.NET SDK 1.0.0
Taking things to the next level.
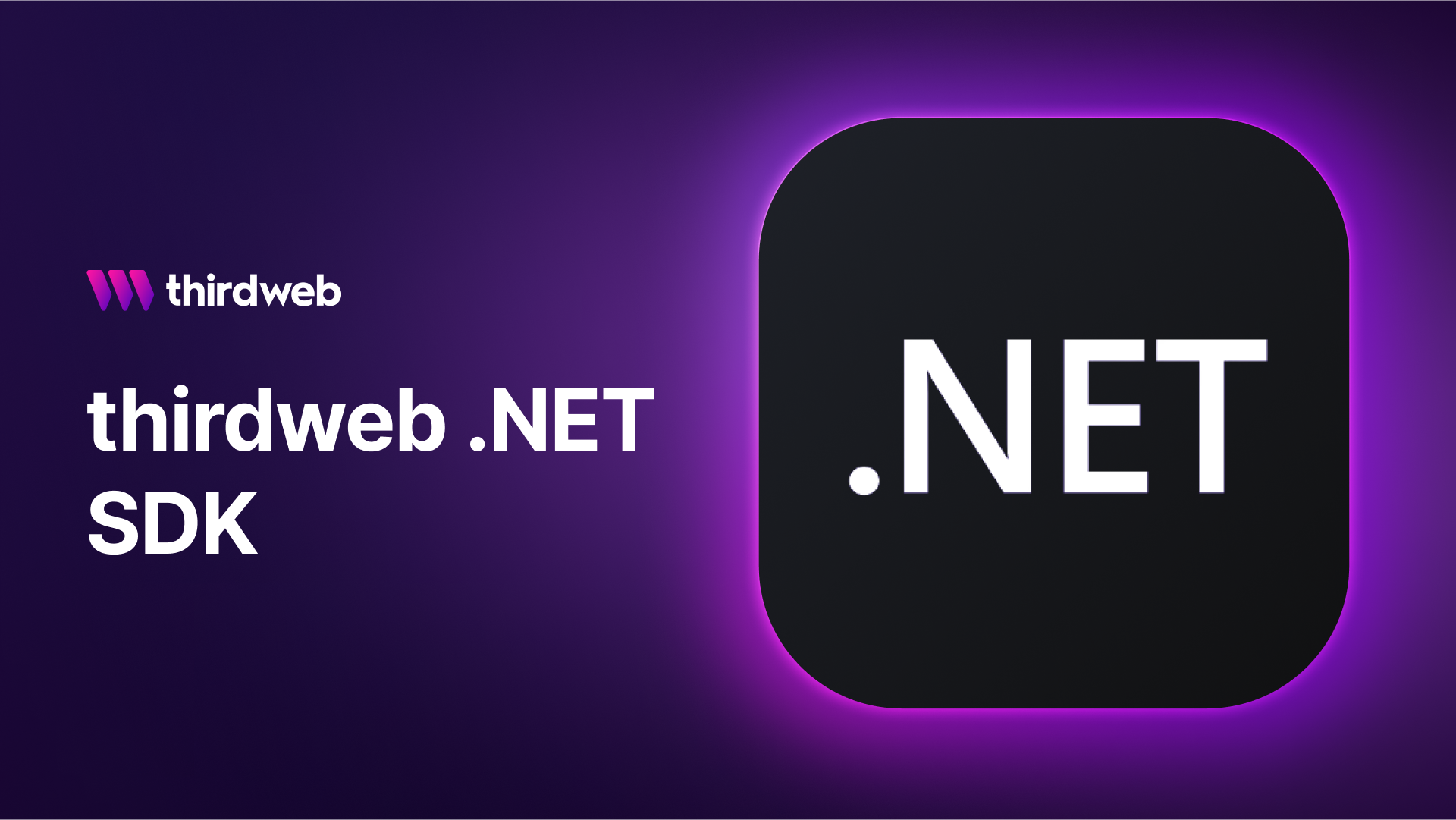
Thirdweb's .NET SDK has been in development for a couple of months now, and we're finally releasing the first major version packed with a ton of features!
If you want to jump straight into the docs, click here.
The NuGet Package is available here.
Platform-Agnostic & Performant
All internal classes and some external APIs have been reworked to be more generic and target even more platforms and frameworks. These changes allow for maximum customization and will also lay the ground work for our upcoming Unity major release.
Outside of gaming frameworks, we've also drastically improved performance when it comes to both networking and cryptography, respectively implementing throttling, batching and caching techniques as well as better algorithms for encryption and decryption. We've also made sure nothing blocks the main thread — specially useful for single threaded environments.
Finally, we've simplified some external APIs, so you may notice less required parameters and more optional parameters.
Extension Framework
We've added a very large amount of extensions that makes interacting with any contract much easier. These extensions simplify the process of interacting with smart contracts by providing a more intuitive and user-friendly API.
// Without extensions
var receiver = await wallet.GetAddress();
var quantity = BigInteger.One;
var currency = Constants.NATIVE_TOKEN_ADDRESS;
var pricePerToken = BigInteger.Zero;
var allowlistProof = new object[] { new byte[] { }, BigInteger.Zero, BigInteger.Zero, Constants.ADDRESS_ZERO };
var data = new byte[] { };
var receipt = await ThirdwebContract.Write(smartAccount, contract, "claim", 0, receiver, quantity, currency, pricePerToken, allowlistProof, data);
// With extensions
var receipt = await contract.DropERC20_Claim(wallet, receiver, amount);
// Can also do read operations and much more
var balance = await contract.ERC20_BalanceOf("0xOwnerAddress");
Contract extensions for common ERC20, ERC721 and ERC1155 have been added.
Contract extensions for thirdweb's TokenERC20, DropERC20, TokenERC721, DropERC721, TokenERC1155, DropERC1155 have been added.
Contract & Wallet extensions to get the native or ERC20 balance has been added.
NFT Extensions to get all 721 or 1155 NFTs from a contract or user address has also been added (supports enumerable contracts too). The returned type is a List of NFT
which contains all your NFT details and metadata. Extensions for that type also exist to download the image bytes, rather than going through our storage classes.
Speaking of storage, we've also added UploadRaw
for in-memory uploads.
Thirdweb Pay
Unlocking OnRamps and Cross-Chain crypto swaps!
Thirdweb Pay is now available in .NET.
Buy With Crypto Example
using Thirdweb.Pay;
// Swap Polygon MATIC to Base ETH
var swapQuoteParams = new BuyWithCryptoQuoteParams(
fromAddress: walletAddress,
fromChainId: 137,
fromTokenAddress: Thirdweb.Constants.NATIVE_TOKEN_ADDRESS,
toTokenAddress: Thirdweb.Constants.NATIVE_TOKEN_ADDRESS,
toChainId: 8453,
toAmount: "0.1"
);
var swapQuote = await ThirdwebPay.GetBuyWithCryptoQuote(client, swapQuoteParams);
Console.WriteLine($"Swap quote: {JsonConvert.SerializeObject(swapQuote, Formatting.Indented)}");
// Initiate swap
var txHash = await ThirdwebPay.BuyWithCrypto(wallet: privateKeyWallet, buyWithCryptoQuote: swapQuote);
Console.WriteLine($"Swap transaction hash: {txHash}");
// Poll for status
var currentSwapStatus = SwapStatus.NONE;
while (currentSwapStatus is not SwapStatus.COMPLETED and not SwapStatus.FAILED)
{
var swapStatus = await ThirdwebPay.GetBuyWithCryptoStatus(client, txHash);
currentSwapStatus = Enum.Parse<SwapStatus>(swapStatus.Status);
Console.WriteLine($"Swap status: {JsonConvert.SerializeObject(swapStatus, Formatting.Indented)}");
await Task.Delay(5000);
}
Buy With Fiat Example
// Get a Buy with Fiat quote
var fiatQuoteParams = new BuyWithFiatQuoteParams(
fromCurrencySymbol: "USD",
toAddress: walletAddress,
toChainId: "137",
toTokenAddress: Thirdweb.Constants.NATIVE_TOKEN_ADDRESS,
toAmount: "20"
);
var fiatOnrampQuote = await ThirdwebPay.GetBuyWithFiatQuote(client, fiatQuoteParams);
Console.WriteLine($"Fiat onramp quote: {JsonConvert.SerializeObject(fiatOnrampQuote, Formatting.Indented)}");
// Get a Buy with Fiat link
var onRampLink = ThirdwebPay.BuyWithFiat(fiatOnrampQuote);
Console.WriteLine($"Fiat onramp link: {onRampLink}");
// Open onramp link to start the process (use your framework's version of this)
var psi = new ProcessStartInfo { FileName = onRampLink, UseShellExecute = true };
_ = Process.Start(psi);
// Poll for status
var currentOnRampStatus = OnRampStatus.NONE;
while (currentOnRampStatus is not OnRampStatus.ON_RAMP_TRANSFER_COMPLETED and not OnRampStatus.ON_RAMP_TRANSFER_FAILED)
{
var onRampStatus = await ThirdwebPay.GetBuyWithFiatStatus(client, fiatOnrampQuote.IntentId);
currentOnRampStatus = Enum.Parse<OnRampStatus>(onRampStatus.Status);
Console.WriteLine($"Fiat onramp status: {JsonConvert.SerializeObject(onRampStatus, Formatting.Indented)}");
await Task.Delay(5000);
}
Custom Authentication
InAppWallet
now includes additional login methods to support custom authentication features thirdweb offers, including OIDC-compatible auth or your own generic authentication.
Learn more here.
Miscellaneous
SmartWallet
creation no longer requires an AccountFactory - on most chains, you may use thirdweb's default Account Factory.
AWS-related code has been migrated to their REST API, the AWS SDK is no longer a dependency, making our SDK a lot more portable (specially to web frameworks).
Large amount of fixes and improvements that we hope you'll notice as you go!
What's Next
Unity 5.0!
Learn More
Always refer to the Portal documentation to get started.
For a deeper insight into every class that is updated frequently, see the Full API Reference.
The source code is available on github, as always!