Improved EIP-5792 support
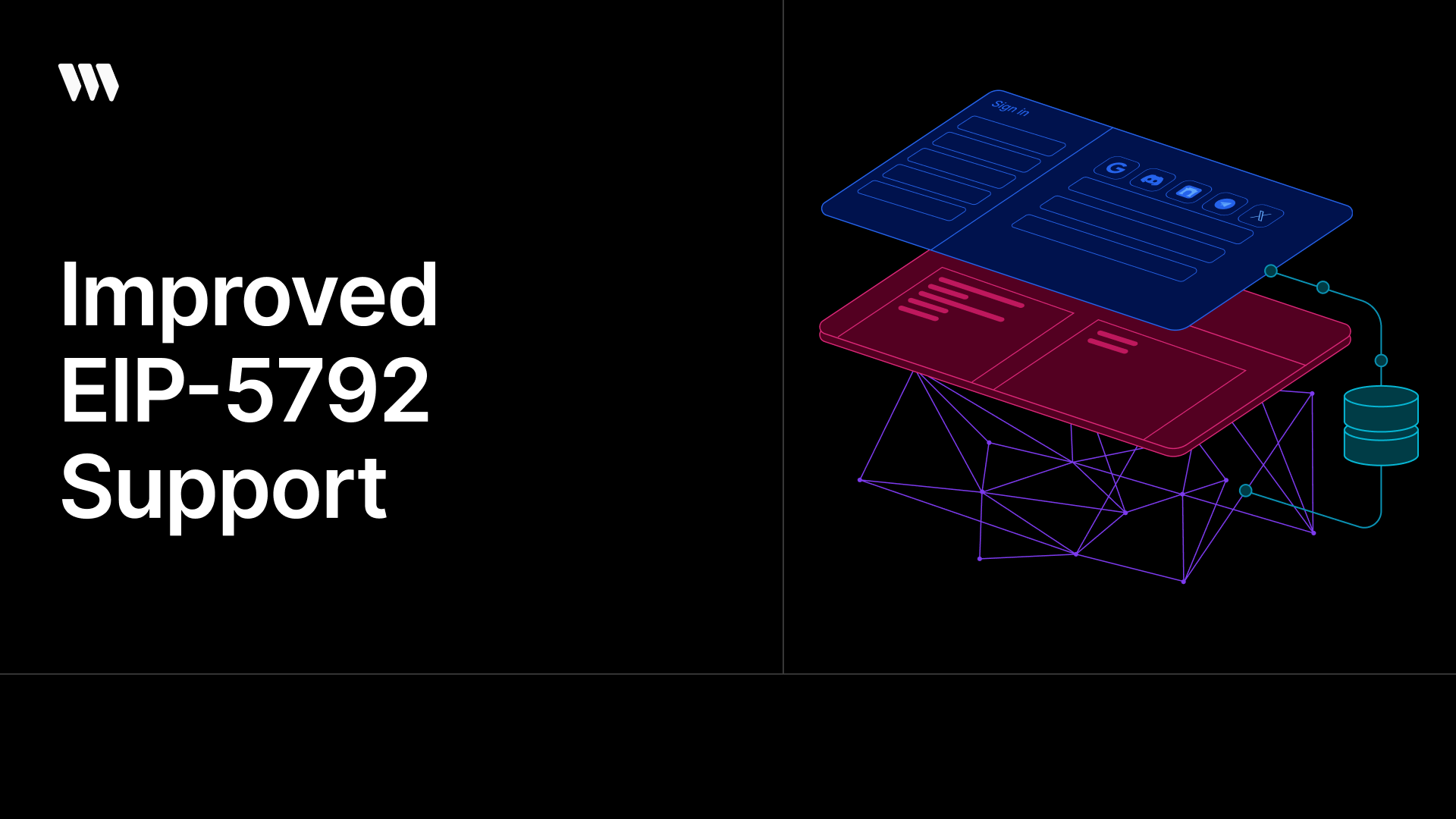
Version 5.99.0 of the TypeScript thirdweb SDK brings first-class support for EIP-5792. The new implementation streamlines how you batch calls, sponsor gas and track confirmations, while aligning our API with the final EIP-5792 spec.
Highlights:
- New React hooks – useSendAndConfirmCalls, useWaitForCallsReceipt
- Simpler useSendCalls / sendCalls signatures – no more explicit client prop, aligned return types with v2.0.0 of the EIP
- Decoupled receipt handling for flexible UI handling of receipts
- Easy wallet-capability detection
Live Demo
As usual, you can try out the integration on the live playground:
Usage
TypeScript (node / backend)
import { sendCalls, waitForCallsReceipt } from "thirdweb";
// Build your calls array (tx1, tx2 … conforming to EIP-5792 structure)
const result = await sendCalls({
calls: [tx1, tx2],
sponsorGas: true, // optional gas sponsorship
});
// Later - or in a different process
const receipt = await waitForCallsReceipt(result.id);
console.log("First tx hash:", receipt.receipts[0].transactionHash);
React (front-end)
1 – Fire-and-forget, then wait for the receipt
const { mutate: sendCalls } = useSendCalls();
const { data: result } = await sendCalls({ calls: [tx1, tx2] });
// later, or in another component
const { data: receipt, isLoading } = useWaitForCallsReceipt(result.id);
2 – One-liner helper
const { mutate: sendAndConfirmCalls, data: receipt } = useSendAndConfirmCalls();
await sendAndConfirmCalls({ calls: [tx1, tx2] });
console.log("Bundle confirmed:", receipt.bundleHash);
Breaking changes
The previous version of the SDK had beta support for 5792, which got some breaking changes in this version, listed below:
React
Before |
After |
---|---|
useSendCalls({ client }) returned string bundleId |
useSendCalls() returns { id, client, chain, wallet } |
useSendCalls({ waitForBundle: true }) returned receipts |
Waiting is now decoupled; use useWaitForCallsReceipt |
N/A |
New helper useSendAndConfirmCalls combines both steps |
TypeScript / non-React
Before |
After |
---|---|
type SendCallsResult = string; |
type SendCallsResult = { id: string; client: ThirdwebClient; chain: Chain; wallet: Wallet } |
getCallsStatus(bundleId) |
getCallsStatus(id) (field renamed) |
Any existing code that relies on the old return type must now read result.id and call waitForCallsReceipt (or the React hook) to obtain transaction receipts.
We recommend upgrading as soon as possible to leverage gas sponsorship and the simplified developer experience. For a full diff, see PR #7003 on GitHub.