Create A Mint Button to Claim NFTs from your NFT Drop
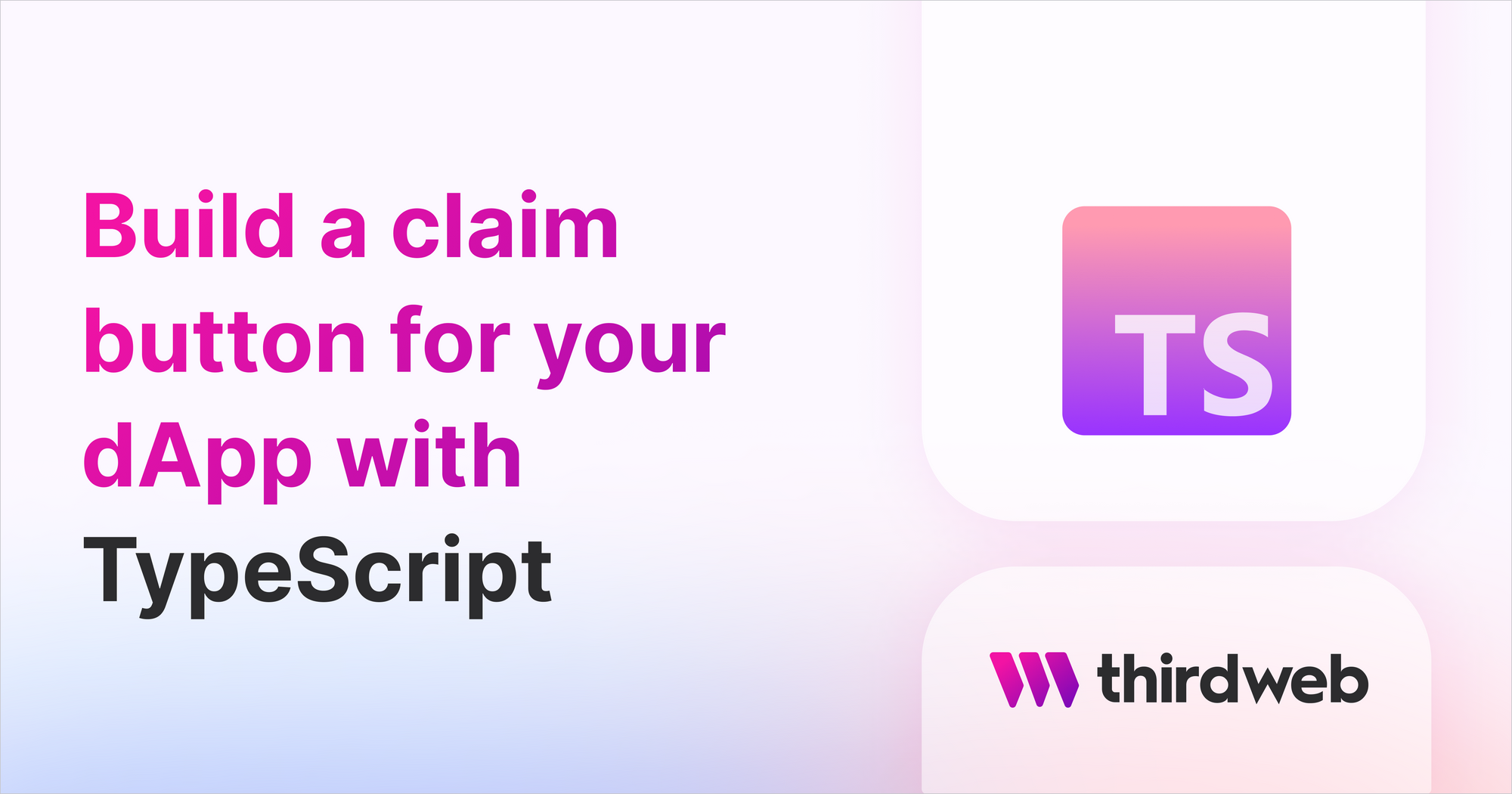
⚠️ Warning: This guide currently uses v4 of the Connect SDK. For v5 (latest) code snippets, please check out our documentation while this guide is being updated. ⚠️
In this guide, we'll show you how to create a claim button on your website that allows users to claim an NFT from your drop.
Full code and demo: https://contract-hub.thirdweb-example.com/nft-drop
If you're starting from scratch, you can create a new thirdweb project by running:
npx thirdweb create
Or integrate into an existing project by installing the required dependencies:
npm install @thirdweb-dev/react @thirdweb-dev/sdk ethers@5
Setting Up The ThirdwebProvider
In your index.js
(or _app.js
if you're using Next.js) file, we wrap our application in the ThirdwebProvider
component.
import { ThirdwebProvider, ChainId } from "@thirdweb-dev/react";
function MyApp({ Component, pageProps }) {
const desiredChainId = ChainId.Goerli;
/**
* Make sure that your app is wrapped with these contexts.
* If you're using React, you'll have to replace the Component setup with {children}
*/
return (
<ThirdwebProvider desiredChainId={desiredChainId}>
<Component {...pageProps} />
</ThirdwebProvider>
);
}
export default MyApp;
The Claim button
Now let's create a components under components/ClaimButton.jsx
.
export default function ClaimButton() {
const nftDrop = useNFTDrop("<your-contract-address-here>");
const { mutate: claimNft } = useClaimNFT(nftDrop);
return (
<button
onClick={() =>
claimNft({
quantity: 1,
to: address,
})
}
>
Claim
</button>
);
}
Then, we need to import this component on our homepage App.js
(or index.js
for Next.js) file:
import { ClaimButton } from "../components/ClaimButton";
const Home = () => {
return (
<div>
<ClaimButton />
</div>
);
};
export default Home;
That's it!
If you have any questions, drop by our Discord!