New Modular Contract TypeScript API
Deploy, configure and interact with the new thirdweb Modular Contracts.
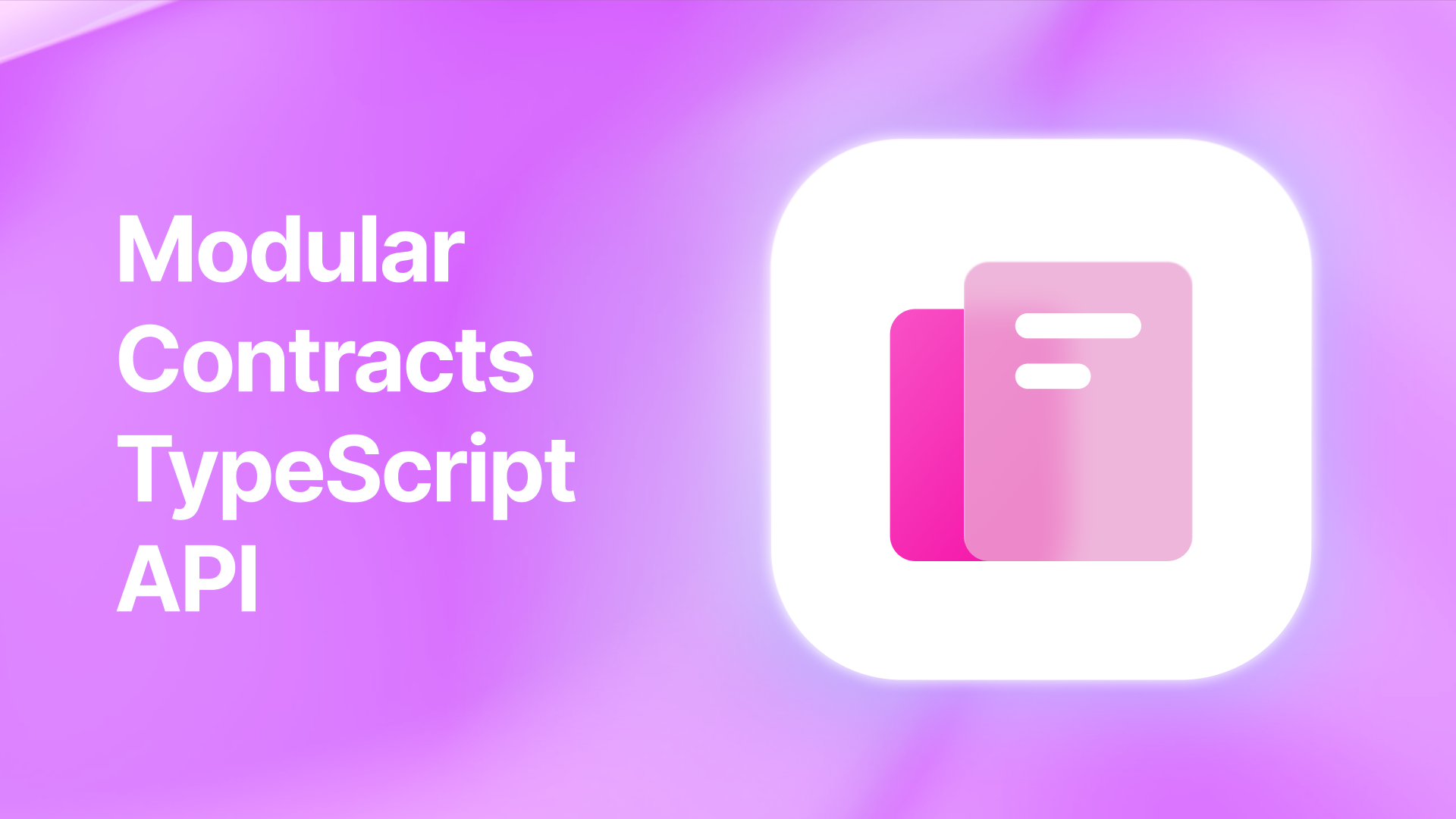
We just released v5.52.0 of the thirdweb TypeScript SDK which unlocks the ability to deploy, configure and interact with our new Modular Contracts.
Deploying a Modular Contract
You can now easily build your personalized contract by picking the modules suited for your use case.
Here's how to deploy a ERC721 contract with the Claimable and BatchMetadata module, which together create a Drop functionality.
import {
ClaimableERC721,
BatchMetadataERC721,
deployModularContract,
} from "thirdweb/modules";
const deployed = deployModularContract({
client,
chain,
account,
core: "ERC721",
params: {
name: "My Modular NFT Contract",
},
modules: [
ClaimableERC721.module({
primarySaleRecipient: ...,
}),
BatchMetadataERC721.module(),
],
});
Upgrading a Modular Contract
These modules can be swapped at any time, and new ones can also be added post deployment. Here's how to install a new module programmatically:
import {
RoyaltyERC721,
} from "thirdweb/modules";
const transaction = RoyaltyERC721.install({
contract: coreContract,
account,
params: {
royaltyRecipient: account.address,
royaltyBps: 100n,
transferValidator: ZERO_ADDRESS,
},
});
})
Interacting with a Modular Contract
We have core contracts for ERC20, ERC721 and ERC1155 standards. Those follow the standard ERCs and can be interacted with like usual with the standard extensions.
The interesting part is to interact with an attached module. This is done by using the defined module name spaced API:
import { ClaimableERC721 } from "thirdweb/modules";
const transaction = ClaimableERC721.mint({
contract,
to: "0x...", // Address to mint tokens to
quantity: 2, // Amount of tokens to mint
});
// Send the transaction
await sendTransaction({ transaction, account });
This makes it easy to discover the available API for each module.
You will also soon be able to generate the namespaced TypeScript API for your own modules via the thirdweb CLI.
To learn more about modular contracts and how to create modules, head over to the documentation.