Add Sign In With Ethereum (SIWE) to a React App
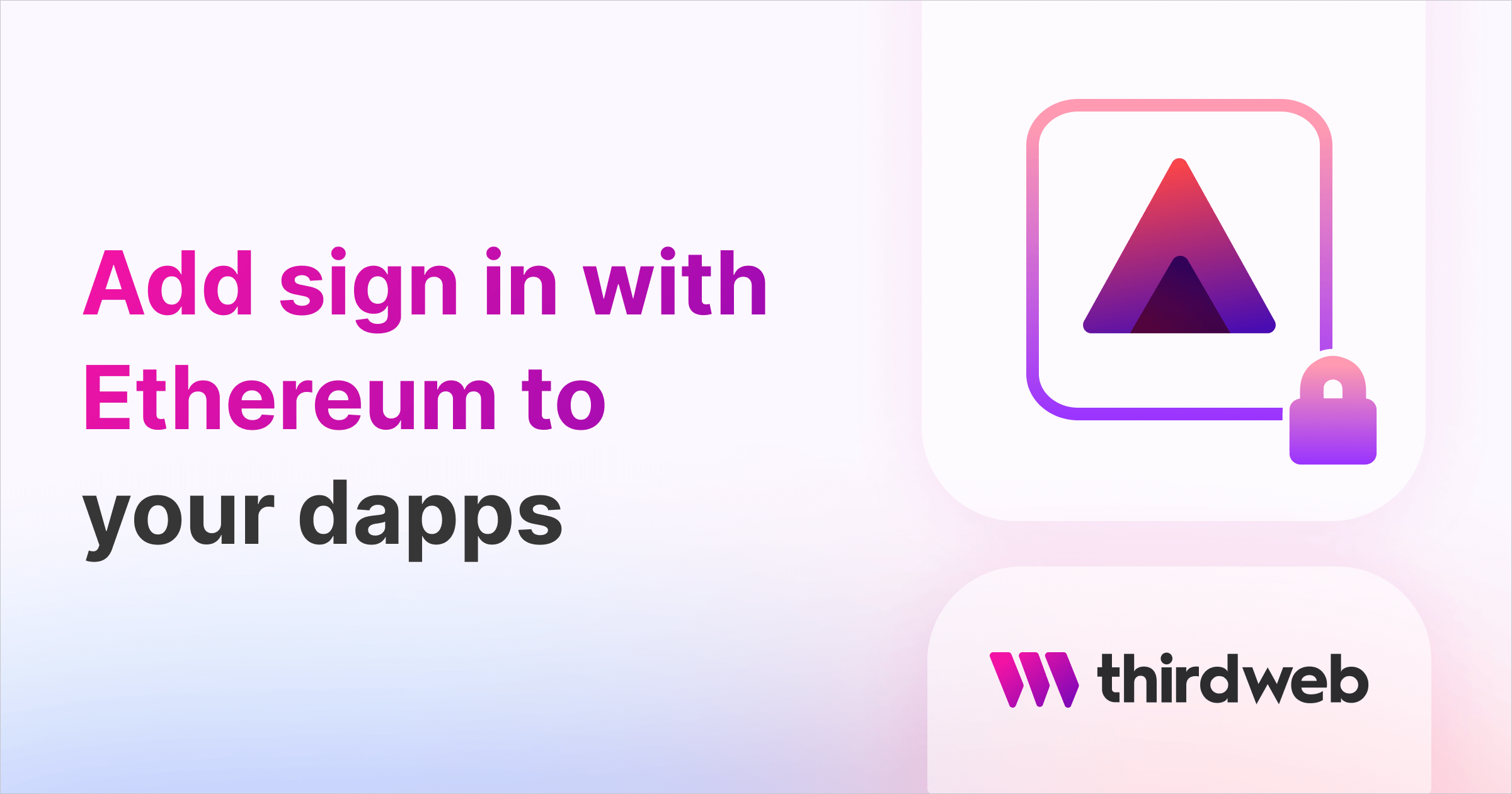
Introduction
⚠️ Warning: This guide currently uses v4 of the Connect SDK. For v5 (latest) code snippets, please check out our documentation while this guide is being updated. ⚠️
In this guide, we are going to use Auth to add sign in with Ethereum to our app!
By the end, we'll have a full React application that allows users to connect their wallet and sign in with Ethereum that looks like this:
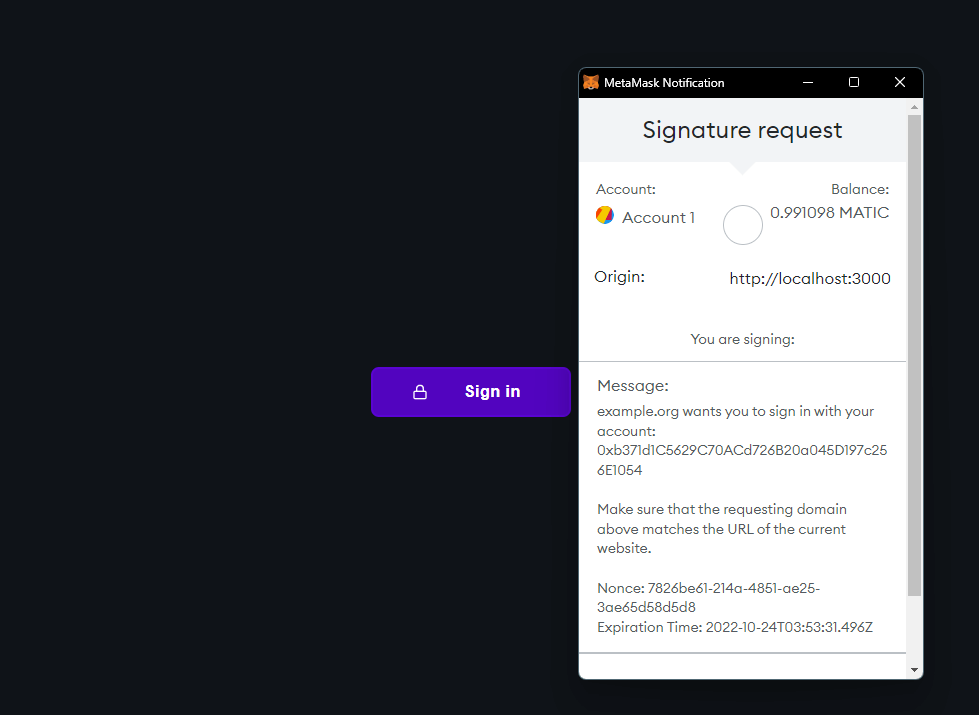
You can get started with the full code we write in this guide by running:
npx thirdweb create --template sign-in-button
Let's begin!
Why use web3 sign-in?
Sign-in with Ethereum allows you to securely log in using a wallet and verify the wallet on the backend! Our Auth SDK utilizes JSON Web Tokens (JWT) to prove a user owns a wallet in a server-side environment.
Setup
Creating Next.js App
I am going to use the Next javascript starter template for this guide.
If you are following along with the guide, you can create a project with the
Next Javascript template using the thirdweb CLI:
npx thirdweb create app --next --js
If you already have a Next.js app you can simply follow these steps to get started:
- Install
@thirdweb-dev/react
and@thirdweb-dev/sdk
andethers@5
- Add MetaMask authentication to the site. You can follow this guide to do this.
Setting up thirdweb auth
Firstly, we need to install the thirdweb auth package:
npm i @thirdweb-dev/auth # npm
yarn add @thirdweb-dev/auth # yarn
Now, create a file called auth.config.js
and the following:
import { ThirdwebAuth } from "@thirdweb-dev/auth/next";
import { PrivateKeyWallet } from "@thirdweb-dev/auth/evm";
export const { ThirdwebAuthHandler, getUser } = ThirdwebAuth({
domain: process.env.NEXT_PUBLIC_THIRDWEB_AUTH_DOMAIN as string,
wallet: new PrivateKeyWallet(process.env.THIRDWEB_AUTH_PRIVATE_KEY || ""),
});
Learn how we suggest using a secret manager to securely store your private key.
To configure the auth API, create a new folder inside pages/api
called auth
and a [...thirdweb].js
file inside it!
From within this file, we need to export the ThirdwebAuthHandler
that we created!
import { ThirdwebAuthHandler } from "../../../auth.config";
export default ThirdwebAuthHandler();
Finally, inside the _app.tsx
file, add the authConfig
prop to ThirdwebProvider
:
<ThirdwebProvider
activeChain={activeChain}
authConfig={{
authUrl: "/api/auth",
domain: "example.org",
}}
>
<Component {...pageProps} />
</ThirdwebProvider>
Building the frontend
Inside pages/index.tsx
update the return statement with the following:
return (
<div>
<ConnectWallet
auth={{
loginOptional: false,
}}
/>
{user && <p>You are signed in as {user.address}</p>}
</div>
);
Here, we are using the thirdweb's ConnectWallet
which also has an auth option!
We can also use the useUser
hook to get the currently authenticated user:
import { ConnectWallet, useUser } from "@thirdweb-dev/react";
const { user } = useUser();
And, it was this easy to add sign in with Ethereum to a dapp with thirdweb!
Conclusion
In this guide, we learned how to use thirdweb auth to add sign in with Ethereum. Share your amazing Dapps that you built using sign in with Ethereum on the thirdweb discord! If you want to take a look at the code, check out the GitHub Repository.