Request to Switch Networks After Connecting a Wallet
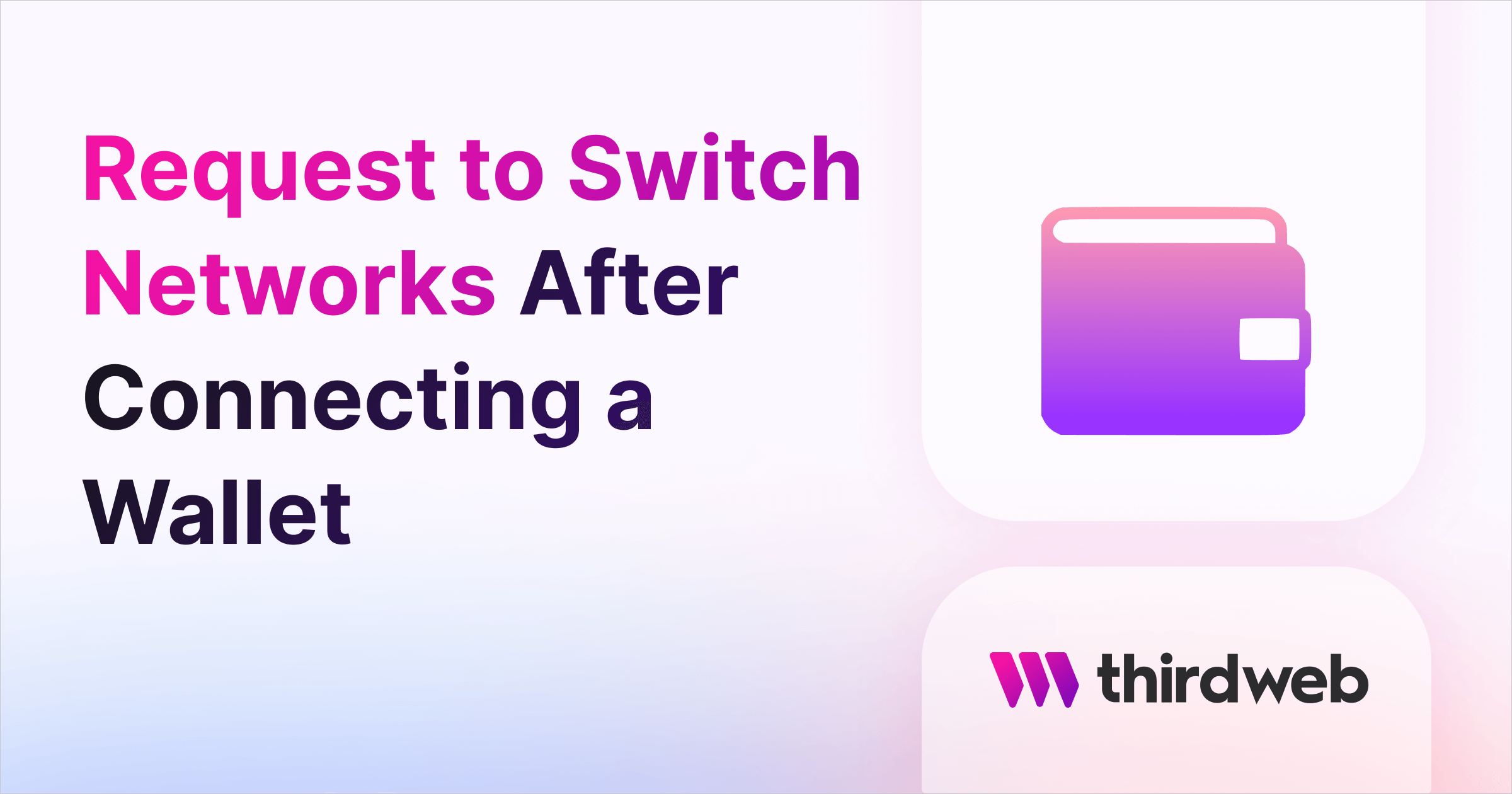
⚠️ Warning: This guide currently uses v4 of the Connect SDK. For v5 (latest) code snippets, please check out our documentation while this guide is being updated. ⚠️
Switching networks, and finding the correct network can be a painful process. In this tutorial, we will see how thirdweb's SDK simplifies this process and keeps the user on the network to which the contract is deployed.
First, we will create a new Next.js project using the following command.
npx thirdweb@latest create app --next
Press Enter
on all the options it provides you after the command is executed.
Now you should have a Next.js project ready to go.
To begin, we will head over to our _app.js
file.
You should be able to see something similar in your _app.js
file.
import { ThirdwebProvider } from "@thirdweb-dev/react";
import "../styles/globals.css";
// This is the chain your dApp will work on.
// Change this to the chain your app is built for.
// You can also import additional chains from `@thirdweb-dev/chains` and pass them directly.
const activeChain = "ethereum";
function MyApp({ Component, pageProps }) {
return (
<ThirdwebProvider activeChain={activeChain}>
<Component {...pageProps} />
</ThirdwebProvider>
);
}
export default MyApp;
Now let's look at how we can use the React SDK's hooks to detect an incorrect chain connection.
We will create a new component and import the useNetworkMismatch
hook from the React SDK.
import { useNetworkMismatch } from "@thirdweb-dev/react";
export const Detector = () => {
const isMismatched = useNetworkMismatch();
return <div>{isMismatched}</div>;
};
After rendering this component, you will be able to see if you are connected to the correct network or not.
We can also use the useNetwork
hook to request users to change networks and create another component.
import { useNetworkMismatch, useNetwork, ChainId } from "@thirdweb-dev/react";
// Here, we show a button to the user if they are connected to the wrong network
// When they click the button, they will be prompted to switch to the desired chain
export const Network = () => {
const isMismatched = useNetworkMismatch();
const [, switchNetwork] = useNetwork();
return (
<div>
<p>{isMismatched}</p>
{isMismatched && (
<button onClick={() => switchNetwork(ChainId.Mainnet)}>
Switch Network
</button>
)}
</div>
);
};
If you are connected to the wrong network, you should be able to see a switch network
button that will connect you to the correct network.
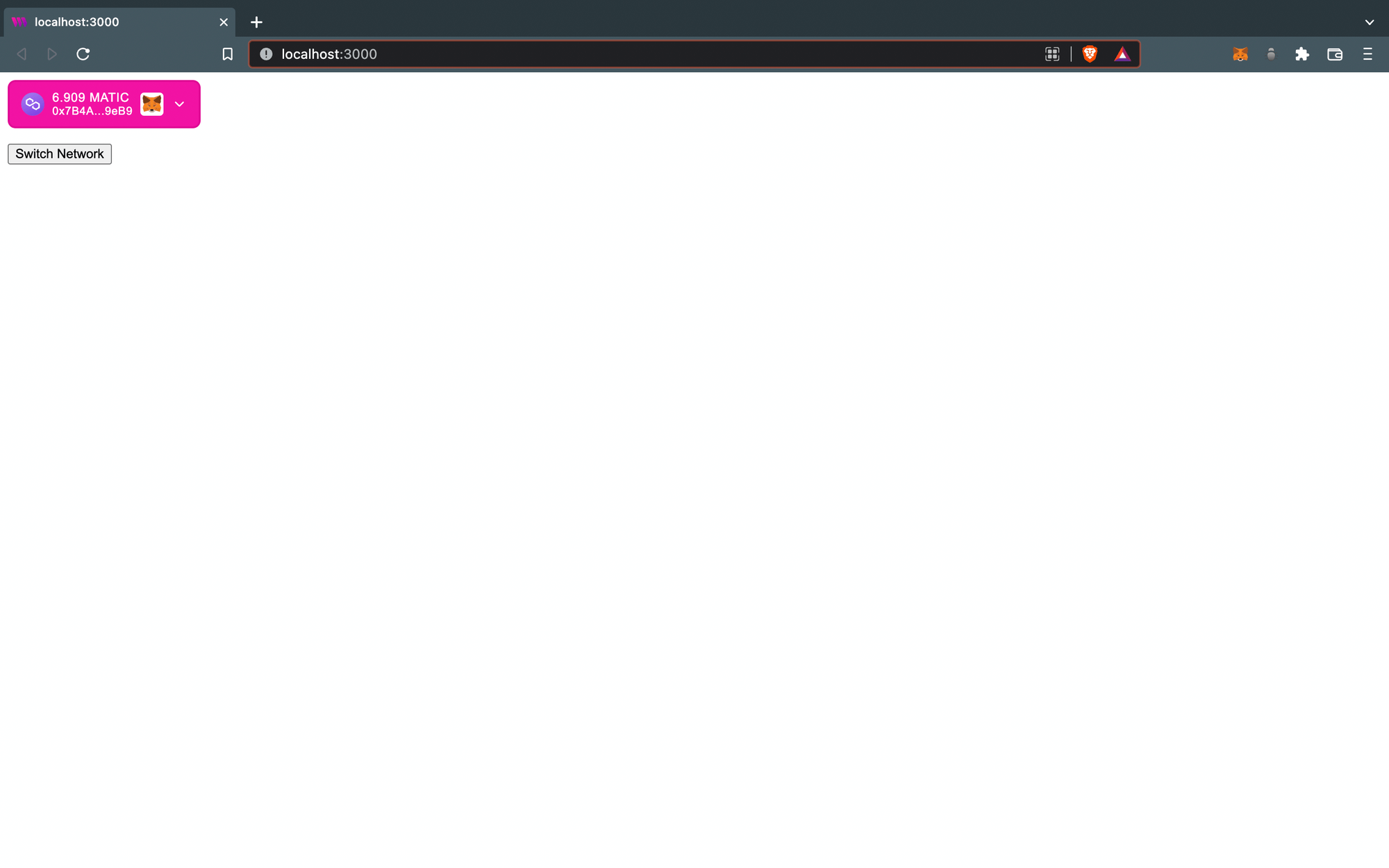
Now let's go ahead and see how we can listen for when the user connects their wallet, and then immediately ask them to switch to the desired chain.
We will create a new component called AutoConnect
which will require the user to automatically change their network to the deployed contract network every time the user connects their wallet.
import { useEffect } from "react";
import {
ChainId,
useNetworkMismatch,
useNetwork,
useChainId,
ConnectWallet,
useAddress
} from "@thirdweb-dev/react";
export const AutoConnect = () => {
const address = useAddress(); // Get connected wallet address
const [, switchNetwork] = useNetwork(); // Switch to desired chain
const isMismatched = useNetworkMismatch(); // Detect if user is connected to the wrong network
useEffect(() => {
// Check if the user is connected to the wrong network
if (isMismatched) {
// Prompt their wallet to switch networks
switchNetwork(ChainId.Mainnet); // the chain you want here
}
}, [address]); // This above block gets run every time "address" changes (e.g. when the user connects)
return <ConnectWallet/>
};
Conclusion
We were able to ensure that the user is connected to the correct network using different methods and also learned how the React SDK's hooks help us in doing that accurately. If you still happen to have any questions, Join our discord and feel free to ask us.