Insight Adds Token Prices
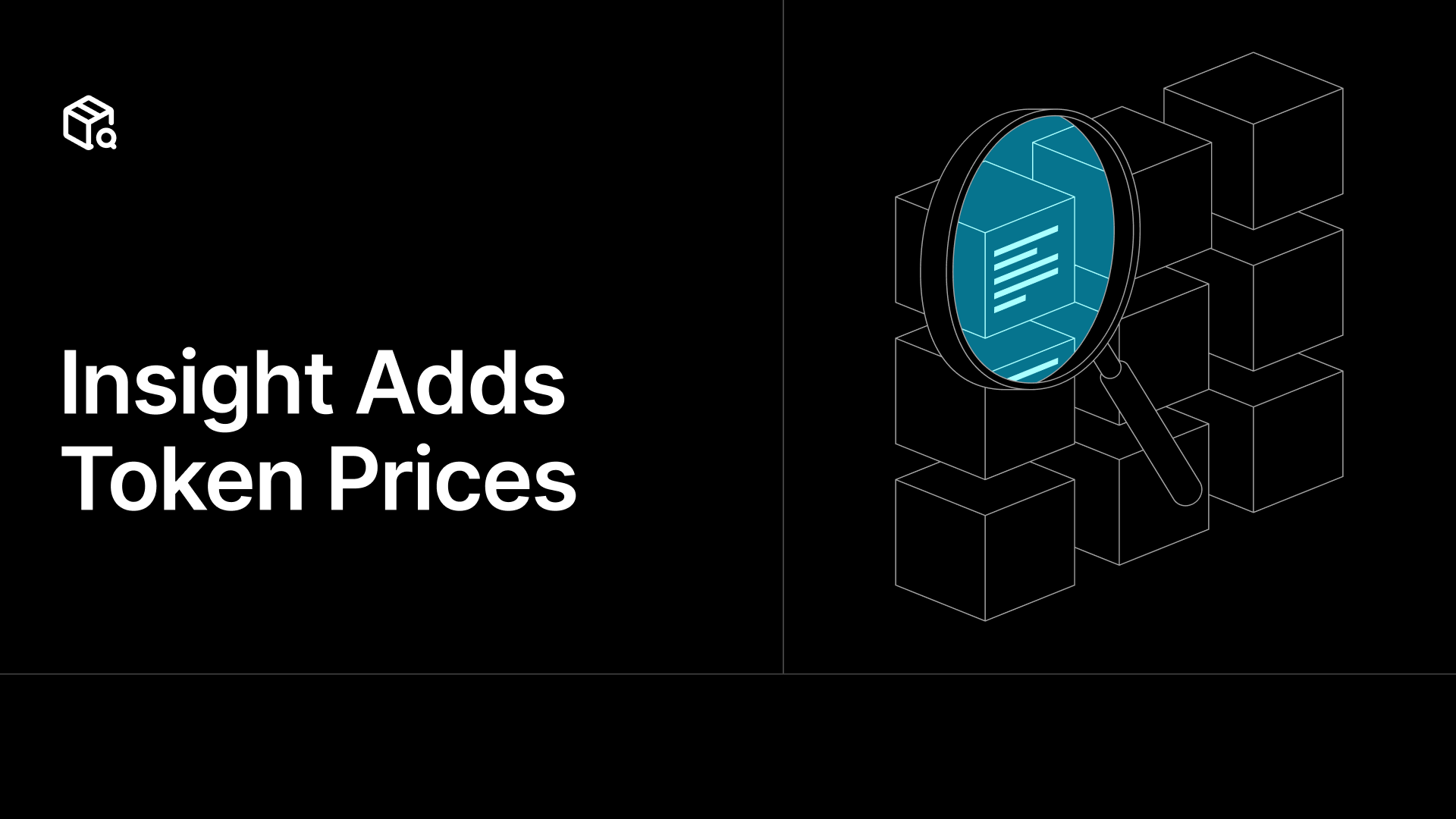
Querying token prices in USD is now supported by Insight API!
Try it out in our playground
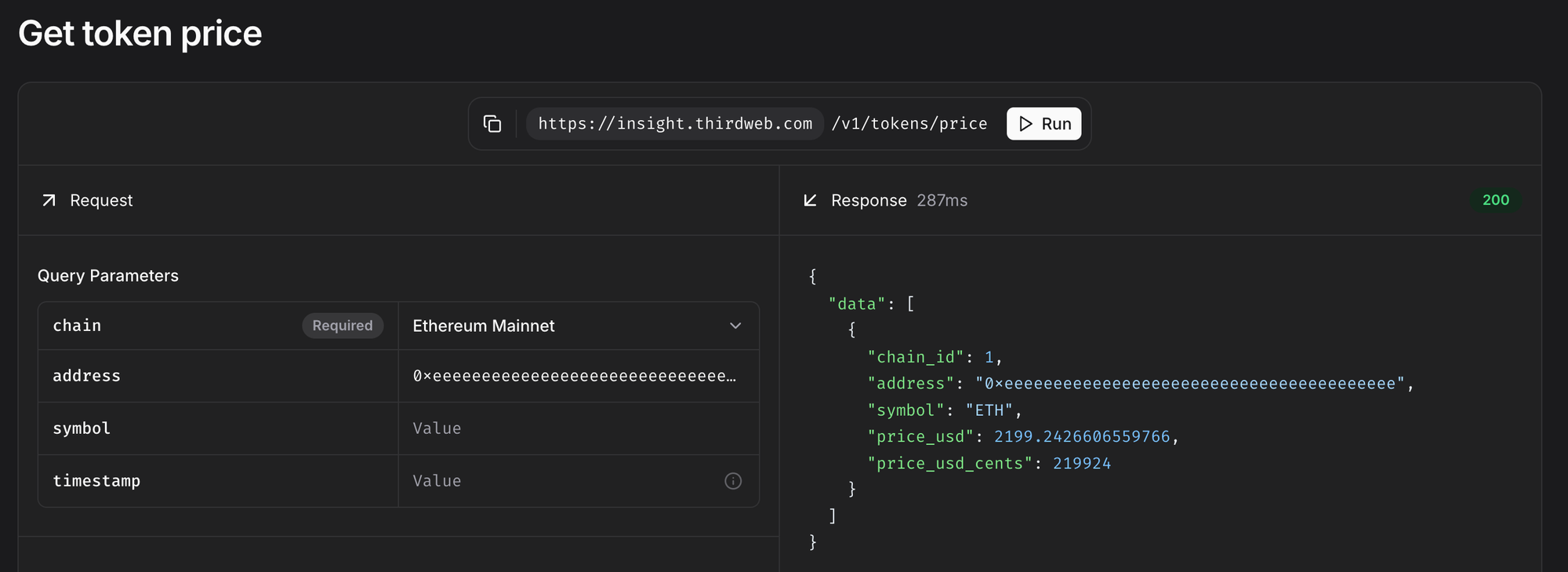
To fetch a token's price by its contract address
(0xeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeee
is used for native tokens)
const getPrice = async () => {
try {
const response = await fetch(
"https://1.insight.thirdweb.com/v1/tokens/price?address=0xeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeee",
{
headers: {
"x-client-id": <THIRDWEB_CLIENT_ID>,
},
},
);
const priceData = await response.json();
return priceData.data;
} catch (error) {
console.error("Error:", error);
}
};
Response
[
{
chain_id: 1,
address: '0xeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeee',
symbol: 'ETH',
price_usd: 2192.917084798869,
price_usd_cents: 219291
}
]
You can optionally define a timestamp and the API will return the closest data it has to that timestamp.
const getPrice = async () => {
try {
const ts = Date.now() - 3 * 60 * 60 * 1000; // 3 hours ago
const response = await fetch(
`https://1.insight.thirdweb.com/v1/tokens/price?address=0xeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeee×tamp=${ts}`,
{
headers: {
"x-client-id": <THIRDWEB_CLIENT_ID>,
},
},
);
const priceData = await response.json();
return priceData.data;
} catch (error) {
console.error("Error:", error);
}
};
To fetch token prices by symbol
const getPriceBySymbol = async () => {
try {
const response = await fetch(
"https://1.insight.thirdweb.com/v1/tokens/price?symbol=ETH",
{
headers: {
"x-client-id": <THIRDWEB_CLIENT_ID>,
},
},
);
const priceData = await response.json();
return priceData.data;
} catch (error) {
console.error("Error:", error);
}
};
Note that there can be multiple tokens with the same symbol
We support more than 6000 tokens currently and keep adding more.
We also provide an endpoint to fetch the list of supported tokens for each chain.
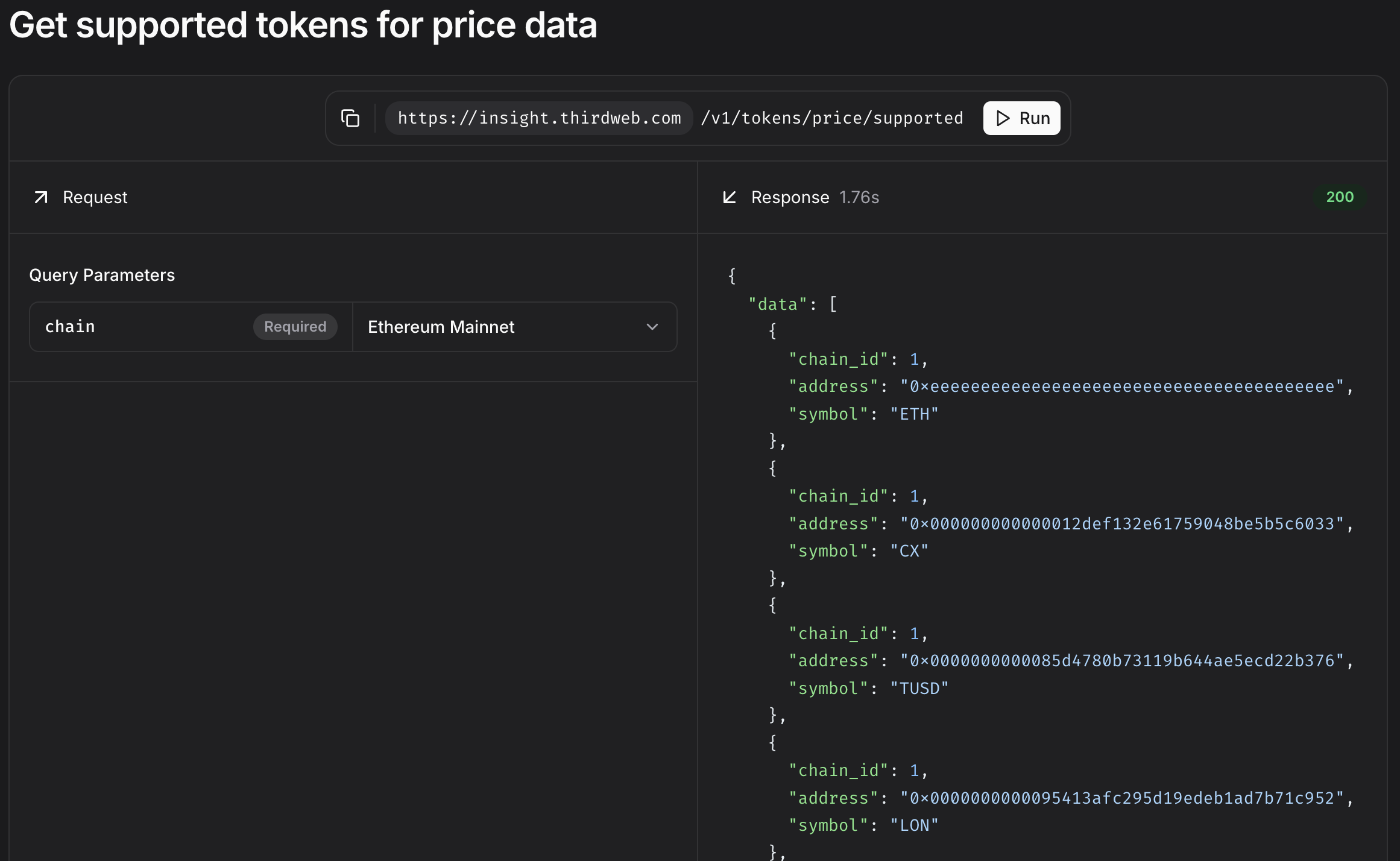
π Learn more about Insight - itβs open source and ready to power your applications!