How to Build a Web3 Telegram Clicker Game
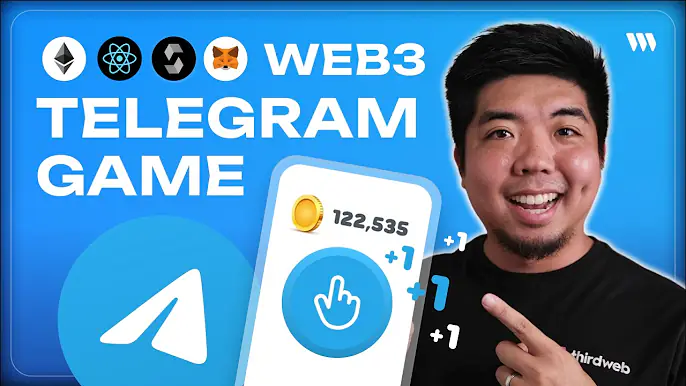
In this tutorial, we'll walk through the process of building a web3 clicker game mini app for Telegram using thirdweb's tools. Users will be generated a wallet from their Telegram account, play the game, and earn ERC20 tokens as rewards, all within the Telegram app.
Check out the full video tutorial here:
By the end of this guide, you will have a fully functioning web3 Telegram mini game with the following features:
- Automatically authenticate users and generate a wallet based on their Telegram account
- Allow users to play a clicker game and defeat monsters to earn token rewards
- Mint reward tokens directly to the user's wallet using thirdweb's Engine
Prerequisites
Before we begin, make sure you have the following:
- A thirdweb account
- Subscribed to thirdweb's Growth Plan or higher (Certain features of this build require that you are on Growth)
- Telegram account to create a bot and mini app
Step 1: Clone the Starter Template
To get started, we'll clone the telegram-miniapp repo which provides a base template for creating Telegram mini apps with thirdweb.
Open a terminal and run:
git clone https://github.com/thirdweb-example/telegram-miniapp.git clicker-mini-game <YOUR_APP_NAME>
Open the project in your code editor. You'll see two main folders:
next-app
: Where we'll build our mini app frontendtelegram-bot
: Code for our Telegram bot
Step 2: Install Dependencies
In the terminal, navigate to the telegram-bot
folder and install the dependencies:
cd telegram-bot
yarn install
Next, navigate to the next-app
folder and install the dependencies there as well:
cd ../next-app
yarn install
Step 3: Set Up Environment Variables
next-app
In the next-app
folder, rename .env.example
to .env.local
and add your private key:
NEXT_PUBLIC_CLIENT_ID=
ADMIN_SECRET_KEY=
BACKEND_WALLET_ADDRESS=
NFT_CONTRACT_ADDRESS=
ENGINE_URL=
THIRDWEB_SECRET_KEY=
Here's what each variable means:
THIRDWEB_CLIENT_ID
: Your thirdweb client ID which can be found in the thirdweb dashboard settings (Guide on how to create an API key)ADMIN_PRIVATE_KEY
: The same private key you added in thenext-app
env fileBACKEND_WALLET_ADDRESS
,NFT_CONTRACT_ADDRESS
,ENGINE_URL
, andTHIRDWEB_SECRET_KEY
: thirdweb Engine variables that we will set up later
telegram-bot
In the telegram-bot
folder, rename .env.example
to .env.local
and add the following variables:
THIRDWEB_CLIENT_ID=
BOT_TOKEN=
ADMIN_SECRET_KEY=
FRONTEND_APP_ORIGIN=
Here's what each variable means:
THIRDWEB_CLIENT_ID
: Your thirdweb client ID which can be found in the thirdweb dashboard settings (Guide on how to create an API key)BOT_TOKEN
: The token for your Telegram bot which we'll create in the next step with BotFatherADMIN_PRIVATE_KEY
: The same private key you added in thenext-app
env fileFRONTEND_APP_ORIGIN
: The URL where your mini app will be hosted. For local development you can use a tool like ngrok to create a public URL that forwards to your localhost
Step 4: Create a Telegram Bot
Open the Telegram app and search for the @BotFather
bot or use the link below. This is a tool will be used to create our Telegram bot and app.

- Type
/newbot
and follow the prompts to set a name and username for your bot. The username must end inbot
. - Once created, you'll be given an API token. Copy this token and paste it as the
BOT_TOKEN
value in yourtelegram-bot/.env.local
file.
Step 5: Create a Telegram Mini App
Now that we have out bot we can create an app for that bot we just created. Still using @BotFather
follow these steps:
- Type
/newapp
to create a new mini app. Select the bot you just created in the previous step. - Enter the title for the app and a short description for it.
- Upload an image to use for the app and a GIF (optional)
- Next add the URL your game will be hosted on. If you are going to be just testing this locally, pause here and we will come back to this in a later section.
Our Telegram mini app is now ready! Next, we need to setup Authentication Endpoints for our app with thirdweb to use our own endpoint to verify and generate wallets for our users.
Step 7: Setting up Authentication Endpoint
Custom Authentication Endpoints is a feature your get on thirdweb's Growth plan that allows you to set up your own endpoint for authenticating users to generate them an In-App wallet.
- Head over to your thirdweb dashboard and go to the "Connect" tab
- In the left navigation go to your "In-App Wallets" and toggle it to the "Configuration" tab
- In the "Authentication" section turn on "Custom Authentication Endpoint"
- Add your
<YOUR_URL>/api/auth/telegram
this will point to the authentication endpoint in the template that will verify the user's Telegram account
Now your Telegram mini app is ready and should generate a unique link for your users that will authenticate and generate them an In-App wallet when the link is opened. Next, we need to build our mini game.
Step 6: Deploy an ERC20 Token Contract
For our clicker game, we'll be rewarding users with an ERC20 token. Let's deploy a token contract to use.
- Head to the thirdweb dashboard and go to the Deploy tab. Select the ERC20 contract and configure the name, symbol, etc.
- Choose which network to deploy to (I'm using Sepolia for this tutorial) and hit "Deploy Now".
Once deployed, copy the contract address to the NFT_CONTRACT_ADDRESS
variable in the next-app
environment variables.
Step 7: Run the Telegram Bot
Now that we have our environment variables set up and token contract deployed, let's run our Telegram bot.
- In the
telegram-bot
folder, opensrc/bot/start.ts
. This file contains the code that will run when a user types the/start
command to our bot.
.env.local
file you can add the following code:const config = require("dotenv").config({ path: ".env.local" });
- Now run the bot with:
npm run dev
You should see a message that the bot is running.
Step 8: Build the Mini App Frontend
Open the next-app
folder in your code editor.
The mini app URL should match the FRONTEND_APP_ORIGIN
you set in your telegram-bot/.env.local
file.
Next, open pages/index.tsx
. This is the main page of our mini app.
For our mini game example we built a simple clicker game. A random health is generated from 30-100 and based on the generated health a matching monster is generated as well. User can then click on the monster the reduce their health. As they clicker they have a buffer and have to wait for that to charge before they can attack again. Once the monster is defeated you can claim some tokens depending on the monster.
Here is the sample code:
Step 9: Setup Engine to Claim Tokens
Now that we have our game created lets create a way to interact with the blockchain and collect the ERC-20 tokens we deployed earlier. We'll be using thirdweb Engine to do this.
Getting Started with Engine
Understanding Backend Wallets with Engine
- Create an Engine instance through thirdweb's dashboard or you can run you own local instance for testing.
- Create an file in your app
src/app/api/mintToken/route.ts
- Create our API call to Engine to mint tokens to the user when a monster is defeated
- In our game make sure to add to call our
mintToken
Engine API:
const handleRewardClaim = async () => {
if (!address || isClaiming) return;
setIsClaiming(true);
try {
const response = await fetch('/api/mintToken', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({
userWalletAddress: address,
amount: currentReward,
}),
});
const result = await response.json();
if (response.ok) {
console.log('Claim successful:', result);
setShowReward(false);
generateMonster();
} else if (response.status === 408) {
console.log('Transaction not mined within timeout period:', result);
} else {
console.error('Claim failed:', result);
}
} catch (error) {
console.error('Error claiming reward:', error);
} finally {
setIsClaiming(false);
refetchTokenBalance();
}
};
Step 10: Run the Mini App Frontend
- In the
next-app
folder, run the following command to start the frontend:
npm run dev
This will start the frontend locally.
However, Telegram will not be able to access your local server. To get a public URL, we can use a tool like ngrok.
- Install
ngrok
and run:
ngrok http <YOUR_LOCAL_HOST>
This will create a public URL that forwards to your local server. Copy this URL and paste it into your telegram-bot/.env.local
file for the FRONTEND_APP_ORIGIN
Step 11: Update Mini App URL
Now that we have a public URL for our mini app, let's update it with BotFather.
- Open the chat with
@BotFather
and type/myapps
. - Select your bot and select
Edit Web App URL
- Enter the forwarding URL you got from ngrok
Our mini app is ready and should now open our next-app
from our project.
Step 12: Test the Mini App
Go to your bot chat and type /start
again. This time, when you click the link, it should open your mini app!
You'll be generated a wallet from your Telegram account and have a monster generated to play the game and collect tokens.
And that's it! We've now built a basic web3 Telegram mini app that generates a wallet for our users, lets users play a mini game, and then allows users to claim ERC-20 tokens to their generated wallet. All this while abstracting away the web3 complexities from the game.
Of course, there are many ways you can expand on this and build more complex mini apps. Feel free to continue experimenting and building!
Conclusion
In this tutorial, we learned how to:
- Set up a Telegram bot and mini app using BotFather
- Deploy an ERC20 token contract using thirdweb
- Build a mini app frontend with Next.js and thirdweb's React SDK
- Authenticate users and generate wallets using thirdweb's authentication endpoints
- Mint tokens to users using thirdweb's engine
thirdweb provides a powerful suite of tools for building web3 apps, and the Telegram mini app platform opens up a lot of possibilities for bringing web3 functionality to Telegram's massive user base.
I hope this tutorial was helpful and gave you a starting point for building your own web3 Telegram mini apps. Happy building!