Insight: Token Lookup And Collection Metadata
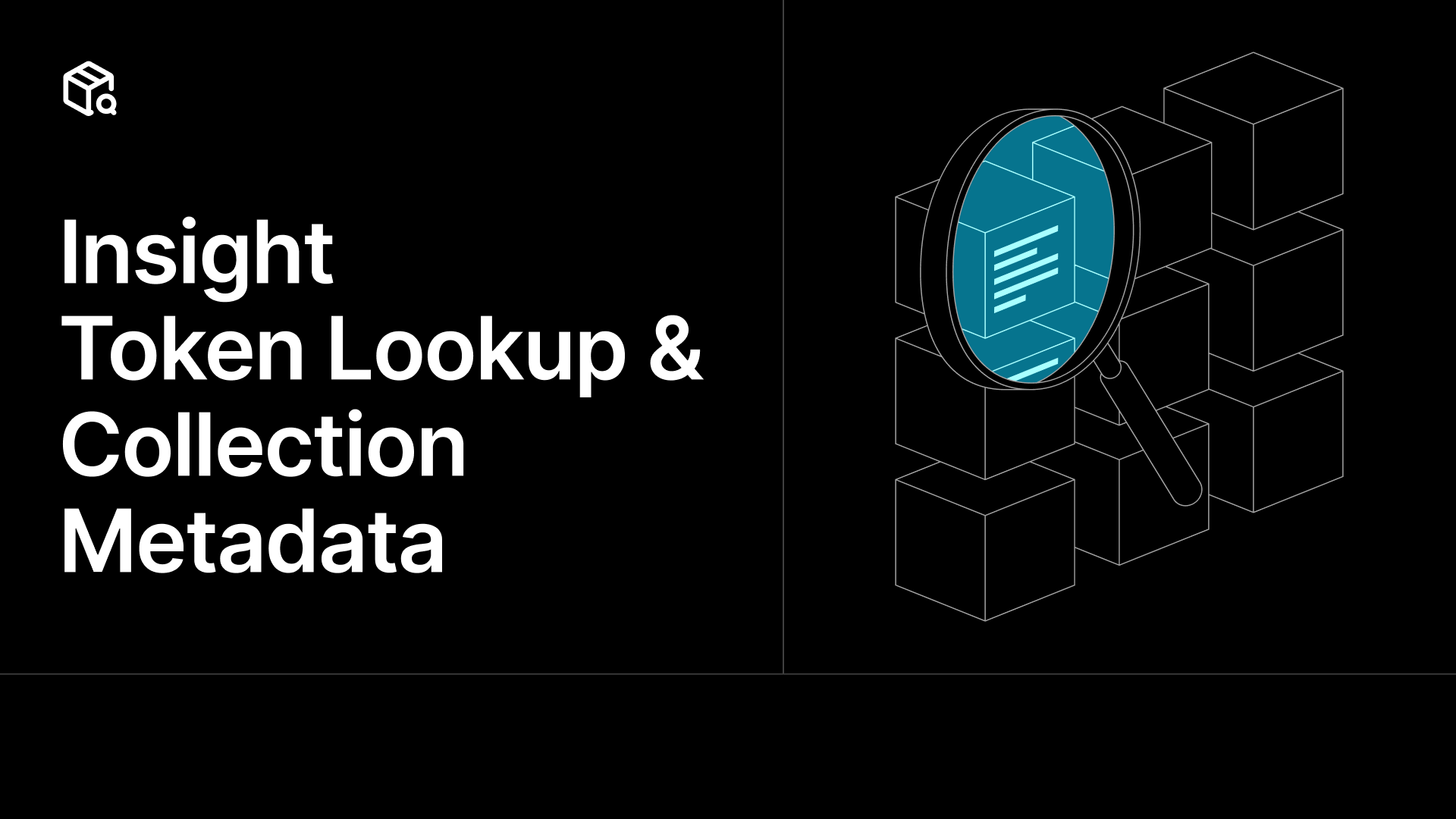
Insight adds two new endpoints - one to lookup a token based on symbols and one to retrieve an NFT collection's metadata.
Token Lookup
Search for tokens by their symbol across 6000+ tokens.
Symbols are not unique nor strictly curated however, so be careful with how you use them!
To fetch all tokens with symbol ETH
on Ethereum and B3
const lookupTokens = async (symbol: string) => {
try {
const response = await fetch(
`https://insight.thirdweb.com/v1/tokens/lookup?symbol=${symbol}&chain=1`,
{
headers: {
"x-client-id": <THIRDWEB_CLIENT_ID>,
},
},
);
const res = await response.json();
return res.data;
} catch (error) {
console.error("Error:", error);
}
};
await lookupTokens("ETH")
Try it out in our playground
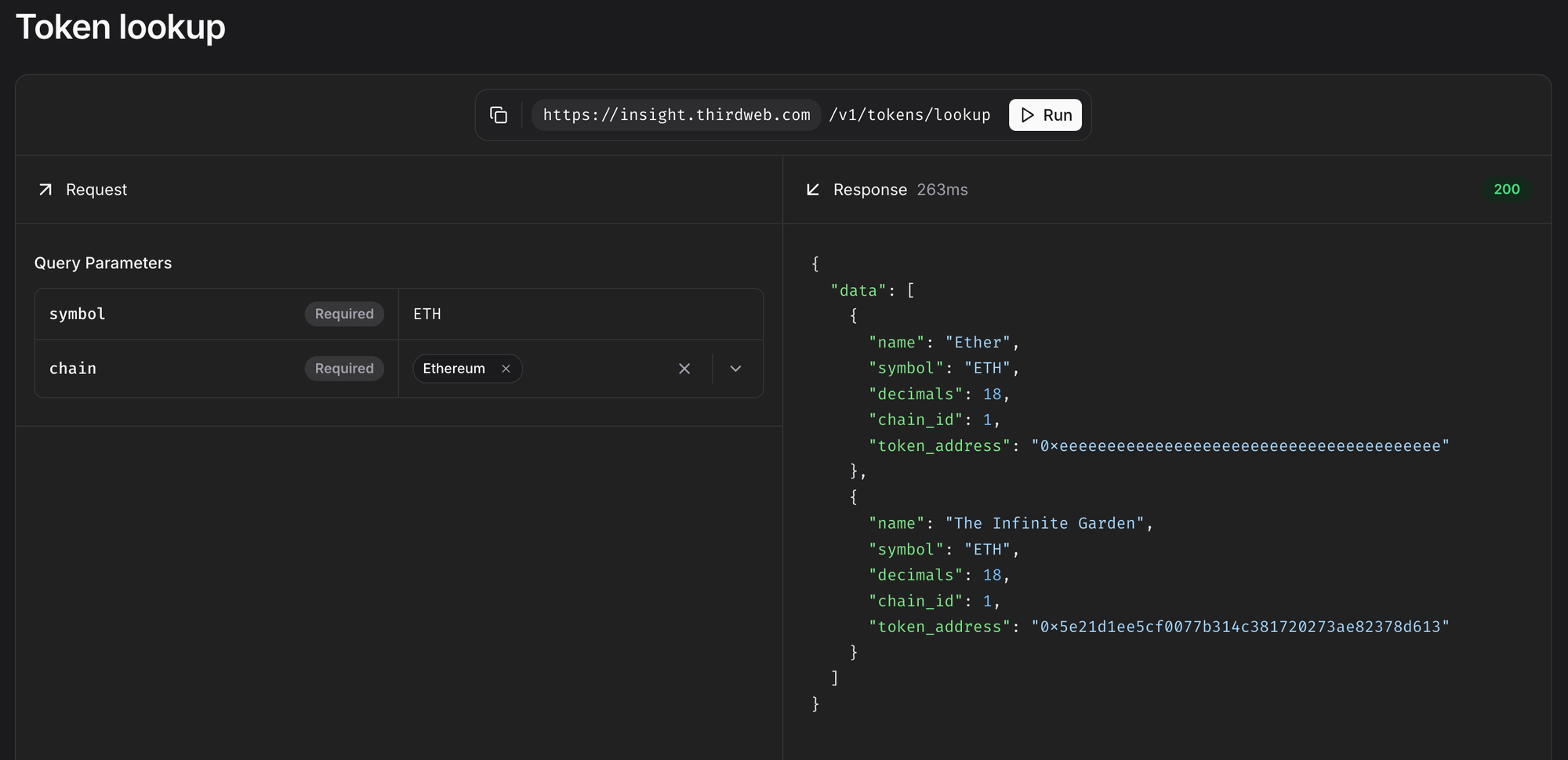
Collection Metadata
Get metadata about an NFT collection.
By including a include_stats=true
query param, you can also get the following statistics about a collection:
owner_count
- Amount of distinct addresses that hold the NFTsmint_count
- Amount of NFT tokens that have been mintedtoken_count
- Amount of distinct token IDs that currently existtotal_quantity
- Amount of tokens that currently exist
const getCollectionMetadata = async (contractAddress: string) => {
try {
const response = await fetch(
`https://1.insight.thirdweb.com/v1/nfts/collection/${contractAddress}?include_stats=true`,
{
headers: {
"x-client-id": <THIRDWEB_CLIENT_ID>,
},
},
);
const res = await response.json();
return res.data;
} catch (error) {
console.error("Error:", error);
}
};
Try it out in our playground
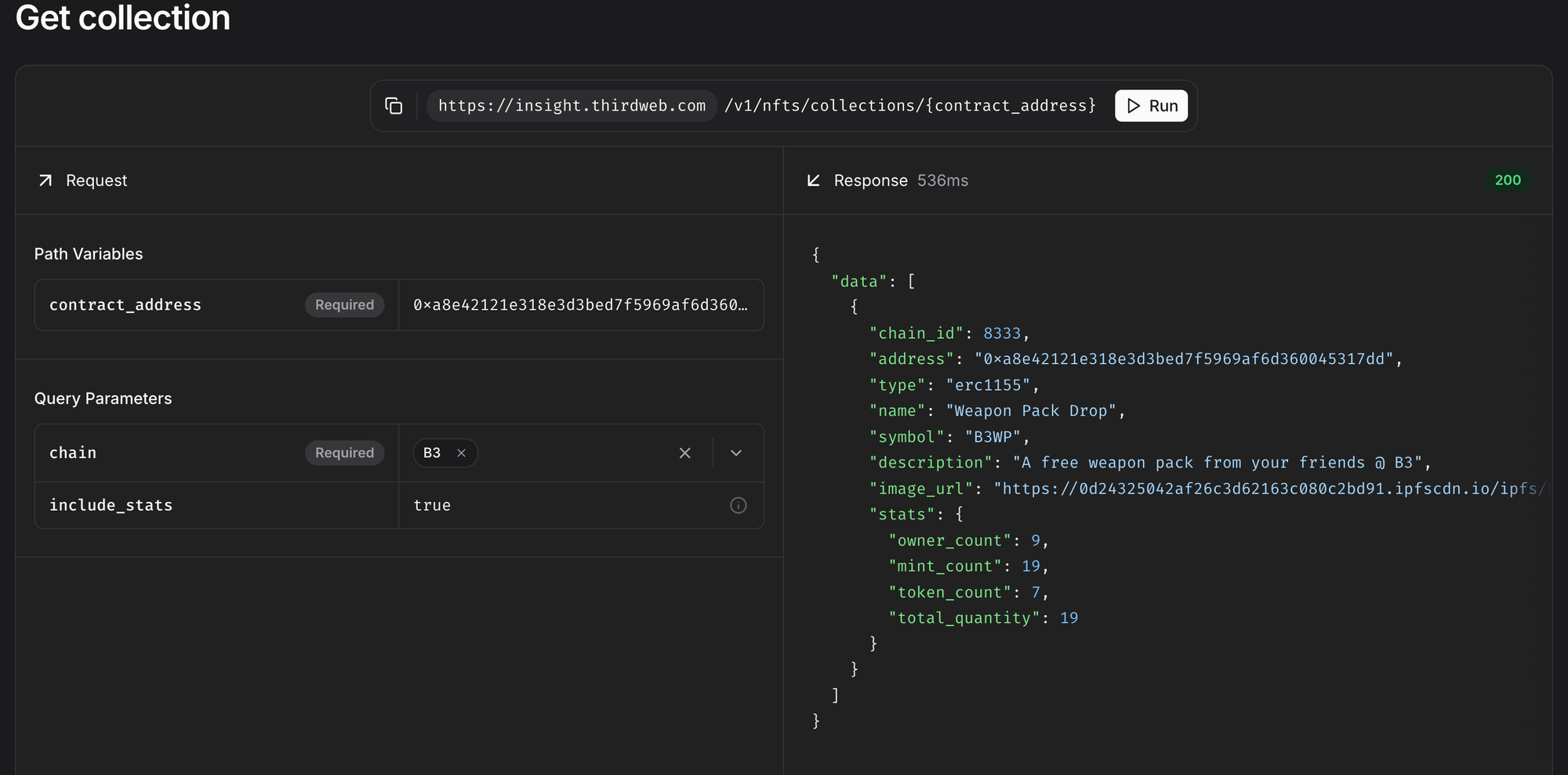
π Learn more about Insight - itβs open source and ready to power your applications!