Build Your First Web3 App: A Step-by-Step Guide
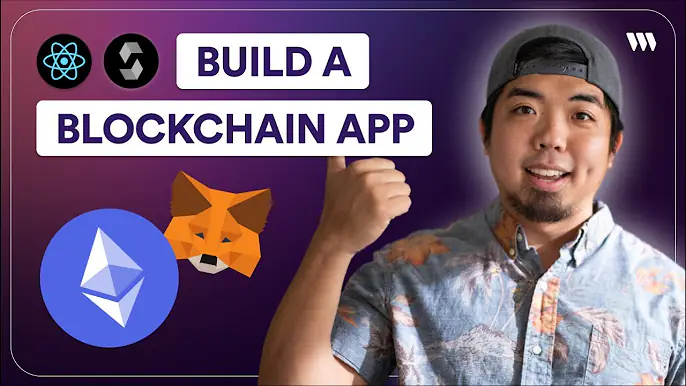
Building your first web3 application can seem daunting, but with the right tools and guidance, it's easier than you might think. In this tutorial, we'll walk you through creating a simple counter app using thirdweb's latest Connect SDK and deploying your own smart contract.
By the end, you'll have a fully functional web3 app that allows users to connect their wallet, view the current count stored on the blockchain, and increment or decrement the value.
Ready to dive in? Let's get started!
Want to see the full tutorial? Check out the video below:
Prerequisites
Before we begin, make sure you have the following:
- A basic understanding of React and TypeScript
- Node.js installed on your machine
- A code editor like VS Code
- A web3 wallet like MetaMask
- Some test funds on a testnet
Creating the Smart Contract
The first step is to create a simple counter smart contract that will store the count value and provide functions to increment and decrement it.
- Create a new smart contract
npx thirdweb create contract
- Name your project and select the framework you want to use.
- Name the contract
Counter
and chooseEmpty Contract
. - Open the
Counter.sol
file in your code editor.
contract
folder. If using Forge file is located in src
folder.Replace the contents with the following code:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract Counter {
uint256 public count;
constructor() {
count = 0;
}
function increment() public {
count++;
}
function decrement() public {
require(count > 0, "Count cannot go below 0");
count--;
}
function getCount() public view returns (uint256) {
return count;
}
}
Deploying the Contract
Now that we've written our smart contract, it's time to deploy it to a testnet.
- Open your terminal and navigate to contract directory.
- Run
npx thirdweb deploy
- A tab in your browser should open. Click "Deploy Now" to deploy you contract to any EVM compatible blockchain
Setting Up the Project
Let's start by creating a new Next.js project or clone our Next starter template
Install the thirdweb SDK:
npm install thirdweb
or
yarn add thirdweb
Next, we need to create our client
and chain
:
const CLIENT_ID = process.env.NEXT_PUBLIC_CLIENT_ID;
export const client = createThirdwebClient({
clientId: CLIENT_ID as string;
});
export const chain = defineChain("Your chain ID");
Last we need to wrap our app with ThirdwebProvider
.
export default function RootLayout({
children,
}: ReadOnly<{
children: React.ReactNode;
}>) {
return (
<html lang="en">
<body classsname={inter.classname}>
<ThirdwebProvider>
{children}
</ThirdwebProvider>
</html>
);
}
Great! We now have our project set up with Next.js, TypeScript, and the thirdweb SDK. Let's start building out our app.
Connecting a Wallet
To be able to interact with the blockchain we will need to allow a user to connect a wallet to our app.
Lets create a new component and add the ConnectButton
React component from thirdweb. Providing it with the client
and chain
we created earlier.
const Login: React.FC = () = => {
return (
<ConnectButton
client={ client }
chain={ chain }
/>
)
};
Now we have a way for a user to connect any wallet to our app!
Connecting to the Contract
To interact with our deployed contract, we need to connect to it using the thirdweb SDK.
export const CONTRACT = getContract({
client: client,
chain: chain,
address: "0x1234..."
abi: [{
"Your contract abi..."
}]
});
address
will be your smart contract address and abi
will be the ABI of your contract, which you can get from the contract dashboard.Building the Counter
Let's build out the UI for our counter app.
1. Get the count for the counter from our smart contract
const { data: count, isLoading: loadingCount } = useReadContract({
contract: CONTRACT,
method: "getCount"
});
{loadingCount ? (
<p>...</p>
) : (
<h2>{count?.toString()}</h2>
)}
2. Now lets build the increment and decrement buttons for our counter app
<TransactionButton
transaction={() => prepareContractCall({
contract: CONTRACT,
method: "decrement"
})}
onTransactionSent={() => console.log("decrementing...")}
onTransactionConfirmed={() => alert("Decremented")}
>-</TransactionButton>
<TransactionButton
transaction={() => prepareContractCall({
contract: CONTRACT,
method: "increment"
})}
onTransactionSent={() => console.log("incrementing...")}
onTransactionConfirmed={() => alert("Incremented")}
>+</TransactionButton>
Conclusion
In this tutorial, we walked through the process of building a simple web3 counter application from start to finish using thirdweb.
The key steps we covered:
- Creating and deploying a Counter smart contract with thirdweb
- Setting up a new Next.js project with the thirdweb Connect SDK
- Connecting to our deployed smart contract
- Building UI components to read and write to the contract
By leveraging thirdweb's powerful tools and SDKs, we were able to accomplish this fairly quickly and easily. The thirdweb Connect SDK provides convenient hooks and components that make interacting with smart contracts a breeze.
I hope this tutorial gave you a solid foundation for building your own web3 apps with thirdweb. The possibilities are endless - you can extend the contract, add more features to the UI, integrate with other web3 protocols, and much more.
If you have any questions or need further assistance, feel free to reach out on the thirdweb support channels. Happy building!