Create an ERC20 token with TypeScript
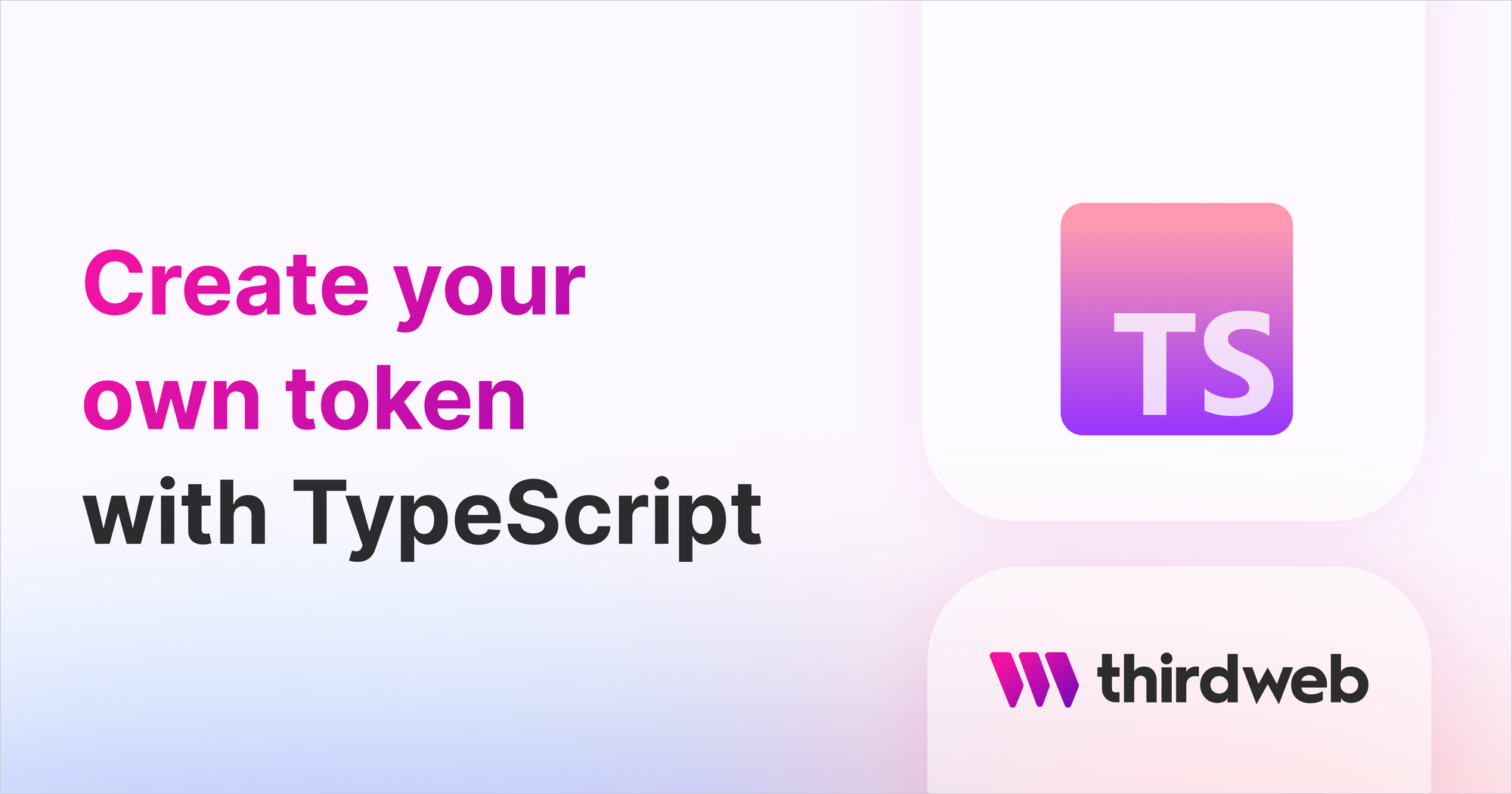
⚠️ Warning: This guide currently uses v4 of the Connect SDK. For v5 (latest) code snippets, please check out our documentation while this guide is being updated. ⚠️
You can deploy a Token via the dashboard, but in this guide, we'll demonstrate how to do it using TypeScript!
Create a new folder on your computer called my-token
, and within this folder, create a file called mint-token.ts
.
Installing TypeScript SDK
Install the required dependencies to get started with thirdweb, by running the following command inside your my-token
folder.:
npm install @thirdweb-dev/sdk
Deploying a Token Contract
To deploy a Token using the SDK, we can make use of the sdk.deployer,
which can create any of our pre-built contracts!
To deploy from your wallet, you'll first need to export your wallet's private key.
Learn how to export your private key from your wallet.
Ensure you store and access your private key securely.
- Never commit any file that may contain your private key to your source control.
Learn more about securely accessing your private key.
Now we're ready to deploy the Token!
import { ThirdwebSDK } from "@thirdweb-dev/sdk";
async function main() {
const NETWORK = "mumbai";
// Learn more about securely accessing your private key: https://portal.thirdweb.com/web3-sdk/set-up-the-sdk/securing-your-private-key
const sdk = ThirdwebSDK.fromPrivateKey(
"<your-private-key-here>"
NETWORK,
);
const deployedAddress = await sdk.deployer.deployToken({
name: "My Token",
primary_sale_recipient: "0x-your-public-wallet-address-here",
});
console.log(deployedAddress);
// Add the next code snippet here
}
main();
Before we run this script, let's add some code to some tokens immediately after we create the smart contract!
Minting Tokens
To mint tokens, we:
- Get the token contract using its contract address
- Call the
mintTo
function with our address and desired quantity
Be sure to replace the primary_sale_recipient
and toAddress
with a valid wallet address.
const tokenContract = sdk.getToken(deployedAddress);
const toAddress = "0x-your-public-wallet-address-here"; // Address of the wallet you want to mint the tokens to
const amount = "1.5"; // The amount of this token you want to mint
const transaction = await tokenContract.mintTo(toAddress, amount);
console.log(transaction);
To run the script, run:
npx ts-node mint-token.ts
That's it!
Congratulations! You have created your own ERC20 token!
Learn more about what you can do with this contract via the SDK from the pre-built contract page.