LINE Auth, Fetch Users by Email, Contract Deploy Salts, and More
thirdweb SDK v5.54.0
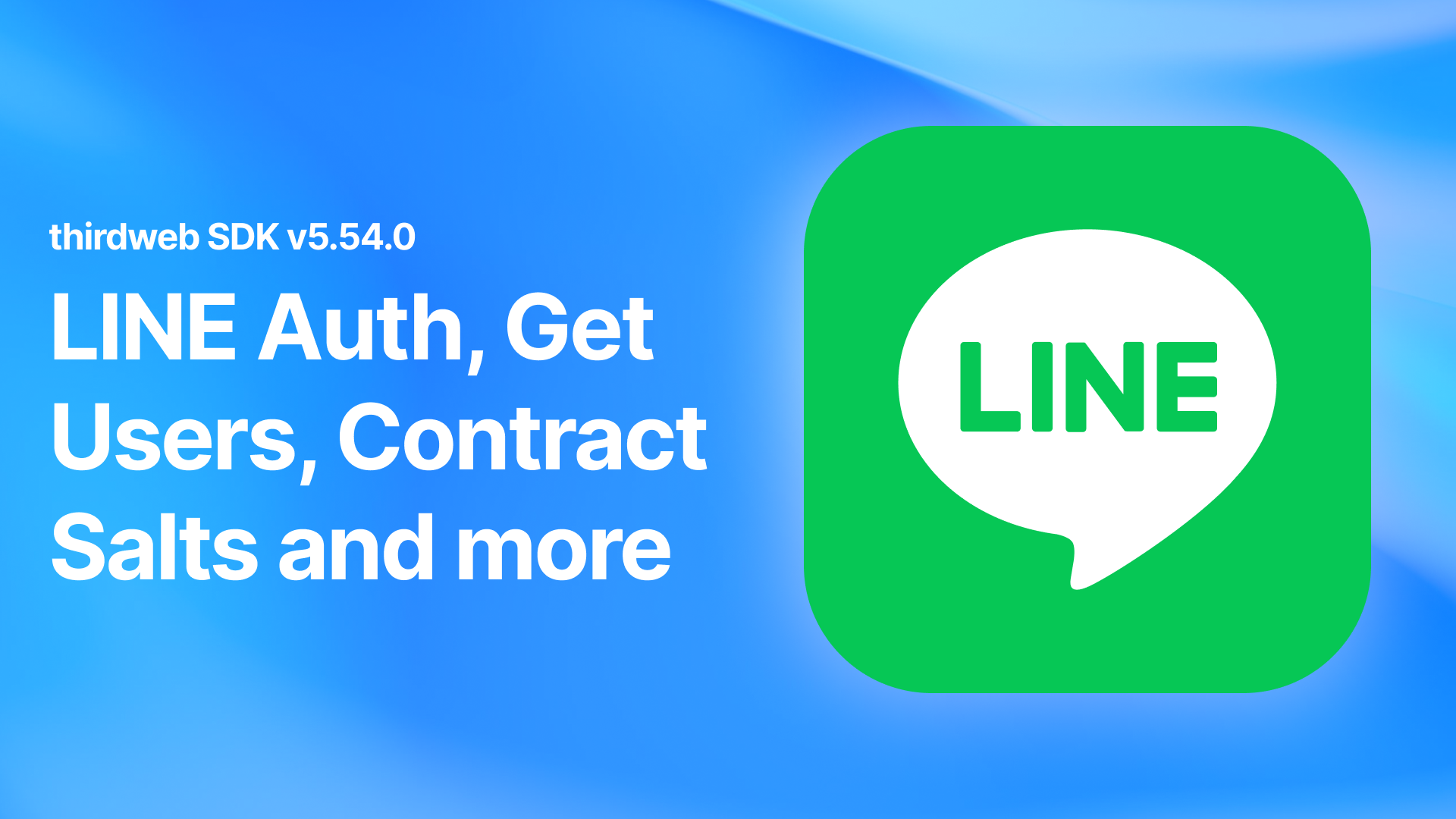
LINE Authentication
LINE is now supported as an authentication method across the SDK.
import { inAppWallet } from "thirdweb/wallets";
const wallet = inAppWallet();
const account = await wallet.connect({
client,
chain,
strategy: "line",
});
This includes linking an account to form a unified identity.
import { inAppWallet, linkProfile } from "thirdweb/wallets";
const wallet = inAppWallet();
await wallet.connect({ strategy: "google" });
await linkProfile(wallet, { strategy: "line" });
Fetch Users on the Server with getUser
You can now retrieve users by email, phone number, wallet address, or user ID from the server in a single line of code.
import { getUser } from "thirdweb/wallets";
const user = await getUser({
client,
email: "vitalik@ethereum.org"
});
⚠️
This function will only work with a secret key and should not be used on the client under any circumstances.
Salts for Deterministic Contract Deploys
You can now use a salt to deterministically deploy published contracts.
const address = await deployPublishedContract({
client,
chain,
account,
contractId: "Airdrop",
contractParams: {
defaultAdmin: "0x...",
contractURI: "ipfs://...",
},
salt: "test", // <--- deterministic deploy
});
This also works with unpublished contracts deployed via deployContract
.
const address = await deployContract({
client,
chain,
account,
bytecode: "0x...",
abi: contractAbi,
constructorParams: {
param1: "value1",
param2: 123,
},
salt: "test", // <--- deterministic deploy
});
deployPublishedContract
Constructor Parameters Format
Constructor parameters passed to deployPublishedContract
are now passed as an object rather than an array.
const address = await deployPublishedContract({
account,
chain,
client,
contractId: "Airdrop",
contractParams: {
defaultAdmin: TEST_ACCOUNT_A.address,
contractURI: "",
},
});
Bug Fixes and Other Improvements
- Added support for React Native 0.75
- Fixed ERC721 delayed reveal detection
- Multiple smart wallet transactions can now be sent in parallel
- Added the
toFunctionSelector
utility - Social profiles now display in the linked accounts list
- Polygon MATIC is now POL