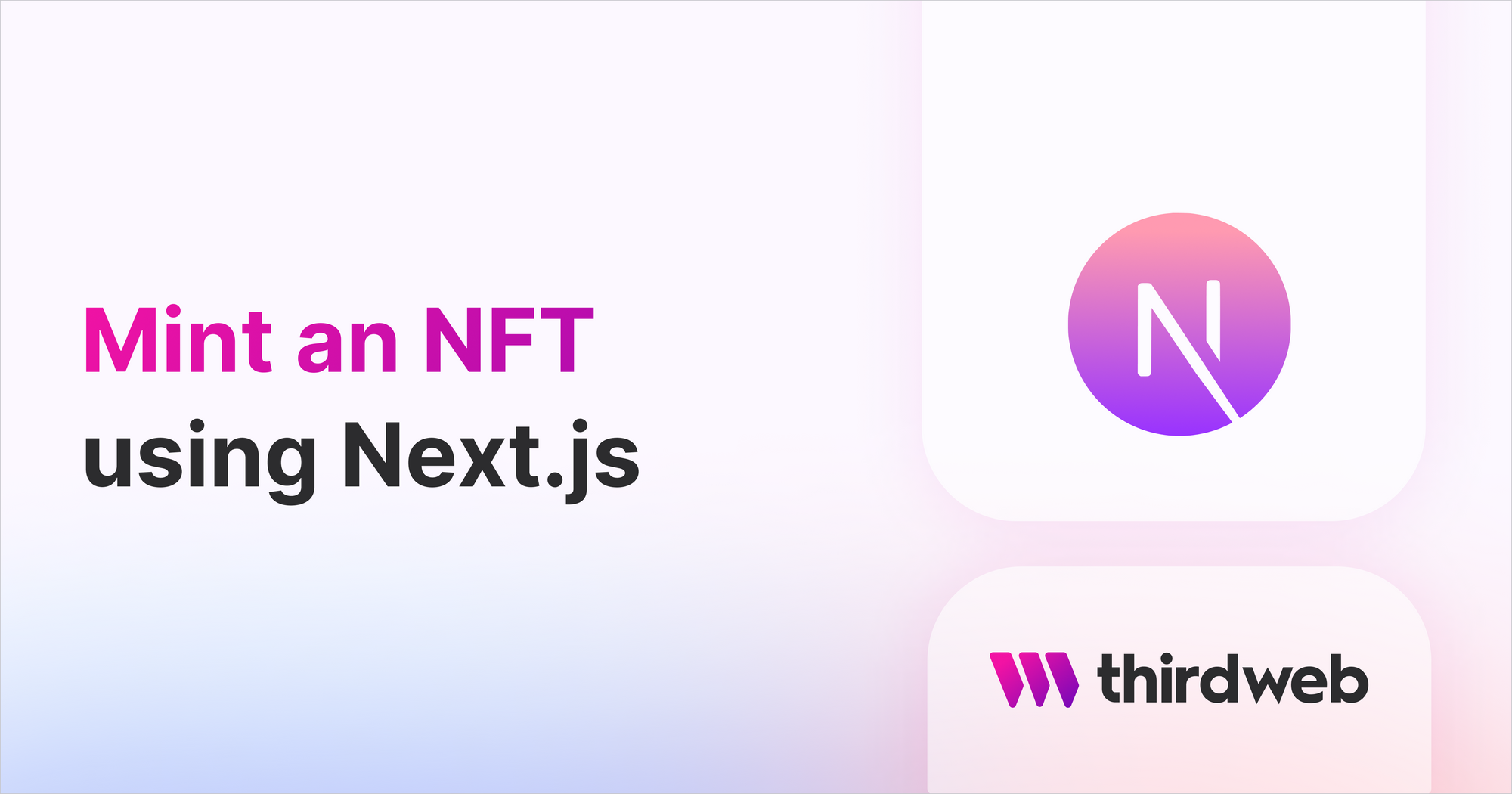
⚠️ Warning: This guide currently uses v4 of the Connect SDK. For v5 (latest) code snippets, please check out our documentation while this guide is being updated. ⚠️
In this guide, we'll show you how to use Next.js to:
- View all NFTs in an NFT Collection
- Allow a connected wallet to mint new NFTs if they have the
minter
role.
Create an NFT Collection contract
- Go to https://thirdweb.com/dashboard, connect your wallet and click on the Deploy new contract button.
- Select the NFT Collection from the Pre-built contracts and click Deploy Now
- Enter the metadata for your contract, such as image, name, symbol, description and royalty fees.
- Select the network you want to deploy to, learn more about the available networks in our Which Blockchain & Network Should I Use Guide
- Click Deploy Now and confirm the transaction in your wallet.
- Click to copy the Contract Address at the top left of the page.
Creating the Project
You can create a new Next.js app with our Web3 SDK pre-configured by using the CLI:
npx thirdweb create --next my-minting-app
Alternatively, install our Web3 SDKs into your Next app:
npm install @thirdweb-dev/sdk @thirdweb-dev/react ethers@5
Setting up the ThirdwebProvider
Inside the _app.js
file, we wrap our application in the ThirdwebProvider
:
This comes pre-configured when you use our CLI.
Be sure to change the activeChainId
to the chain you deployed to.
import { ChainId, ThirdwebProvider } from "@thirdweb-dev/react";
// This is the chainId your dApp will work on.
const activeChainId = ChainId.Mumbai;
function MyApp({ Component, pageProps }) {
return (
<ThirdwebProvider desiredChainId={activeChainId}>
<Component {...pageProps} />
</ThirdwebProvider>
);
}
export default MyApp;
Viewing all NFTs from the collection
We can use the useNFTCollection and
useNFTs hooks from the React SDK to:
- Get the NFT Collection smart contract in our application
- View all the NFTs and their metadata
Use this code in your index.js
file:
import {
ThirdwebNftMedia,
useNFTCollection,
useNFTs,
} from "@thirdweb-dev/react";
export default function NFTCollectionView() {
// Get the NFT collection using its contract address
const nftCollection = useNFTCollection(
"your-nft-collection-contract-address-here",
);
// Load all the NFTs from the collection (with a loading flag)
const { data: nfts, isLoading } = useNFTs(nftCollection);
// .map over the nfts array, rendering each NFT as a div containing the media asset and name.
return (
<div>
{!isLoading ? (
<div>
{nfts?.map((nft) => (
<div key={nft.metadata.id.toString()}>
<ThirdwebNftMedia metadata={nft.metadata} />
<h3>{nft.metadata.name}</h3>
</div>
))}
</div>
) : (
<p>Loading...</p>
)}
{/* Here's where we put the next section (the mint button) */}
</div>
);
}
We use the ThirdwebNftMedia to display the NFT's media asset, which
is a component that renders the asset correctly based on the file type. e.g. uses an <img>
tag if the
NFT media is an image, or a <video>
tag if it's a video.
Minting new NFTs
For a wallet to mint NFTs in an NFT Collection contract, they need to have the minter
role on the contract.
You can configure this via the dashboard, in the Permissions tab of your contract, by adding a wallet address in the Creator
section.
We can use the useMintNFT hook from the React SDK to mint new NFTs from the connected wallet address.
import { useMintNFT } from "@thirdweb-dev/react";
const { mutate: mintNft, isLoading, error } = useMintNFT(nftCollection);
Then, beneath our previous div
, we can add a button that calls this function with some NFT metadata:
To call the mintNft
function, we'll need a signer to sign the mint transaction, so we can show the user a "Connect Wallet" button if they are not connected.
To do that, we can use the useAddress
hook to view the connected address, and the useMetamask
hook to connect the wallet:
import { useAddress, useMetamask } from "@thirdweb-dev/react";
const address = useAddress();
const connectWithMetamask = useMetamask();
Then show a different button depending on the value of address
:
{
address ? (
<button
onClick={() =>
mintNft({
metadata: {
name: "My awesome NFT",
},
to: address,
})
}
>
Mint!
</button>
) : (
<button onClick={() => connectWithMetamask()}>Connect Wallet</button>
);
}