Mint an NFT Collection with TypeScript
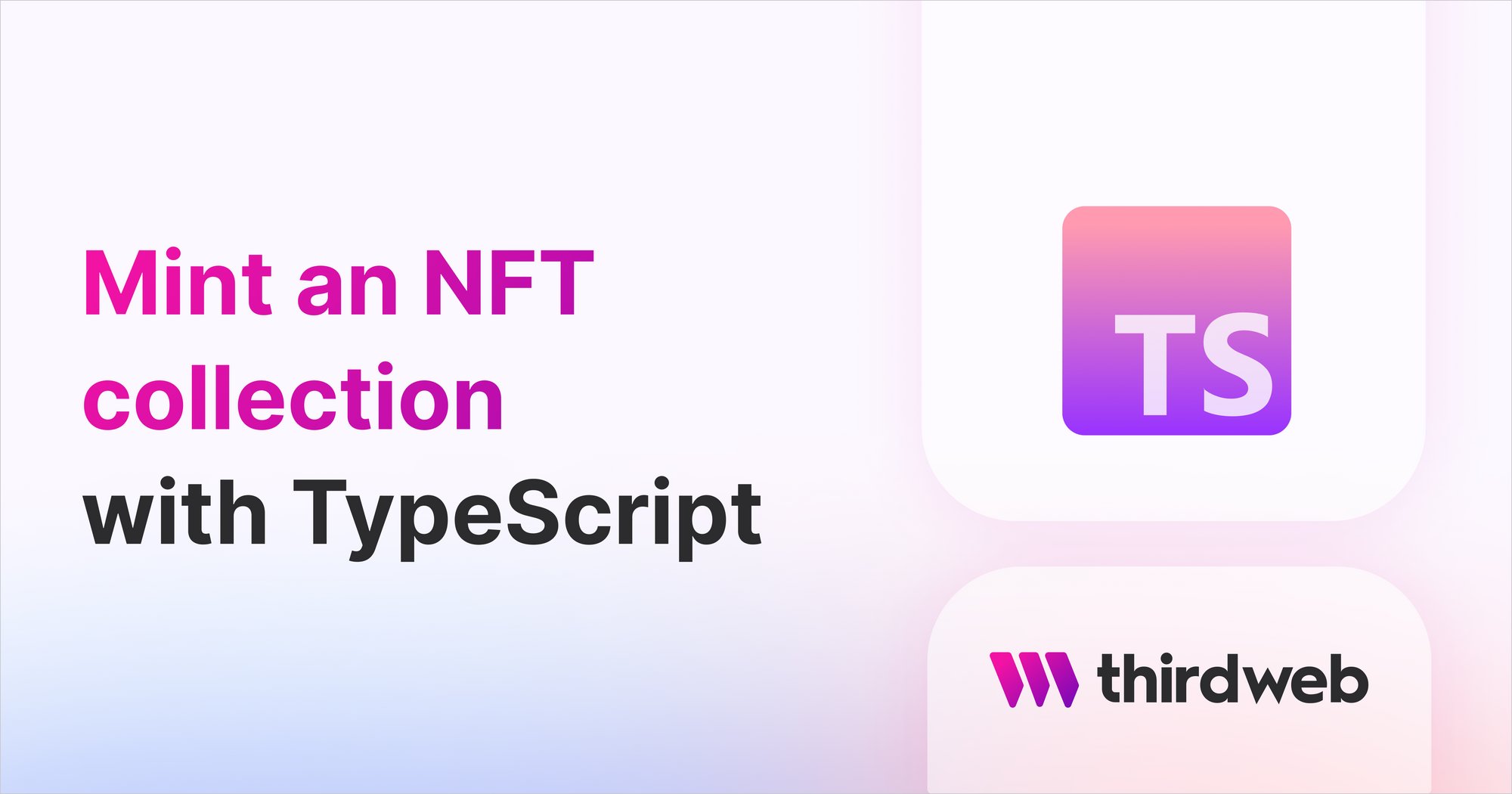
⚠️ Warning: This guide currently uses v4 of the Connect SDK. For v5 (latest) code snippets, please check out our documentation while this guide is being updated. ⚠️
You can deploy an NFT Collection via the dashboard,
but in this guide, we'll demonstrate how to do it using TypeScript!
Create a new directory called my-nft-collection
, and within this folder, create a file called mint-nft-collection.ts
.
Installing TypeScript SDK
Install the required dependencies to get started with thirdweb, by running the following command inside your my-nft-collection
folder.:
npm install @thirdweb-dev/sdk
Deploying an NFT Collection
To deploy an NFT Collection using the SDK, we can make use of the sdk.deployer,
which can create any of our pre-built contracts!
To deploy from your wallet, you'll first need to export your wallet's private key.
Learn how to export your private key from your wallet.
Ensure you store and access your private key securely.
- Never commit any file that may contain your private key to your source control.
Learn more about securely accessing your private key.
Now we're ready to deploy the NFT Collection!
import { ThirdwebSDK } from "@thirdweb-dev/sdk";
async function main() {
const NETWORK = "mumbai";
// Learn more about securely accessing your private key: https://portal.thirdweb.com/web3-sdk/set-up-the-sdk/securing-your-private-key
const sdk = ThirdwebSDK.fromPrivateKey("<your-private-key-here>", NETWORK);
const deployedAddress = await sdk.deployer.deployNFTCollection({
name: "My NFT Collection",
primary_sale_recipient: "0x-your-public-wallet-address-here",
});
console.log(deployedAddress);
// here, we'll add minting of NFTs in the next step.
}
main();
Now we are ready to deploy our NFT Collection, but before we do, let's mint some NFTs in our script.
Minting NFTs
The parameters used here are the same as minting an NFT inside the dashboard.
If you want to familiarize yourself with the process, check out the dashboard!
const nftCollection = sdk.getNFTCollection(deployedAddress);
const nft = await nftCollection.mintTo("0x-your-public-wallet-address-here", {
name: "My NFT",
});
console.log(nft);
So the only thing left is to run our code! Open a terminal and paste the following:
# Runs the TypeScript file
npx ts-node mint-nft-collection.ts
That's it!
You have minted your own NFT Collection using thirdweb and the TypeScript SDK, you should be proud!