Enhancing Unity IAP with Blockchain Interactions
On-ramp gamers to your chain using the power of Mobile In-App Purchasing!
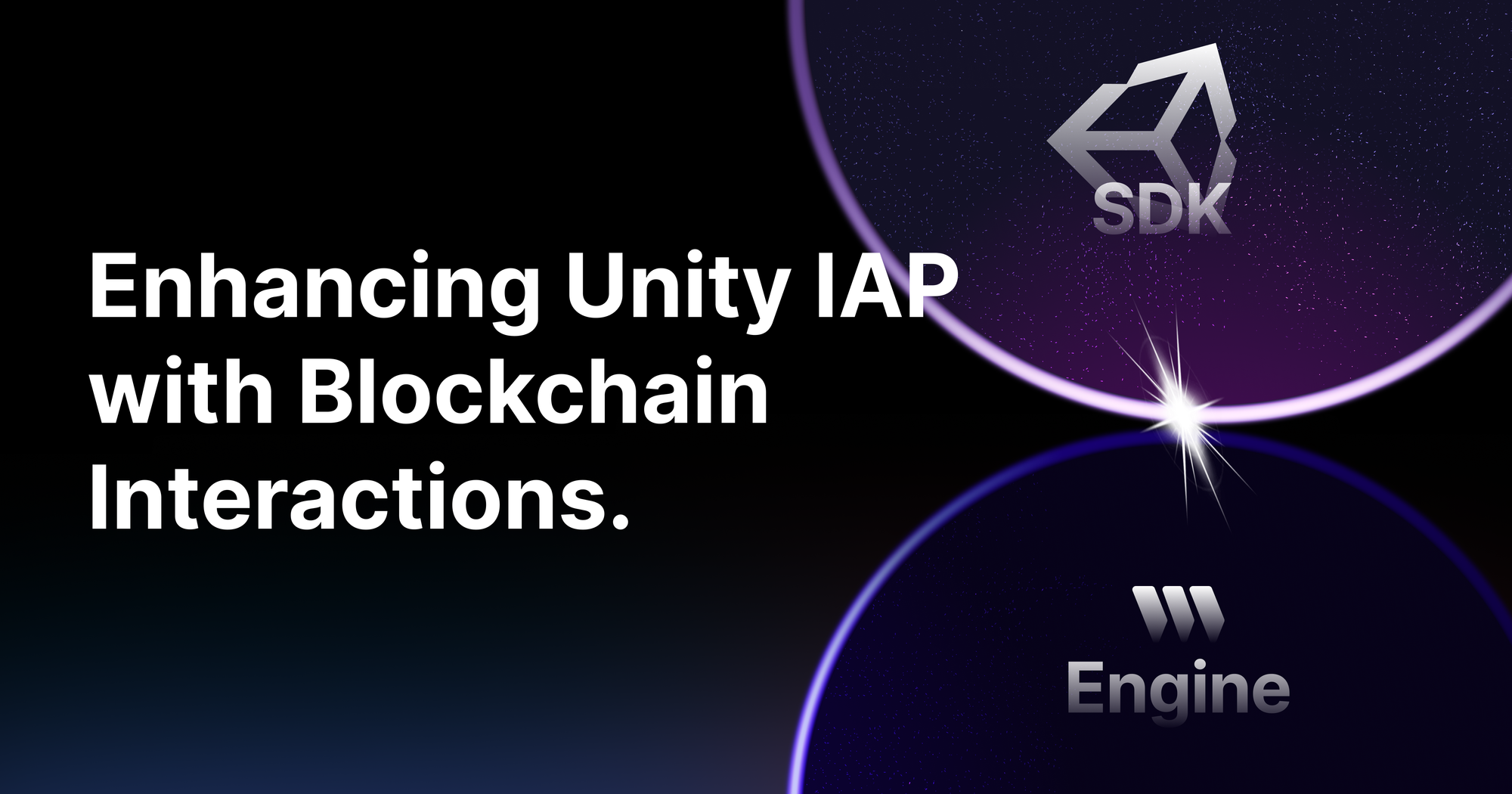
Introduction
This guide is tailored for intermediate or advanced game developers aiming to integrate Unity's In-App Purchases (IAP) with blockchain functionalities, creating a dynamic user experience by leveraging the power of the thirdweb Unity SDK and thirdweb Engine. This integration allows for a seamless blend of traditional gaming monetization with the innovative benefits of blockchain technology
I'm already a pro, just show me how to integrate the blockchain
We highly recommend still going through the guide, but we know some of you are experienced web2 game developers with IAP already in their projects, and want an example of how to enhance these rewards with even more blockchain rewards!
- Client Side: https://github.com/thirdweb-example/unity-iap-client
- Server Side: https://github.com/thirdweb-example/unity-iap-server
- thirdweb Unity SDK Documentation.
- thirdweb Engine Documentation.
Technology Used
Please refer to these links when they are mentioned in subsequent sections.
- Unity In-App Purchasing Package for client integration.
- Apple App Store In-App Purchase for store integration.
- Apple App Store Server API for server-side receipt validation using an In-App Purchase API Key for server-side authentication.
- Google Play Store In-App Products for store integration.
- Google Play Developer API for server-side receipt validation using a Google Cloud Service Account for server-side authentication.
- thirdweb Unity SDK for wallet connection and balance polling.
- thirdweb Engine for backend blockchain interactions.
- thirdweb API Key to enable all of the above (free).
This is a lot, I know! Thankfully, that's what this template is for, unifying all this tech stack into simple projects you can clone and test in different environments.
Whether you're familiar with IAP in Unity, or are reading through the above docs now, I believe it's time for you to start integrating! Practice makes perfect.
Part 1: Setting Up In-App Purchases (IAP) on Digital Stores
Overview of IAP in Unity
IAP in Unity simplifies - and unifies - interaction with different app stores and product types, using a single package and interface. It allows you to not only initialize each service, but also process transactions, obfuscate license keys and do some client side validation and receipt parsing before, in our case, validating server-side. This is extremely helpful as it allows us to control the flow every step of the way. Before we dive right into Unity, let's do some housekeeping.
Configuring IAP on iOS and Android
Before integrating IAP into your Unity project, you must first set up IAP on the respective digital stores. We'll focus on Mobile during this guide, using a simple consumable IAP product.
Important: You must fill in billing and other financial details for IAP to work at all, this is very important specially for licensed testing - the ability to use test cards. We will not go over setting up testing environments in detail, please refer to Apple and Google's documentation to do so. It is recommended to test live builds in Sandbox mode, more on that later.
Apple App Store Setup
- Log in to your Apple Developer account and navigate to "App Store Connect" to create an application.
- Select your app and go to the "Features" tab, then click on "In-App Purchases."
- Click "+", choose the type of IAP you want to create (e.g., consumable), and fill in the details such as product ID and price. Take note of the product ID.
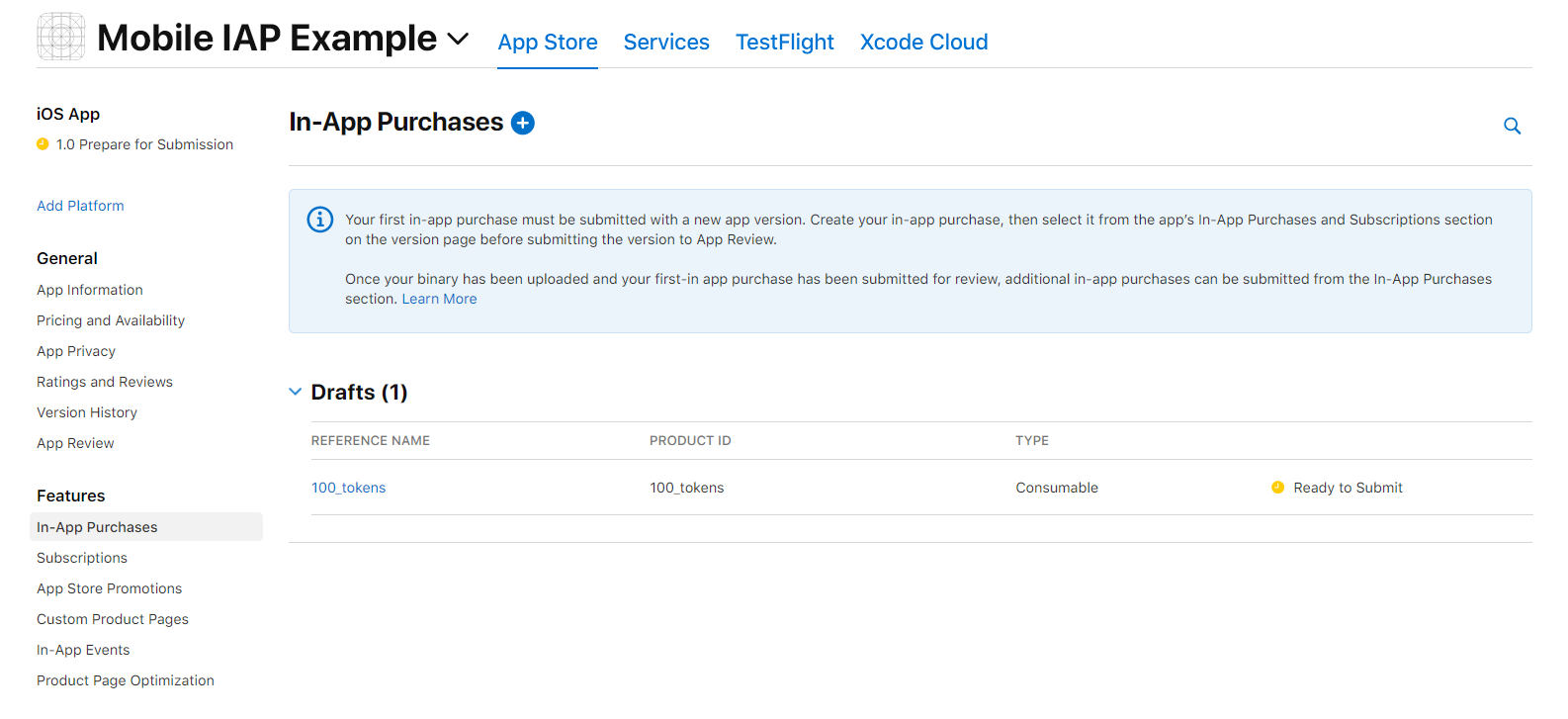
Google Play Store Setup
- Log in to your Google Play Console and select your app.
- Under the "Monetize" section, find "In-app products" and click on "Manage."
- Click on "Create product" and enter the product details, including the product ID and price. Take note of the product ID.
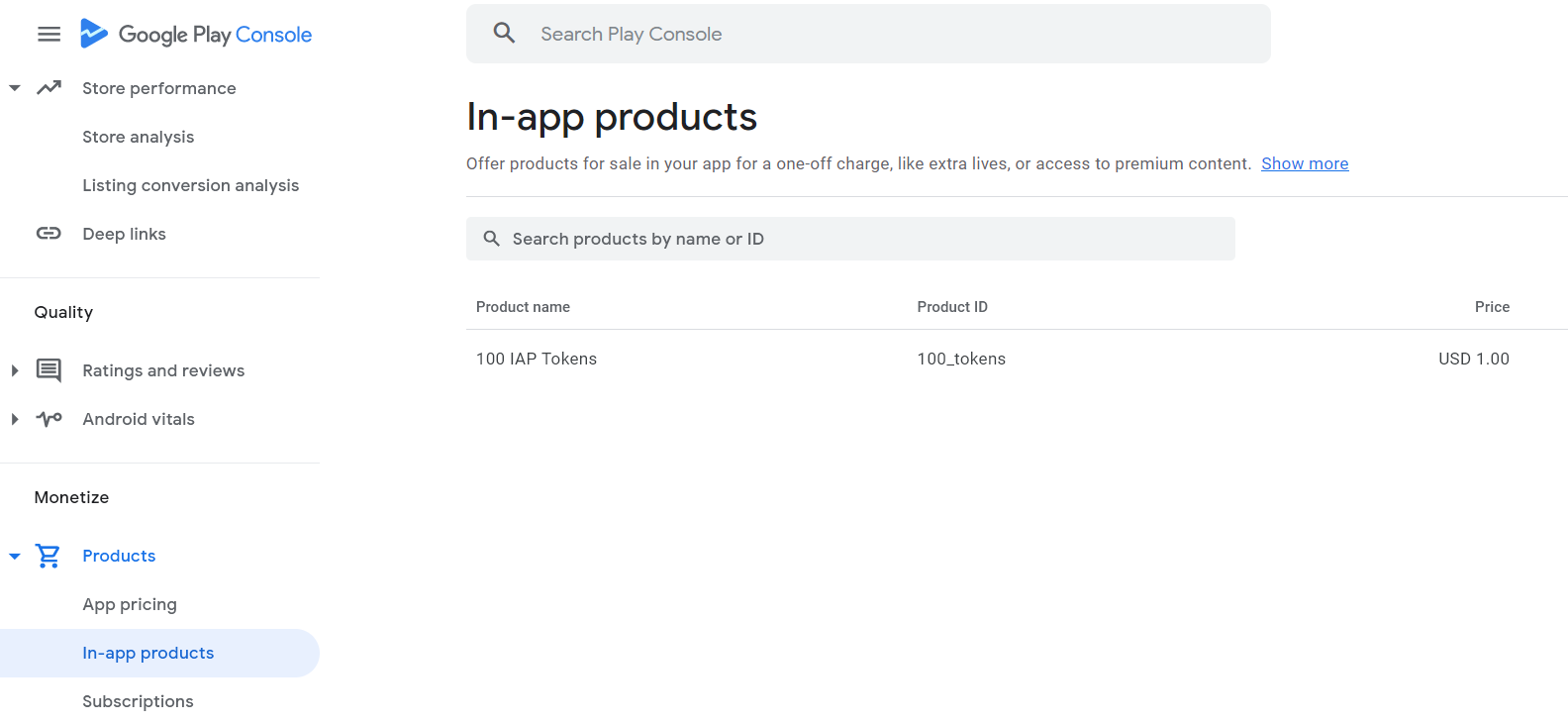
Recommendation: while you're here, you should set up internal testing tracks, create email groups to invite to these tracks, and in Google's case, set them as Licensed Testers so they can use test credit cards.
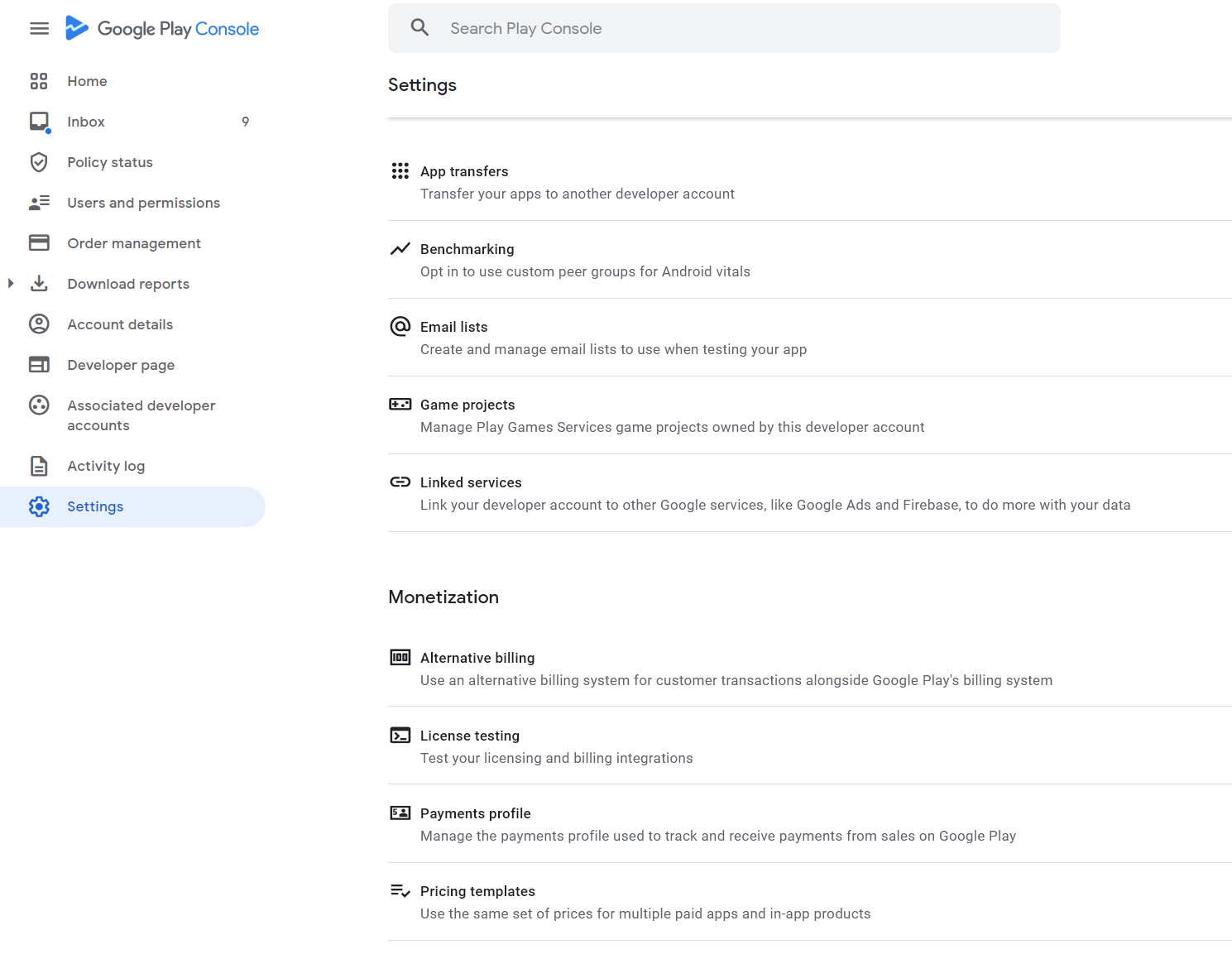
Integrating Unity IAP into Your Project
The fun part!
Clone our client template https://github.com/thirdweb-example/unity-iap-client
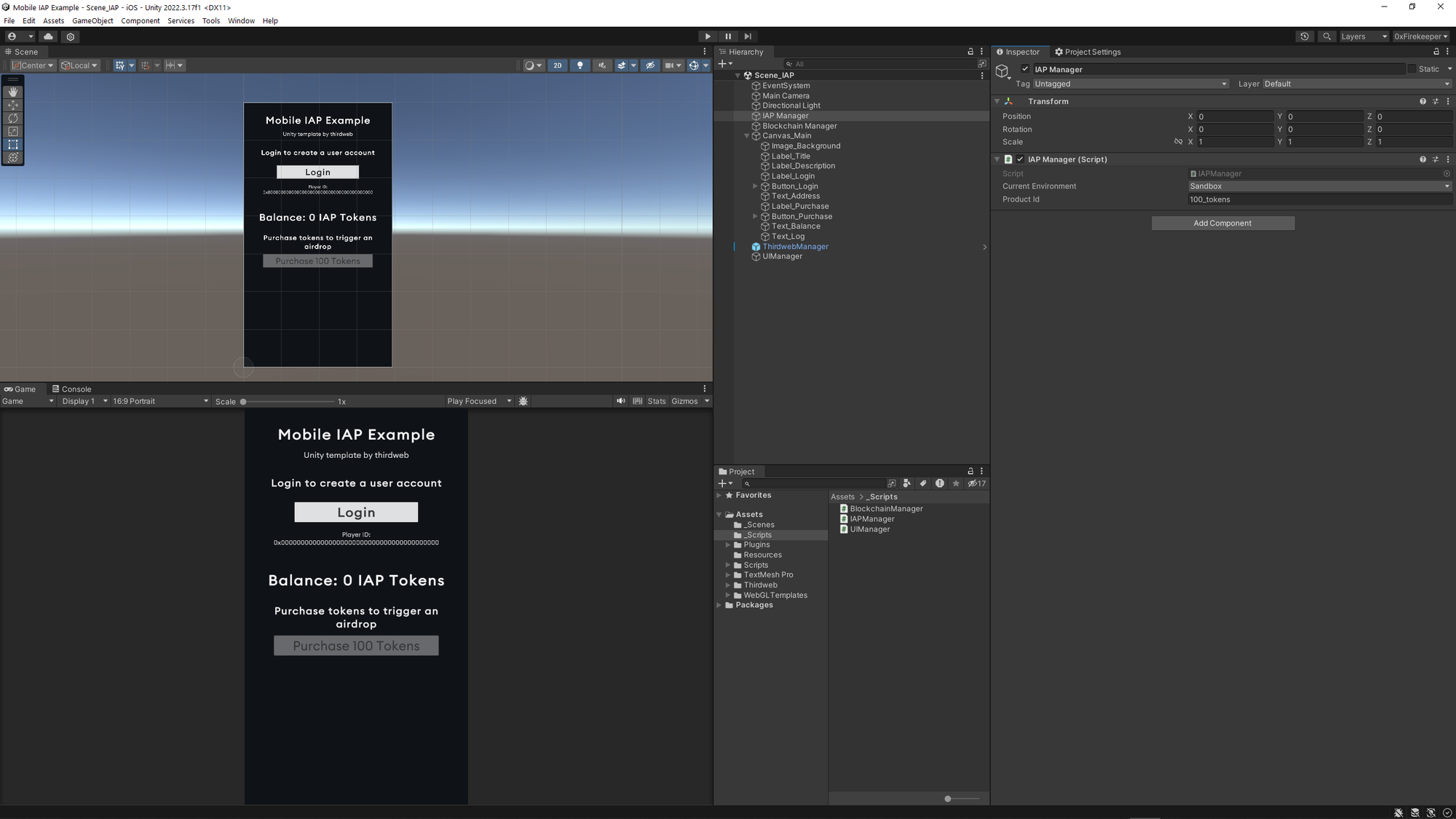
Import the Unity In App Purchasing package from the Package Manager
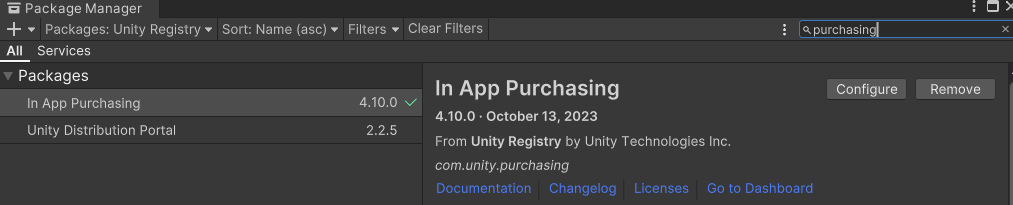
Head into your Project Settings to setup IAP, make sure you also generate the Tangle classes, we'll be using them in live builds for client side processing.
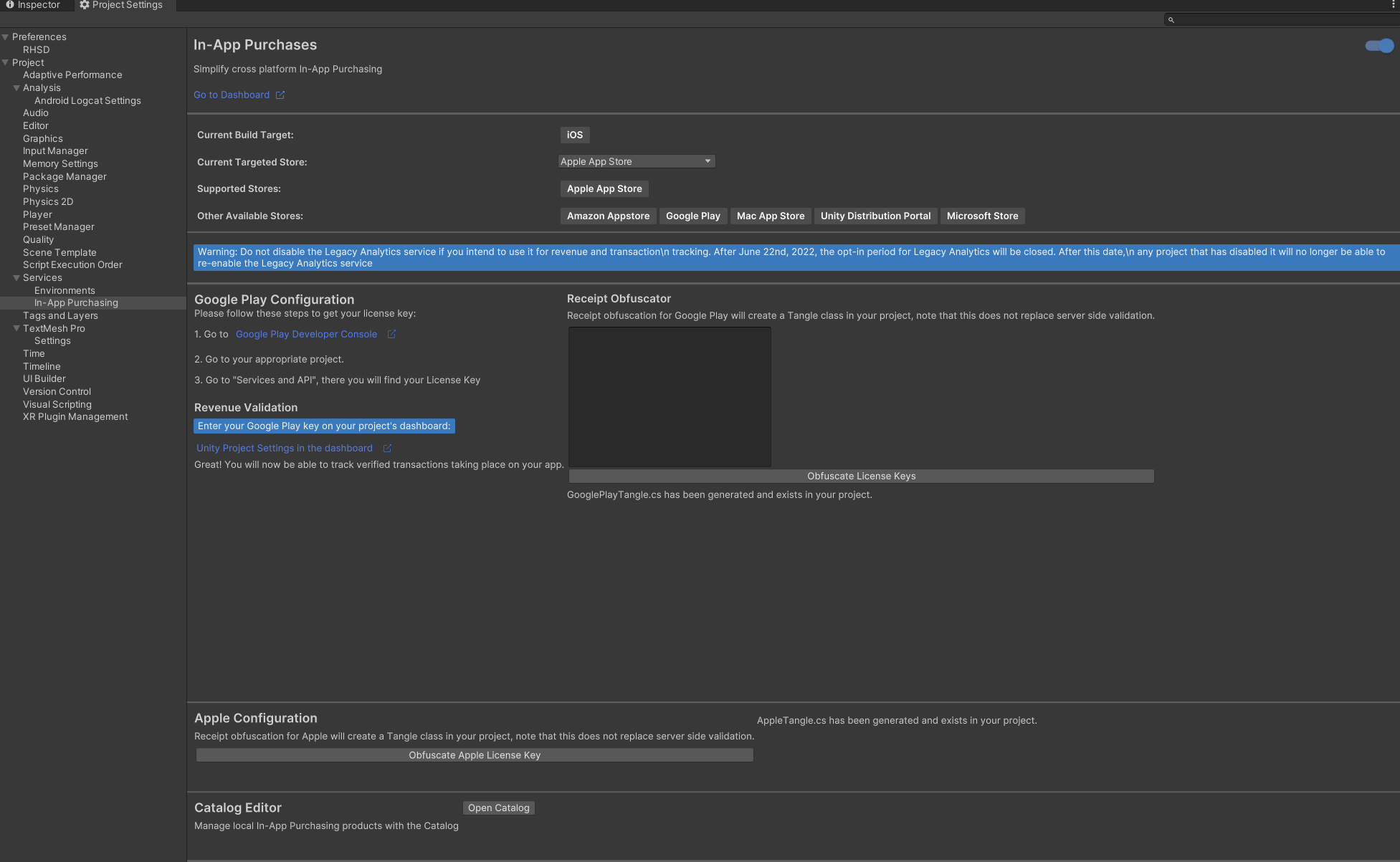
Part 2: Setting Up the Client for Blockchain Interactions
The client template project is set up with everything you need to interact with the blockchain, using the thirdweb Unity SDK.
Check out the ThirdwebManager if you want to customize the chain you're using, by default it is set to Arbitrum Sepolia, and uses Smart Wallets for user account creation and login. This allows for client side gasless testing convenience.
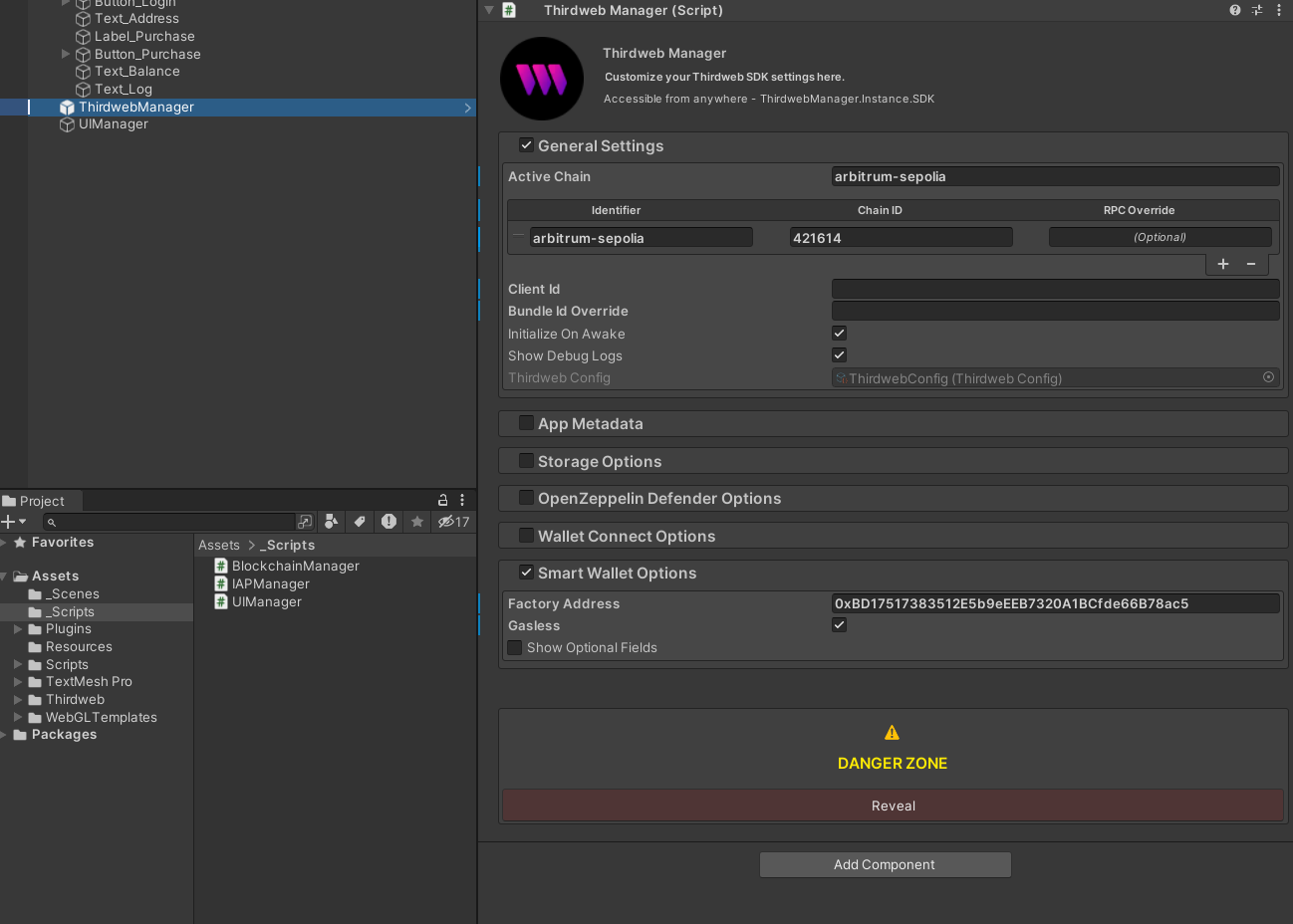
Let's explore the other scripts:
- IAP Manager - Initializes Unity IAP, adds your consumable product, and validates receipts based on your Environment. This template does not use the Codeless API or Catalog, all is done within this script.
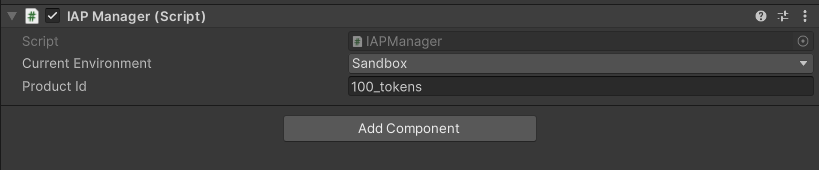
- Blockchain Manager - Leverages thirdweb's Unity SDK to quickly Connect to a Smart Wallet, poll for your balance every 5 seconds, and award tokens.
Awarding tokens in this scenario means either:- In Local Environment, generate an unsafe client side signed payload and mint, useful for quick testing in editor.
- In Production and Sandbox environments, in live builds, after receipts are process it will POST to the Server Path provided, which in our case interacts with our backend to validate IAP receipts and subsequently airdrop tokens to the user wallet using thirdweb Engine. It then waits until the balance is updated and voila!
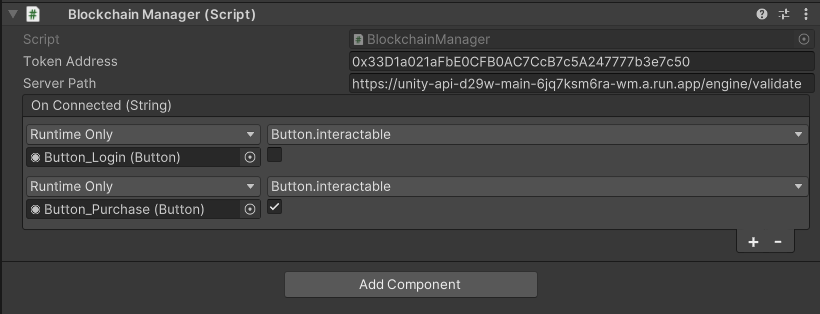
- UI Manager - Simple script to update UI.
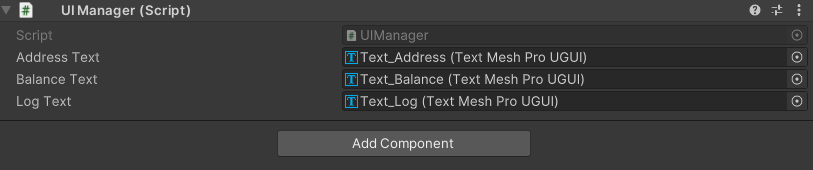
Part 3: Server-Side Setup and Configuration
An overview of the open-sourced server code and its role in verifying IAP receipts and airdropping tokens. This template is an opinionated example of implementing your own verification. It is not meant for production and is provided as-is for demonstration purposes. It will help you integrate Apple, Google and thirdweb APIs more easily and servers as a great starting point, compatible with Unity IAP.
Setting Up the Server Environment
Clone https://github.com/thirdweb-example/unity-iap-server to get started.
Make sure you set up IAP on the Apple and Google stores for this to work.
Follow their respective guides to setup sandbox mode as well, whereby testers can use test credit cards.
Thirdweb Engine
This guide does not cover setting up your thirdweb Engine, please visit our documentation instead here.
When you're done, set up these environment variables:
THIRDWEB_ENGINE_URL
- thirdweb Engine endpoint.THIRDWEB_ENGINE_BACKEND_WALLET
- thirdweb Engine backend wallet that will mint tokens to the user post receipt validation.THIRDWEB_CHAIN_ID
- chain id where your token reward resides.THIRDWEB_API_SECRET_KEY
- thirdweb API Key secret
Google Setup
In this example, we interact with the Google Play API using a service account stored at root service-account-file.json
.
Head to the google cloud console to create a service account with the right permissions, and make sure its email is added to your Google Play project User Permissions (important).
Download the service account file, it should look like service-account-file.json.example
.
Apple Setup
In this example, we interact with the Apple Connect Server API using an In-App Purchasing Key.
Head to the Apple dashboard and create a key, all the information you need will be on that page.
Download the .p8 file and set it at root in subscription-key.p8
, it should look like subscription-key.p8.example
.
Environment Variables:
APPLE_APP_STORE_ISSUER_ID
- Available on the Keys page where you created your In-App Purchasing Key.APPLE_APP_STORE_KEY_ID
- Available on the Keys page where you created your In-App Purchasing Key.APPLE_APP_STORE_BUNDLE_ID
- The bundle identifier of your Unity game, such as com.thirdweb.myepicgame
- found in Project Settings.
Further setup
The src/controllers/engineController.ts
script contains all the code used for receipt validation and interaction with thirdweb Engine. When you're done testing out the basics with our default configuration and checks, we highly recommend you extend this script to use a database, perhaps add retrying functionality and any additional behavior for other product types such as subscriptions. We've also hardcoded the environment to Sandbox for the apple example, feel free to turn that into a variable as well once you're comfortable.
Part 4: Testing and Deployment
Testing Your Integration
Run yarn dev
to run the server locally. In most cases, you'll need a live deployment to interact with it from a sandbox live mobile Unity build.
We recommend using Zeet or other services to quickly iterate and test your deployments. Make sure your service account for Google Play has valid permissions on your Google Play dashboard, and Google Console. Make sure your billing information is filled on the respective store dashboards. Make sure at least one internal testing build is uploaded to the stores for IAP to show up. Some of these changes require a few hours to start working if you've just updated them.
Conclusion
As we wrap up this comprehensive guide on integrating Unity's In-App Purchases (IAP) with blockchain functionalities using the thirdweb Unity SDK and thirdweb Engine, it's important to reflect on the transformative potential this integration offers. By marrying traditional gaming monetization strategies with the innovative and transparent nature of blockchain technology, game developers are empowered to create more engaging and rewarding experiences for their players. The seamless blend of Unity IAP with blockchain not only opens up new avenues for digital rewards but also fosters a more interconnected and dynamic gaming ecosystem.
This guide has taken you through the journey from setting up IAPs on digital stores, integrating blockchain functionalities on the client side, to configuring the server-side logic for secure and efficient blockchain interactions. Each step has been designed to equip you with the knowledge and tools necessary to enhance your gaming projects with blockchain rewards, making them stand out in a crowded marketplace.
The potential of blockchain in gaming is vast and largely untapped. As you embark on or continue your journey in integrating these technologies, remember that practice, experimentation, and iteration are key to unlocking innovative solutions and creating truly immersive gaming experiences. The templates and documentation provided are not just a starting point but a foundation upon which you can build, customize, and innovate.
Thank you for following this guide, and here's to the future of gaming—immersive, interactive, and interconnected. Happy onramping!