Universal Bridge: Advanced Filters
The thirdweb SDK v5.97.0 and the Universal Bridge API have new advanced filtering options.
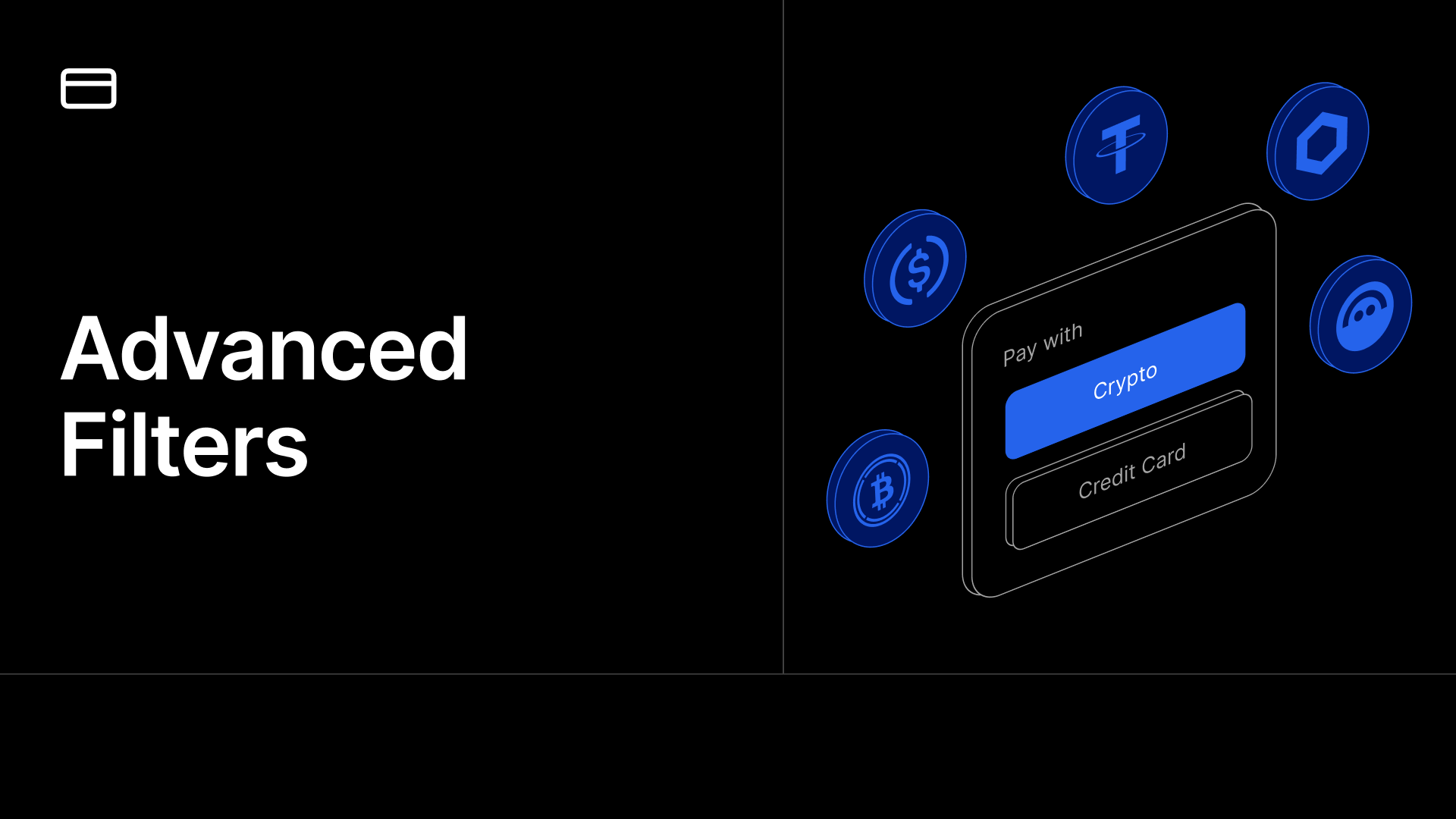
With the latest release of Universal Bridge, we've added a number of new filters to make building advanced payment UIs more convenient. These are available starting in v5.97.0 of the TypeScript SDK.
The sortBy
Filter
The /routes
endpoint (API) and Bridge.routes
function (SDK) now have an optional sortBy
option to determine how the returned routes will be ordered. The first option we've made available is sortBy: "popularity"
, which will ordered the returned routes based on the number of times they've been used. The following will return the 10 most popular routes originating from ETH on mainnet:
import { Bridge } from "thirdweb";
const routes = await Bridge.routes({
originChainId: 1,
originTokenAddress: "0xEeeeeEeeeEeEeeEeEeEeeEEEeeeeEeeeeeeeEEeE",
limit: 10,
offset: 0,
sortBy: "popularity",
client: thirdwebClient,
});
[
{
"originToken": {
"chainId": 8453,
"address": "0x833589fCD6eDb6E08f4c7C32D4f71b54bdA02913",
"iconUri": "https://coin-images.coingecko.com/coins/images/6319/large/usdc.png?1696506694",
"symbol": "USDC",
"name": "USD Coin",
"decimals": 6
},
"destinationToken": {
"chainId": 466,
"address": "0x675C3ce7F43b00045a4Dab954AF36160fb57cB45",
"iconUri": "https://coin-images.coingecko.com/coins/images/6319/large/usdc.png?1696506694",
"symbol": "USDC",
"name": "USD Coin",
"decimals": 6
}
},
{
"originToken": {
"chainId": 42161,
"address": "0xaf88d065e77c8cC2239327C5EDb3A432268e5831",
"iconUri": "https://ethereum-optimism.github.io/data/USDC/logo.png",
"symbol": "USDC",
"name": "USD Coin",
"decimals": 6
},
"destinationToken": {
"chainId": 466,
"address": "0x675C3ce7F43b00045a4Dab954AF36160fb57cB45",
"iconUri": "https://coin-images.coingecko.com/coins/images/6319/large/usdc.png?1696506694",
"symbol": "USDC",
"name": "USD Coin",
"decimals": 6
}
},
{
"originToken": {
"chainId": 1,
"address": "0xEeeeeEeeeEeEeeEeEeEeeEEEeeeeEeeeeeeeEEeE",
"iconUri": "https://assets.relay.link/icons/1/light.png",
"symbol": "ETH",
"name": "Ether",
"decimals": 18
},
"destinationToken": {
"chainId": 8453,
"address": "0xEeeeeEeeeEeEeeEeEeEeeEEEeeeeEeeeeeeeEEeE",
"iconUri": "https://assets.relay.link/icons/1/light.png",
"symbol": "ETH",
"name": "Ether",
"decimals": 18
}
},
...
]
The maxSteps
Filter
The maxSteps
filter already allows you to filter routes
based on the number of steps required to fulfill a routes. With this update, we've added this same option to the Buy.quote
, Buy.prepare
, Sell.quote
, and Sell.prepare
functions, as well as their corresponding API endpoints. You can now guarantee the quote returned uses a certain number of steps or fewer:
import { Bridge } from "thirdweb"
const quote = await Bridge.Buy.quote({
originChainId: 1,
originTokenAddress: "0x...",
destinationChainId: 137,
destinationTokenAddress: "0x...",
amount: 1000000n,
maxSteps: 2,
});
const preparedQuote = await Bridge.Buy.prepare({
originChainId: 1,
originTokenAddress: "0x...",
destinationChainId: 137,
destinationTokenAddress: "0x...",
amount: 1000000n,
sender: "0x...",
receiver: "0x...",
maxSteps: 2,
});
const quote = await Bridge.Sell.quote({
originChainId: 1,
originTokenAddress: "0x...",
destinationChainId: 137,
destinationTokenAddress: "0x...",
amount: 1000000n,
maxSteps: 3,
});
const preparedQuote = await Bridge.Sell.prepare({
originChainId: 1,
originTokenAddress: "0x...",
destinationChainId: 137,
destinationTokenAddress: "0x...",
amount: 1000000n,
sender: "0x...",
receiver: "0x...",
maxSteps: 3,
});
The Chains Endpoint
We've added a new endpoint and SDK function to get all chains supported by Universal Bridge along with their name, icon, and native currency.
import { Bridge } from "thirdweb";
const chains = await Bridge.chains({
client: thirdwebClient,
});
This will return:
[
{
"chainId": 1868,
"name": "Soneium Mainnet",
"icon": "ipfs://QmPSGdP5WKe2bC5JCo43JjWff8Agw7dg6WQpmUktyW7sat",
"nativeCurrency": {
"name": "Ethereum",
"symbol": "ETH",
"decimals": 18
}
},
{
"chainId": 466,
"name": "AppChain",
"icon": "ipfs://bafybeigx76uxvcvet7365sjdzuxxcgl5auzck6vbbigu2jeg6ixl5k3tya",
"nativeCurrency": {
"name": "Ether",
"symbol": "ETH",
"decimals": 18
}
},
{
"chainId": 42161,
"name": "Arbitrum One",
"icon": "ipfs://QmcxZHpyJa8T4i63xqjPYrZ6tKrt55tZJpbXcjSDKuKaf9/arbitrum/512.png",
"nativeCurrency": {
"name": "Ether",
"symbol": "ETH",
"decimals": 18
}
},
...
]
Bug Fixes
- Fixed an issue where Coinbase onramps were not returning the associated transaction hash
- Fixed routes returning the zero address rather than the native token address