.NET/Unity - Insight Indexer and Engine Wallet Integration
Bringing our best products right to your favorite framework, fully integrated with other APIs.
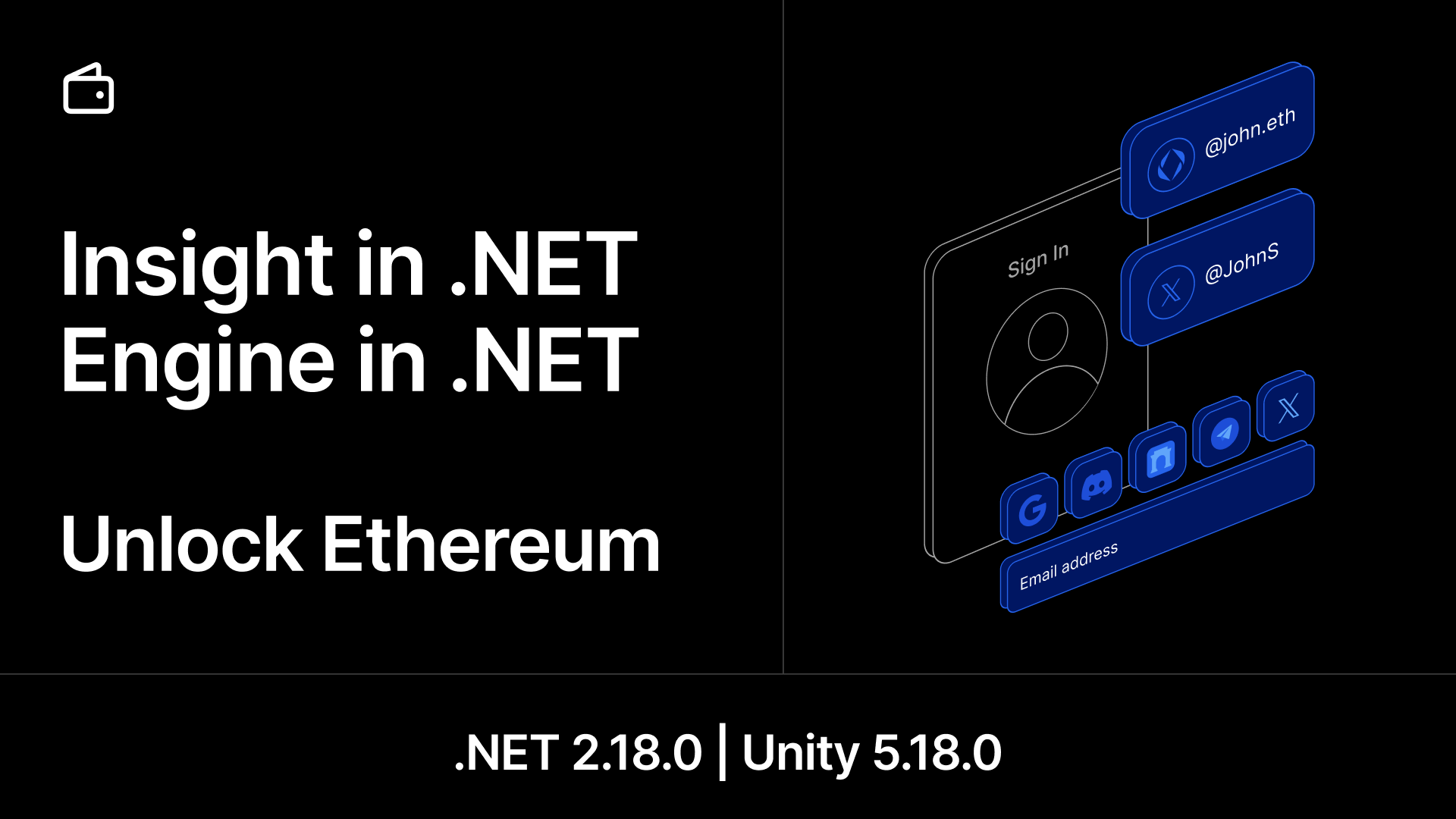
What's Changed
Insight Indexer .NET Integration
Insight is an extremely useful and performant tool to query blockchain data and can decorate it quite nicely too.
Did you know that vitalik, on Ethereum, Polygon and Arbitrum alone owns 7811 ERC20s, 34,362 ERC721s and 2,818 ERC1155s?
Let's go through some examples!
Instantiation
using Thirdweb.Indexer;
// Create a ThirdwebInsight instance
var insight = await ThirdwebInsight.Create(client);
Simple Args
// Setup some filters
var address = await Utils.GetAddressFromENS(client, "vitalik.eth");
var chains = new BigInteger[] { 1, 137, 42161 };
Fetching all tokens owned by a given address
// Fetch all token types
var tokens = await insight.GetTokens(address, chains);
Console.WriteLine($"ERC20 Count: {tokens.erc20Tokens.Length} | ERC721 Count: {tokens.erc721Tokens.Length} | ERC1155 Count: {tokens.erc1155Tokens.Length}");
Fetching a specific type of token owned by a given address
// Fetch ERC20s only
var erc20Tokens = await insight.GetTokens_ERC20(address, chains);
Console.WriteLine($"ERC20 Tokens: {JsonConvert.SerializeObject(erc20Tokens, Formatting.Indented)}");
// Fetch ERC721s only
var erc721Tokens = await insight.GetTokens_ERC721(address, chains);
Console.WriteLine($"ERC721 Tokens: {JsonConvert.SerializeObject(erc721Tokens, Formatting.Indented)}");
// Fetch ERC1155s only
var erc1155Tokens = await insight.GetTokens_ERC1155(address, chains);
Console.WriteLine($"ERC1155 Tokens: {JsonConvert.SerializeObject(erc1155Tokens, Formatting.Indented)}");
Fetching Events
Note: most of these parameters are optional (and some are not showcased here)
// Fetch events (great amount of optional filters available)
var events = await insight.GetEvents(
chainIds: new BigInteger[] { 1 }, // ethereum
contractAddress: "0xbc4ca0eda7647a8ab7c2061c2e118a18a936f13d", // bored apes
eventSignature: "Transfer(address,address,uint256)", // transfer event
fromTimestamp: Utils.GetUnixTimeStampNow() - 3600, // last hour
sortBy: SortBy.TransactionIndex, // block number, block timestamp or transaction index
sortOrder: SortOrder.Desc, // latest first
limit: 5 // last 5 transfers
);
Console.WriteLine($"Events: {JsonConvert.SerializeObject(events, Formatting.Indented)}");
Engine Blockchain API .NET Integration
Engine is one of thirdweb's most important products. We've created an EngineWallet
class that can be used as an IThirdwebWallet
and as such benefits from being compatible with the entire SDK!
Usage:
// EngineWallet is compatible with IThirdwebWallet and can be used with any SDK method/extension
var engineWallet = await EngineWallet.Create(
client: client,
engineUrl: Environment.GetEnvironmentVariable("ENGINE_URL"),
authToken: Environment.GetEnvironmentVariable("ENGINE_ACCESS_TOKEN"),
walletAddress: Environment.GetEnvironmentVariable("ENGINE_BACKEND_WALLET_ADDRESS"),
timeoutSeconds: null, // no timeout
additionalHeaders: null // can set things like x-account-address if using basic session keys
);
// Simple self transfer
var receipt = await engineWallet.Transfer(chainId: 11155111, toAddress: await engineWallet.GetAddress(), weiAmount: 0);
Console.WriteLine($"Receipt: {receipt}");
Integrated with last December's EIP-7702 release!
Additional Changes
- Arbitrum, Arbitrum Nova and Arbitrum Sepolia gas price related methods now get shortcut early and return maxFeePerGas = maxPriorityFeePerGas = current gas price.
Utils.GetChainMetadata
chain id related variables updated fromint
toBigInteger
.