thirdweb SDKs Major Update
We're excited to announce version 3.0 of our Typescript and React SDKs! 🥳
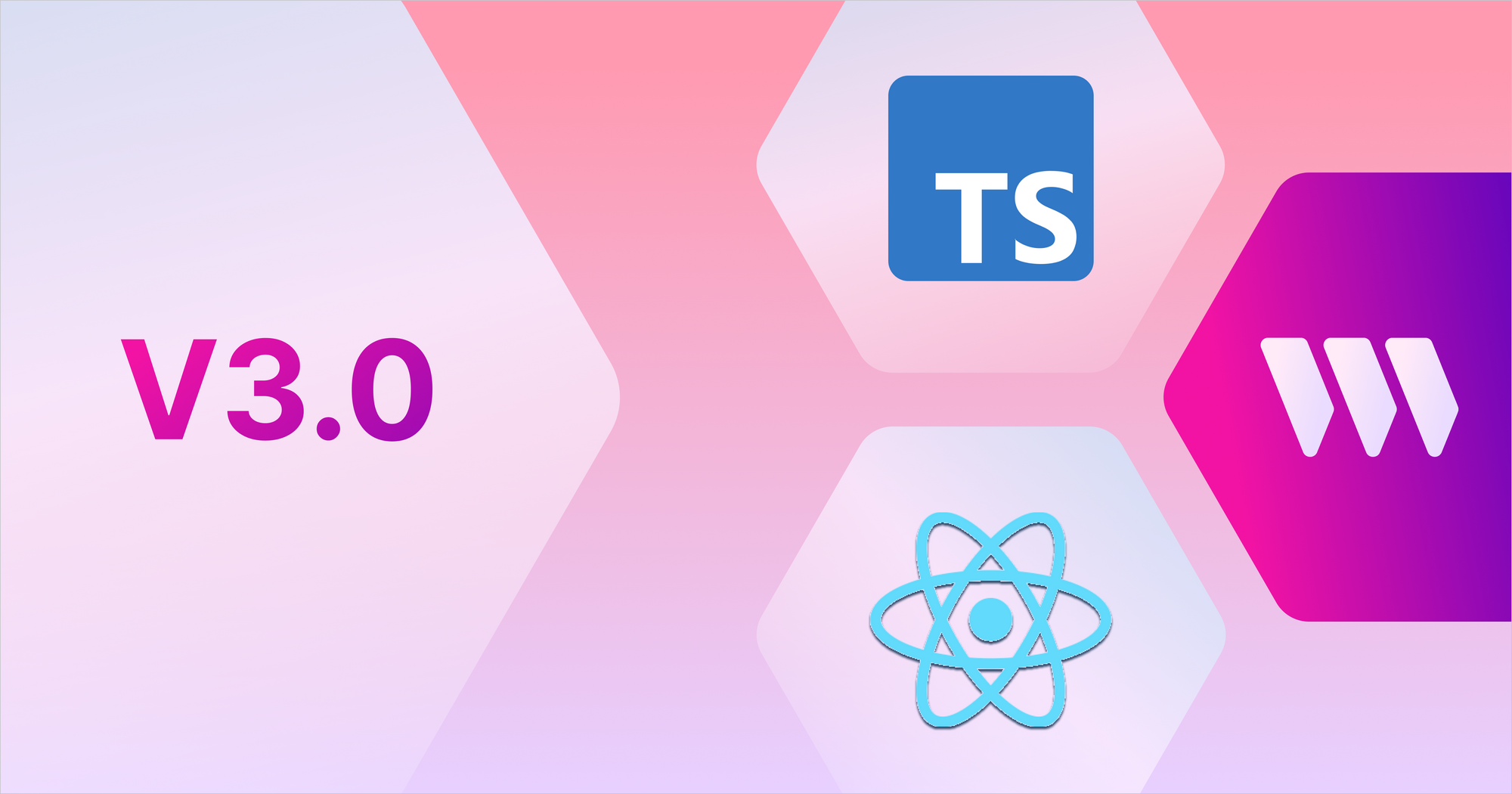
We're excited to announce version 3.0 of our Typescript and React SDKs! 🥳
🚀 How to upgrade
npm install @thirdweb-dev/sdk @thirdweb-dev/react
Let's take a look at the most notable changes.
🤯 Package sizes reduced by 8x!
The size of the Typescript and React SDKs has been reduced by 87%! This is possible using dynamic imports, meaning we only load the things you use at runtime, rather than everything up front.
Here are the differences in size and download times for the v2.x vs v3.x Typescript SDK:
version 2.x | version 3.x | Change | |
---|---|---|---|
Minified size | 2.4 MB | 314.4 Kb | -86.9% |
Minified + Gzip | 481.4 Kb | 60.8 Kb | -87.4% |
Download time (4g) | 0.55 s | 69 ms | -87.5% |
Download time (3g) | 9.63 s | 1.22 s | -87.3% |
⭐️ Improved API for interacting with your contracts
Both Typescript and React SDKs now treat your contracts as first class citizens with a new high level API. That means you get all the power of the SDK with contracts that you write yourself using our contract Extensions.
Let’s say I want to build my own ERC1155 with lazy minting capability and my own logic. I can use the thirdweb CLI to quickly setup a contract project, starting from a base contract.
$ npx thirdweb create
? What type of project do you want to create?
> Contract
? What contract do you want to start from?
> ERC1155 + Lazy Mint
Once I finish adding my own logic to this base contract, I can deploy using thirdweb deploy.
$ npx thirdweb deploy
That automatically generates a dashboard page for my contract, and lets me use the SDKs to interact with my deployed contract. Since my contract is an ERC1155, I get all the ERC1155 high level APIs
TypeScript:
const myContract = await sdk.getContract(MY_CONTRACT_ADDRESS);
// lazy mint some NFTs, automatically uploaded to IPFS
await myContract.erc1155.lazyMint(myNFTs);
// get all NFTs (with IPFS metadata) from my contract
await myContract.erc1155.getAll();
// claim an NFT to the connected wallet
await myContract.erc1155.claim(tokenId, quantity);
React:
const myContract = useContract(MY_CONTRACT_ADDRESS);
// lazy mint some NFTs, automatically uploaded to IPFS
const { mutate: lazyMint } = useLazyMint(myContract);
// get all NFTs (with IPFS metadata) from my contract
const { data: nfts } = useNFTs(myContract);
// claim an NFT to the connected wallet
const { mutate: claim } = useClaimNFT(myContract);
Using our Web3Button
component
<Web3Button
contractAddress={MY_CONTRACT_ADDRESS}
action={(contract) => contract.erc1155.claim(tokenId, quantity) }
>
Claim
</Web3Button>
This pattern works for erc20
/ erc721
and erc1155
standards and supports a wide variety of convenience functions to read and write data to your own contracts. For a full list of all the functionality you can unlock in the SDKs, take a look at the Extensions section and SDK section on our portal.
Breaking changes
These improvements come with some breaking changes that you should watch out for when you upgrade.
Typescript SDK
Getting contracts is now async. This allows dynamically importing contracts and reduces the weight of the SDK significantly.
before:
const token = sdk.getToken(...)
const nftDrop = sdk.getNFTDrop(...)
after:
// need to add 'await' here to get each contract
const token = await sdk.getToken(...)
const nftDrop = await sdk.getNFTDrop(...)
React SDK
All contracts, prebuilt or custom, can now be used using the useContract()
hook. For prebuilt contracts, you can specify the contract type in the second parameter.
before:
const contract = useNFTCollection(MY_CONTRACT_ADDRESS);
after:
// contract type in second param (eg. nft-drop, nft-collection)
const { contract } = useContract(MY_CONTRACT_ADDRESS, "nft-collection");
React Hooks now accept custom contracts directly and handle the logic internally
before:
const { contract } = useContract(...)
const { data: nfts } = useNFTs(contract?.nft)
const { mutation: claim } = useClaimNFT(contract?.nft)
after:
const { contract } = useContract(...)
// works with any ERC721/ERC1155 contract
const { data: nfts} = useNFTs(contract)
const { mutation: claim } = useClaimNFT(contract)
Custom contract hooks to read and write data got renamed:
before:
useContractData(...)
useContractCall(...)
after:
useContractRead(...)
useContractWrite(...)
Community
We'd like to thank our community in Discord for constantly shipping with us and giving your feedback to make the experience of using our SDKs better. Keep shipping! 🚢