How to add 'Sign-in with Phone' to your web3 app 'Connect Wallet' Button.
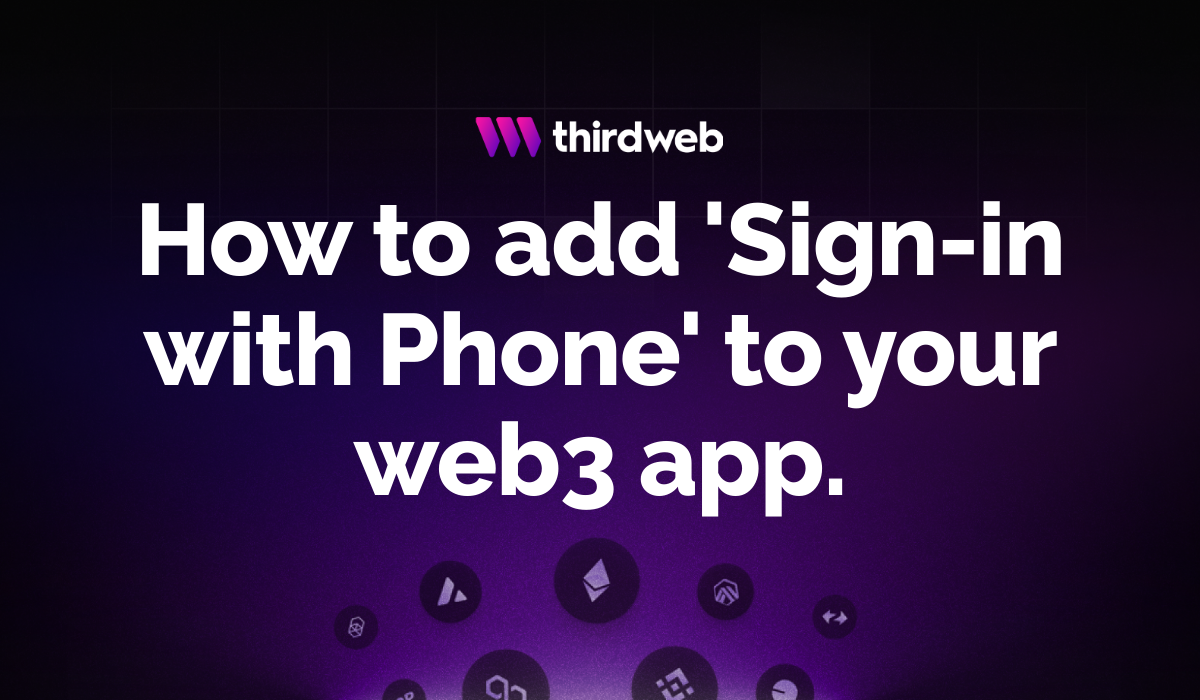
Introduction
In the future, every user on the internet will have a web3 wallet as their decentralized account. With the transaction time and cost trending towards zero, any developer can now build and scale onchain applications with UX that rivals web2 apps.
One of the key components to building a seamless web3 app is having a great onboarding flow for first time users. In-App Wallets make this possible by allowing users to sign-in with their phone, phone number or social media account.
Using thirdweb's Connect SDK, developers can enable any user to sign-up in their application regardless of their web3 knowledge. This can be combined with Account Abstraction, creating smart accounts for every user to sponsor gas transactions, so that users don't have to pay and find the correct gas token.
Here's how In-App Wallets work under the hood:
When a user signs into an application using their phone or social logins for the first time, a wallet is generated on the user's device. The corresponding wallet key for this wallet is securely split into three shards using Shamir's Secret Sharing algorithm.
- Shard A is stored securely on the user's device. For web applications, this is stored on the browser, or for mobile apps, it is in a secure enclave.
- Shard B is encrypted and stored in Amazon KMS using a key known to thirdweb. This process uses hardware security modules (HSMs) to protect the confidentiality and integrity of keys. The encrypted string is stored in thirdweb's database.
- Shard C is encrypted by user authentication and stored in Amazon's KMS using hardware security modules (HSMs) to protect the confidentiality and integrity of keys. Despite the key stored on thirdweb's servers, thirdweb has no way of decrypting and accessing the key.
In this tutorial, we'll show you how to add the Connect Button UI component from the thirdweb Connect SDK to your app, and add 'Sign-in with Phone' to your onboarding flow.
This will let anyone sign-in to your app or game using their Phone account – You can check out the video version of this tutorial here:
By the end of this guide, you'll know how to:
- Implement the default Connect Button
- Add a sign-in with phone option
- Configure the button's appearance and labels
- Customize the theme to match your app's branding
Prerequisites
Before we begin, make sure you have the following:
- A thirdweb account
- A wallet like MetaMask to connect to thirdweb
- Some test funds on a testnet of choice
Step 1: Set Up the Next.js Project
First, let's set up our Next.js project:
- Open a terminal and run:
npx thirdweb create app
- Select
Next.js
for the framework - Open the project in your code editor
- Edit the
.env
file and add yourclientID
Step 2: Implement the Default Connect Button
To implement the default Connect Button, all we need to do is add the ConnectButton
UI component and provide it with our client
:
<ConnectButton client={client} />
The client
is created for us in the client.ts
file:
import { createClient } from "thirdweb";
export const client = createClient({
clientId: process.env.NEXT_PUBLIC_CLIENT_ID,
});
With that one line of code, you now have a Connect Button where a user can connect a web3 wallet or create a web3 wallet and connect it to your application.
You can also change the modal size by adding the size
prop:
<ConnectButton
client={client}
connectModal={{
size: "compact",
}}
/>
Step 3: Add 'Sign-in With Phone'
Next, let's customize the wallets that are shown to the user in the Connect Modal.
thirdweb provides flexible options for onboarding both crypto native and non-crypto native users. thirdweb provides support for 350+ web3 wallets and with In-App wallets we can generate wallets for non-crypto native users with web3 authentication methods.
Add 'Sign-in with Phone' as an option on the connect modal. Set the Connect Button's supported wallets to inAppWallet
and set it's providers to phone
import { ConnectButton } from "thirdweb/react";
import { inAppWallet } from "thirdweb/wallets";
const client = createThirdwebClient({ clientId });
export default function App() {
return (
<ConnectButton
client={client}
wallets={inAppWallet({
providers: [
"phone"
],
})}
/>
);
}
Step 4: Configure the Button Appearance
You can also customize the appearance of the Connect Button, such as changing the button label and the modal title.
To change the button label, add the label
prop:
<ConnectButton
client={client}
connectButton={{
label: "Sign in",
}}
/>;
To customize the modal title, use the modalTitle
prop:
<ConnectButton
client={client}
connectModal={{
title: "Sign in to MyApp",
}}
/>
Step 5: Customize the Button Theme
Finally, let's customize the theme of the Connect Button to match your application's branding. You can change colors, fonts, and more to seamlessly integrate the button into your web3 app.
To get started, check out the Connect Button Playground in the documentation. Here, you can visually customize the button and see a live preview of your changes.
In the playground, you can:
- Toggle between compact and full-size modal
- Enable/disable phone and social logins
- Add or remove specific wallets
- Recommend certain wallets
- Change the button title and labels
- Customize the color scheme
Once you're happy with your customizations, go to the "code" tab. You'll find a code snippet with all your changes applied to the ConnectButton
component. Simply copy this code and paste it into your project.
Conclusion
In this tutorial, we covered the Connect Button UI component from the thirdweb Connect SDK. We learned how to:
- Implement the default Connect Button with a single line of code
- Customize the wallets displayed to users and recommend specific wallets
- Configure the button's appearance, labels, and modal size
- Customize the theme, colors, and fonts to match your application's branding
The Connect Button provides a powerful and flexible way to add wallet connection functionality to your web3 app. With its extensive customization options and support for 350+ wallets and web3 authentication methods, you can create a seamless onboarding experience for your users.