How to Build a Web3 Telegram Mini App
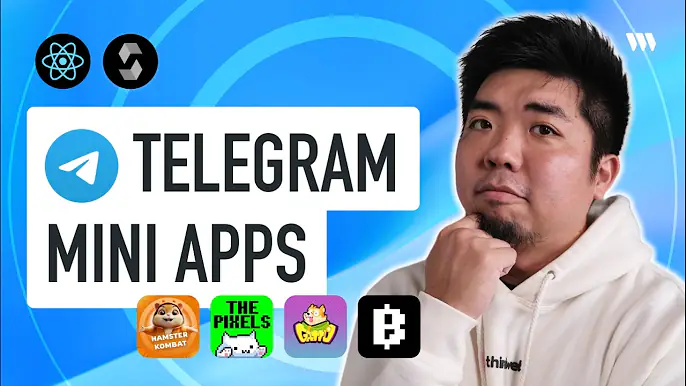
In this tutorial, we'll walk through the process of building a web3 Telegram mini game using thirdweb's SDK. The game will be a simple rock paper scissors game where users can connect their wallet, play the game, and earn tokens for winning.
By the end of this guide, you will have a fully functioning web3 Telegram mini game that allows users to:
- Connect their wallet by signing in with an email
- Play rock paper scissors against the computer
- Claim token prizes for winning games
- View their token balance and wallet address
Check out the full video tutorial here: Build a Web3 Telegram Mini Game with thirdweb
Prerequisites
- thirdweb account
- Wallet with testnet funds on the chain of your choice
- Telegram account
Step 1: Create a Telegram Mini App with BotFather
The first step is to create a new Telegram bot and mini app using the BotFather bot.
- Add BotFather to your Telegram
- Enter the command
/newbot
(new bot must be created first before creating an app) - Choose and enter a name and username for your bot
- You will be given an access token. Securely store that on side as you will need it later on.
- Next, create a new mini app with BotFather. Enter command
/nexapp
- Select the bot you created to create you app in
- Enter the prompts given to you for the apps name, description, and photo
- When asked for the URL of your app, enter the domain you will host your app on. If you are running this in a dev environment then pause here and we'll come back
Step 2: Set Up the Vite React Project
Now, let's set up a new Vite React project for our mini game and web app.
- Open your terminal and run:
bun create vite
You can usenpm
,yarn
, orpnpm
as well. - Choose a project name and select
React
as the framework andTypeScript + SWC
as the variant - Change into the project directory:
cd project-name
- Open your project in your code editor
Step 3: Configure the Web App as a Telegram Mini App
Now, we will configure our web app to be opened as a Telegram mini app when opened through the Telegram app.
- Open the
index.html
file in your project - Add this script in the
<head>
tags of the file:<script src="https://telegram.org/js/telegram-web-app.js"></script>
- Run your app locally:
npm run dev
- Open your browser to where your app is being hosted
At this point we have our web app running, but we need to provide the URL to Telegram so it knows where to open our mini app.
Since we're just running the web app locally, we need a way to create a public URL that tunnels to our localhost URL. We can use a tool like ngrok for this.
- Install ngrok (If you don't have it installed already)
- In a new terminal window, run:
ngrok http http://localhost:<port number>
- Copy the forwarding address provided (e.g.
https://1a2b-3c4d-5e6f-7g8h-9i0j.ngrok.io
) - Head back to the Telegram chat with BotFather and provide this address as the URL for the mini app
- Finish providing the rest of the information for your app. You'll be provided a URL which you can open to see your web app loaded in Telegram
Step 4: Install and setup thirdweb
Now that we have our mini app setup, let's start building our app with thirdweb so we can connect a wallet and interact with contracts onchain.
- In your project install thirdweb:
npm i thirdweb
- Create a new file called
client.ts
in thesrc
directory with the following:
import { createThirdwebClient } from "thirdweb";
const clientId = import.meta.env.VITE_TEMPLATE_CLIENT_ID;
export const client = createThirdwebClient({
clientId: clientId,
});
- Create a
.env
file in the root directory and add yourVITE_TEMPLATE_CLIENT_ID
(Provide a thirdweb client ID. Don't have one, learn how to create one here.) - Head to your
main.tsx
file and wrap your<App />
with<ThirdwebProvider>
Step 5: Add a Connect Wallet Button
Let's add a connect wallet button using the ConnectButton
component from thirdweb. We'll configure it to only allow users to connect with email and connect a Smart Wallet (Account Abstraction) so user doesn't have to pay for gas and we can sponsor it with a paymaster.
- Create a components folder and file for your game. Example in repo in a RockPaperScissors game.
- In the game file add the ConnectButton component. Provide it your client, configure the wallet to only email with In-App wallets, and configure accountAbstraction to allow sponsorGas:
<ConnectButton
client={client}
accountAbstraction={{
chain: <YOUR SELECTED CHAIN>,
sponsorGas: true
}}
wallets={[
inAppWallet({
auth: {
options:[ "email" ]
}
})
]}
/>
Now when a user clicks the connect button in our mini app, they'll be prompted to enter their email to sign in. thirdweb will generate a wallet for them and use that as an EOA wallet to a Smart Wallet.
Step 6: Create the Game Component
Next, let's build out our game that we want to show our user once they have signed in and connected a wallet.
- Build your own game in the game component file we created above or you can copy the Rock, Paper, Scissors game we created here:
The next step is to create the token contract that will allow us to mint tokens to the user's wallet when they win.
Step 7: Create the Token Contract
Now that we have our game set up to claim tokens, we need to create the token contract that will mint the tokens to the user's wallet.
- Go to the thirdweb dashboard , then to the "Contracts" tab, and click "Deploy Contract" in the top right corner.
- Choose the "Token Drop" contract, which is a claimable ERC20 token contract.
- Give your token a name (e.g. "Rock Paper Scissors Token"), symbol (e.g. "RPS"), and optional description and image.
- Scroll down and click "Deploy Now", confirm the gas, and sign the request to add the contract to your dashboard.
- Once deployed, click "View Contract" to go to the contract dashboard.
- Go to the "Claim Conditions" tab and set up a public claim where anyone can claim any amount of tokens.
Step 8: Interact with the Token Contract in the App
Now that our token contract is deployed, let's interact with it in our app to allow users to claim tokens when they win.
- In your game component let's first get our token contract we just deployed:
const contract = getContract({
client: client,
chain: <YOUR_CHAIN>,
address: "<YOUR_TOKEN_CONTRACT_ADDRESS>"
});
- Next we can create a
TransactionButton
to show when a user is allowed to claim a prize. This will execute theclaimTo
function from the contract and claim tokens to the user's wallet
<TransactionButton
transaction={() => claimTo({
contract: contract,
to: account.address,
quantity: "10"
})}
onTransactionConfirmed={() => {
alert('Prize claimed!')
}}
>Claim Prize</TransactionButton>
Step 10: Display Token Balance
The final step is to display the user's token balance next to their wallet address.
- In your game file, let's first get the balance of the token from the user's wallet:
const { data: tokenbalance } = useReadContract(
getBalance,
{
contract: contract,
address: account?.address!
}
)
- Now we can display that balance in our game for the user to see
<p>Balance: {tokenBalance?.displayValue}</p>
Conclusion
And there you have it! We've built a fully functional web3 mini game as a Telegram mini app using thirdweb. Users can:
- Connect their wallet by signing in with email (no gas required with Account Abstraction)
- Play rock paper scissors against the computer
- Claim token prizes when they win (no gas required)
- View their token balance and wallet address
By leveraging thirdweb's powerful SDK, we were able to easily implement wallet authentication, account abstraction for gasless transactions, and seamless interaction with our token contract.
The mini app works smoothly on both desktop and mobile versions of Telegram, providing an engaging and user-friendly experience.
Feel free to customize and expand upon this game to make it your own! You can change the game mechanics, add new features, or even create additional mini games.
I hope you found this tutorial helpful and informative. If you have any questions or need further assistance, please refer to the thirdweb documentation or reach out to their support team.
Happy building with thirdweb!