Building an AI-Powered Blockchain Explorer with Nebula
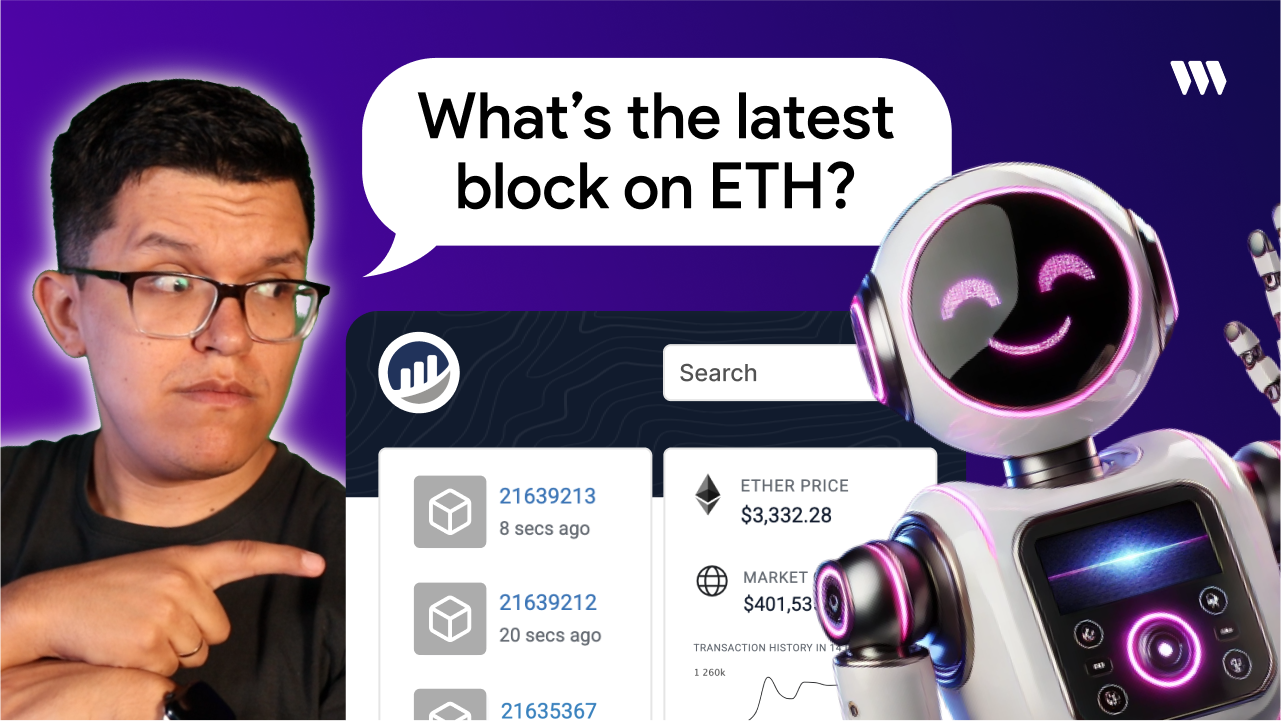
Is blockchain data confusing you? Transactions and smart contract interactions can be difficult to understand at first glance.
In this tutorial, we'll build a fully functional blockchain explorer powered by AI that allows us to get information from any EVM-compatible blockchain through a simple chat interface.
AI agents are changing how we interact with technology, and blockchain is no exception. Traditional blockchain explorers display raw data that can be challenging to interpret, especially for newcomers. Our AI-powered explorer will let users ask questions in plain English about contracts, transactions, and addresses - making blockchain data accessible to everyone.
Follow along with the vide tutorial:
What You'll Build
We'll create a web application that allows users to:
- Enter any smart contract address and select a blockchain network
- Get a detailed analysis of the contract through natural language interactions
- Ask questions about the contract's functions and state
- Even execute transactions directly through the chat interface
Project Overview
The project consists of three main parts:
- Backend Script: Connecting to Nebula (an AI agent for blockchain data)
- User Interface: Creating a chat-based explorer experience
- Transaction Execution: Adding the ability to trigger wallet transactions via chat
Let's break this down step by step.
Step 1: Connecting to Nebula API
First, we need to set up the script that will interact with Nebula's API.
The Nebula API requires four key components:
- A base URL for endpoints
- Authentication via API key
- Session management
- Context filters to specify contract/chain scope
Let's create a script (scripts/Nebula.mjs) to handle these interactions:
const API_BASE_URL = "https://nebula-api.thirdweb.com";
const SECRET_KEY = ""; // Add your API key here
// Utility function to make API requests
async function apiRequest(endpoint, method, body = {}) {
const response = await fetch(`${API_BASE_URL}${endpoint}`, {
method,
headers: {
"Content-Type": "application/json",
"x-secret-key": SECRET_KEY,
},
body: Object.keys(body).length ? JSON.stringify(body) : undefined,
});
if (!response.ok) {
const errorText = await response.text();
console.error("API Response Error:", errorText);
throw new Error(`API Error: ${response.statusText}`);
}
return response.json();
}
// Create a new session
async function createSession(title = "Smart Contract Explorer") {
const response = await apiRequest("/session", "POST", { title });
const sessionId = response.result.id;
return sessionId;
}
// Query information about a contract
async function queryContract(contractAddress, chainId, sessionId) {
const message = `Give me the details of this contract and provide a structured list of all functions available...`;
const requestBody = {
message,
session_id: sessionId,
context_filter: {
chain_ids: [chainId.toString()],
contract_addresses: [contractAddress],
},
};
const response = await apiRequest("/chat", "POST", requestBody);
return response.message;
}
// Handle follow-up user messages
async function handleUserMessage(userMessage, sessionId, chainId, contractAddress) {
const response = await apiRequest("/chat", "POST", {
message: userMessage,
session_id: sessionId,
context_filter: {
chain_ids: [chainId.toString()],
contract_addresses: [contractAddress],
},
});
return response.message;
}
// Export functions for use in our app
export {
createSession,
queryContract,
handleUserMessage,
// Other functions will be added later
};
Security Tip: Never expose your API keys directly in your code, especially if pushing to public repositories. Use environment variables for production applications.
Step 2: Building the User Interface
Now let's create the frontend that will allow users to interact with our blockchain explorer. We'll use Next.js and Tailwind CSS for this project.
First, let's create the homepage where users can enter a contract address and select a chain:
// src/app/components/Hero.tsx
"use client";
import { useState } from "react";
import { useRouter } from "next/navigation";
import { Button } from "@/app/components/ui/button";
import { Input } from "@/app/components/ui/input";
import { Select, SelectContent, SelectItem, SelectTrigger, SelectValue } from "@/app/components/ui/select";
const blockchains = [
{ id: "1", name: "Ethereum Mainnet" },
{ id: "56", name: "BNB Smart Chain Mainnet" },
{ id: "11155111", name: "Ethereum Sepolia" },
// Add other chains as needed
];
export function Hero() {
const [searchTerm, setSearchTerm] = useState("");
const [selectedChain, setSelectedChain] = useState("");
const router = useRouter();
const handleSearch = () => {
router.push(`/explorer?chainId=${selectedChain}&searchTerm=${encodeURIComponent(searchTerm)}`);
};
return (
<div className="bg-gray-50 min-h-screen flex items-center justify-center">
<div className="max-w-7xl mx-auto px-4 sm:px-6 lg:px-8 text-center">
<h1 className="text-4xl font-extrabold text-gray-900 sm:text-5xl md:text-6xl mb-8">
<span className="block">AI Blockchain Explorer</span>
<span className="block text-indigo-600">Powered by Nebula</span>
</h1>
<p className="mt-3 max-w-md mx-auto text-base text-gray-500 sm:text-lg md:mt-5 md:text-xl md:max-w-3xl mb-8">
Enter any contract address, and get a detailed analysis, functions,
live information, and more.
</p>
<div className="max-w-3xl mx-auto mt-10">
<div className="flex flex-col sm:flex-row gap-4">
<Input
type="text"
placeholder="Enter contract address or transaction hash"
value={searchTerm}
onChange={(e) => setSearchTerm(e.target.value)}
className="flex-grow"
/>
<Select value={selectedChain} onValueChange={setSelectedChain}>
<SelectTrigger className="w-full sm:w-[200px]">
<SelectValue placeholder="Select chain" />
</SelectTrigger>
<SelectContent>
{blockchains.map((chain) => (
<SelectItem key={chain.id} value={chain.id}>
{chain.name}
</SelectItem>
))}
</SelectContent>
</Select>
<Button onClick={handleSearch}>Search</Button>
</div>
</div>
</div>
</div>
);
}
Next, we need to create the explorer page that will display the chat interface for interacting with the contract:
// src/app/components/BlockchainExplorer.tsx
"use client";
import { useState, useEffect } from "react";
import { useSearchParams } from "next/navigation";
import ReactMarkdown from "react-markdown";
import { Send, Search } from "lucide-react";
// Import our Nebula API functions
import { createSession, queryContract, handleUserMessage } from "../../../scripts/Nebula.mjs";
export function BlockchainExplorer() {
const searchParams = useSearchParams();
const chainId = searchParams.get("chainId");
const contractAddress = searchParams.get("searchTerm");
const [sessionId, setSessionId] = useState(null);
const [messages, setMessages] = useState([]);
const [input, setInput] = useState("");
const [isTyping, setIsTyping] = useState(false);
// Initialize the session and query contract details when the component loads
useEffect(() => {
const initSession = async () => {
try {
setIsTyping(true);
const newSessionId = await createSession("Blockchain Explorer Session");
setSessionId(newSessionId);
const contractDetails = await queryContract(
contractAddress,
chainId,
newSessionId
);
setMessages([
{ role: "system", content: "Welcome to the Blockchain Explorer." },
{ role: "system", content: contractDetails },
]);
setIsTyping(false);
} catch (error) {
console.error("Error:", error);
setMessages([
{ role: "system", content: "Failed to load contract details." }
]);
setIsTyping(false);
}
};
initSession();
}, [contractAddress, chainId]);
// Handle user message submission
const handleSend = async () => {
if (!input.trim() || !sessionId) return;
const userMessage = input.trim();
setMessages((prev) => [...prev, { role: "user", content: userMessage }]);
setInput("");
try {
setIsTyping(true);
const response = await handleUserMessage(
userMessage,
sessionId,
chainId,
contractAddress
);
setMessages((prev) => [...prev, { role: "system", content: response }]);
setIsTyping(false);
} catch (error) {
console.error("Error:", error);
setMessages((prev) => [
...prev,
{ role: "system", content: "Failed to process your query." },
]);
setIsTyping(false);
}
};
return (
<div className="flex h-screen bg-gray-100">
<div className="flex flex-col flex-grow p-4">
<div className="flex items-center mb-4">
<Search className="w-6 h-6 text-gray-500 mr-2" />
<h1 className="text-xl font-bold">Blockchain Explorer</h1>
</div>
<div className="flex-grow bg-white rounded-lg shadow-md p-4 mb-4 overflow-y-auto">
{messages.map((message, index) => (
<div
key={index}
className={`mb-2 ${message.role === "user" ? "text-right" : "text-left"}`}
>
{message.role === "system" ? (
<div className="bg-gray-200 text-gray-800 rounded-lg p-2">
<ReactMarkdown>{message.content}</ReactMarkdown>
</div>
) : (
<span className="inline-block bg-blue-500 text-white rounded-lg p-2">
{message.content}
</span>
)}
</div>
))}
{isTyping && (
<div className="text-left mb-2">
<span className="inline-block p-2 rounded-lg bg-gray-200 text-gray-800 animate-pulse">
Typing...
</span>
</div>
)}
</div>
<div className="flex">
<input
type="text"
value={input}
onChange={(e) => setInput(e.target.value)}
placeholder="Ask a question about this contract..."
className="flex-grow p-2 rounded-l-lg border border-gray-300 focus:outline-none"
/>
<button
onClick={handleSend}
className="bg-blue-500 text-white p-2 rounded-r-lg"
>
<Send className="w-6 h-6" />
</button>
</div>
</div>
</div>
);
}
Step 3: Adding Transaction Execution Support
Now for the exciting part! Let's add the ability to execute transactions directly through our chat interface. This requires:
- Adding wallet connectivity
- Updating our Nebula API script with execute functionality
- Enhancing our UI to handle transaction signing
First, let's add the execute function to our Nebula.mjs script:
// Function to execute transaction
async function executeCommand(
message,
signerWalletAddress,
userId = "default-user",
stream = false,
chainId,
contractAddress,
sessionId
) {
const requestBody = {
message,
user_id: userId,
stream,
session_id: sessionId,
execute_config: {
mode: "client", // Only client mode is supported
signer_wallet_address: signerWalletAddress,
},
context_filter: {
chain_ids: [chainId.toString()],
contract_addresses: [contractAddress],
},
};
const response = await apiRequest("/execute", "POST", requestBody);
return response; // Return the full response including message and actions
}
// Add this to the exports
export { executeCommand };
Next, let's update our BlockchainExplorer component to add wallet connectivity and execution capability:
// src/app/components/BlockchainExplorer.tsx
"use client";
import { useState, useEffect } from "react";
import { Search, Send, Terminal } from "lucide-react";
import { useSearchParams } from "next/navigation";
import ReactMarkdown from "react-markdown";
// Import Nebula functions
import {
createSession,
queryContract,
handleUserMessage,
executeCommand,
} from "../../../scripts/Nebula.mjs";
// Import thirdweb components for wallet & transactions
import { useActiveAccount } from "thirdweb/react";
import {
sendAndConfirmTransaction,
prepareTransaction,
defineChain,
} from "thirdweb";
import { client } from "../client";
export function BlockchainExplorer() {
// Same code as before for params, session, messages...
// Get connected wallet
const account = useActiveAccount();
const walletAddress = account?.address;
// Add execution handler function
const handleExecute = async () => {
if (!account?.address || !input.includes("execute")) return;
const executeMessage = input.trim();
setMessages((prev) => [...prev, { role: "user", content: executeMessage }]);
setInput("");
try {
setIsTyping(true);
// Execute the command with Nebula API
const executeResponse = await executeCommand(
executeMessage,
account.address,
"default-user",
false,
chainId,
contractAddress,
sessionId
);
// Check if the response contains actions and a transaction to sign
const action = executeResponse.actions?.find(
(a) => a.type === "sign_transaction"
);
if (action) {
const transactionData = JSON.parse(action.data);
// Prepare the transaction using thirdweb
const preparedTransaction = prepareTransaction({
to: transactionData.to,
value: transactionData.value,
data: transactionData.data,
chain: defineChain(transactionData.chainId),
client,
});
// Send and confirm the transaction
const receipt = await sendAndConfirmTransaction({
transaction: preparedTransaction,
account,
});
// Add the transaction receipt to the chat
setMessages((prev) => [
...prev,
{
role: "system",
content: `Transaction sent successfully! Hash: ${receipt.transactionHash}`,
},
]);
} else {
setMessages((prev) => [
...prev,
{
role: "system",
content: "No transaction to sign in the response.",
},
]);
}
setIsTyping(false);
} catch (error) {
console.error("Error executing transaction:", error);
setMessages((prev) => [
...prev,
{
role: "system",
content: "Failed to execute the command. Please try again.",
},
]);
setIsTyping(false);
}
};
// Then update your UI to include an execute button
return (
<div className="flex h-screen bg-gray-100">
{/* Same UI as before... */}
<div className="flex">
<input
type="text"
value={input}
onChange={(e) => setInput(e.target.value)}
placeholder="Ask a question or type 'execute...' to run a function"
className="flex-grow p-2 rounded-l-lg border border-gray-300 focus:outline-none"
/>
<button
onClick={handleSend}
className="bg-blue-500 text-white p-2 rounded-r-lg"
>
<Send className="w-6 h-6" />
</button>
{input.includes("execute") && (
<button
onClick={handleExecute}
className="ml-2 bg-green-500 text-white p-2 rounded-lg"
>
<Terminal className="w-6 h-6" />
</button>
)}
</div>
</div>
);
}
Finally, let's add wallet connectivity to our app's layout:
// src/app/components/Navigation.tsx
"use client";
import Link from "next/link";
import { ConnectButton } from "thirdweb/react";
import { client } from "../client";
export function Navigation() {
return (
<nav className="bg-white shadow-sm">
<div className="max-w-7xl mx-auto px-4 sm:px-6 lg:px-8">
<div className="flex justify-between h-16">
<div className="flex">
<div className="flex-shrink-0 flex items-center">
<Link href="/" className="text-xl font-bold text-gray-800">
Nebula Chain Explorer
</Link>
</div>
<div className="hidden sm:ml-6 sm:flex sm:space-x-8">
<Link
href="/"
className="border-transparent text-gray-500 hover:border-gray-300 hover:text-gray-700 inline-flex items-center px-1 pt-1 border-b-2 text-sm font-medium"
>
Home
</Link>
</div>
</div>
<ConnectButton client={client} connectModal={{ size: "compact" }} />
</div>
</div>
</nav>
);
}
Using the Blockchain Explorer
Now our AI-powered blockchain explorer is ready to use! Here's how to interact with it:
- Connect your wallet - Click the Connect button in the navigation bar
- Enter a contract address - Paste any EVM contract address on the homepage
- Select a blockchain - Choose the network where the contract is deployed
- Explore the contract - Chat with the AI to learn about the contract's functions
- Execute transactions - Type "execute [function_name]" to run contract functions
For example, if you're looking at a simple number storage contract, you might:
- Ask "What functions does this contract have?"
- Type "What is the current number stored?"
- Execute a function with "execute add to number"
When executing a function, MetaMask (or your wallet) will prompt you to sign the transaction. Once confirmed, you'll see the transaction hash in the chat, indicating the function was executed successfully.
Conclusion
We've built a powerful AI-powered blockchain explorer that makes interacting with smart contracts intuitive and accessible. Using Nebula's AI capabilities, users can now:
- Understand contract functionality through natural language
- Query on-chain data in a conversational way
- Execute transactions directly through chat
This approach dramatically simplifies blockchain interactions and opens up new possibilities for dApp interfaces. The future of blockchain UX is conversational!
To enhance this project further, you might consider:
- Adding transaction history viewing
- Supporting NFT visualization
- Adding multi-wallet support
- Implementing a voice interface
The complete code for this project is available on GitHub. Feel free to fork, modify, and build upon it for your own projects!