How to Integrate Smart Contracts in a Unity Game
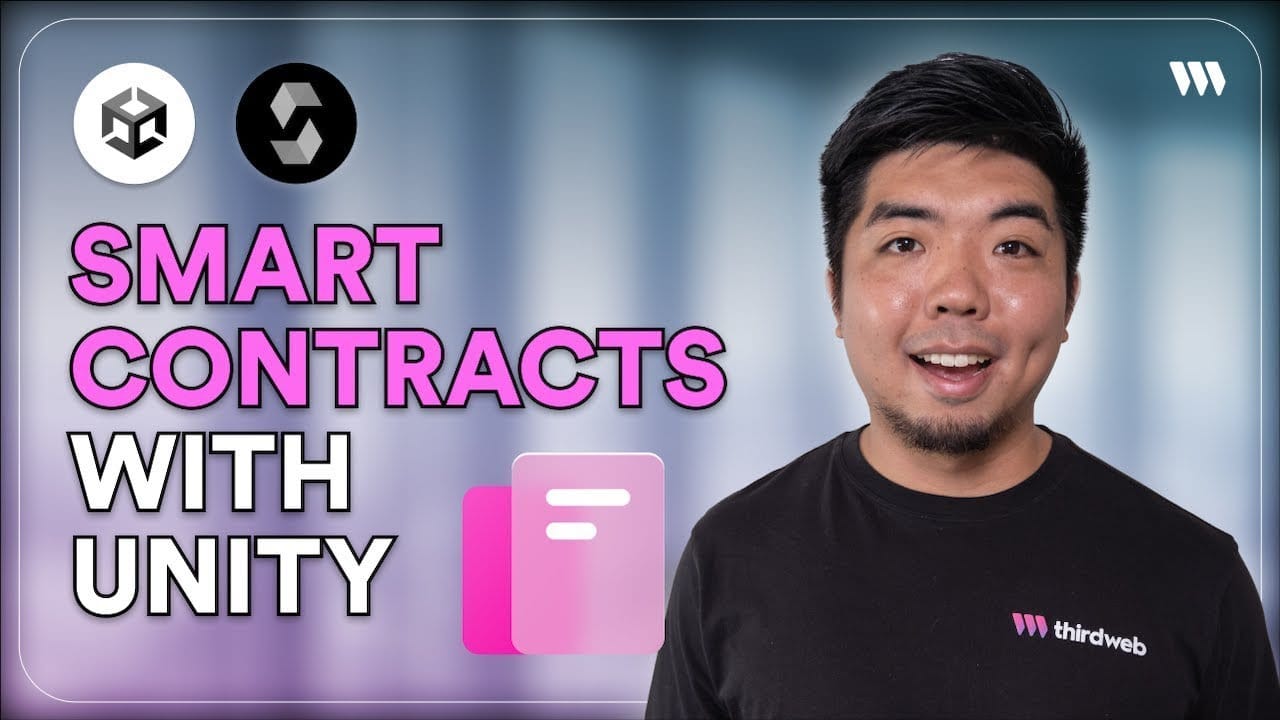
In this guide, we'll cover how to utilize smart contracts in your Unity game using the thirdweb Unity SDK. We'll go over interacting with different types of smart contracts like ERC20, ERC721, and ERC1155 smart contracts you deploy yourself.
By the end, you'll be able to easily read data from contracts and execute contract functions right from your Unity game. Let's get started!
Video Tutorial
Setting Up the Unity Project
To begin, create a new 3D project in Unity Hub and give it a name. Once the project opens in the Unity editor:
- Import the thirdweb Unity SDK package via Assets -> Import Package -> Custom Package.
- Ensure all items are selected and click Import.
- Go to the thirdweb prefabs folder and drag the thirdweb manager prefab into your scene hierarchy.
The thirdweb manager allows you to configure settings for using thirdweb in your game:
- Set the active chain to match the network your contracts are deployed to
- Input your thirdweb API key from the thirdweb dashboard
- Configure wallet connection settings
With the thirdweb manager set up, you're ready to start interacting with smart contracts.
Building the Game UI
For this example, we'll create a simple UI with sections to display info and interact with different types of contracts - ERC20, ERC721, and an ERC1155 smart contract.
- Create a new Canvas via right-click in hierarchy -> UI -> Canvas
- Add a Connect Wallet button using the prefab under thirdweb/Examples/Prefabs
- Create empty GameObjects for each contract section as children of the Canvas
- Add UI elements to each section:
- Text components to display titles and data like balances/totals
- Input fields to enter data
- Buttons to trigger contract interactions
Configuring the Backend
Create an empty GameObject and add a new script component called BlockchainManager. This will handle the logic for contract interactions.
Open the script and add the following:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using Thirdweb;
using TMPro;
public class BlockchainManager : MonoBehaviour
{
void Start()
{
var sdk = ThirdwebManager.Instance.SDK;
}
}
We initialize the ThirdwebSDK. This gives us access to all of thirdweb's functionality.
Next, let's go through adding support for each contract type.
Interacting with ERC20 Contracts
To use an ERC20 contract, we need to:
- Deploy an ERC20 contract via thirdweb dashboard (use the Token Drop contract)
- Copy the contract address
- Create a reference to the balance text in the UI
- Write functions to get the token balance and claim tokens
The key steps in code:
// Create reference to UI
public GameObject ERC20TokenBalanceText;
private string tokenContractAddress = "0x1234";
// Get token balance
public async void GetTokenBalance()
{
string address = await sdk.wallet.GetAddress();
Contract contract = sdk.GetContract(tokenContractAddress);
var data = await contract.ERC20.BalanceOf(address);
ERC20TokenBalanceText.GetComponent<TMPro.TextMeshProGUI>().text = data.displayValue;
}
// Claim tokens
public async void ClaimERC20Token()
{
Contract contract = sdk.GetContract(tokenContractAddress);
var data = await contract.ERC20.Claim("10");
GetTokenBalance();
}
Interacting with ERC721 Contracts
The process is similar for an ERC721 contract:
- Deploy ERC721 contract (NFT Drop) and configure claim conditions
- Reference the balance text and write functions to get NFT balance and claim NFTs
// Create reference to UI
public GameObject NFTBalanceText;
private string nftContractAddress = "0x1234";
// Get owned NFTs
public async void GetNFTBalance()
{
string address = await sdk.wallet.GetAddress();
Contract contract = sdk.GetContract(nftContractAddress);
List<NFT> nftList = await contract.ERC721.GetOwned(address);
if(nftList.Count > 0)
{
NFTBalanceText.GetComponent<TMPro.TextMeshProGUI>().text =
"Total: " + nftList.Count;
}
else
{
NFTBalanceText.GetComponent<TMPro.TextMeshProGUI>().text =
"Total: 0";
}
}
// Claim NFT
public async void ClaimERC20Token()
{
Contract contract = sdk.GetContract(nftContractAddress);
var data = await contract.ERC721.Claim(1);
GetNFTBalance();
}
Interacting with ERC1155 Contracts
For ERC1155:
- Deploy Edition Drop contract
- Reference item balance texts
- Write functions to get item balances and claim items by token ID
// Create reference to UI
public GameObject ItemBalance01;
public GameObject ItemBalance02;
private string itemContractAddress = "0x1234";
// Get balance of token 0
public async void GetItemBalance()
{
string address = await sdk.wallet.GetAddress();
Contract contract = sdk.GetContract(itemContractAddress);
var item1Balance = await contract.ERC1155.BalanceOf(address, "0");
var item2Balance = await contract.ERC1155.BalanceOf(address, "1");
ItemBalance01.GetComponent<TMPro.TextMeshProGUI>().text =
"Item: " + item1Balance;
ItemBalance02.GetComponent<TMPro.TextMeshProGUI>().text =
"Item: " + item2Balance;
}
// Claim item
public async void ClaimERC20Token(string tokenId)
{
Contract contract = sdk.GetContract(itemContractAddress);
var data = await contract.ERC1155.Claim(tokenId, 1);
GetItemBalance();
}
Connecting the Dots
With all the contract interactions in place, the final steps are:
- Trigger the token/NFT/item balance updates when the Connect Wallet button is clicked
- Hook up the claim buttons to call the respective claim functions
- Display updated balances in the UI
Wrapping Up
In this guide, we covered how to utilize various smart contracts in your Unity game using the thirdweb Unity SDK. We went through the process of setting up a Unity project with thirdweb, building a simple game UI, and writing code to interact with ERC20, ERC721, and ERC1155 smart contracts.
The key steps were:
- Deploying the desired contracts via the thirdweb dashboard
- Referencing UI elements in scripts
- Writing functions to read contract data and execute transactions
- Triggering updates on wallet connection and button clicks
- Displaying the updated data in the game UI
By using thirdweb's Unity SDK, you can easily integrate blockchain functionality into your game without dealing with the complexities of web3 development directly. The SDK provides a simple, intuitive interface for interacting with smart contracts.
We hope this guide helped you understand the process of building web3 games with Unity and thirdweb. The potential for blockchain-based games is immense, and we can't wait to see what you build!
If you have any questions or need further assistance, feel free to reach out via the thirdweb support channels. Happy building!