X Authentication
thirdweb SDK v5.55.0
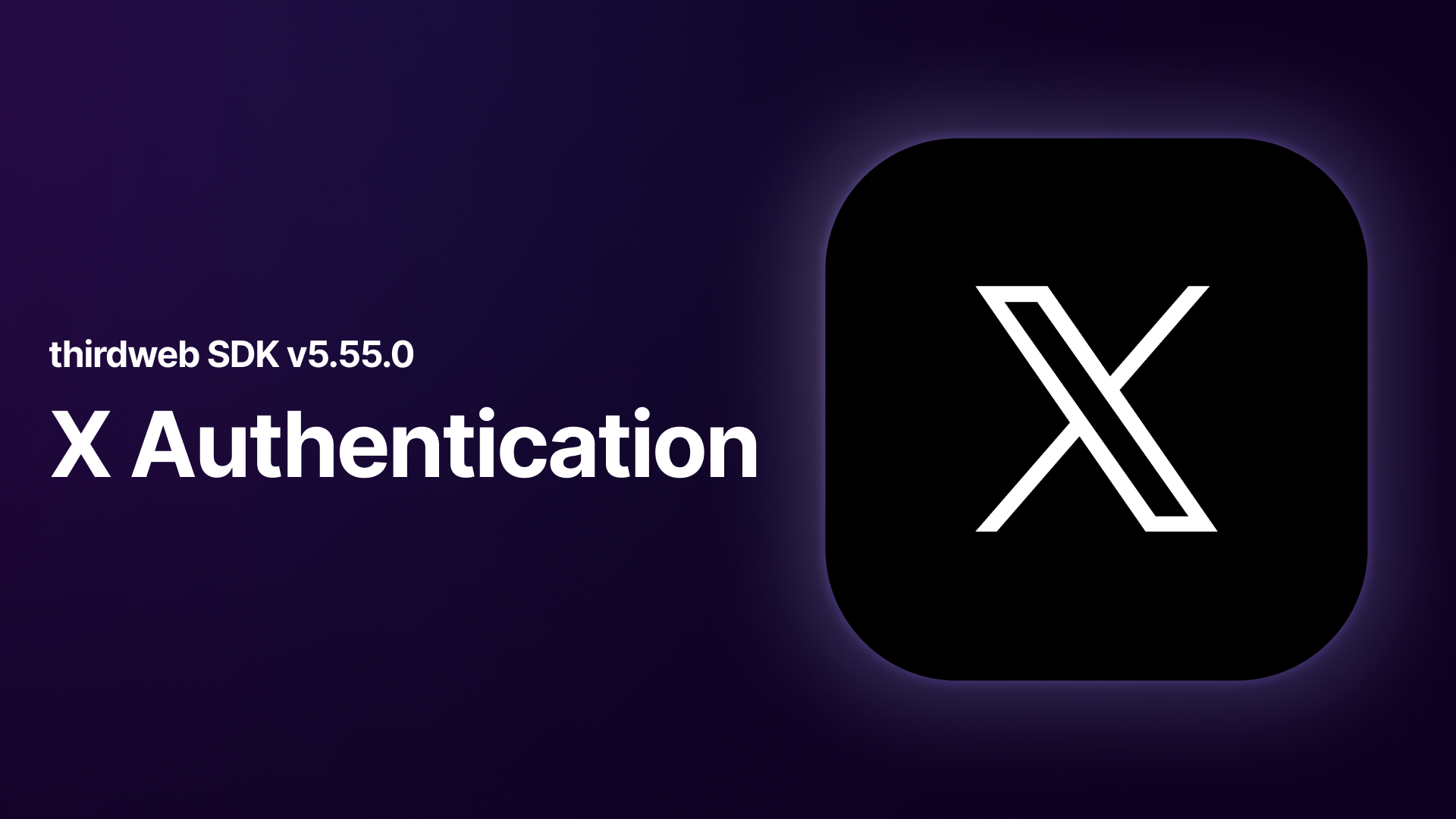
You can now login to an in-app wallet using X/Twitter.
import { inAppWallet } from "thirdweb/wallets";
const wallet = inAppWallet();
const account = await wallet.connect({
client,
chain,
strategy: "x",
});
This also works for linking accounts to an existing wallet.
import { inAppWallet, linkProfile } from "thirdweb/wallets";
const wallet = inAppWallet();
await wallet.connect({ strategy: "google" });
await linkProfile(wallet, { strategy: "x" });
Parse NFT URIs
We've added a parseNFTUri
utility function to parse NFT image URIs of all formats to an IPFS hash. We've also added a parseAvatarRecord
function to specifically parse ENS avatars to a usable HTTPS URL.
import { parseAvatarRecord } from "thirdweb/extensions/ens";
import { parseNftUri } from "thirdweb/extensions/common";
const avatarUrl = await parseAvatarRecord({
client,
uri: "...",
});
const nftUri = await parseNftUri({
client,
uri: "...",
});
Hide Specific Wallets in the Wallet Switcher
You can now hide specific wallet types in the React wallet switcher UI. This can be helpful if you'd like to use smart wallets without exposing the wallet address to the user, or in the opposite case to hide the underlying admin wallet from the user.
<ConnectButton
client={client}
detailsModal={{
// We hide the smart wallet from the user
hiddenWallets: ["smart"],
}}
accountAbstraction={{
chain: baseSepolia,
sponsorGas: true,
}}
/>
Bug Fixes and Other Improvements
- Specify your preferred provider on the
buyWithFiat
function usingpreferredProvider