Localization API added in React SDK
Localization API added in thirdweb React SDK
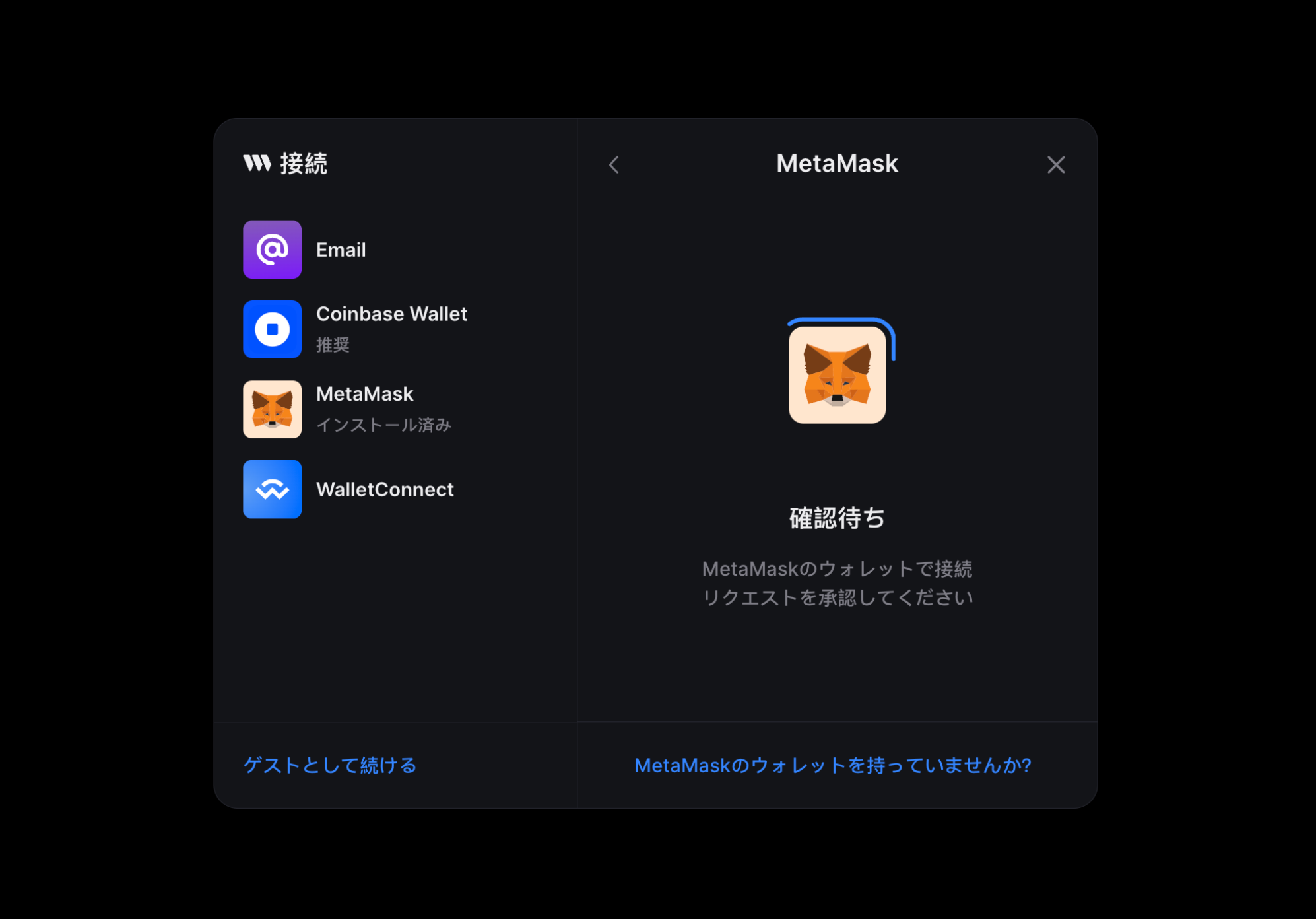
thirdweb React SDK now has a localization API that allows you to change the language used in UI components or override the texts used in the UI
A new prop locale
is added to the ThirdwebProvider
component that takes a locale object containing all the strings used by all the components.
React SDK comes out of the box with Spanish and Japanese locale functions, but you can add support for any language you want just by passing an object with the required strings
Using the default locales
Spanish
import { ThirdwebProvider, es } from "@thirdweb-dev/react";
const spanish = es();
<ThirdwebProvider locale={spanish}>
<App />
</ThirdwebProvider>
Setting Spanish locale
Japanese
import { ThirdwebProvider, ja } from "@thirdweb-dev/react";
const japanese = ja();
<ThirdwebProvider locale={japanese}>
<App />
</ThirdwebProvider>
setting Japanese locale
English ( default )
If no locale
prop is set, it defaults to en
as shown below
import { ThirdwebProvider, en } from "@thirdweb-dev/react";
const english = en();
<ThirdwebProvider locale={english}>
<App />
</ThirdwebProvider>
default
Try the ConnectWallet Playground to see the localized UI in action!
Overriding the locale object
Since locale
takes an object, you can decide to pass a completely custom object or override the default locale objects. There is a handy API for overriding locale object on the locale function:
import { ThirdwebProvider, en } from "@thirdweb-dev/react";
// override some texts
const english = en({
connectWallet: {
confirmInWallet: "Confirm in your wallet",
},
wallets: {
metamaskWallet: {
connectionScreen: {
inProgress: "Awaiting Confirmation",
instruction: "Accept connection request in your MetaMask wallet",
},
},
},
});
<ThirdwebProvider locale={english}>
<App />
</ThirdwebProvider>;
use the default English locale texts and override some texts
Custom locale object
If you want to support any other language, you can pass a custom object to locale
prop with all the required strings.
import { ThirdwebProvider } from "@thirdweb-dev/react";
<ThirdwebProvider locale={{ .... }}>
<App />
</ThirdwebProvider>;