Build An Auction Button For Your NFT Marketplace
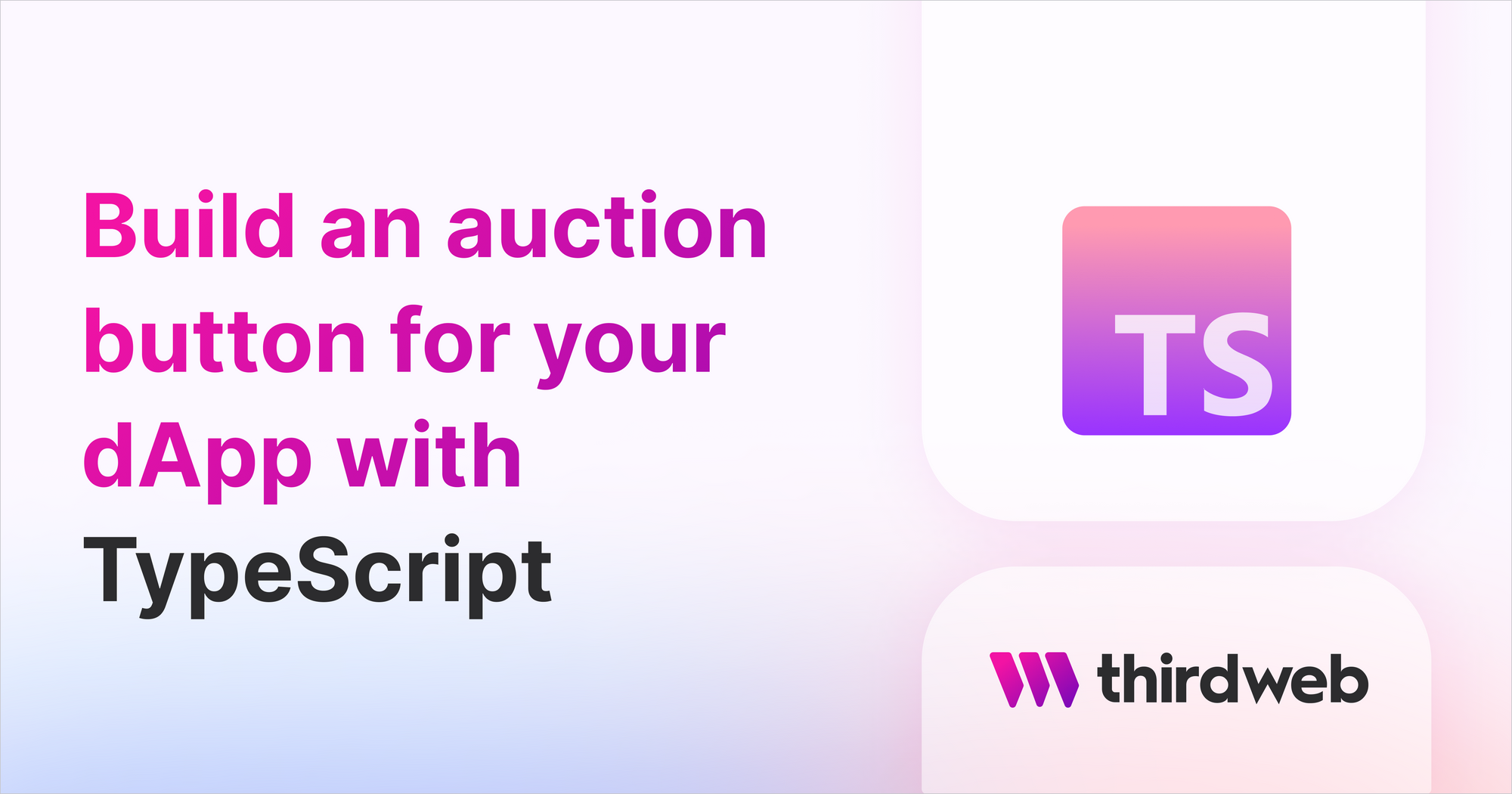
⚠️ Warning: This guide currently uses v4 of the Connect SDK. For v5 (latest) code snippets, please check out our documentation while this guide is being updated. ⚠️
In this guide, we'll show you how to build a simple React component that can list an NFTs for auction on a marketplace smart contract when clicked!
If you're starting from scratch, use the thirdweb CLI to create a new project,
npx thirdweb create --app --cra --js
If you're integrating into an existing project,
install the required dependencies to get started with thirdweb, by running the following command:
npm install @thirdweb-dev/sdk @thirdweb-dev/sdk ethers@5
Setting up the project
In your App.jsx
, we import the two components we're going to create in this guide:
Connect
componentAuction
component
import AuctionComponent from "./components/Auction";
import Connect from "./components/Connect";
function ExampleApp() {
return (
<>
<Connect />
<AuctionComponent />
</>
);
}
export default ExampleApp;
Connect Wallet button
Create a new folder called components
inside the src
directory, that will host all custom components of your app.
After that, create a new file called Connect.js
.
The component is very simple and only consists of the following code:
import { useAddress, useMetamask } from "@thirdweb-dev/react";
const Connect = () => {
const address = useAddress();
const connectWithMetamask = useMetamask();
if (address) {
return <p>Welcome, {address}</p>;
}
return (
<button onClick={() => connectWithMetamask()}>
Connect Metamask Wallet
</button>
);
};
export default Connect;
The Auction button
Create another file called Auction.js
inside your components
folder.
In this component, we'll create a form that allows a user to create an auction listing.
First, we import all the necessary dependencies.
import { useMarketplace } from "@thirdweb-dev/react";
import { NATIVE_TOKEN_ADDRESS } from "@thirdweb-dev/sdk";
Then fetch our marketplace using the useMarketplace
hook:
export default function Auction() {
// Connect to our marketplace contract via the useMarketplace hook
const marketplace = useMarketplace(
"0x277C0FB19FeD09c785448B8d3a80a78e7A9B8952", // Your marketplace contract address here
);
// rest of the code goes here
}
Here is the function that we'll run when the form is submitted, to create an auction listing:
async function createAuctionListing(e) {
// prevent page from refreshing
e.preventDefault();
// De-construct data from form submission
let { contractAddress, tokenId, price } = e.target.elements;
contractAddress = contractAddress.value;
tokenId = tokenId.value;
price = price.value;
try {
const transaction = await marketplace?.auction.createListing({
assetContractAddress: contractAddress, // Contract Address of the NFT
buyoutPricePerToken: price, // Maximum price, the auction will end immediately if a user pays this price.
currencyContractAddress: NATIVE_TOKEN_ADDRESS, // NATIVE_TOKEN_ADDRESS is the crpyto curency that is native to the network. e.g. ETH.
listingDurationInSeconds: 60 * 60 * 24 * 7, // When the auction will be closed and no longer accept bids (1 Week)
quantity: 1, // How many of the NFTs are being listed (useful for ERC 1155 tokens)
reservePricePerToken: 0, // Minimum price, users cannot bid below this amount
startTimestamp: new Date(), // When the listing will start
tokenId: tokenId, // Token ID of the NFT.
});
return transaction;
} catch (error) {
console.error(error);
}
}
Now let's create a form and store the values the user enters.
Declare some variables that will be passed through to identify our NFT we want to list:
return (
<form onSubmit={(e) => createAuctionListing(e)}>
{/* NFT Contract Address Field */}
<input
type="text"
name="contractAddress"
placeholder="NFT Contract Address"
/>
{/* NFT Token ID Field */}
<input type="text" name="tokenId" placeholder="NFT Token ID" />
{/* Sale Price For Listing Field */}
<input type="text" name="price" placeholder="Sale Price" />
{/* Submit button */}
<button type="submit">List NFT</button>
</form>
);
Conclusion
That's it! You can now check your demo by running:
npm run start
Learn more about what you can do with the marketplace contract on the
Marketplace pre-built contract page!