Account Abstraction Improvements
thirdweb SDK v5.81.0
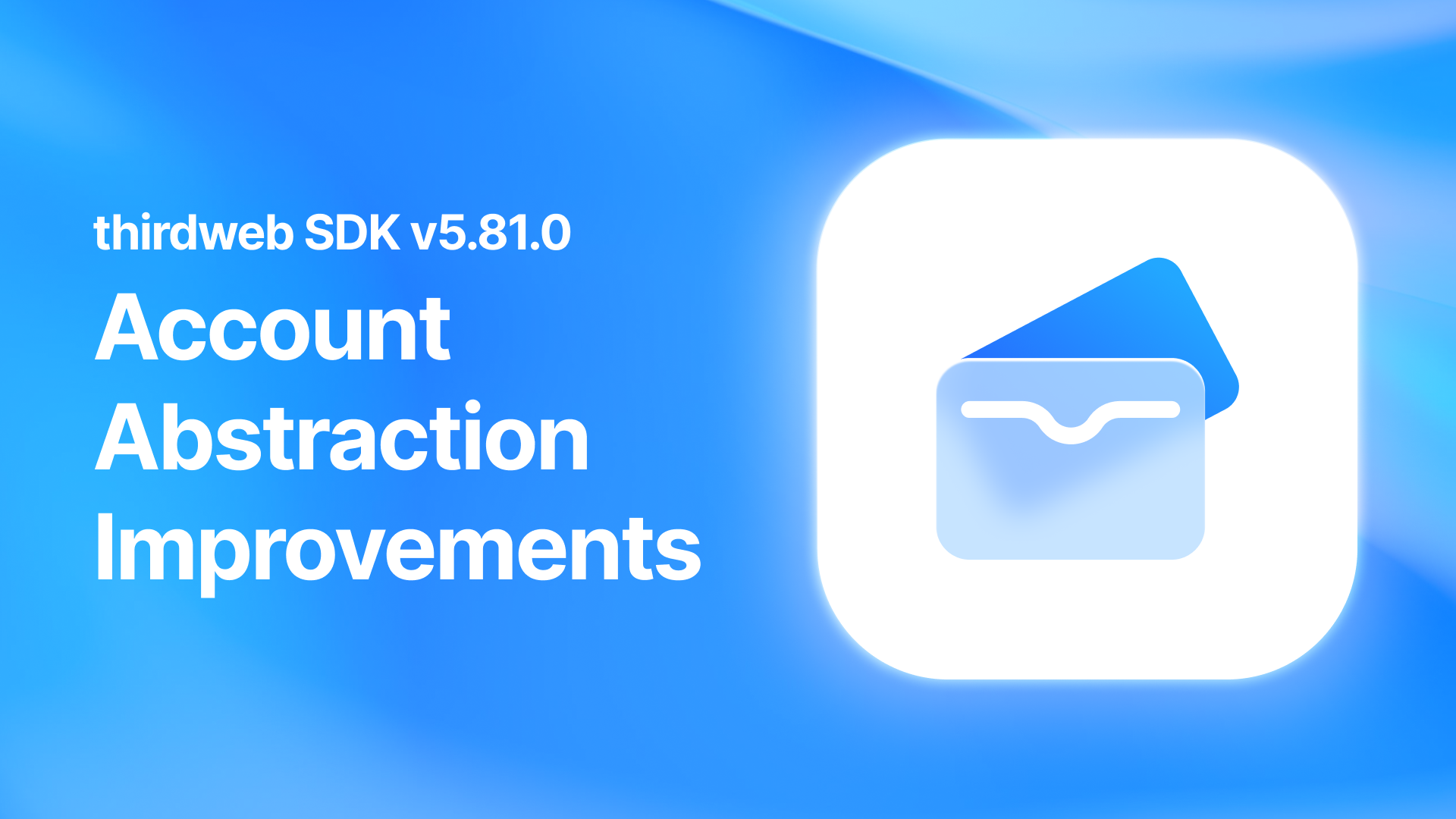
Features 🚀
Retrieve In-App Wallet Smart Wallet Admin Account
We've added the getAdminAccount
method to inAppWallet interfaces when using Account Abstraction (AA), including gasless ecosystem wallets. This addition allows developers to retrieve the admin account for AA-compatible in-app wallets.
// Initialize the in-app wallet with sponsored gas enabled
const wallet = inAppWallet({
smartAccount: {
chain: sepolia,
sponsorGas: true,
},
});
// Connect to the wallet using a specific client and strategy
const account = await wallet.connect({
client, // Your defined client instance
strategy: "google", // Specify your authentication strategy
});
// Retrieve the admin account
const adminAccount = await wallet.getAdminAccount();
// Log the admin account address
console.log("Admin Account Address:", adminAccount.address);
Improvements 🔧
Enhanced Modal Control
We've added a new onClose
callback option to Connect Details modal, providing developers with greater control over modal behavior and user interactions. The callback receives the last active screen name when the user closes the modal, enabling better UX flows and analytics.
<ConnectButton
detailsModal={{
onClose: (screen: string) => {
console.log(`Modal closed while on screen: ${screen}`);
// Handle post-close logic here
},
}}
/>
Auto-Connect onTimeout Callback
We've also added an onTimeout
callback in useAutoConnect hook to handle connection timeout scenarios gracefully. Timeouts can happen if the user has previously connected a wallet, but we're unable to reconnect to that wallet.
<AutoConnect
onTimeout={() => {
console.log("Auto connection timed out")
}}
/>
Bug Fixes 🐛
Smart Account Transactions
We fixed an issue where smart accounts could not execute transactions on chains besides the one they were initially created on. AA transactions on chains besides the initial one now deploy the smart account on the new chain before executing the transaction
// Configure the smart wallet
const wallet = smartWallet({
chain: sepolia,
sponsorGas: true,
});
// Connect the smart wallet
const smartAccount = await wallet.connect({
client,
personalAccount,
});
// Prepare a transaction on another chain
const transaction = prepareTransaction({
value: 10000n,
to: "0x..."
chain: baseSepolia,
client
});
// This will deploy the smart account on Base Sepolia
const result = await sendTransaction({
account: smartAccount,
transaction
});
Removals and Deprecations 🗑️
Wallet Support Changes
We've removed co.lobstr from the available wallets list as it is not EVM-compatible