Getting started with Hardhat
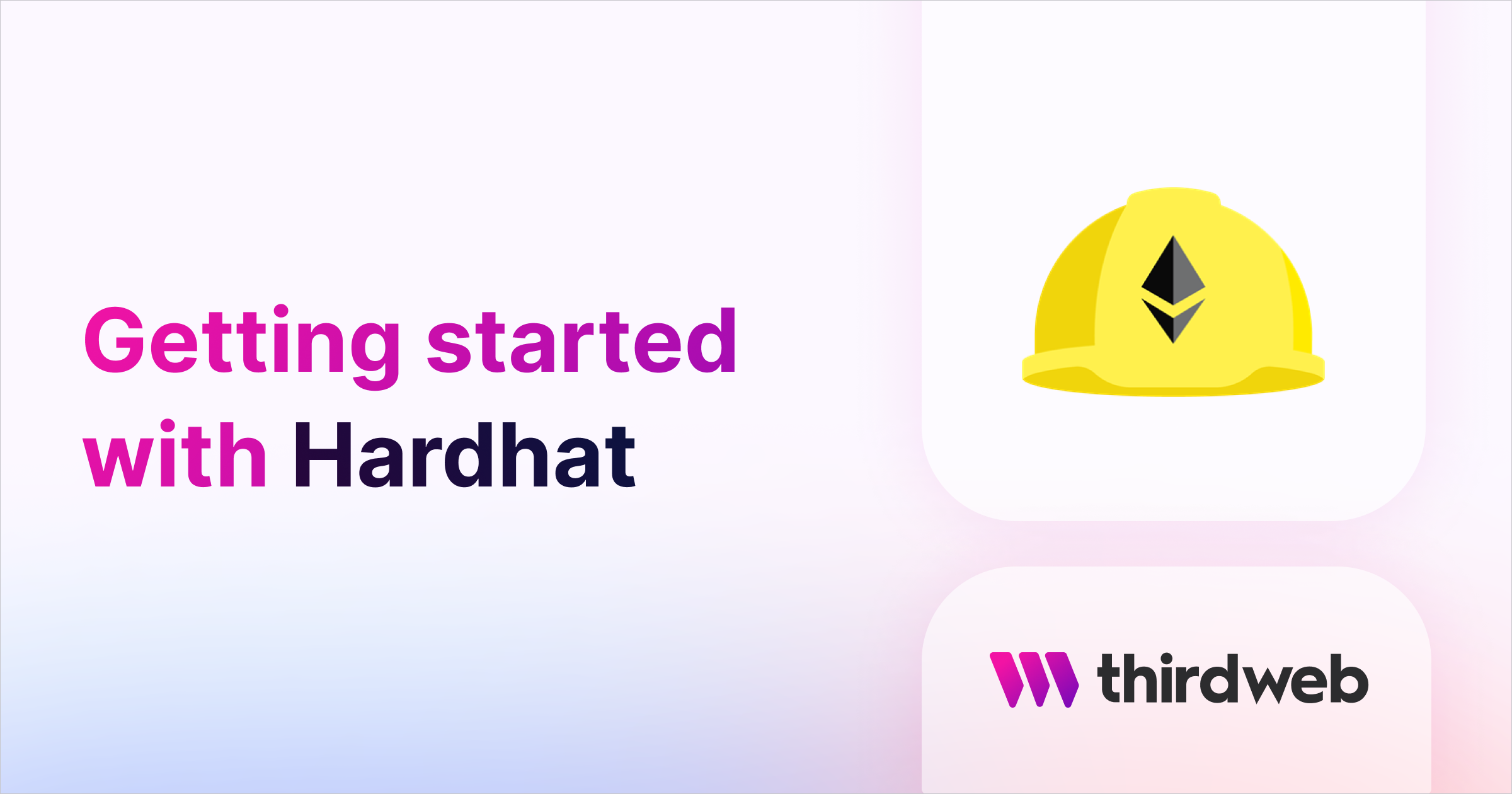
In this guide, we will look at what Hardhat is and how it can help you to develop smart contracts. We will also look at a basic tutorial to get you started with writing your smart contracts using Hardhat.
By the end of the guide, you should be able to create a Hardhat project and write & deploy smart contracts using it.
What is Hardhat?
Hardhat is a development environment for Ethereum smart contracts. It allows you to create, debug, test, and deploy smart contracts. It provides all the tools you need to help you debug and test your smart contracts, such as setting up a local node for testing and the ability to write tests within the project quickly.
In this guide, we will use Hardhat to write a simple ERC-721 smart contract and deploy it on your desired network.
Creating a Hardhat project
To create a new Hardhat project, go to a safe directory and run the following command to use the installation wizard:
npx thirdweb create contract
This should ask you some questions for setting up your project:
- Project name
- Framework to use, choose
Hardhat
here - Select ERC721 as the base contract
- Select
Drop
as an optional extension
Feel free to tinker around with the configuration and use the one that suits you the best. Since this guide covers creating an ERC-721 contract using Hardhat, we recommend choosing the above configuration.
The reason we use the CLI to set up the Hardhat project is that the CLI installs all the dependencies you need to get started, that includes the Solidity SDK (@thirdweb-dev/contracts
) that makes the process of writing smart contracts simpler for you.
Project structure
Now, let's look at the project structure Hardhat offers and the various features and configurations you can play around with.
contracts
folder
All the Solidity code you write should reside under the contracts folder. To get started, you can create a new Solidity file (ending with .sol) inside the contracts folder or edit the one that already exists within the folder.
hardhat.config.js
file
This is the Hardhat configuration file that holds all the configurations of your Hardhat project, like the Solidity version, bytecode optimizer, RPC setup, etc. This is your place to go if you want to change the behavior of how Hardhat compiles your contracts. You can check Hardhat configuration documentation for more information.
artifacts
folder
You may not see this folder if you open your project directory for the first time. This folder is generated by Hardhat whenever a contract is compiled. To compile the contracts in the contracts folder, run the following command:
npx thirdweb build
This should make Hardhat compile the contract and place related compilation information (such as bytecodes, ABIs, etc.) in the artifacts folder. A cache folder is also generated, although you probably would never need to open it.
Writing contracts
You can import any package you have installed through npm
or related package managers directly into your Solidity files. In that similar way, we have imported ERC721Drop
extension from @thirdweb-dev/contracts
package. You can check the auto-generated file created by the CLI inside the contracts
folder and you should have a similar file (considering you used the same configuration):
// SPDX-License-Identifier: Apache-2.0
pragma solidity ^0.8.0;
import "@thirdweb-dev/contracts/base/ERC721Drop.sol";
contract HardhatStarter is ERC721Drop {
constructor(
string memory _name,
string memory _symbol,
address _royaltyRecipient,
uint128 _royaltyBps,
address _primarySaleRecipient
)
ERC721Drop(
_name,
_symbol,
_royaltyRecipient,
_royaltyBps,
_primarySaleRecipient
)
{}
}
The above code is for a simple ERC-721 Drop contract that allows people to claim your NFTs lazy minted. Now, you can add additional functionality on top of this contract; let's create a function to mint NFTs to a specific wallet:
function mint(address _to, uint256 _amount) external {
require(_amount > 0, 'You must mint at least one token!');
_safeMint(_to, _amount);
}
Similarly, you can create new Solidity files in the contracts
folder and they will be treated as separate contracts by Hardhat with no additional configuration.
Deploying smart contracts
Traditionally, a private key was required in Hardhat configuration to deploy contracts to the blockchain. Although, using thirdweb CLI, you can deploy the contracts through your browser without involving private keys on any chain. Run the following command to deploy your contract:
npx thirdweb deploy
This should compile the contracts in the contracts folder, upload the contract data, such as the bytecode, to IPFS and open the browser to deploy the contract. You should see the following screen:
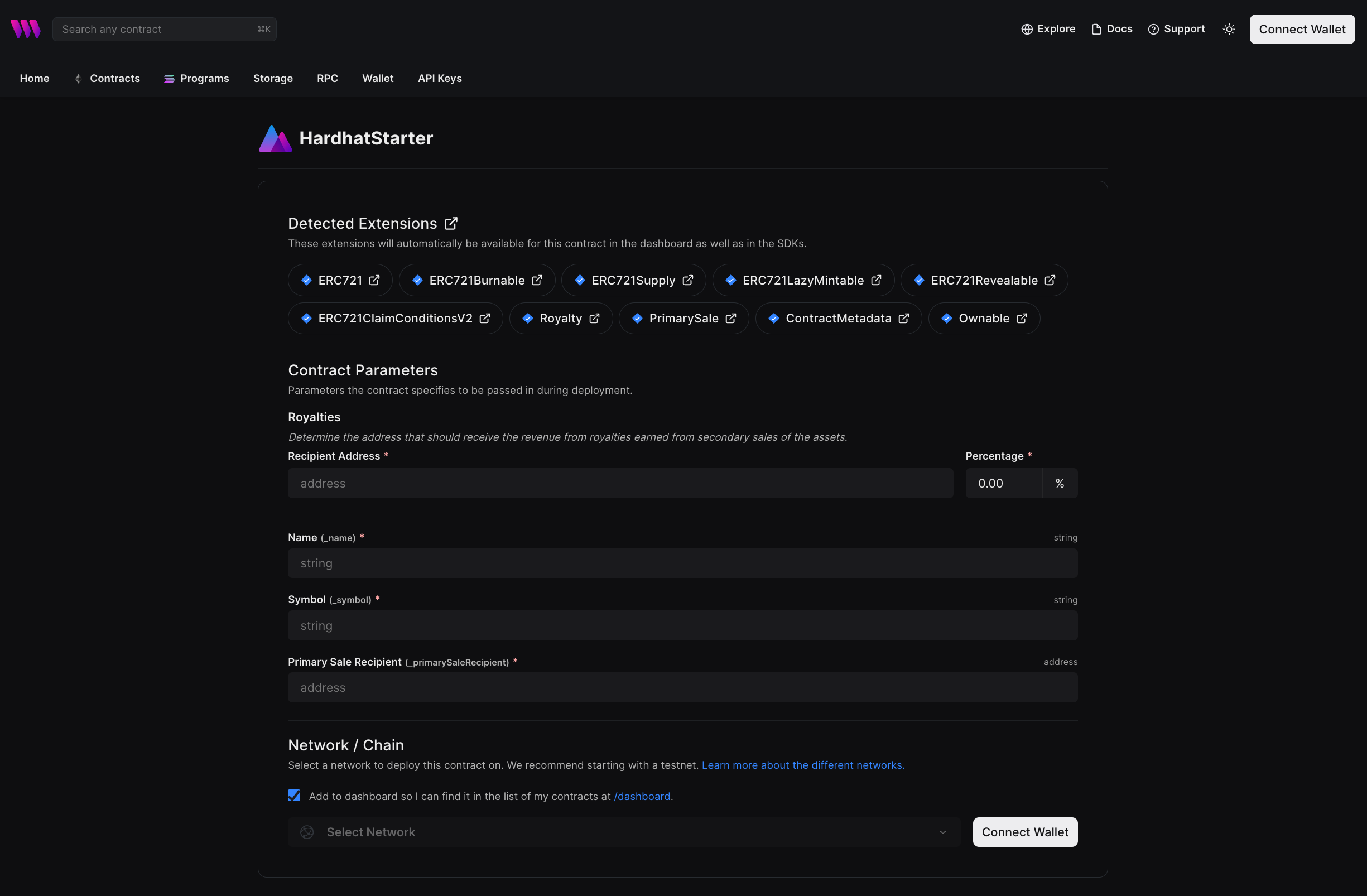
Now you can add the constructor parameters. If you use any royalty-based extension, thirdweb automatically detects it and presents you with a friendly percentage selector.
After filling in the constructor fields:
- Connect your wallet using the Connect Wallet button.
- Choose your desired network (we support all EVM networks).
- Click Deploy Now.
This should prompt a transaction request on your connected wallet, as deploying a contract requires gas fees and transaction authorization.
After approving the transaction, your contract should be deployed, and you should be redirected to the contract dashboard, where you can copy your contract address and manage the contract from your browser.
Wrapping up
Hardhat is a very powerful tool to write smart contracts. In this guide we saw how you can use Hardhat to write smart contracts.
If you have any question or need support, feel free to join our Discord server. For any feedback related to thirdweb, feel free to leave them in the feedback board.