Bridge Any Asset with the TypeScript SDK
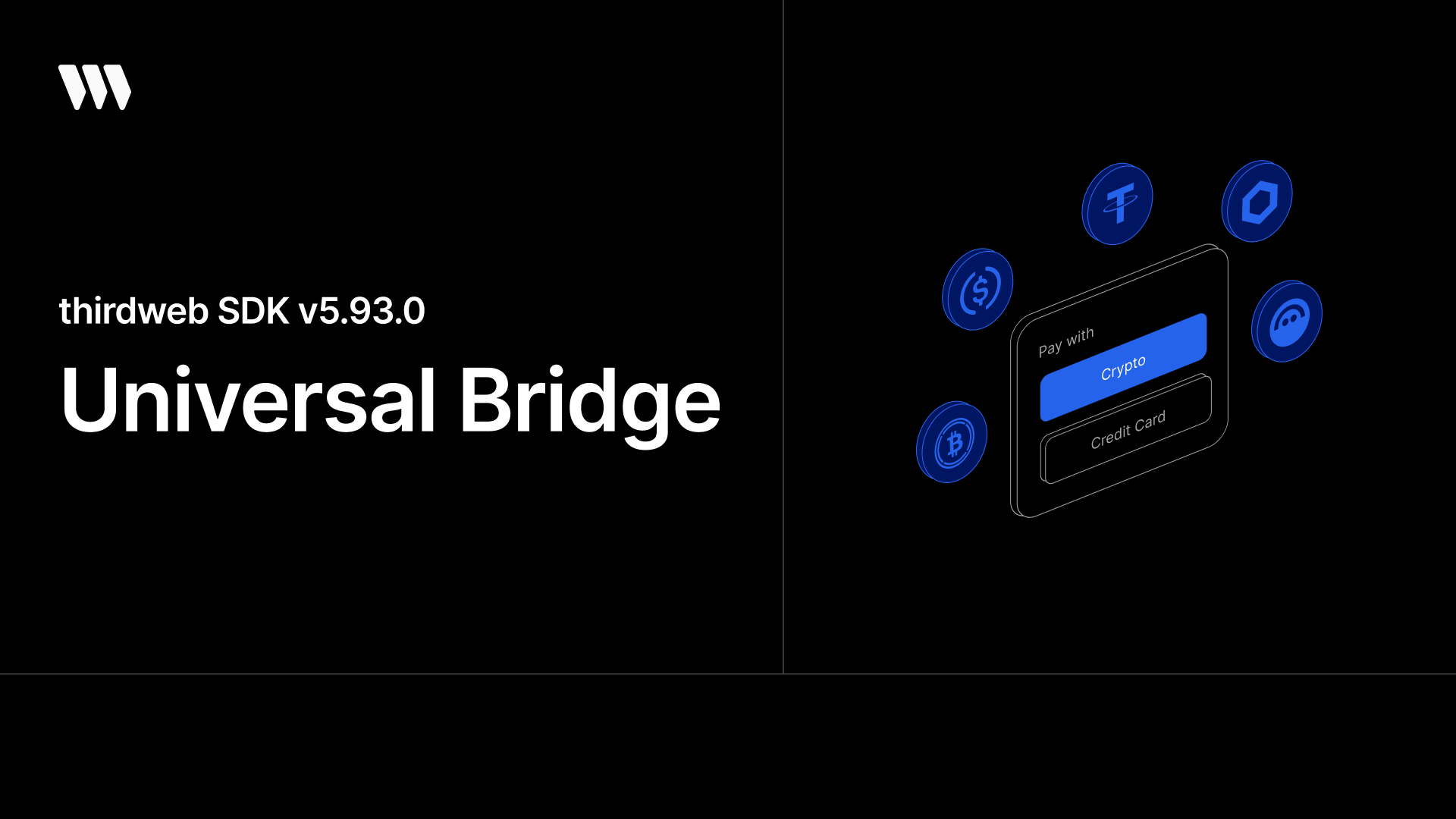
With v5.93.0 of the TypeScript SDK, we've added full beta functionality for thirdweb's new Universal Bridge. The bridge currently covers 50+ chains and over 30,000 routes.
- Bridge.Buy - Specify an exact destination amount to receive
quote
: Get estimates without a wallet connectionprepare
: Get finalized quotes with transaction data
- Bridge.Sell - Specify the exact origin amount to send
quote
: Get estimates without a wallet connectionprepare
: Get finalized quotes with transaction data
How to Send Transactions
When you call prepare
, you might get multiple transactions back. You must send all transactions in order and sequentially for the full route to succeed. Before sending each transaction, call Bridge.status
for the previous transaction until it returns COMPLETED
. Do not simply wait for the transaction receipt, as this doesn't account for the destination chain transaction (if there is one).
The transactions returned do not include approvals. Send any necessary approvals before their corresponding transactions.
Route Discovery & Transaction Tracking
- Bridge.routes - Advanced function to discover and filter available bridge routes
- Filter by token address, chain ID, or both
- Full pagination support with customizable limit and offset
- Bridge.status - Comprehensive transaction status tracking
- Clear status indicators: "COMPLETED", "PENDING", "FAILED", or "NOT_FOUND"
- Detailed transaction reporting including origin and destination amounts and chains
Types
- Standardized error handling with descriptive, formatted error messages
- Four underlying types are exported for use with the bridging functions:
Route
: Defines bridge routes between chains and tokensStatus
: Represents bridge transaction status dataQuote
: Contains detailed bridge transaction quote informationPreparedQuote
: Extends Quote with complete transaction data
Implementation Examples
Bridge.Buy Usage Example
import { Bridge, toWei, NATIVE_TOKEN_ADDRESS } from "thirdweb";
// First, get a quote to see approximately how much you'll pay
const buyQuote = await Bridge.Buy.quote({
originChainId: 1, // Ethereum
originTokenAddress: NATIVE_TOKEN_ADDRESS,
destinationChainId: 10, // Optimism
destinationTokenAddress: NATIVE_TOKEN_ADDRESS,
buyAmountWei: toWei("0.01"), // I want to receive 0.01 ETH on Optimism
client: thirdwebClient,
});
console.log(
`To get ${buyQuote.destinationAmount} wei on destination chain, you need to pay ${buyQuote.originAmount} wei`,
);
// When ready to execute, prepare the transaction
const preparedBuy = await Bridge.Buy.prepare({
originChainId: 1,
originTokenAddress: NATIVE_TOKEN_ADDRESS,
destinationChainId: 10,
destinationTokenAddress: NATIVE_TOKEN_ADDRESS,
buyAmountWei: toWei("0.01"),
sender: "0x...", // Your wallet address
receiver: "0x...", // Recipient address (can be the same as sender)
client: thirdwebClient,
});
// The prepared quote contains the transactions you need to execute
console.log(`Transactions to execute: ${preparedBuy.transactions.length}`);
Bridge.Sell Usage Example
import { Bridge, toWei } from "thirdweb";
// First, get a quote to see approximately how much you'll receive
const sellQuote = await Bridge.Sell.quote({
originChainId: 1, // Ethereum
originTokenAddress: NATIVE_TOKEN_ADDRESS,
destinationChainId: 10, // Optimism
destinationTokenAddress: NATIVE_TOKEN_ADDRESS,
sellAmountWei: toWei("0.01"), // I want to sell 0.01 ETH from Ethereum
client: thirdwebClient,
});
console.log(
`If you send ${sellQuote.originAmount} wei, you'll receive approximately ${sellQuote.destinationAmount} wei`,
);
// When ready to execute, prepare the transaction
const preparedSell = await Bridge.Sell.prepare({
originChainId: 1,
originTokenAddress: NATIVE_TOKEN_ADDRESS,
destinationChainId: 10,
destinationTokenAddress: NATIVE_TOKEN_ADDRESS,
sellAmountWei: toWei("0.01"),
sender: "0x...", // Your wallet address
receiver: "0x...", // Recipient address (can be the same as sender)
client: thirdwebClient,
});
// Execute the transactions in sequence
for (const tx of preparedSell.transactions) {
// Send the transaction using your wallet
// Wait for it to be mined
}
Route Discovery Example
import { Bridge, NATIVE_TOKEN_ADDRESS } from "thirdweb";
// Get all available routes
const allRoutes = await Bridge.routes({
client: thirdwebClient,
});
// Filter routes for a specific token or chain
const filteredRoutes = await Bridge.routes({
originChainId: 1, // From Ethereum
originTokenAddress: NATIVE_TOKEN_ADDRESS,
destinationChainId: 10, // To Optimism
client: thirdwebClient,
});
// Paginate through routes
const paginatedRoutes = await Bridge.routes({
limit: 10,
offset: 0,
client: thirdwebClient,
});
Transaction Status Monitoring Example
import { Bridge } from "thirdweb";
// Check the status of a bridge transaction
const bridgeStatus = await Bridge.status({
transactionHash:
"0xe199ef82a0b6215221536e18ec512813c1aa10b4f5ed0d4dfdfcd703578da56d",
chainId: 8453, // The chain ID where the transaction was initiated
client: thirdwebClient,
});
// The status will be one of: "COMPLETED", "PENDING", "FAILED", or "NOT_FOUND"
if (bridgeStatus.status === "completed") {
console.log(`
Bridge completed!
Sent: ${bridgeStatus.originAmount} wei on chain ${bridgeStatus.originChainId}
Received: ${bridgeStatus.destinationAmount} wei on chain ${bridgeStatus.destinationChainId}
`);
} else if (bridgeStatus.status === "pending") {
console.log("Bridge transaction is still pending...");
} else {
console.log("Bridge transaction failed");
}
Error Handling Implementation
try {
await Bridge.Buy.quote({
// ...params
});
} catch (error) {
// Errors will have the format: "ErrorCode | Error message details"
console.error(error.message); // e.g. "AmountTooHigh | The provided amount is too high for the requested route."
}
Module Integration
The Bridge module is accessible as a top-level export:
import { Bridge } from "thirdweb";
Use Bridge.Buy
, Bridge.Sell
, Bridge.routes
, and Bridge.status
to access the corresponding functionality.
Or, import the functions directly from the module:
import { Buy, Sell, routes, status } from "thirdweb/bridge";