Introducing: thirdweb Headless UI components
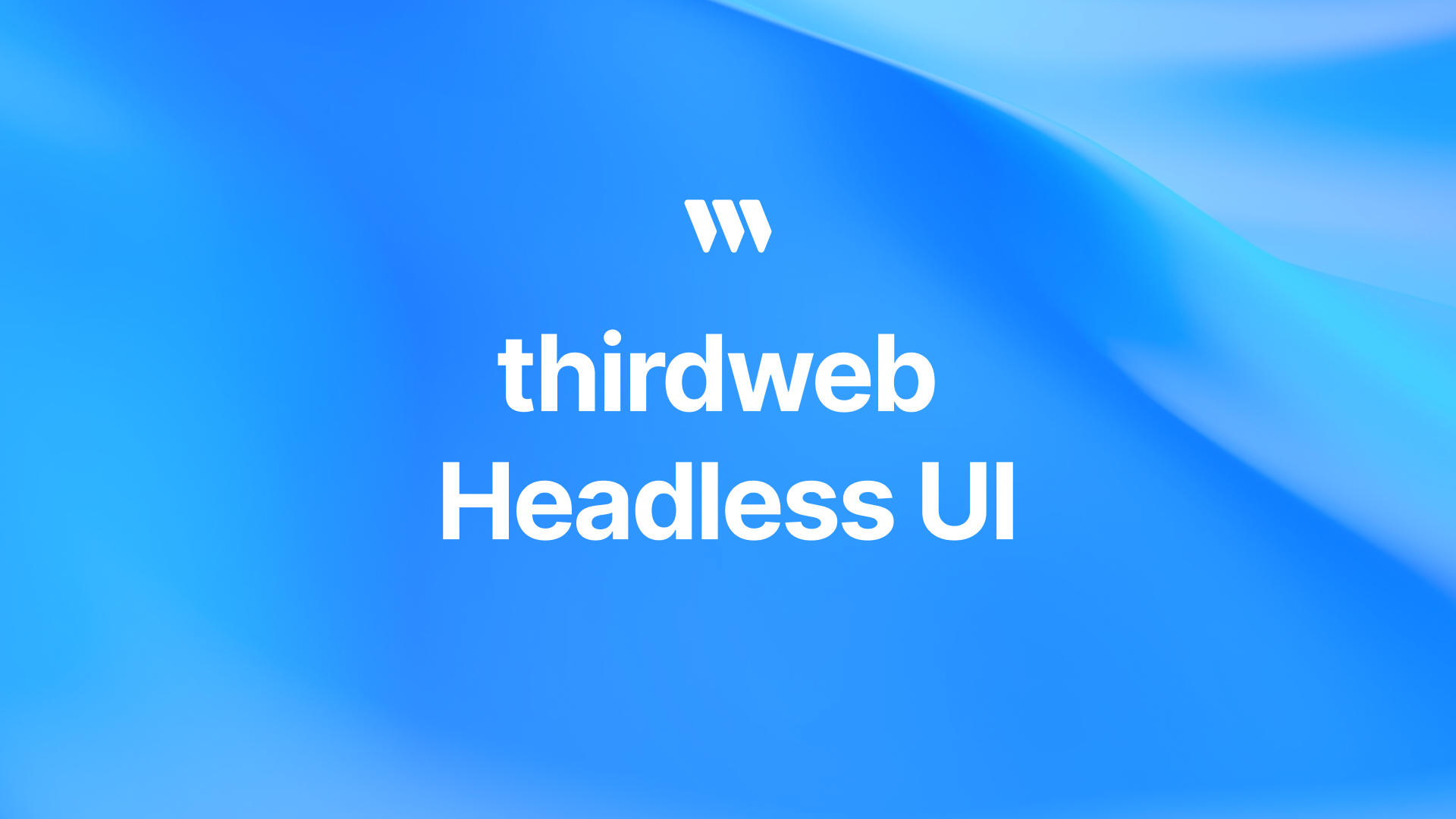
A few months ago we released an update for the NFT components. Today, we are excited to announce the most recent updates to our Headless UI component series: Account, Token and NFT - enabling you to build web3 apps faster & easier than ever.
Account components
Account components are used to display the info of a given wallet address. You can display the social avatar, name & the wallet balance for a specific token with just a few lines of code. Here's a demo to show how easy it is to replicate our ConnectButton
in the connected state.
const BasicConnectedButton = () => {
const roundUpBalance = (num: number) => Math.round(num * 10) / 10;
return (
<AccountProvider address="" client={thirdwebClient}>
<Button className="flex h-12 w-40 flex-row justify-start gap-3 px-2">
<AccountAvatar className="h-10 w-10 rounded-full" />
<div className="flex flex-col items-start">
<AccountName />
<AccountBalance
chain={sepolia}
formatFn={roundUpBalance}
/>
</div>
</Button>
</AccountProvider>
);
};
Result:
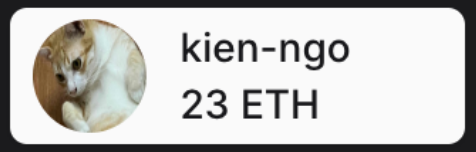
In reality, you would have to deal with loading state, fallback state (if something fails to resolve) & different theming options. Our headless components let you control all of those logic. Head over to our docs to learn more!
Token components
Token components are used to display the info about a native token, or an ERC20 token on a specific chain. The components included in this release are: TokenIcon
, TokenName
, TokenSymbol
. Similar to Account, they should be wrapped inside a TokenProvider
.
Basic usage:
import { TokenProvider, TokenName, TokenSymbol, TokenIcon } from "thirdweb/react";
function () {
return <TokenProvider
address={NATIVE_TOKEN_ADDRESS}
client={thirdwebClient}
chain={ethereum}
>
<TokenName /> // "Ether"
<TokenSymbol /> // "ETH"
<TokenIcon />
</TokenProvider>
}
Head over to our docs to learn more about the Token components.
NFT components
This update introduces the breaking changes to the original NFT components. The reason for these changes are mainly for better performance and more fine-grained control over the inner logic of said components.
Old syntax:
import { NFT } from "thirdweb/react";
function App() {
return <NFT contract={nftContract} tokenId={0n}>
{/* Show the info for tokenId #0 from the `nftContract` */}
<Suspense fallback={"Loading info..."}>
<NFT.Media />
<NFT.Name />
<NFT.Description />
</Suspense>
</NFT>
}
New syntax:
import { NFTProvider, NFTMedia, NFTName, NFTDescription } from "thirdweb/react";
function App() {
return <NFTProvider contract={nftContract} tokenId={0n}>
<NFTMedia />
<NFTName />
<NFTDescription />
</NFTProvider>
}
As you can see, the new syntax is cleaner and doesn't require React.Suspense to work. The code is also better tree-shaken since we no longer wrap everything inside the NFT object.
Learn more about this component here.