Auth (SIWE) from React Native
First class support for Sign in with Ethereum (SIWE) in React Native
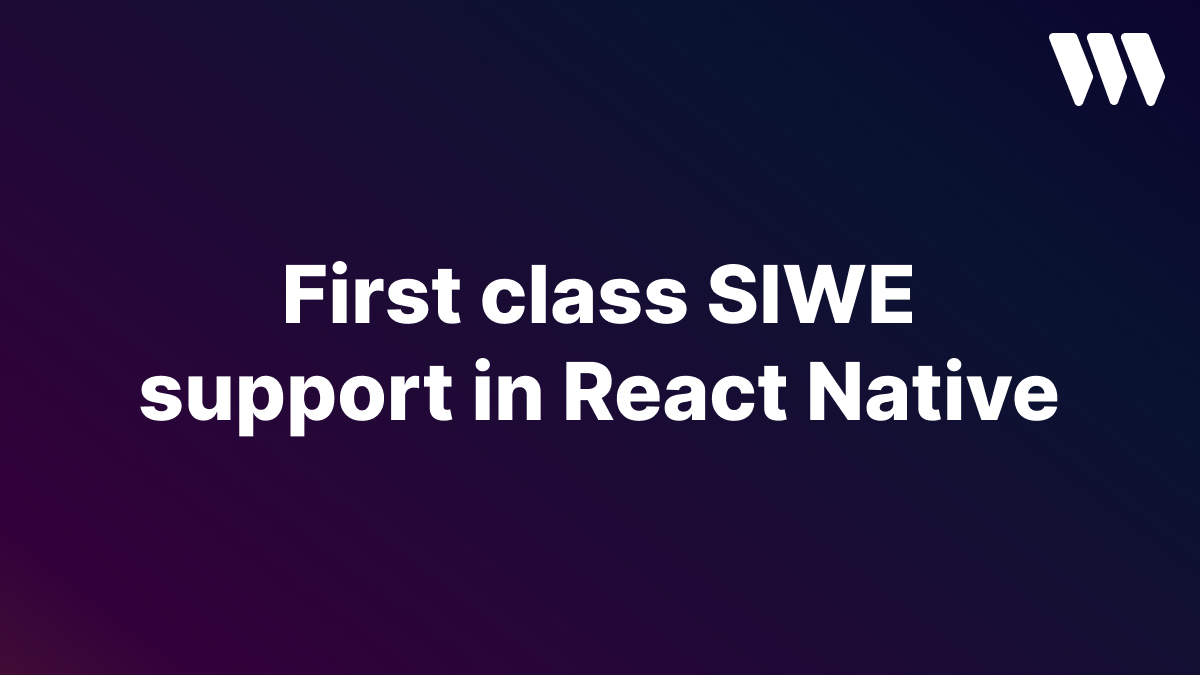
A common use case for onchain apps is to securely store the user's wallet address on a backend. To do so we recommend using Auth, which streamlines setting up the Sign in with Ethereum (SIWE) protocol between your app and your backend.
In v5.35 of the connect SDK, we added first class support for Auth in react native.
You can now setup the ConnectButton
and ConnectEmbed
to automatically show a sign in button to authenticate with your backend using SIWE.
// this auth helper should live server side
const thirdwebAuth = createAuth({
domain: "localhost:3000",
client,
});
<ConnectButton
client={client}
auth={{
getLoginPayload: async (params) => {
// here you should call your backend, using generatePayload to return
// a SIWE compliant login payload to the client
return thirdwebAuth.generatePayload(params);
},
doLogin: async (params) => {
// here you should call your backend to verify the signed payload passed in params
// this will verify that the signature matches the intended wallet
const verifiedPayload =
await thirdwebAuth.verifyPayload(params);
setLoggedIn(verifiedPayload.valid);
},
isLoggedIn: async () => {
// here you should ask you backend if the user is logged in
// can use cookies, storage, or your method of choice
return loggedIn;
},
doLogout: async () => {
// here you should call your backend to logout the user if needed
// and delete any local auth tokens
setLoggedIn(false);
},
}}
/>
And of course you can also use the Auth utility methods to build your own UI flow.
Learn more about setting up Auth in the documentation.
Happy Building! 🛠️