How to Support Multiple Chains In Your thirdweb Dapp
This guide will show you how to create a Dapp that works on multiple chains. In this Dapp we will allow users to select chains and claim an NFT from the respective chain.
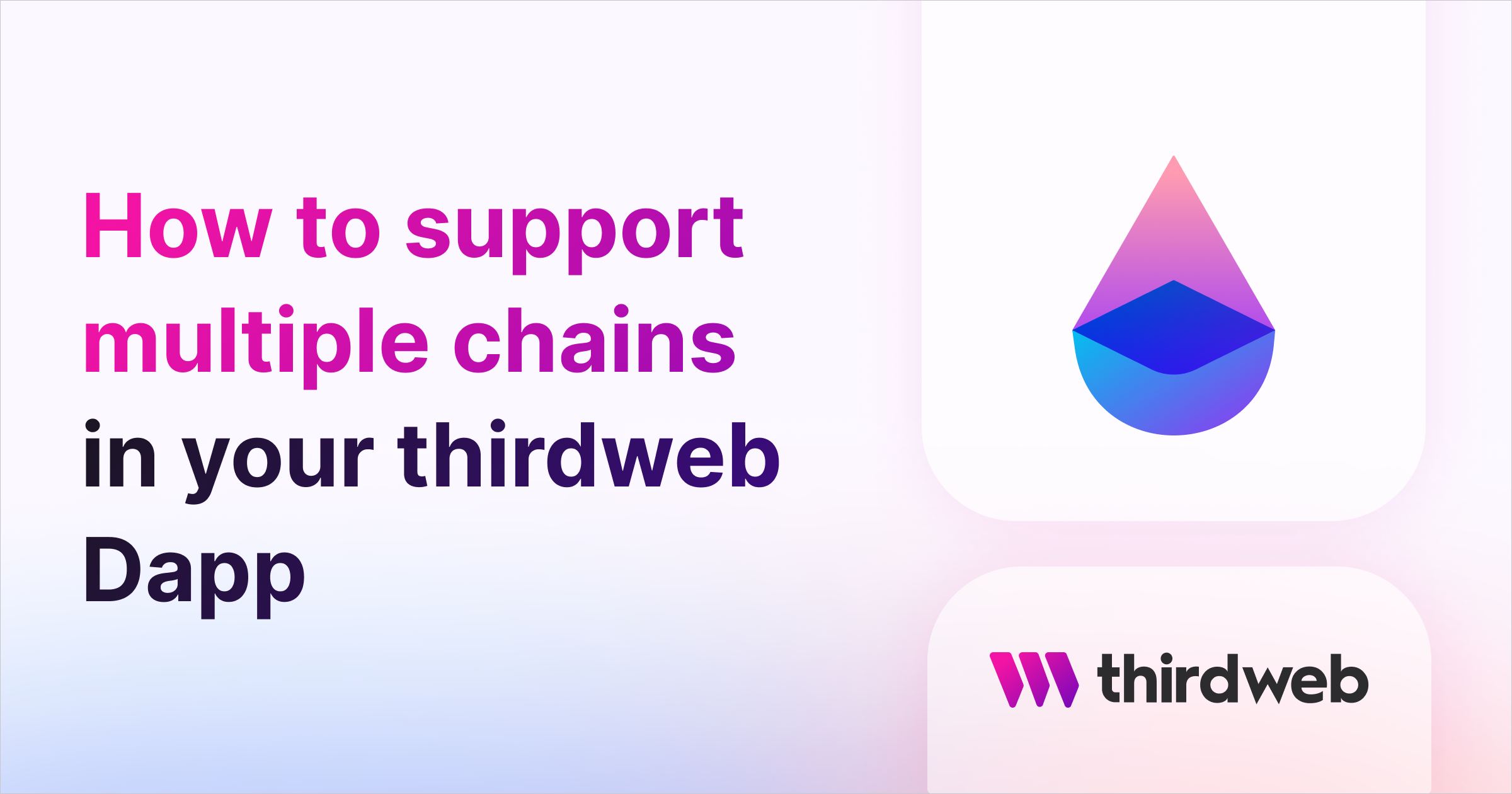
This guide will show you how to create a Dapp that works on multiple chains. In this Dapp we will allow users to select chains and claim an NFT from the respective chain. I am going to show Goerli and Mumbai but you could do it on any network and go crazy with it.
Before we get started, below are some helpful resources where you can learn more about the tools we will use in this guide.
Let's get started.
Setup
Setting up our project
I am going to use the Next.js Typescript starter template for this guide.
If you are following along with the guide, you can create a project with the template using the thirdweb CLI:
npx thirdweb@latest create app --next --ts
If you already have a Next.js app you can simply follow these steps to get started:
Install @thirdweb-dev/react
and @thirdweb-dev/sdk
and ethers@5
.
Add MetaMask authentication to the site. You can follow this guide to add metamask auth.
Finally, I have created 2 NFT Drops for our Dapp, one in Mumbai and one in Goerli. So that we can test out our Dapp. You can use whatever contract that you want to have in your Dapp!
Getting into the code
Creating a context to store chain
Create a new file called Context/Chain.ts
and add the following:
import { createContext } from "react";
const ChainContext = createContext({
selectedChain: "goerli",
setSelectedChain: (chain: string) => {},
});
export default ChainContext;
This will allow us to create a global state for storing and changing our chain.
Now, head over to _app.tsx
and replait ce with the following:
import type { AppProps } from "next/app";
import { useState } from "react";
import ChainContext from "../context/Chain";
import { ThirdwebProvider } from "@thirdweb-dev/react";
function MyApp({ Component, pageProps }: AppProps) {
const [selectedChain, setSelectedChain] = useState("mumbai");
return (
<ChainContext.Provider value={{ selectedChain, setSelectedChain }}>
<ThirdwebProvider activeChain={selectedChain}>
<Component {...pageProps} />
</ThirdwebProvider>
</ChainContext.Provider>
);
}
export default MyApp;
We are adding the react context here, so we can access the state everywhere!
Creating dropdown for networks
We will create a simple dropdown for users to switch between networks. So, in pages/index.tsx
add this select element that will create a dropdown:
<select
value={String(selectedChain)}
onChange={(e) => setSelectedChain(e.target.value)}
>
<option value="mumbai">Mumbai</option>
<option value="goerli">Goerli</option>
</select>
We need to access the react context state that we just created, so we will use the useContext
hook to do this:
const { selectedChain, setSelectedChain } = useContext(ChainContext);
We also need to import this:
import ChainContext from "../context/Chain";
Now, we will create a JSON object that will have the addresses of the contract addresses:
const addresses: Record<string, string> = {
["mumbai"]: "0x25CB5C350bD3062bEaE7458805Fb069200e37fD5",
["goerli"]: "0xA72234a2b9c1601593062f333D739C93291dF49F",
};
This is creating a string key of the chain with the contract address on their respective chains.
We will use the Web3Component
for performing functions. Since I created an NFT Drop I am calling the call function but it might be different for you!
<Web3Button
contractAddress={addresses[selectedChain]}
action={(contract) => contract.erc721.claim(1)}
>
Claim
</Web3Button>
If we go to localhost and check out the app, we can switch between networks and it all works completely fine! 🎉
Conclusion
In this guide, we learnt how to allow users to claim NFTs from different NFTs in the same Dapp using the react sdk. If you have any queries hop on the thirdweb discord! If you want to take a look at the code, check out the GitHub Repository.