Building a LINE Mini App with Web3 Integration: Rock Paper Scissors Game
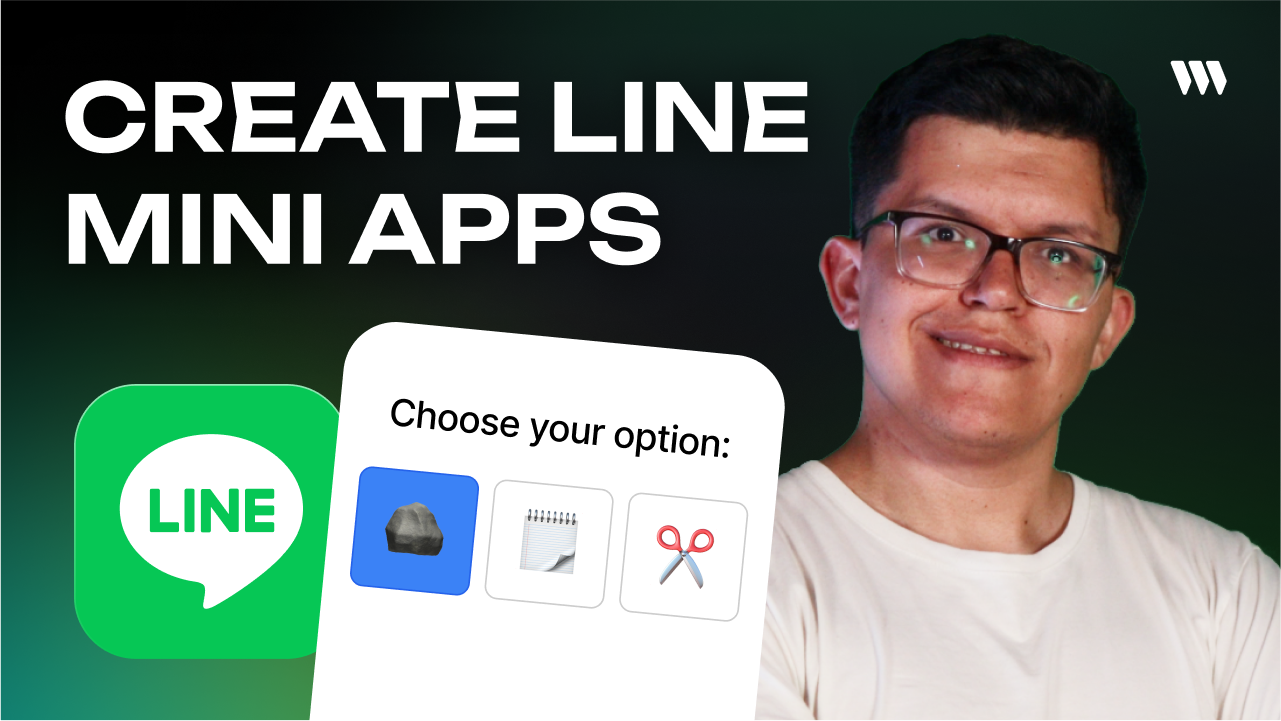
Ever wondered how to integrate blockchain features directly into a LINE messaging app? In this tutorial, I'll walk you through creating a Rock Paper Scissors game as a LINE Mini App with web3 integration, complete with gasless transactions and token rewards.
Users can connect via a simple email login, play the game against the computer, and win tokens - all without needing any crypto knowledge or gas funds.
Follow along with this video:
What are LINE Mini Apps?
LINE Mini Apps are lightweight applications that run directly inside the LINE messaging app. With over 196 million users, LINE Mini Apps provide an excellent opportunity to build blockchain-powered experiences for a massive audience.
What We'll Build
Today, we'll create a simple but complete Rock Paper Scissors game that:
- Runs directly in the LINE messaging app
- Lets users log in via email (no crypto wallet needed)
- Awards tokens when players win
- Uses gasless transactions (users don't pay gas fees)
Here's the GitHub repo for reference: LINE-Mini-App
Prerequisites
Before we start, you'll need:
- A LINE Developer Account
- A thirdweb client ID
- Basic React knowledge
Step 1: Create a LINE Developer Account
First, we need to set up a LINE Business ID and create a provider:
- Create a LINE Business ID account
- Log in to the LINE Developer Console
- Click "Create" in the providers section
- Name your provider (e.g., "Rock Paper Scissors Provider")
Step 2: Create a LINE Mini App Channel
Next, we'll create a channel for our Mini App:
- Click "Create a LINE Mini App Channel"
- Select your region (we'll use Japan in this example)
- Upload a logo for your app
- Fill in the channel name and description
- Add your contact email
- Accept the terms and conditions
Once created, you need to make note of two important URLs:
- LIFF URL: This is what identifies your Mini App (note the LIFF ID at the end)
- Endpoint URL: This is where your web app will be hosted
Step 3: Set Up the Next.js Project
Let's create our project using thirdweb's template:
npx thirdweb create
Choose "app" as the project type, name it "rock-paper-scissors", and select "Next.js" as the framework.
Next, install the LINE LIFF package:
yarn add @line/liff
Step 4: Configure the Web App
First, we need to set up our environment variables. Create a .env
file based on the .env.example
:
NEXT_PUBLIC_TEMPLATE_CLIENT_ID=your-thirdweb-client-id
NEXT_PUBLIC_TEMPLATE_LINE_LIFF=your-liff-id
NEXT_PUBLIC_TOKEN_CONTRACT=your-token-contract-address
Configuring the Layout
We need to initialize the LINE LIFF client in our layout component. Here's how to modify src/app/layout.tsx
:
"use client";
import type { Metadata } from 'next';
import { Inter } from 'next/font/google';
import './globals.css';
import { ThirdwebProvider } from 'thirdweb/react';
import liff from '@line/liff';
import { useEffect, useState } from 'react';
const inter = Inter({ subsets: ['latin'] });
export default function RootLayout({
children,
}: Readonly<{
children: React.ReactNode;
}>) {
const [isLiffReady, setIsLiffReady] = useState(false);
const liffId = process.env.NEXT_PUBLIC_TEMPLATE_LINE_LIFF!;
useEffect(() => {
if (typeof window !== 'undefined') {
const initializeLiff = async () => {
try {
await liff.init({ liffId: liffId });
setIsLiffReady(true);
} catch (error) {
console.error('LIFF initialization failed', error);
}
};
initializeLiff();
}
}, [liffId]);
return (
<html lang="en">
<body className={inter.className}>
{isLiffReady ? (
<ThirdwebProvider>{children}</ThirdwebProvider>
) : (
<div>Loading LIFF...</div>
)}
</body>
</html>
);
}
This code does a few important things:
- Marks the component as a client component with
use client
- Sets up a state to track if LIFF is ready
- Initializes the LIFF client with our LIFF ID
- Only renders our app when LIFF is ready
Setting Up the Main Page
Let's simplify our page.tsx
to just render our game component:
import RockPaperScissors from "./components/RockPaperScissors";
export default function Home() {
return (
<main className="p-4 pb-10 min-h-[100vh] flex items-center justify-center container max-w-screen-lg mx-auto">
<div className="py-20">
<RockPaperScissors />
</div>
</main>
);
}
Step 5: Create the Token Contract
Before building our game component, we need to create a token contract to reward players:
- Go to the thirdweb dashboard
- Create a new project called "rock-paper-scissors"
- Click on "Contracts" then "Deploy Contract"
- Choose the "Token Drop (ERC20)" contract
- Name it "Rock Paper Scissors Points"
- Deploy it on a testnet like Kaiyo testnet (or BaseSepolia in our example)
- Set up claim conditions to allow unlimited claims
Make sure to copy the contract address and add it to your .env
file.
Step 6: Build the Game Component
Now for the fun part - creating our Rock Paper Scissors game. Create a file at src/app/components/RockPaperScissors.tsx
:
The game component includes:
- Logic for determining the winner
- Connect button for wallet login
- Game UI for selecting rock, paper, or scissors
- Prize claiming functionality
Key parts of the code include:
Setting up the Web3 Connection
<ConnectButton
client={client}
accountAbstraction={{
chain: baseSepolia,
sponsorGas: true,
}}
wallets={[
inAppWallet({
auth: {
options: ["email"],
},
}),
]}
/>
This creates a connect button that:
- Uses account abstraction for gasless transactions
- Only allows email login for simplicity
Claiming Tokens When Players Win
<TransactionButton
transaction={() =>
claimTo({
contract: contract,
to: account.address,
quantity: "10",
})
}
onTransactionConfirmed={() => {
alert("Prize claimed!");
setShowModal(false);
setPrizeClaimed(true);
}}
>
Claim Prize
</TransactionButton>
This button:
- Calls the
claimTo
function on our token contract - Sends 10 tokens to the player's wallet
- Shows a confirmation when the transaction completes
Step 7: Testing the App Locally
To test your app locally with LINE integration:
- Start your development server:
yarn dev
- Use ngrok to create a public URL for your local server:
ngrok http 3000
- Copy the ngrok URL and update the "Endpoint URL" in your LINE Developer Console
- Add yourself as a tester in the LINE Developer Console:
- Go to the "Roles" tab
- Click "Invite"
- Enter your LINE account email and set role as "Tester"
- In the LINE app, create a new group chat
- Paste your LIFF URL into the chat
- Click on it to open your Mini App
The User Experience
When users open your Mini App:
- They'll see the "Loading LIFF..." message while the app initializes
- Once loaded, they'll see the Connect button
- After connecting with their email, they can play Rock Paper Scissors
- When they win, they can claim 10 tokens as a prize
- The transaction happens without them needing any funds
- They can see their token balance and play again
Conclusion
By combining LINE Mini Apps with thirdweb's tools, we've created a user-friendly blockchain game that doesn't require any crypto knowledge. The game demonstrates several powerful concepts:
- Embedding web3 functionality in a mainstream messenger app
- Using email login instead of crypto wallets
- Implementing gasless transactions with account abstraction
- Rewarding users with tokens for in-game actions
This approach dramatically lowers the barrier to entry for blockchain applications, making them accessible to millions of users who may have never interacted with crypto before.
You can now customize this game further, create more complex reward systems, or build entirely different Mini Apps using the same principles.
What will you build next?