Deploy an NFT Drop with TypeScript
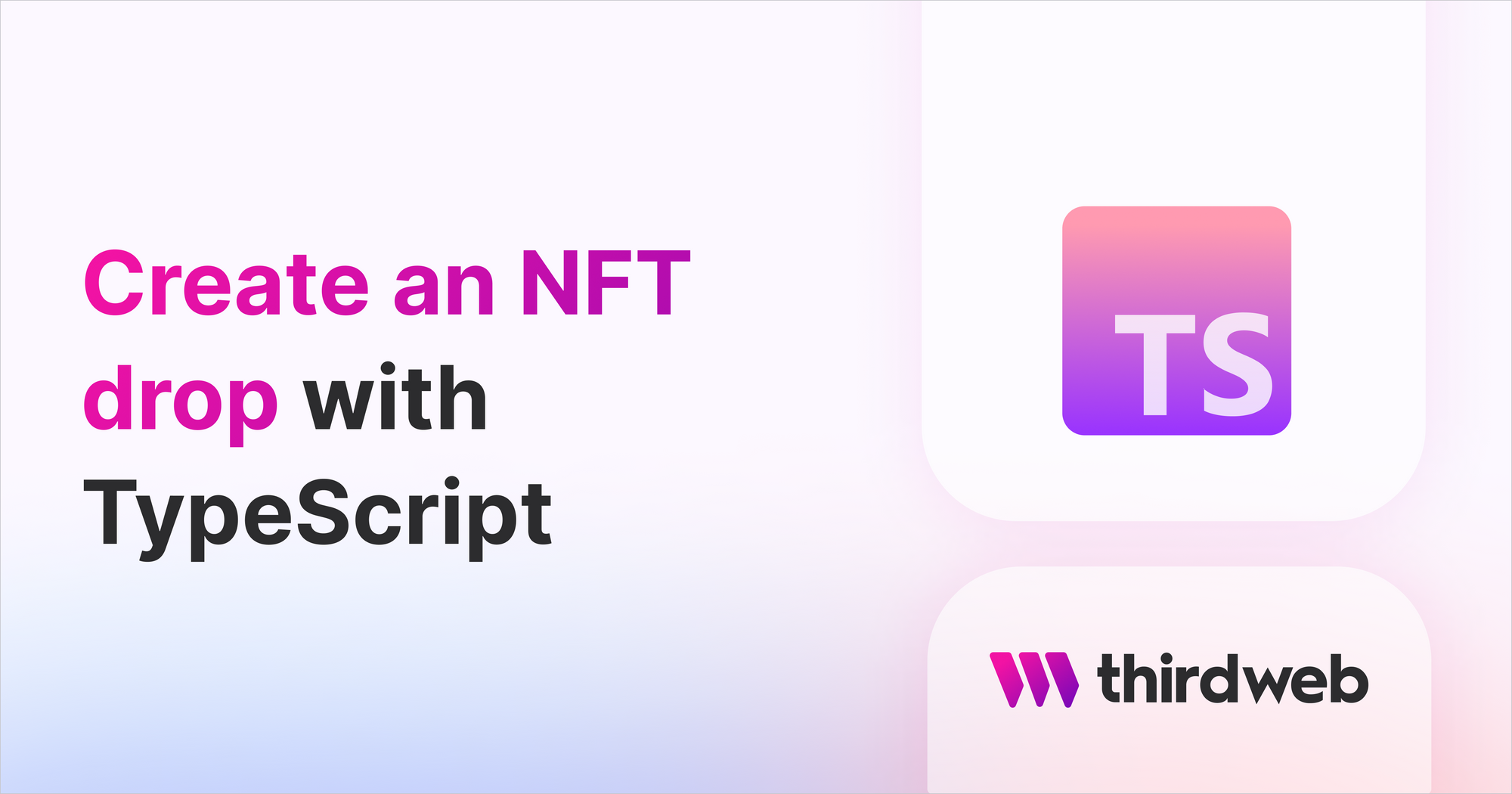
In this guide, we'll show you how to deploy and configure an NFT Drop with the TypeScript SDK!
You can deploy an NFT Drop via the dashboard, but in this guide, we'll demonstrate how to do it using TypeScript!
Create a new folder on your computer called my-nft-drop
, and within this folder, create a file called create-nft-drop.ts
.
Installing TypeScript SDK
Install the required dependencies to get started with thirdweb, by running the following command inside your my-nft-drop
folder.:
npm install @thirdweb-dev/sdk
Deploying an NFT Drop Contract
To deploy an NFT Drop using the SDK, we can make use of the sdk.deployer,
which can create any of our pre-built contracts!
To deploy from your wallet, you'll first need to export your wallet's private key.
Learn how to export your private key from your wallet.
Ensure you store and access your private key securely.
- Never commit any file that may contain your private key to your source control.
Learn more about securely accessing your private key.
Now we're ready to deploy the NFT Drop!
Be sure to replace the primary_sale_recipient
with your wallet's address.
import { ThirdwebSDK } from "@thirdweb-dev/sdk";
import "dotenv/config";
async function main() {
const NETWORK = "mumbai";
const sdk = ThirdwebSDK.fromPrivateKey(
// Learn more about securely accessing your private key: https://portal.thirdweb.com/web3-sdk/set-up-the-sdk/securing-your-private-key
"<your-private-key-here>",
NETWORK,
);
const deployedAddress = await sdk.deployer.deployNFTDrop({
name: "My Drop",
primary_sale_recipient: "0x-your-public-wallet-address-here",
});
console.log(deployedAddress);
// Put the next snippet here
}
main();
Lazy Minting NFTs
We can lazy-mint NFTs in batch using an array of metadata objects.
Lazy minting means we prepare the metadata for other people to mint the NFTs into their wallets.
Be sure to replace the image
fields with file paths or URLs to images for your NFTs!
const contract = sdk.getNFTDrop(deployedAddress);
// Custom metadata of the NFTs to create
const metadatas = [
{
name: "Cool NFT",
description: "This is a cool NFT",
image: fs.readFileSync("path/to/image.png"), // This can be an image url or file
},
{
name: "Cool NFT",
description: "This is a cool NFT",
image: fs.readFileSync("path/to/image.png"),
},
];
const results = await contract.createBatch(metadatas); // uploads and creates the NFTs on chain
// Put the next snippet here
Set up claim phases
Once your NFT Drop contract is created and has some NFTs, it's time to set the Claim Phases.
These phases are rules we'll apply when someone wants to claim an NFT, such as price, max amount of tokens claimed, wallets allowed to claim, etc.
In this guide, we will set two claim phases - you can set more or less depending on your needs.
The first claim phase will be a presale with an added snapshot. Only the addresses listed will be able to claim NFTs from the drop.
We are also adding maxQuantity
so only 20 NFTs can be minted in this phase.
The second phase does not add a snapshot so everyone can claim.
Be sure to replace the addresses in the allowList
with valid wallet addresses!
const presaleStartTime = new Date();
const publicSaleStartTime = new Date(Date.now() + 60 * 60 * 24 * 1000); // today's date + 24 hours
const allowList = ["0x...", "0x..."]; // modify this with your allow list
const claimConditions = [
{
startTime: presaleStartTime, // start the presale now
maxQuantity: 20, // limit how many mints for this presale
price: 0.01, // presale price
snapshot: allowList, // limit minting to only certain wallet addresses
},
{
startTime: publicSaleStartTime, // 24h after presale, start public sale
price: 0.08, // public sale price
},
];
await contract.claimConditions.set(claimConditions);
console.log("Done!");
So the only thing left is to run our code! Open a terminal and paste the following:
npx ts-node create-nft-drop.ts
That's it!
Congratulations! You have created your own NFT Drop!
Learn more about what you can do with this contract via the SDK from the pre-built contract page.