Building a Telegram Mini-App Token Deployer with thirdweb and Nebula
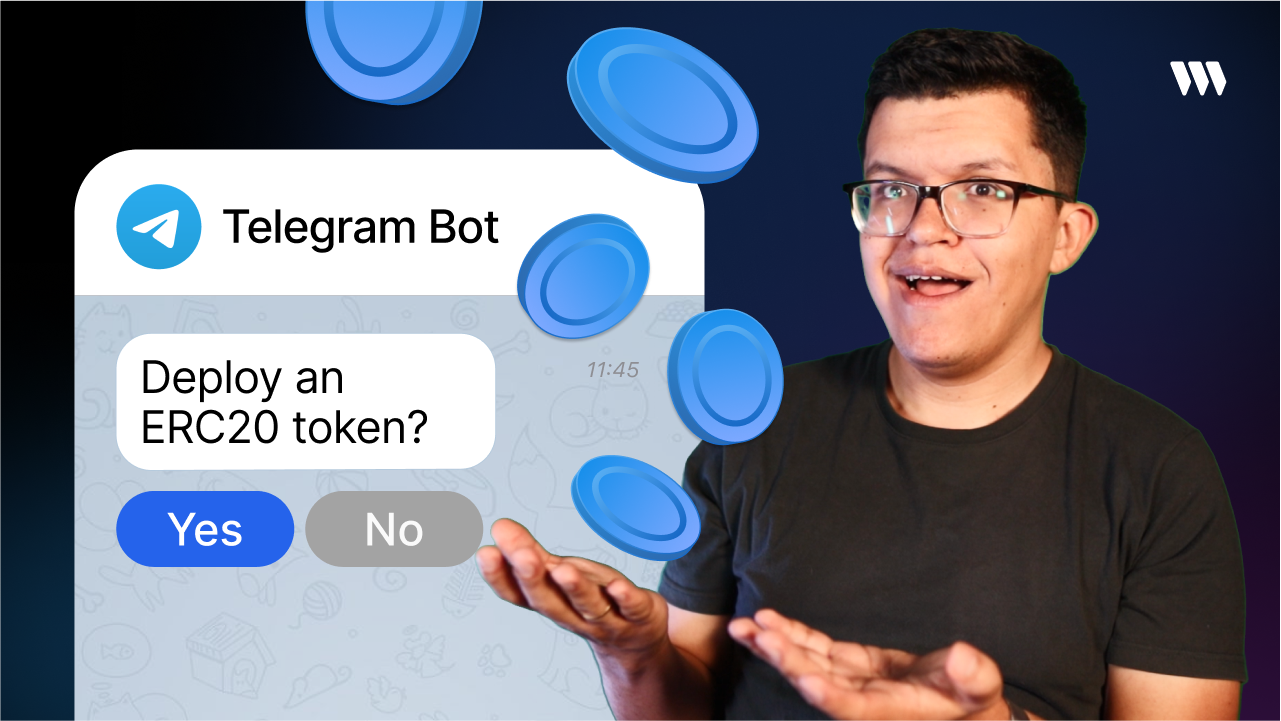
Have you ever wanted to deploy your ERC20 token directly from Telegram without paying gas fees? Today, I'll show you how to build a Telegram Mini-App that lets users deploy ERC20 tokens without the complexity of handling gas fees, keys, or smart contract coding.
In crypto, innovations that simplify complex operations are game-changers. This tutorial combines the powerful capabilities of Thirdweb's account abstraction and Nebula AI to create a seamless token deployment experience within Telegram's mini-app ecosystem.
Follow along with this video:
What We'll Build
We'll create a complete solution with:
- A Telegram bot that interacts with users
- A mini-app interface for token creation
- Account abstraction to handle gas fees
- Integration with Nebula AI for blockchain interactions
Check the entire project on our Github Repo.
Let's dive into the implementation!
Setting Up the Telegram Bot
First, let's create a simple Telegram bot that will act as our entry point. The process is straightforward:
- Search for "BotFather" in Telegram
- Type
/newbot
and follow the instructions - Save the API token for later use
Here's the basic bot code using the Telegraf library:
const { Telegraf, Markup } = require("telegraf");
const botToken = "YOUR_BOT_TOKEN";
const miniAppUrl = "YOUR_MINI_APP_URL";
const API_BASE_URL = "https://nebula-api.thirdweb.com";
const SECRET_KEY = "YOUR_THIRDWEB_SECRET_KEY";
const bot = new Telegraf(botToken);
// Welcome message with button to open mini-app
bot.start((ctx) => {
ctx.reply(
"Welcome! This bot will help you deploy an ERC20 token.\n\n" +
"Click the button below to start the process:",
Markup.inlineKeyboard([
Markup.button.callback("Deploy ERC20 Token", "start_deployment"),
])
);
});
// When user clicks "Deploy Token" button
bot.action("start_deployment", (ctx) => {
ctx.reply(
"Great! Click the link below to open the Telegram Mini-App, then click Next to continue:",
Markup.inlineKeyboard([
Markup.button.url("Open Mini-App", miniAppUrl),
Markup.button.callback("Next", "ask_contract_address"),
])
);
});
// When user clicks "Next"
bot.action("ask_contract_address", (ctx) => {
ctx.reply("Please enter the deployed contract address:");
});
// Handle contract address input and query Nebula
bot.on("text", async (ctx) => {
// Logic to handle text messages will go here
// We'll implement this later with Nebula integration
});
bot.launch();
console.log("Bot is running...");
Creating the Mini-App Interface
Now let's build the actual mini-app interface where users will input their token details.
First, we need to set up a React project:
bun create vite token-deployer-mini-app --template react-ts
cd token-deployer-mini-app
bun install
In our index.html
, we need to add the Telegram Web App script:
<script src="https://telegram.org/js/telegram-web-app.js"></script>
The core of our mini-app is the form where users will enter token details. Here's a simplified version of our React component:
import { useState } from "react";
import { ConnectButton, useActiveAccount } from "thirdweb/react";
import { client } from "./client.ts";
import { sepolia } from "thirdweb/chains";
import { Nebula } from "thirdweb/ai";
import { inAppWallet } from "thirdweb/wallets";
function App() {
const activeAccount = useActiveAccount();
const [tokenName, setTokenName] = useState("");
const [tokenSymbol, setTokenSymbol] = useState("");
const [selectedChain, setSelectedChain] = useState("");
const [modalOpen, setModalOpen] = useState(false);
const [transactionStatus, setTransactionStatus] = useState("");
const [transactionHash, setTransactionHash] = useState("");
const [explorerUrl, setExplorerUrl] = useState("");
const handleSubmit = async (e: React.FormEvent) => {
e.preventDefault();
if (!activeAccount?.address) return;
setModalOpen(true);
setTransactionStatus("in-progress");
const executeMessage = `Deploy a new ERC20 token with name ${tokenName} and symbol ${tokenSymbol} on chain ${selectedChain}`;
try {
const result = await Nebula.execute({
client: client,
account: activeAccount,
message: executeMessage,
contextFilter: {
chains: [sepolia],
},
});
setTransactionHash(result.transactionHash);
setExplorerUrl(`${result.chain.blockExplorers[0].url}/tx/${result.transactionHash}`);
setTransactionStatus("submitted");
} catch (error) {
console.error("Transaction failed", error);
setTransactionStatus("failed");
}
};
return (
<div className="min-h-screen flex items-center justify-center bg-black p-4">
{activeAccount ? (
<form onSubmit={handleSubmit} className="space-y-6">
{/* Form input fields for token name, symbol, chain */}
<button
type="submit"
className="w-full px-6 py-3 bg-purple-600 text-white rounded-lg"
>
Deploy
</button>
</form>
) : (
<ConnectButton
client={client}
accountAbstraction={{
chain: sepolia,
sponsorGas: true,
}}
wallets={[
inAppWallet({
auth: {
options: ["email"],
},
}),
]}
/>
)}
{/* Transaction modal */}
</div>
);
}
Implementing Account Abstraction
One of the most powerful features of our app is the ability to sponsor gas fees. This means users don't need to have ETH in their wallets to deploy tokens. We accomplish this through Thirdweb's account abstraction:
// client.ts
import { createThirdwebClient } from "thirdweb";
const clientId = "YOUR_THIRDWEB_CLIENT_ID";
const secretKey = "YOUR_THIRDWEB_SECRET_KEY";
export const client = createThirdwebClient({
clientId: clientId,
secretKey: secretKey,
});
The magic happens in our ConnectButton
component where we set sponsorGas: true
:
<ConnectButton
client={client}
accountAbstraction={{
chain: sepolia,
sponsorGas: true,
}}
wallets={[
inAppWallet({
auth: {
options: ["email"],
},
}),
]}
/>
With this configuration:
- Users can sign in with just their email
- A smart account is created for them behind the scenes
- All gas fees are covered by your application
Integrating Nebula AI
Nebula AI is Thirdweb's powerful AI assistant that handles blockchain interactions. We'll use it to:
- Deploy the ERC20 token
- Retrieve token information
- Answer user questions about the token
Here's how we use Nebula to deploy the token:
const result = await Nebula.execute({
client: client,
account: activeAccount,
message: executeMessage,
contextFilter: {
chains: [sepolia],
},
});
The execute message is a natural language instruction: "Deploy a new ERC20 token with name X and symbol Y on chain Z." Nebula understands this request and executes the appropriate blockchain operations.
In our Telegram bot, we implement the ability to query contract details:
async function askNebula(sessionId, chainId, contractAddress) {
const message = `Give me all the details of this contract ${contractAddress} on chain ${chainId}`;
const requestBody = {
message,
session_id: sessionId,
context_filter: {
chain_ids: [chainId.toString()],
contractAddress: [contractAddress],
},
};
const response = await apiRequest("/chat", "POST", requestBody);
return response.message;
}
Connecting Everything Together
Now we need to connect all the pieces. When a user deploys a token in the mini-app, they'll return to the Telegram bot and paste the contract address. The bot will use Nebula to retrieve information about the token.
Here's the complete flow:
- User starts bot and opens mini-app
- User connects with email and fills out token details
- Nebula deploys the token (gas fees sponsored)
- User gets transaction hash and block explorer link
- User returns to bot and shares contract address
- Bot uses Nebula to retrieve and display token details
- User can ask additional questions about their token
Deployment and Testing
To deploy your mini-app, you'll need:
- A hosting service for your mini-app (Vercel, Netlify, etc.)
- A server to run your Telegram bot (or use a serverless function)
- Thirdweb API keys
- Nebula API access
Test your application thoroughly, making sure:
- Users can connect with just email
- Token deployment works across different chains
- Transaction status is properly communicated
- Contract details are correctly retrieved
Conclusion
By combining Telegram mini-apps, Thirdweb's account abstraction, and Nebula AI, we've created a powerful yet simple token deployment solution. This approach removes multiple barriers to entry:
- No need for users to understand smart contracts
- No gas fees to worry about
- No complex wallet setup required
The potential applications go far beyond token deployment. You could extend this pattern to NFT minting, DAO creation, or any other smart contract interaction.
Challenge
Try extending this project by adding the ability to execute token functions directly within the Telegram bot. For example, implement minting or transfer functions that users can call through text commands.
Hint: Check the Thirdweb API documentation for the execute
function, which can be integrated into your bot's text handler. You'll need to use account abstraction to sponsor these transactions as well.
Happy building!